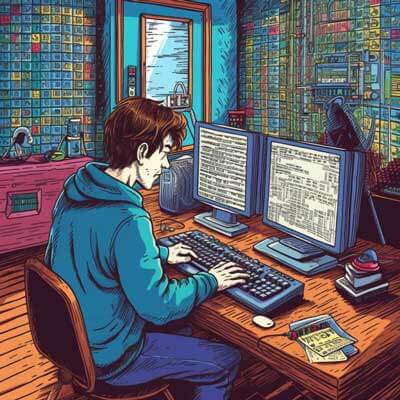
- How to Read a Text File in a Bash Script
- Using Linux Commands to Read Text Files
- Executing a Bash Script that Reads a Text File
- Understanding the Difference Between Reading and Executing a Text File in a Bash Script
- Step-by-Step Guide to Reading a Text File with a Shell Script
- Parsing a Text File in a Bash Script
- Common Methods for Reading Input Files in Bash Scripts
- Tools for Reading Text Files in Linux
- Extracting Data from a Text File Using a Bash Script
- Additional Resources
How to Read a Text File in a Bash Script
When working with Bash scripts in Linux, it is often necessary to read text files as input or to extract data from them. Bash provides various methods for reading text files, allowing you to manipulate and process the information contained within.
One of the simplest ways to read a text file in a Bash script is by using the read
command. The read
command allows you to read a line from standard input or a file and assign it to a variable. Here is an example:
#!/bin/bash file="example.txt" while IFS= read -r line do echo "$line" done < "$file"
In this example, we define a variable file
which holds the name of the text file we want to read. We then use a while
loop to iterate over each line in the file. The read
command reads each line and assigns it to the variable line
. We then echo the value of line
to display it on the console.
Related Article: How To Echo a Newline In Bash
Using Linux Commands to Read Text Files
Aside from using built-in Bash commands, you can also leverage Linux commands to read text files within a Bash script. These commands provide additional functionality and flexibility for processing and manipulating text files.
One such command is cat
, which is commonly used to concatenate and display the contents of files. However, it can also be used to read text files line by line in a Bash script. Here is an example:
#!/bin/bash file="example.txt" cat "$file" | while IFS= read -r line do echo "$line" done
In this example, we use the cat
command to output the contents of the file to standard output. The |
symbol, also known as a pipe, redirects the output of cat
to the while
loop. The read
command then reads each line and assigns it to the variable line
, which we echo to display on the console.
Executing a Bash Script that Reads a Text File
To execute a Bash script that reads a text file, you first need to ensure that the script has execute permissions. You can use the chmod
command to grant execute permissions to the script. Here is an example:
chmod +x script.sh
Once the script has execute permissions, you can run it by simply typing the name of the script in the command line. Here is an example:
./script.sh
In this example, script.sh
is the name of the Bash script file. By prefixing the script name with ./
, we tell the command line to look for the script in the current directory.
Understanding the Difference Between Reading and Executing a Text File in a Bash Script
In the context of a Bash script, reading a text file refers to the process of accessing the contents of the file and manipulating them within the script. This can involve tasks such as extracting specific data, performing calculations, or generating reports based on the file’s contents.
On the other hand, executing a text file in the context of a Bash script refers to running the script itself, which may include reading a text file as part of its execution. When a Bash script is executed, the commands within the script are interpreted and executed by the Bash interpreter.
It’s important to note that executing a text file does not necessarily mean that the file is being read. The file may contain executable code or instructions that are unrelated to reading text files.
Related Article: How to Use If-Else Statements in Shell Scripts
Step-by-Step Guide to Reading a Text File with a Shell Script
To read a text file with a shell script in Linux, follow these step-by-step instructions:
1. Open a text editor and create a new file. This file will serve as your shell script.
2. Begin the script by declaring the shebang, which specifies the interpreter to be used. For example, #!/bin/bash
.
3. Define a variable to hold the name of the text file you want to read. For example, file="example.txt"
.
4. Use a loop, such as a while
loop, to iterate over each line in the file. For example:
while IFS= read -r line do # Do something with each line done < "$file"
5. Within the loop, you can perform any desired operations on each line. For example, you can echo the line to display it on the console, extract specific data, or perform calculations.
6. Save the shell script file with a .sh
extension, such as script.sh
.
7. Grant execute permissions to the script using the chmod
command. For example:
chmod +x script.sh
8. Run the script by typing the command ./script.sh
in the command line.
