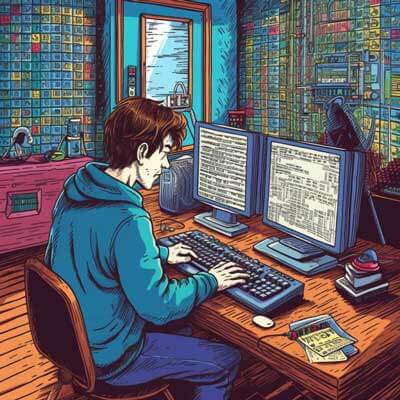
- Introduction to If Else in Shell Scripts
- Example: Checking if a File Exists
- Example: Determining if a Number is Positive or Negative
- Condition Evaluation
- Example: String Comparison
- Example: Numeric Comparison
- Executing Commands Conditionally with If Else
- Conditional Execution
- Conditional Command Execution
- Using Parameters and Variables with If Else
- Using Parameters
- Using Variables
- Case Study 1: File Operations with If Else
- Checking if a File Exists
- Reading Data from a File
- Case Study 2: User Input Validation with If Else
- Validating Numeric Input
- Best Practice 1: Proper Formatting and Indentation
- Consistent Indentation
- Whitespace and Line Breaks
- Commenting
- Best Practice 2: Handling Edge Cases
- Default Actions
- Error Handling
- Input Validation
- Best Practice 3: Avoiding Common Mistakes
- Missing Syntax
- Incorrect Syntax
- Neglecting Quotes
- Incorrect Operators or Conditions
- Real World Example 1: System Monitoring Script
- Scenario
- Solution
- Real World Example 2: User Management Script
- Scenario
- Solution
- Performance Consideration 1: Efficiency of Condition Checks
- Order of Conditions
- Short-Circuit Evaluation
- Numeric Evaluations
- Performance Consideration 2: Impact of Nested If Else Statements
- Code Readability and Maintainability
- Performance Impact
- Testing and Profiling
- Advanced Technique 1: Using If Else with Loops
- While Loop
- For Loop
- Advanced Technique 2: Combining If Else with Functions
- Function with If Else Statement
- Function Returning a Value
- Code Snippet Idea 1: Basic If Else Statement
- Code Snippet Idea 2: If Else with Multiple Conditions
- Code Snippet Idea 3: Nested If Else Statement
- Code Snippet Idea 4: If Else with Logical Operators
- Code Snippet Idea 5: If Else with Regex Matching
- Handling Errors and Exceptions in Shell Scripts
- Exit Codes
- Error Output Redirection
- Error Handling Functions
- Troubleshooting Common Errors with If Else
- Syntax Errors
- Variable Scope
- Condition Evaluation
- Command Execution
- Testing and Debugging If Else Statements
- Manual Testing
- Logging and Debug Output
- Debugging Tools
Introduction to If Else in Shell Scripts
If Else statements are a fundamental part of shell scripting, allowing you to execute different code blocks based on certain conditions. With If Else statements, you can create branching logic in your scripts, making them more flexible and powerful.
Here is a basic syntax example of an If Else statement in shell scripts:
if [ condition ]; then # Code to execute if the condition is true else # Code to execute if the condition is false fi
In this example, the “condition” represents a logical test that evaluates to either true or false. If the condition is true, the code block following the “then” keyword is executed. Otherwise, the code block following the “else” keyword is executed. The “fi” keyword is used to mark the end of the If Else statement.
Let’s look at a practical example to better understand how If Else statements work.
Related Article: How To Echo a Newline In Bash
Example: Checking if a File Exists
Suppose you want to check if a file exists in a specific directory and perform different actions based on the result. You can use the following script:
#!/bin/bash filename="example.txt" if [ -f "$filename" ]; then echo "The file $filename exists." else echo "The file $filename does not exist." fi
In this example, the script assigns the name of the file to the variable “filename. The If Else statement checks if the file exists using the “-f” flag with the file’s path. If the file exists, it displays a message stating so. Otherwise, it displays a message indicating that the file does not exist.
Example: Determining if a Number is Positive or Negative
Another common use case for If Else statements is to determine the sign of a number. Consider the following script:
#!/bin/bash number=-10 if ((number > 0)); then echo "The number is positive." elif ((number " operator. If the condition is true, it displays a message indicating that the number is positive. If the condition is false, it checks if the number is less than 0 using the "<" operator. If this condition is true, it displays a message indicating that the number is negative. If both conditions are false, it displays a message indicating that the number is zero. Using If Else statements in shell scripts allows you to perform different actions based on conditions, making your scripts more dynamic and versatile. <h2>Syntax and Structure of If Else Statements</h2> <h3>Basic Syntax</h3> The basic syntax of an If Else statement in shell scripting is as follows: ```bash if [ condition ]; then # Code to execute if the condition is true else # Code to execute if the condition is false fi
In this syntax, the “condition” represents a logical test that evaluates to either true or false. If the condition is true, the code block following the “then” keyword is executed. Otherwise, the code block following the “else” keyword is executed. The “fi” keyword marks the end of the If Else statement.
