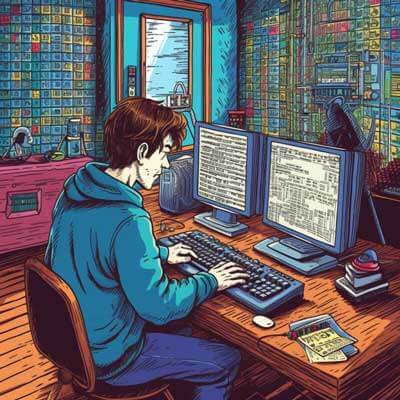
Table of Contents
The getopts
command in Bash is a useful tool that allows you to parse command-line options and arguments in your shell scripts. It provides a convenient way to handle user input and control the behavior of your script based on the specified options.
Step 1: Understanding the Basics of Getopts
Before diving into a practical example, it's important to understand the basics of how getopts
works. The getopts
command takes three arguments: the option string, the variable name to store the current option, and the variable name to store the argument (if any) of the current option.
The option string is a sequence of characters that represent the valid options for your script. Each character in the option string corresponds to a single option. If a character is followed by a colon (e.g., a:
), it means that the option requires an argument.
Here's a simple example that demonstrates the basic usage of getopts
:
#!/bin/bashwhile getopts "a:b" option; do case $option in a) echo "Option a is set with argument: $OPTARG" ;; b) echo "Option b is set" ;; \?) echo "Invalid option: -$OPTARG" exit 1 ;; esacdone
In this example, we define two options: -a
and -b
. The -a
option requires an argument, while the -b
option does not. The getopts
command parses the options and arguments passed to the script, and the case
statement handles each option accordingly.
Related Article: Displaying Memory Usage in Bash Scripts on Linux
Step 2: Running the Script and Testing the Options
To run the script and test the options, you can save it to a file (e.g., script.sh
), make it executable using the chmod
command (chmod +x script.sh
), and then execute it with the desired options.
Here are a few examples of how you can run the script and test the options:
$ ./script.sh -a argumentOption a is set with argument: argument$ ./script.sh -bOption b is set$ ./script.sh -cInvalid option: -c
As you can see, the script correctly handles the options and arguments based on the defined logic in the case
statement.
Step 3: Handling Multiple Options and Arguments
In addition to handling individual options, getopts
can also handle multiple options and arguments together. You can specify multiple characters in the option string, and getopts
will iterate over each option passed to the script.
Here's an example that demonstrates how to handle multiple options and arguments:
#!/bin/bashwhile getopts "a:b:c:" option; do case $option in a) echo "Option a is set with argument: $OPTARG" ;; b) echo "Option b is set" ;; c) echo "Option c is set with argument: $OPTARG" ;; \?) echo "Invalid option: -$OPTARG" exit 1 ;; esacdone
In this example, we define three options: -a
, -b
, and -c
. The -a
and -c
options require arguments, while the -b
option does not. The getopts
command will iterate over each option and argument passed to the script.
Step 4: Best Practices and Alternative Ideas
When using getopts
in your Bash scripts, it's important to follow some best practices to ensure clean and maintainable code:
1. Use meaningful option characters: Choose option characters that are easy to understand and remember. This will make your script more user-friendly and reduce confusion.
2. Provide clear help messages: If your script has complex options or requires specific arguments, consider providing a help message that explains the usage and expected input.
3. Validate and sanitize user input: Before using the values of options and arguments, validate and sanitize them to prevent potential security vulnerabilities or unexpected behavior.
4. Use functions for code modularity: If your script has multiple sections that require different options, consider using functions to handle each section separately. This will make your code more modular and easier to maintain.
5. Consider using external libraries: If your script requires advanced option handling or complex argument parsing, consider using external libraries like getopt
or argparse
to simplify your code and handle edge cases more effectively.