Parsing a Text File in a Bash Script
Parsing a text file in a Bash script refers to the process of analyzing the contents of the file and extracting specific data or information. This can be done using various techniques and commands available in Bash.
One common method for parsing a text file is by using the awk
command. Awk is a useful text processing tool that allows you to define patterns and actions to be performed on each line of a file. Here is an example:
#!/bin/bash file="example.txt" awk '/pattern/ { action }' "$file"
In this example, we define a pattern to search for in the file using the /pattern/
syntax. When the pattern is matched, the corresponding action is executed. Actions can be any valid Bash command or a series of commands enclosed in curly braces {}
.
Another method for parsing a text file is by using the grep
command. Grep is a command-line utility that searches for patterns in files and displays matching lines. Here is an example:
#!/bin/bash file="example.txt" grep "pattern" "$file"
In this example, we specify the pattern to search for within double quotes "pattern"
. The grep
command then searches for lines in the file that contain the specified pattern and displays them on the console.
Common Methods for Reading Input Files in Bash Scripts
When it comes to reading input files in Bash scripts, there are several common methods that can be used depending on the specific requirements of your script. Some of these methods include:
1. Using the read
command: This method allows you to read a text file line by line and assign each line to a variable. This can be useful when you need to process each line individually. See the previous section for an example.
2. Using Linux commands: Linux commands such as cat
, awk
, and grep
can be used to read and process text files within a Bash script. These commands provide additional functionality and flexibility for manipulating text files. See the previous sections for examples.
3. Using command-line arguments: Bash scripts can accept command-line arguments, which can be used to specify the input file to be read. This allows for greater flexibility as the input file can be easily changed without modifying the script itself. Here is an example:
#!/bin/bash file="$1" while IFS= read -r line do echo "$line" done < "$file"
In this example, we use the special variable $1
to access the first command-line argument passed to the script. This argument is then assigned to the variable file
, which is used to read the text file.
Related Article: How to Manipulate Quotes & Strings in Bash Scripts
Tools for Reading Text Files in Linux
In addition to built-in Bash commands and Linux utilities, there are several tools available in Linux that can be used to read text files. These tools provide advanced features and functionality for processing and manipulating text files.
One such tool is sed
, which is a stream editor for filtering and transforming text. Sed allows you to perform operations such as search and replace, insertion, deletion, and more on text files. Here is an example:
#!/bin/bash file="example.txt" sed 's/pattern/replacement/' "$file"
In this example, we use the sed
command to search for a pattern in the file and replace it with the specified replacement text. The 's/pattern/replacement/'
syntax defines the search and replace operation.
Another tool that can be useful for reading text files is awk
, as mentioned earlier. Awk is a versatile tool that allows for complex text processing and data extraction. Here is an example:
#!/bin/bash file="example.txt" awk '/pattern/ { print $2 }' "$file"
In this example, we use awk
to search for lines in the file that match the specified pattern. When a match is found, the second field of the line (denoted by $2
) is printed.
Extracting Data from a Text File Using a Bash Script
Extracting data from a text file using a Bash script involves searching for specific patterns or information within the file and extracting the relevant data. This can be done using various techniques, such as regular expressions and Linux commands.
One common method for extracting data from a text file is by using the grep
command. Grep allows you to search for patterns in files and display matching lines. Here is an example:
#!/bin/bash file="example.txt" grep "pattern" "$file" | awk '{ print $1 }'
In this example, we use grep
to search for lines in the file that contain the specified pattern. The |
symbol, known as a pipe, redirects the output of grep
to the awk
command. Awk
is then used to extract the first field of each line and print it.
Another method for extracting data from a text file is by using regular expressions. Regular expressions are a useful pattern matching language that allows for complex searches and data extraction. Here is an example:
#!/bin/bash file="example.txt" while IFS= read -r line do if [[ $line =~ [0-9]+ ]] then echo "${BASH_REMATCH[0]}" fi done < "$file"
In this example, we use a while
loop to iterate over each line in the file. We then use the =~
operator to match the line against a regular expression pattern. If a match is found, the matched portion is stored in the BASH_REMATCH
array. We can then access and print the matched portion using ${BASH_REMATCH[0]}
.
These are just a few examples of how you can extract data from a text file using a Bash script. The specific method you choose will depend on the structure and content of the file, as well as the desired outcome.
Additional Resources
– Common bash commands
– Bash Scripting Tutorial – 11. Writing and calling functions