Condition Evaluation
The condition in an If Else statement can be any valid expression that evaluates to true or false. Here are some common types of conditions:
– String comparisons using operators such as “==”, “!=”, “”, etc.
– Numeric comparisons using operators such as “-eq”, “-ne”, “-lt”, “-gt”, etc.
– File checks using operators such as “-f”, “-d”, “-r”, “-w”, etc.
– Logical operations using operators such as “&&” (AND), “||” (OR), “!” (NOT), etc.
Let’s look at some examples to understand the syntax and structure of If Else statements in more detail.
Related Article: Executing a Bash Script with Multivariables in Linux
Example: String Comparison
Suppose you want to check if a user input matches a specific string. You can use the following script:
#!/bin/bash read -p "Enter your name: " name if [ "$name" == "John" ]; then echo "Welcome, John!" else echo "Access denied." fi
In this example, the script prompts the user to enter their name using the “read” command and stores it in the variable “name”. The If Else statement compares the value of the “name” variable with the string “John” using the “==” operator. If the condition is true, it displays a welcome message. Otherwise, it displays an “Access denied” message.
Example: Numeric Comparison
Suppose you want to determine whether a number is even or odd. You can use the following script:
#!/bin/bash read -p "Enter a number: " number if ((number % 2 == 0)); then echo "The number is even." else echo "The number is odd." fi
In this example, the script prompts the user to enter a number and stores it in the variable “number”. The If Else statement checks if the number is divisible by 2 (i.e., if the remainder of the division is 0) using the “%” operator. If the condition is true, it displays a message indicating that the number is even. Otherwise, it displays a message indicating that the number is odd.
Understanding the syntax and structure of If Else statements is crucial for writing effective shell scripts. It enables you to execute different code blocks based on specific conditions, making your scripts more versatile and powerful.
Executing Commands Conditionally with If Else
Related Article: How to Manipulate Quotes & Strings in Bash Scripts
Conditional Execution
In shell scripting, you can execute commands conditionally using If Else statements. This allows you to control the flow of your script and perform different actions based on specific conditions. By executing commands conditionally, you can make your scripts more dynamic and responsive.
Here is an example that demonstrates how to execute commands conditionally in shell scripts:
#!/bin/bash read -p "Enter your age: " age if [ "$age" -ge 18 ]; then echo "You are eligible to vote." echo "Please cast your vote." else echo "You are not eligible to vote yet." echo "Please wait until you turn 18." fi
In this example, the script prompts the user to enter their age and stores it in the variable “age”. The If Else statement checks if the age is greater than or equal to 18 using the “-ge” operator. If the condition is true, it displays a message indicating that the user is eligible to vote and prompts them to cast their vote. Otherwise, it displays a message indicating that the user is not yet eligible to vote and advises them to wait until they turn 18.
Conditional Command Execution
In addition to executing different commands based on conditions, you can also conditionally execute specific commands within the If Else statement itself. This allows you to perform more complex tasks based on specific conditions.
Consider the following example:
#!/bin/bash filename="example.txt" if [ -f "$filename" ]; then echo "The file $filename exists." echo "The file contents are:" cat "$filename" else echo "The file $filename does not exist." echo "Creating a new file..." touch "$filename" echo "File created." fi
In this example, the script checks if a file with the name specified in the variable “filename” exists using the “-f” flag. If the file exists, it displays a message indicating so and proceeds to display its contents using the “cat” command. If the file does not exist, it displays a message indicating that the file does not exist and creates a new file using the “touch” command.
By conditionally executing commands in your shell scripts, you can create more dynamic and responsive scripts that adapt to different scenarios.
Using Parameters and Variables with If Else
Related Article: How to Import JSON from a Bash Script on Linux
Using Parameters
In shell scripting, you can use parameters to pass values to your scripts from the command line. This allows you to make your scripts more flexible and reusable. You can also use parameters with If Else statements to conditionally execute commands based on user input or other external factors.
