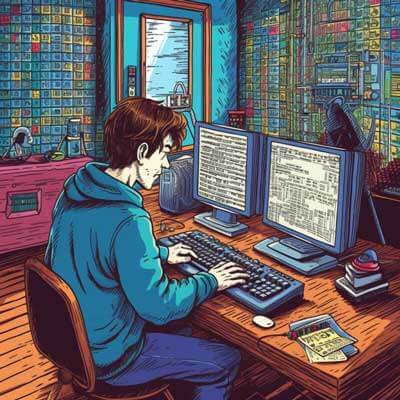
Table of Contents
Bash Script Variables in PHP
In many cases, developers find themselves needing to utilize variables from a Bash script in PHP on Linux. This can be useful when you have certain values or configurations defined in a Bash script that you want to access and use in your PHP code. Fortunately, there are several ways to achieve this integration and make the most out of both Bash and PHP.
Related Article: How to Handle Quotes with Md5sum in Bash Scripts
Passing Variables from Bash to PHP
To pass variables from a Bash script to a PHP script, you can make use of command-line arguments. By passing the variables as arguments when executing the PHP script, you can access them within your PHP code.
Here's an example of how you can pass variables from Bash to PHP:
#!/bin/bash # Define variables name="John" age=25 # Execute PHP script and pass variables as arguments php myscript.php $name $age
In the above script, we have defined two variables, name
and age
, and then we execute a PHP script called myscript.php
, passing the variables as arguments. Now, let's see how you can access these variables in your PHP script.
Using Bash Variables in PHP
To access the variables passed from the Bash script in your PHP code, you can make use of the $argv
array. This array contains all the command-line arguments passed to the PHP script, including the variables we passed from the Bash script.
Here's an example of how you can access the variables in PHP:
<?php // Access variables from command-line arguments $name = $argv[1]; $age = $argv[2]; // Use the variables in your PHP code echo "Name: " . $name . "\n"; echo "Age: " . $age . "\n"; ?>
In the above PHP script, we access the variables $argv[1]
and $argv[2]
, which correspond to the first and second command-line arguments passed to the script. We then use these variables in our PHP code, in this case, printing them out using echo
.
Accessing Bash Script Variables in PHP
Now that we have seen how to pass variables from a Bash script to a PHP script, let's take a look at how we can access variables defined within the Bash script itself.
To access Bash script variables in PHP, we can make use of environment variables. Environment variables are variables that are set in the shell and are accessible by any processes running within that shell session, including PHP scripts.
Here's an example of how you can access Bash script variables in PHP using environment variables:
#!/bin/bash # Define variables export name="John" export age=25 # Execute PHP script php myscript.php
In the above Bash script, we use the export
command to set the variables name
and age
as environment variables. This makes them accessible to any processes running within the same shell session, including our PHP script.
Now, let's see how we can access these variables in our PHP script.
<?php // Access environment variables $name = getenv('name'); $age = getenv('age'); // Use the variables in your PHP code echo "Name: " . $name . "\n"; echo "Age: " . $age . "\n"; ?>
In the above PHP script, we use the getenv
function to access the values of the environment variables name
and age
. We then use these variables in our PHP code, similar to what we did in the previous example.
Related Article: Troubleshooting: Unable to Run Bash Script as Root in Linux
PHP Script with Bash Variables
Using variables from a Bash script in a PHP script can be particularly useful when you have complex configurations or sensitive information that you want to keep separate from your PHP code. By defining these variables in a Bash script, you can easily update or modify them without touching your PHP code.
Let's take a look at an example of a PHP script that utilizes variables defined in a Bash script:
#!/bin/bash # Define variables export db_host="localhost" export db_user="myuser" export db_password="mypassword" # Execute PHP script php myscript.php
In the above Bash script, we define three environment variables: db_host
, db_user
, and db_password
, which represent the host, username, and password for a database connection, for example.
Now, let's see how we can use these variables in our PHP script:
<?php // Access environment variables $db_host = getenv('db_host'); $db_user = getenv('db_user'); $db_password = getenv('db_password'); // Use the variables in your PHP code $conn = new mysqli($db_host, $db_user, $db_password); // ... ?>
In the above PHP code, we access the environment variables using the getenv
function, and then use them to establish a database connection using the mysqli
extension.
PHP and Bash Integration
Integrating PHP and Bash scripts can be a useful way to combine the strengths of both languages and create more robust and flexible solutions. Whether you need to pass variables between the two scripts, access Bash variables in PHP, or execute one script from another, there are various options available to achieve seamless integration.
Sharing Variables Between Bash and PHP
One way to share variables between Bash and PHP scripts is by using environment variables, as we have seen in the previous examples. By exporting variables in Bash and accessing them using getenv
in PHP, you can easily pass information between the two scripts.
