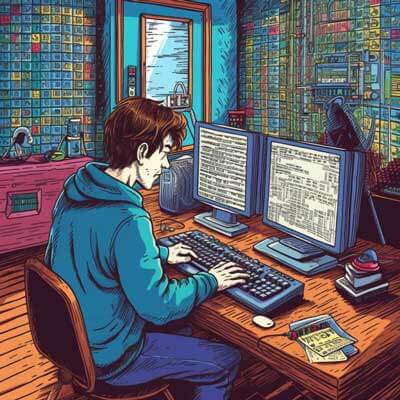
Appending Date to Filename
In Linux, appending the date to a filename is a common task in shell scripting. It allows you to create unique filenames that include the current date, making it easier to organize and manage your files. This can be useful for tasks such as creating backup files, generating log files, or creating timestamped versions of files.
To append the date to a filename, you can use the date
command in combination with string manipulation in a Linux bash script. The date
command allows you to format the current date and time in various ways, including specifying the format for the filename.
Related Article: How To Echo a Newline In Bash
Appending Current Date to File Name using Bash Script
Now let’s dive into the specific steps for appending the current date to a filename using a bash script.
Step 1: Define the source file and destination folder variables:
source_file="example.txt" destination_folder="output"
Step 2: Get the current date using the date
command:
date=$(date +"%Y-%m-%d")
Step 3: Extract the file extension from the source file:
extension="${source_file##*.}"
Step 4: Append the current date to the filename:
new_filename="${source_file%.*}_${date}.${extension}"
Step 5: Specify the destination path:
destination_path="${destination_folder}/${new_filename}"
Step 6: Copy the source file to the destination path:
cp "$source_file" "$destination_path"
Step 7: Print the new filename and destination path:
echo "New filename: $new_filename" echo "Destination path: $destination_path"
Putting it all together, here’s the complete bash script:
#!/bin/bash source_file="example.txt" destination_folder="output" date=$(date +"%Y-%m-%d") extension="${source_file##*.}" new_filename="${source_file%.*}_${date}.${extension}" destination_path="${destination_folder}/${new_filename}" cp "$source_file" "$destination_path" echo "New filename: $new_filename" echo "Destination path: $destination_path"
When you run this script, it will create a copy of the source file with the current date appended to the filename in the specified destination folder.
Specific Considerations for File Name Manipulation in Linux
When manipulating filenames in Linux, there are a few specific considerations to keep in mind to ensure compatibility and avoid issues:
– Case sensitivity: Filenames in Linux are case-sensitive, so be aware of the case of filenames when performing file name manipulations. It’s a good practice to use lowercase letters for filenames to avoid confusion.
– Special characters: Some special characters have special meanings in the Linux command line, so it’s important to be cautious when using them in filenames. It’s best to stick to alphanumeric characters, underscores, and hyphens to ensure compatibility and avoid issues.
– Path separators: Linux uses forward slashes (/) as path separators, so be careful when manipulating filenames that include directories. Always use the appropriate path separator to ensure compatibility across different systems.
– Length limitations: Different filesystems have different limitations on the length of filenames. It’s important to be aware of these limitations to avoid issues when creating, manipulating, or accessing files with long filenames.
Linux File Naming Conventions
When working with filenames in Linux, it’s important to follow certain conventions to ensure compatibility and readability across different systems. Here are some best practices for naming files in Linux:
– Use lowercase letters: Filenames in Linux are case-sensitive, so it’s best to use lowercase letters to avoid confusion.
– Avoid spaces and special characters: Spaces and special characters can cause issues when working with files in the command line, so it’s best to use underscores or hyphens instead.
– Be descriptive: Choose filenames that accurately describe the contents of the file. This will make it easier to understand and manage your files.
– Use file extensions: File extensions help identify the file type and determine how it should be handled. Use appropriate file extensions for your files.
– Keep filenames short and concise: Long filenames can be cumbersome to work with, so try to keep them as short and concise as possible while still conveying the necessary information.
Related Article: How to Use If-Else Statements in Shell Scripts
The Date Command
The date
command in Linux is used to display or manipulate the current date and time. It provides a wide range of formatting options, allowing you to customize the output according to your needs.
Here are a few examples of how to use the date
command:
– Display the current date and time:
date
This will output the current date and time in the default format.
– Format the date:
date +"%Y-%m-%d"
This will output the current date in the format “YYYY-MM-DD”.
– Format the time:
date +"%H:%M:%S"
This will output the current time in the format “HH:MM:SS”.
– Format both the date and time:
date +"%Y-%m-%d %H:%M:%S"
This will output the current date and time in the format “YYYY-MM-DD HH:MM:SS”.
These are just a few examples of the many formatting options available with the date
command. You can consult the date
man page for a comprehensive list of formatting options and further usage instructions.