Here is an example that demonstrates how to use parameters with If Else statements:
#!/bin/bash age="$1" if [ "$age" -ge 18 ]; then echo "You are eligible to vote." echo "Please cast your vote." else echo "You are not eligible to vote yet." echo "Please wait until you turn 18." fi
In this example, the script accepts an age parameter from the command line using the “$1” syntax. The If Else statement checks if the age is greater than or equal to 18 using the “-ge” operator. If the condition is true, it displays a message indicating that the user is eligible to vote and prompts them to cast their vote. Otherwise, it displays a message indicating that the user is not yet eligible to vote and advises them to wait until they turn 18.
You can run this script with the age parameter as follows:
./script.sh 20
This would output:
You are eligible to vote. Please cast your vote.
Using Variables
In addition to parameters, you can also use variables within your shell scripts to store and manipulate data. Variables allow you to store values temporarily and refer to them later in your script.
Consider the following example:
#!/bin/bash filename="example.txt" if [ -f "$filename" ]; then echo "The file $filename exists." echo "The file contents are:" cat "$filename" else echo "The file $filename does not exist." echo "Creating a new file..." touch "$filename" echo "File created." fi
In this example, the script uses the variable “filename” to store the name of the file. The If Else statement checks if a file with the specified name exists using the “-f” flag. If the file exists, it displays a message indicating so and proceeds to display its contents using the “cat” command. If the file does not exist, it displays a message indicating that the file does not exist and creates a new file using the “touch” command.
By utilizing parameters and variables in your shell scripts, you can create more flexible and customizable If Else statements that adapt to different inputs and conditions.
Case Study 1: File Operations with If Else
Related Article: Appending Dates to Filenames in Bash Scripts
Checking if a File Exists
One common use case for If Else statements in shell scripting is performing file operations based on file existence. You can use If Else statements to check if a file exists before performing specific actions, such as reading or writing data.
Consider the following example:
#!/bin/bash filename="example.txt" if [ -f "$filename" ]; then echo "The file $filename exists." else echo "The file $filename does not exist." fi
In this example, the script checks if a file with the name specified in the variable “filename” exists using the “-f” flag. If the file exists, it displays a message indicating so. Otherwise, it displays a message indicating that the file does not exist.
Reading Data from a File
Another file operation that can be performed conditionally using If Else statements is reading data from a file. You can use If Else statements to check if a file exists and, if it does, read its contents.
Consider the following example:
#!/bin/bash filename="example.txt" if [ -f "$filename" ]; then echo "The file $filename exists." echo "The file contents are:" cat "$filename" else echo "The file $filename does not exist." fi
In this example, the script checks if a file with the name specified in the variable “filename” exists using the “-f” flag. If the file exists, it displays a message indicating so and proceeds to display its contents using the “cat” command. If the file does not exist, it displays a message indicating that the file does not exist.
By using If Else statements, you can conditionally perform file operations based on the existence or non-existence of files, making your scripts more robust and adaptable.
Case Study 2: User Input Validation with If Else
Related Article: Making Bash Scripts Executable with Chmod in Linux
Validating Numeric Input
If Else statements can be used to validate user input and ensure that it meets specific criteria. This is particularly useful when working with numeric input, where you may want to check if the input is within a certain range or if it fulfills other conditions.
Consider the following example:
#!/bin/bash read -p "Enter a number between 1 and 10: " number if ((number >= 1 && number = 1 && <= 10" condition. If the number meets the criteria, it displays a message indicating valid input. Otherwise, it displays a message indicating invalid input and prompts the user to enter a number between 1 and 10. <h3>Validating String Input</h3> You can also use If Else statements to validate string input provided by the user. This allows you to check if the input matches a specific pattern, length, or any other condition you define. Consider the following example: ```bash #!/bin/bash read -p "Enter your name: " name if [[ "$name" =~ ^[A-Za-z]+$ ]]; then echo "Valid input." else echo "Invalid input. Please enter a valid name." fi
In this example, the script prompts the user to enter their name using the “read” command and stores it in the variable “name”. The If Else statement uses a regular expression pattern to check if the name consists only of alphabetic characters. If the input matches the pattern, it displays a message indicating valid input. Otherwise, it displays a message indicating invalid input and prompts the user to enter a valid name.
By using If Else statements to validate user input, you can ensure that your scripts only process valid and expected data, improving the reliability and security of your programs.
Best Practice 1: Proper Formatting and Indentation
When writing If Else statements in shell scripts, it is crucial to follow proper formatting and indentation practices. This enhances code readability and makes it easier for others (including your future self) to understand and maintain the script.