Another option is to use temporary files to store and retrieve variables. This can be useful when you have large or complex data structures that need to be shared between the scripts. For example, you can write the variables to a file in Bash and then read the file in PHP to access the values.
Here's an example of how you can share variables between Bash and PHP using temporary files:
#!/bin/bash # Define variables name="John" age=25 # Write variables to a temporary file echo "$name" > /tmp/variables.txt echo "$age" >> /tmp/variables.txt # Execute PHP script php myscript.php
In the above Bash script, we write the variables name
and age
to a temporary file called variables.txt
using the echo
command. We use the >
and >>
operators to redirect the output to the file.
Now, let's see how we can read the variables in our PHP script:
<?php // Read variables from the temporary file $lines = file('/tmp/variables.txt'); $name = trim($lines[0]); $age = trim($lines[1]); // Use the variables in your PHP code echo "Name: " . $name . "\n"; echo "Age: " . $age . "\n"; ?>
In the above PHP code, we use the file
function to read the contents of the temporary file into an array. We then use the trim
function to remove any leading or trailing whitespace from the lines, and assign the values to the variables $name
and $age
. Finally, we use these variables in our PHP code, similar to the previous examples.
Bash and PHP Variable Communication
When integrating Bash and PHP scripts, it's important to establish clear communication between the two. This includes passing variables, exchanging data, and handling errors or exceptions that may occur during the process.
To ensure smooth communication between Bash and PHP scripts, you can make use of standard input and output streams. By redirecting the output of one script to the input of another, you can pass data between the two scripts.
Here's an example of how you can communicate between Bash and PHP using standard input and output streams:
#!/bin/bash # Define variables name="John" age=25 # Pass variables to PHP script via standard input echo "$name" | php myscript.php echo "$age" | php myscript.php
In the above Bash script, we use the echo
command to pass the variables name
and age
to the PHP script using the pipe (|
) operator. This redirects the output of the echo
command to the standard input of the PHP script.
Now, let's see how we can read the variables in our PHP script:
<?php // Read variables from standard input $name = trim(fgets(STDIN)); $age = trim(fgets(STDIN)); // Use the variables in your PHP code echo "Name: " . $name . "\n"; echo "Age: " . $age . "\n"; ?>
In the above PHP code, we use the fgets
function to read the variables from the standard input stream. The STDIN
constant represents the standard input stream in PHP. We then use the trim
function to remove any leading or trailing whitespace from the variables, and finally, use them in our PHP code.
Related Article: Terminate Bash Script Loop via Keyboard Interrupt in Linux
PHP Script Calling Bash Script
In addition to using Bash script variables in PHP, you may also need to execute a Bash script from within your PHP code. This can be useful when you have certain tasks or operations that are better suited to be executed in a Bash script.
To execute a Bash script from PHP, you can make use of the exec
function. The exec
function allows you to run a command in the shell and capture the output. You can use this function to execute a Bash script and retrieve any output or result from it.
Here's an example of how you can call a Bash script from a PHP script:
<?php // Execute Bash script and capture the output $output = exec("bash myscript.sh"); // Print the output echo $output; ?>
In the above PHP code, we use the exec
function to execute the Bash script myscript.sh
. The output of the script is captured and stored in the variable $output
. Finally, we print the output using echo
.
It's important to note that when executing a Bash script from PHP, you need to ensure that the Bash script has executable permissions. You can set the permissions using the chmod
command, like this:
chmod +x myscript.sh
Bash Script Calling PHP Script
Similarly to calling a Bash script from PHP, you may also need to execute a PHP script from within your Bash script. This can be useful when you have certain tasks or computations that are better suited to be executed in PHP.
To call a PHP script from a Bash script, you can use the php
command followed by the path to the PHP script. You can also pass any necessary arguments to the PHP script using the command-line arguments.
Here's an example of how you can call a PHP script from a Bash script:
#!/bin/bash # Define variables name="John" age=25 # Call PHP script and pass variables as arguments php myscript.php $name $age
In the above Bash script, we define the variables name
and age
, and then call the PHP script myscript.php
, passing the variables as command-line arguments.
Now, let's see how we can access these variables in our PHP script:
<?php // Access variables from command-line arguments $name = $argv[1]; $age = $argv[2]; // Use the variables in your PHP code echo "Name: " . $name . "\n"; echo "Age: " . $age . "\n"; ?>
In the above PHP code, we access the variables passed as command-line arguments using the $argv
array, similar to what we have seen in previous examples. We then use these variables in our PHP code, in this case, printing them out using echo
.
Best Way to Share Variables Between Bash and PHP Scripts
When it comes to sharing variables between Bash and PHP scripts, there are several approaches you can take. The best way to share variables depends on the specific requirements and constraints of your project.