Here are some best practices to consider:
Consistent Indentation
It is essential to use consistent indentation throughout your script, including within If Else statements. This helps visually distinguish different code blocks and improves code readability.
if [ condition ]; then # Code block for the "if" branch else # Code block for the "else" branch fi
In the example above, both the code block for the “if” branch and the code block for the “else” branch are indented with four spaces. This indentation style is commonly used in shell scripting, but you can choose any consistent indentation style that works for you.
Related Article: Formatting and Displaying Dates with Bash Scripts in Linux
Whitespace and Line Breaks
Using whitespace and line breaks appropriately also contributes to code readability. Here are some guidelines:
– Leave spaces around square brackets in condition statements for better readability. For example: [ "$name" == "John" ]
instead of ["$name"=="John"]
.
– Use line breaks to separate different parts of the If Else statement, such as the condition, the “then” keyword, and the code blocks. This makes it easier to scan and understand the structure of the statement.
if [ condition ] then # Code block for the "if" branch else # Code block for the "else" branch fi
– Avoid excessive line breaks within code blocks, as they can make the code harder to read. Stick to a consistent and minimal use of line breaks.
Commenting
Adding comments to your code is essential for documentation and future reference. Include comments to explain the purpose and logic behind your If Else statements. This is particularly useful when the conditions or code blocks are complex or non-obvious.
# Check if the file exists if [ -f "$filename" ]; then # Display the file contents echo "The file $filename exists." cat "$filename" else # Create a new file echo "The file $filename does not exist." touch "$filename" fi
Best Practice 2: Handling Edge Cases
When writing If Else statements in shell scripts, it is essential to consider and handle edge cases. Edge cases are scenarios that fall outside the normal range of expected inputs or conditions. By accounting for edge cases, you can make your scripts more robust and handle unexpected situations gracefully.
Here are some best practices for handling edge cases in If Else statements:
Related Article: Locating and Moving Files in Bash Scripting on Linux
Default Actions
Consider including default actions within your If Else statements to handle unexpected conditions or inputs. By providing a fallback action, you can ensure that your script does not crash or produce undesired results when faced with unexpected scenarios.
if [ condition ]; then # Code block for the "if" branch else # Code block for the "else" branch fi
In the example above, you can add a default action within the “else” branch to handle cases that do not match the expected conditions. This default action can be a generic error message, logging, or any other appropriate response.
Error Handling
Consider incorporating error handling mechanisms within your If Else statements to capture and handle potential errors gracefully. This can include checking return codes, using conditional statements to handle specific error conditions, or displaying informative error messages.
if [ condition ]; then # Code block for the "if" branch else # Code block for the "else" branch fi
Within the code blocks, you can include error handling logic using tools like conditional statements, error codes, or error messages. By anticipating and handling potential errors, you can improve the reliability of your scripts.
Input Validation
Validate inputs within your If Else statements to ensure that they meet the expected criteria or format. By validating inputs, you can prevent unexpected behavior or security vulnerabilities caused by malicious or incorrect data.
if [ condition ]; then # Code block for the "if" branch else # Code block for the "else" branch fi
Within the code blocks, you can include input validation logic using conditional statements, regular expressions, or other techniques. This validation step helps to filter out invalid or unexpected inputs, reducing the chances of errors or unwanted behavior.
By considering and handling edge cases within your If Else statements, you can create more robust and reliable shell scripts that gracefully handle unexpected scenarios and inputs.
Related Article: How to Make a Bash Script Continue to Run After an Error
Best Practice 3: Avoiding Common Mistakes
When working with If Else statements in shell scripts, it is crucial to be aware of common mistakes and pitfalls. By avoiding these mistakes, you can write more efficient and error-free scripts. Here are some common mistakes to avoid:
Missing Syntax
Ensure that you include the necessary syntax elements when writing If Else statements. Missing keywords, brackets, or operators can lead to syntax errors and script failures.
if [ condition ]; then # Code block for the "if" branch else # Code block for the "else" branch fi
In the example above, make sure to include the “if”, “then”, “else”, and “fi” keywords, as well as the square brackets for the condition.
Incorrect Syntax
Pay attention to the correct syntax and usage of operators, brackets, and other elements within your If Else statements. Incorrect syntax can result in unexpected behavior or errors.
if [ "$name" == "John" ]; then # Code block for the "if" branch else # Code block for the "else" branch fi
In the example above, ensure that the equality operator “==” is used correctly and that the condition is enclosed in double quotes.
Related Article: How to Check the Success of a Bash Script
Neglecting Quotes
When working with variables or strings in If Else statements, ensure that you properly enclose them in quotes. Neglecting quotes can lead to unexpected results, especially when handling whitespace or special characters.
if [ "$name" == "John" ]; then # Code block for the "if" branch else # Code block for the "else" branch fi
In the example above, make sure to enclose the variable “$name” and the string “John” in double quotes to handle potential whitespace or special characters in the input.
Incorrect Operators or Conditions
Double-check the operators and conditions used within your If Else statements to ensure they match your intended logic. Incorrect operators or conditions can lead to incorrect results or unexpected behavior.
if [ -f "$filename" ]; then # Code block for the "if" branch else # Code block for the "else" branch fi
In the example above, ensure that the correct file check operator “-f” is used and that the condition matches your intended logic.
By being aware of these common mistakes and paying attention to syntax and usage, you can avoid errors and write more reliable If Else statements in your shell scripts.
Real World Example 1: System Monitoring Script
Related Article: How to Extract Numbers from Strings in Bash
Scenario
Suppose you need to write a shell script to monitor the CPU usage of a Linux system. You want the script to perform different actions based on the CPU usage percentage.
Solution
#!/bin/bash cpu_usage=$(top -bn1 | grep "Cpu(s)" | awk '{print $2 + $4}') if ((cpu_usage > 80)); then echo "CPU usage is high!" echo "Sending an email to the administrator..." echo "Subject: High CPU Usage" | mail -s "High CPU Usage" admin@example.com else echo "CPU usage is normal." fi
In this example, the script uses the “top” command to retrieve the CPU usage percentage of the system. It extracts the percentage value using “grep” and “awk” commands and stores it in the “cpu_usage” variable.
The If Else statement checks if the CPU usage percentage is greater than 80 using the “>” operator. If the condition is true, it displays a message indicating high CPU usage and sends an email to the system administrator using the “mail” command. Otherwise, it displays a message indicating normal CPU usage.
This script provides a simple example of how If Else statements can be used to handle real-world scenarios, such as system monitoring and alerting.
Real World Example 2: User Management Script
Related Article: How to Read Text Files in Linux with Bash Scripts
Scenario
Suppose you are tasked with writing a shell script to manage user accounts on a Linux system. The script should allow the administrator to create, delete, or modify user accounts based on user input.
Solution
#!/bin/bash read -p "Enter the username: " username if id "$username" >/dev/null 2>&1; then echo "User $username already exists." read -p "Do you want to delete the user? (y/n): " delete_choice if [ "$delete_choice" == "y" ]; then sudo userdel "$username" echo "User $username deleted." else echo "No action taken." fi else read -p "Enter the password for $username: " password sudo useradd -m -p "$password" "$username" echo "User $username created." fi
In this example, the script prompts the administrator to enter a username. It then checks if the user already exists using the “id” command. If the user exists, it displays a message indicating that the user already exists and prompts the administrator to confirm if they want to delete the user.
If the administrator chooses to delete the user, the script uses the “userdel” command with the “sudo” prefix to delete the user. Otherwise, it displays a message indicating that no action was taken.
If the user does not exist, the script prompts the administrator to enter a password for the new user and uses the “useradd” command with appropriate options and the “sudo” prefix to create the user.
This script demonstrates how If Else statements can be used to manage user accounts based on user input, providing a practical example of real-world shell scripting.
Performance Consideration 1: Efficiency of Condition Checks
When writing If Else statements in shell scripts, it is important to consider the efficiency of condition checks. Efficient condition checks can help improve the performance of your scripts, especially when dealing with large datasets or frequent evaluations.
Here are some tips to optimize the efficiency of condition checks:
Related Article: How to Pass Parameters to Scripts in Bash
Order of Conditions
Consider the order of conditions within your If Else statements. Placing conditions that are likely to be true more often at the beginning of the statement can improve performance by reducing unnecessary evaluations.
if [ condition1 ] && [ condition2 ] && ...; then # Code block for the "if" branch else # Code block for the "else" branch fi
In the example above, if “condition1” is more likely to be true than “condition2”, it is more efficient to place “condition1” before “condition2” in the If Else statement. This way, if “condition1” evaluates to false, the script can skip evaluating “condition2” altogether.
Short-Circuit Evaluation
Take advantage of short-circuit evaluation in If Else statements to improve efficiency. Short-circuit evaluation means that if the result of the condition is determined by the first part of a logical AND (“&&”) or OR (“||”) operation, the second part is not evaluated.
if [ condition1 ] || [ condition2 ]; then # Code block for the "if" branch else # Code block for the "else" branch fi
In the example above, if “condition1” evaluates to true, the script skips evaluating “condition2” because the result of the OR operation is already determined.