One common approach is to use environment variables. By exporting variables in Bash and accessing them using getenv
in PHP, you can easily pass information between the two scripts. This approach is simple and works well for most use cases.
Here's an example of how you can share variables between Bash and PHP using environment variables:
#!/bin/bash # Define variables export name="John" export age=25 # Execute PHP script php myscript.php
In the above Bash script, we use the export
command to set the variables name
and age
as environment variables. This makes them accessible to any processes running within the same shell session, including our PHP script.
Now, let's see how you can access these variables in your PHP script:
<?php // Access environment variables $name = getenv('name'); $age = getenv('age'); // Use the variables in your PHP code echo "Name: " . $name . "\n"; echo "Age: " . $age . "\n"; ?>
In the above PHP code, we use the getenv
function to access the values of the environment variables name
and age
. We then use these variables in our PHP code, in this case, printing them out using echo
.
Another option for sharing variables between Bash and PHP scripts is to use temporary files. This can be useful when you have large or complex data structures that need to be shared between the scripts. For example, you can write the variables to a file in Bash and then read the file in PHP to access the values.
Here's an example of how you can share variables between Bash and PHP using temporary files:
#!/bin/bash # Define variables name="John" age=25 # Write variables to a temporary file echo "$name" > /tmp/variables.txt echo "$age" >> /tmp/variables.txt # Execute PHP script php myscript.php
In the above Bash script, we write the variables name
and age
to a temporary file called variables.txt
using the echo
command. We use the >
and >>
operators to redirect the output to the file.
Now, let's see how you can read the variables in your PHP script:
<?php // Read variables from the temporary file $lines = file('/tmp/variables.txt'); $name = trim($lines[0]); $age = trim($lines[1]); // Use the variables in your PHP code echo "Name: " . $name . "\n"; echo "Age: " . $age . "\n"; ?>
In the above PHP code, we use the file
function to read the contents of the temporary file into an array. We then use the trim
function to remove any leading or trailing whitespace from the lines, and assign the values to the variables $name
and $age
. Finally, we use these variables in our PHP code, similar to the previous examples.
How to Call a Bash Script from a PHP Script and Receive Variables?
To call a Bash script from a PHP script and receive variables, you can make use of the exec
function in PHP. The exec
function allows you to run a command in the shell and capture the output. You can use this function to execute a Bash script and retrieve any output or result from it.
Here's an example of how you can call a Bash script from a PHP script and receive variables:
<?php // Execute Bash script and capture the output $output = exec("bash myscript.sh"); // Process the output and extract variables list($name, $age) = explode(',', $output); // Use the variables in your PHP code echo "Name: " . $name . "\n"; echo "Age: " . $age . "\n"; ?>
In the above PHP code, we use the exec
function to execute the Bash script myscript.sh
. The output of the script is captured and stored in the variable $output
. We then process the output and extract the variables name
and age
using the explode
function.
In the Bash script myscript.sh
, you can set the variables and output them in a format that can be easily processed by the PHP script. For example:
#!/bin/bash # Define variables name="John" age=25 # Output variables separated by a comma echo "$name,$age"
In the above Bash script, we define two variables, name
and age
, and then output them separated by a comma. This allows the PHP script to split the output and retrieve the variables.
Related Article: How to Fix Openssl Error: Self Signed Certificate in Certificate Chain on Linux
Limitations and Considerations When Using Bash and PHP Together
When using Bash and PHP together, there are some limitations and considerations to keep in mind to ensure proper integration and avoid potential issues.
One limitation is that Bash and PHP have different syntax and features. While Bash is primarily used for shell scripting and executing commands in the shell, PHP is a full-fledged programming language with a wide range of features and capabilities. It's important to understand the differences between the two languages and consider their specific use cases when integrating them.
Another consideration is the security implications of passing variables between Bash and PHP scripts. When passing variables as command-line arguments or using environment variables, it's important to sanitize and validate the input to prevent any potential security vulnerabilities, such as command injection or code execution vulnerabilities. Always validate and sanitize user input before using it in your scripts.
Additionally, when executing Bash scripts from PHP or vice versa, it's important to ensure proper permissions and access control. Make sure that the scripts have the necessary permissions to be executed and that they are being executed in a secure and controlled environment. Avoid executing scripts from untrusted or user-controlled sources to prevent any potential security risks.
Furthermore, when integrating Bash and PHP scripts, it's important to consider the performance implications. Running commands or executing scripts can introduce additional overhead and may impact the overall performance of your application. Consider optimizing and refactoring your code to minimize the number of script invocations and improve the overall performance of your application.
Lastly, it's important to maintain proper documentation and version control when working with Bash and PHP scripts together. Keep track of any changes made to the scripts, document any dependencies or requirements, and ensure that the scripts are properly tested and maintained.
Additional Resources
- Integrating PHP with Linux Command Line Operations