Numeric Evaluations
When performing numeric evaluations, consider using arithmetic evaluation instead of invoking external commands. Arithmetic evaluation using double parentheses “(( ))” is generally faster and more efficient.
if ((number > 0)); then # Code block for the "if" branch else # Code block for the "else" branch fi
In the example above, using arithmetic evaluation with double parentheses is faster than invoking an external command such as “test” or “[ ]” for numeric comparisons.
By optimizing the efficiency of condition checks, you can improve the performance of your shell scripts, especially when dealing with large datasets or frequent evaluations.
Related Article: How to Choose the Preferred Bash Shebang in Linux
Performance Consideration 2: Impact of Nested If Else Statements
When using nested If Else statements in shell scripts, it is important to consider the potential impact on performance. Nested If Else statements can introduce additional complexity and overhead, which may affect the execution time of your scripts.
Here are some considerations to keep in mind when working with nested If Else statements:
Code Readability and Maintainability
While nested If Else statements can be useful for handling complex logic, excessive nesting can make your code harder to read and maintain. It is important to strike a balance between code readability and performance when deciding how deeply to nest your If Else statements.
Consider refactoring nested If Else statements into separate functions or using other control structures (e.g., case statements) if the logic becomes too convoluted or difficult to follow.
Performance Impact
Nested If Else statements can introduce additional branching and evaluation overhead, potentially affecting the performance of your scripts. Each level of nesting requires additional evaluations and comparisons, which can lead to increased execution time.
If performance is a critical concern, consider simplifying or optimizing your logic to reduce the number of nested If Else statements. This could involve reorganizing your code, combining conditions where possible, or leveraging other control structures that better suit your needs.
Related Article: Bash Scripting Handbook for Linux SysAdmins
Testing and Profiling
When working with nested If Else statements, it is important to thoroughly test and profile your scripts to identify any performance bottlenecks. Measure the execution time of your scripts with different inputs and conditions to gain insights into potential areas for improvement.
Profiling tools, such as the “time” command or specialized profiling utilities, can help identify performance issues and guide you in optimizing your code.
By carefully considering the impact of nested If Else statements on code readability and performance, you can create efficient and maintainable shell scripts.
Advanced Technique 1: Using If Else with Loops
Using If Else statements in conjunction with loops can enhance the power and flexibility of your shell scripts. By combining conditionals with iterative loops, you can perform complex operations and automate repetitive tasks.
Here are some examples of using If Else statements with loops:
While Loop
You can use If Else statements within a while loop to control the iteration based on specific conditions. This allows you to repeatedly perform actions until a certain condition is met or until a specific number of iterations is reached.
counter=0 while [ "$counter" -lt 5 ]; do if ((counter % 2 == 0)); then echo "$counter is even." else echo "$counter is odd." fi counter=$((counter + 1)) done
In this example, the while loop iterates as long as the value of “counter” is less than 5. Within the loop, an If Else statement is used to determine whether the value of “counter” is even or odd. The appropriate message is then displayed.
Related Article: Executing Bash Scripts Without Permissions in Linux
For Loop
You can also use If Else statements within a for loop to perform different actions based on specific conditions or inputs. This allows you to iterate over a set of values and apply different logic depending on the current value.
for fruit in apple banana cherry; do if [ "$fruit" == "banana" ]; then echo "I like $fruit the most." else echo "I like $fruit." fi done
In this example, the for loop iterates over the values “apple”, “banana”, and “cherry”. Within the loop, an If Else statement is used to determine if the current value is “banana”. If it is, a specific message is displayed. Otherwise, a general message is displayed.
By combining If Else statements with loops, you can develop powerful and dynamic shell scripts that automate repetitive tasks and handle complex logic.
Advanced Technique 2: Combining If Else with Functions
Combining If Else statements with functions allows you to encapsulate complex logic, improve code organization, and enhance code reusability in your shell scripts. By defining functions that contain If Else statements, you can modularize your code and make it more maintainable.
Here are some examples of using If Else statements with functions:
Function with If Else Statement
is_even() { number=$1 if ((number % 2 == 0)); then echo "$number is even." else echo "$number is odd." fi } is_even 4 is_even 7
In this example, a function named “is_even” is defined. The function takes a number as an argument and uses an If Else statement to determine whether the number is even or odd. The appropriate message is then displayed.
The function is invoked twice, with the arguments 4 and 7, respectively. The function outputs:
4 is even. 7 is odd.
Related Article: Tutorial: Functions in Bash Scripts on Linux
Function Returning a Value
is_even() { number=$1 if ((number % 2 == 0)); then return 0 else return 1 fi } if is_even 4; then echo "The number is even." else echo "The number is odd." fi
In this example, the “is_even” function determines whether a number is even or odd, as in the previous example. However, instead of directly displaying a message, the function returns a value: 0 if the number is even, and 1 if it is odd.
The If Else statement outside the function checks the return value of the function and displays an appropriate message.
The output is:
The number is even.
By combining If Else statements with functions, you can create reusable code blocks that encapsulate complex logic and improve the maintainability of your shell scripts.
Code Snippet Idea 1: Basic If Else Statement
if [ condition ]; then # Code to execute if the condition is true else # Code to execute if the condition is false fi
This code snippet demonstrates the basic structure of an If Else statement in shell scripting. The “condition” represents a logical test that evaluates to either true or false. If the condition is true, the code block following the “then” keyword is executed. Otherwise, the code block following the “else” keyword is executed. The “fi” keyword marks the end of the If Else statement.
Code Snippet Idea 2: If Else with Multiple Conditions
if [ condition1 ] && [ condition2 ]; then # Code to execute if both conditions are true elif [ condition3 ] || [ condition4 ]; then # Code to execute if either condition3 or condition4 is true else # Code to execute if none of the conditions are true fi
This code snippet demonstrates the use of multiple conditions within an If Else statement. The “condition1” and “condition2” are evaluated together using the logical AND operator “&&”. If both conditions are true, the code block following the “then” keyword is executed. If not, the “elif” keyword allows for additional conditions to be evaluated. In this case, if either “condition3” or “condition4” is true, the corresponding code block is executed. If none of the conditions are true, the code block following the “else” keyword is executed.
Related Article: How to Handle New Lines in Bash Scripts
Code Snippet Idea 3: Nested If Else Statement
if [ condition1 ]; then # Code to execute if condition1 is true if [ condition2 ]; then # Code to execute if condition2 is true else # Code to execute if condition2 is false fi else # Code to execute if condition1 is false fi
This code snippet demonstrates the use of nested If Else statements. The outer If Else statement checks the condition1. If it is true, the code block following the “then” keyword is executed. Within this code block, there is another If Else statement that checks the condition2. If condition2 is true, the corresponding code block is executed. Otherwise, the code block following the inner “else” keyword is executed. If condition1 is false, the code block following the outer “else” keyword is executed.
Code Snippet Idea 4: If Else with Logical Operators
if [ condition1 ] && [ condition2 ]; then # Code to execute if both condition1 and condition2 are true elif [ condition3 ] || [ condition4 ]; then # Code to execute if either condition3 or condition4 is true else # Code to execute if none of the conditions are true fi
This code snippet demonstrates the use of logical operators within an If Else statement. The logical AND operator “&&” is used to evaluate both condition1 and condition2. If both conditions are true, the code block following the “then” keyword is executed. The logical OR operator “||” is used to evaluate either condition3 or condition4. If either condition is true, the corresponding code block is executed. If none of the conditions are true, the code block following the “else” keyword is executed.
Code Snippet Idea 5: If Else with Regex Matching
if [[ "$string" =~ regex_pattern ]]; then # Code to execute if the string matches the regex pattern else # Code to execute if the string does not match the regex pattern fi
This code snippet demonstrates the use of regex matching within an If Else statement. The “string” variable is checked against the “regex_pattern” using the “=~” operator. If the string matches the pattern, the code block following the “then” keyword is executed. If the string does not match the pattern, the code block following the “else” keyword is executed.
By using these code snippets, you can create If Else statements with different conditions and logic, making your shell scripts more versatile and powerful.
Related Article: How to Use Getopts in Bash: A Practical Example
Handling Errors and Exceptions in Shell Scripts
When working with shell scripts, it is important to handle errors and exceptions gracefully. By implementing error-handling mechanisms, you can improve the reliability and robustness of your scripts.
Here are some techniques for handling errors and exceptions in shell scripts:
Exit Codes
Shell scripts return an exit code upon completion, indicating the success or failure of the script. By convention, an exit code of 0 represents success, while non-zero exit codes indicate errors or exceptions.
You can leverage exit codes to handle errors in your script. For example, you can use conditional statements to check the exit code and perform specific actions based on the result.
#!/bin/bash # Perform some operations command1 command2 command3 # Check the exit code of the last command if [ "$?" -eq 0 ]; then echo "All commands executed successfully." else echo "An error occurred while executing the commands." fi
In this example, the script executes multiple commands (command1, command2, command3). After each command, the script checks the exit code using the special variable “$?”. If the exit code is 0, it displays a success message. Otherwise, it displays an error message.
Error Output Redirection
You can redirect error output to a separate file or discard it altogether using the “>” or “2>” operators. This allows you to separate error messages from regular output and handle them differently.
#!/bin/bash # Perform some operations and redirect error output to a file command1 command2 2> error.log command3 # Check the existence of the error log file if [ -s error.log ]; then echo "An error occurred. Please check the error.log file for details." fi
In this example, the script redirects error output from command2 to the file “error.log” using the “2>” operator. After executing all commands, it checks if the error log file exists and has a non-zero size. If it does, it displays an error message.
Related Article: Should You Use Numbers in Bash Script Names?
Error Handling Functions
You can define custom error handling functions to encapsulate error-handling logic and improve code organization. These functions can be called whenever errors occur within your script.
#!/bin/bash handle_error() { error_message=$1 # Perform error handling actions echo "Error: $error_message" exit 1 } # Perform some operations command1 || handle_error "Error executing command1" command2 || handle_error "Error executing command2" command3 || handle_error "Error executing command3" echo "All commands executed successfully."
In this example, the script defines a custom error handling function named “handle_error”. Whenever an error occurs during the execution of a command, the corresponding error message is passed to the function. The function performs error handling actions, such as displaying an error message and exiting the script with a non-zero exit code.
By using these error handling techniques, you can make your shell scripts more resilient and capable of dealing with unexpected errors or exceptions.
Troubleshooting Common Errors with If Else
When working with If Else statements in shell scripts, it is common to encounter errors or unexpected behavior. Here are some troubleshooting tips for common errors:
Syntax Errors
Syntax errors can occur if you misspell keywords, forget to include necessary brackets, or misuse operators within your If Else statements.
To troubleshoot syntax errors, carefully review your code and ensure that you have correctly used keywords, brackets, and operators. Pay attention to matching pairs of brackets and quotes. Additionally, consider using syntax highlighting in your text editor to catch syntax errors early.
Related Article: How to Hide & Validate User Input in Bash Scripts
Variable Scope
Variable scope issues can occur if you use variables within If Else statements without properly defining or initializing them beforehand.
To troubleshoot variable scope issues, ensure that variables used within If Else statements are properly defined and initialized before they are referenced. Consider using the “local” keyword to limit the scope of variables to specific functions or blocks of code.
Condition Evaluation
Errors can occur if the conditions within your If Else statements are not evaluated as expected.
To troubleshoot condition evaluation issues, double-check the operators, brackets, and syntax used in your conditions. Test your conditions with different values to ensure they produce the desired results. Additionally, consider using echo statements or logging to output intermediate values for debugging purposes.
Command Execution
Errors can arise if the commands within your If Else statements fail to execute properly or produce unexpected results.
To troubleshoot command execution issues, verify that the commands are correctly written and that the necessary dependencies are installed. Check the exit codes of commands using the “$?” variable to identify potential errors. Additionally, consider redirecting error output to a file or using error handling functions to capture and handle command errors.
Related Article: How to Replace a Substring in a String in a Shell Script
Testing and Debugging If Else Statements
Testing and debugging are essential steps in ensuring the correctness and reliability of your If Else statements in shell scripts. By testing your code and using debugging techniques, you can identify and fix potential issues or errors.
Here are some testing and debugging techniques for If Else statements:
Manual Testing
Perform manual testing by running your script with different inputs and conditions. This allows you to observe the behavior of your If Else statements and verify that they produce the expected results.
When manually testing, consider edge cases, invalid inputs, and different scenarios to ensure that your If Else statements handle all possible scenarios correctly.
Logging and Debug Output
Insert echo statements or logging statements within your If Else statements to output intermediate values and debug information. This helps you understand the flow and behavior of your script during execution.
By logging relevant variables, conditions, and execution paths, you can identify potential issues or unexpected behavior within your If Else statements.
#!/bin/bash # Perform some operations echo "Executing command1..." command1 # Check the exit code of command1 if [ "$?" -eq 0 ]; then echo "Command1 executed successfully." else echo "An error occurred while executing command1." fi
In this example, echo statements are used to log the execution of “command1” and the subsequent handling of its exit code. By observing the log output, you can determine whether the command executed successfully or encountered an error.
Related Article: How to Detect if Your Bash Script is Running
Debugging Tools
Consider using debugging tools or utilities specifically designed for shell script debugging. These tools help trace the execution path, set breakpoints, and inspect variables during runtime.
Tools such as “bashdb” or “bashdbgui” provide a graphical user interface for debugging shell scripts. They allow you to step through your code, examine variables, and track the execution flow.
Testing and debugging are iterative processes, and it is important to repeat these steps as you make changes to your If Else statements or encounter new scenarios.