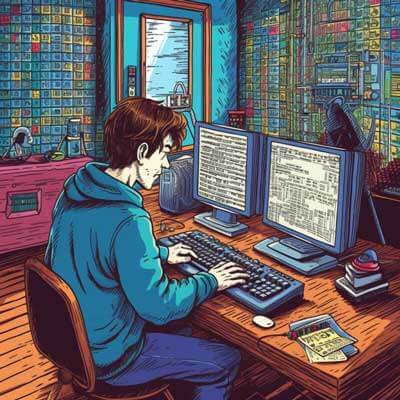
Table of Contents
Rounding in Bash Scripting
Rounding numbers is a common task in many programming languages, including Bash scripting. In Bash, you can use various techniques to round numbers to a specific decimal place. Let's explore some of the methods available.
Related Article: How to Import JSON from a Bash Script on Linux
Method 1: Using the printf Command
One way to round a number in Bash scripting is by using the printf
command. The printf
command allows you to format and print data, including numbers, with precision.
To round a number to 2 decimal places using printf
, you can use the following syntax:
number=3.14159 rounded=$(printf "%.2f" $number) echo $rounded
In this example, we have a variable number
with the value 3.14159
. We then use printf
to format the number with a precision of 2 decimal places (%.2f
). The rounded number is stored in the variable rounded
, and we print it using echo
.
The output of this code will be 3.14
, which is the rounded value of 3.14159
to 2 decimal places.
Method 2: Using the bc Command
Another method to round a number in Bash scripting is by using the bc
command. The bc
command is a useful calculator that supports arbitrary-precision arithmetic.
To round a number to 2 decimal places using bc
, you can use the following syntax:
number=3.14159 rounded=$(echo "scale=2; $number" | bc) echo $rounded
In this example, we set the variable number
to 3.14159
. We then use the echo
command to pipe the expression "scale=2; $number"
to bc
. The scale=2
sets the decimal precision to 2 places. The rounded number is stored in the variable rounded
, and we print it using echo
.
The output of this code will also be 3.14
, which is the rounded value of 3.14159
to 2 decimal places.
Floating Point Arithmetic in Bash Scripting
Performing floating-point arithmetic in Bash scripting can be a bit tricky, as Bash primarily supports integer arithmetic. However, there are ways to work with floating-point numbers in Bash. Let's explore a few techniques.
Related Article: Secure File Transfer with SFTP: A Linux Tutorial
Method 1: Using the bc Command
As mentioned earlier, the bc
command is a versatile calculator that supports floating-point arithmetic. You can use it to perform calculations with floating-point numbers in Bash scripting.
To perform floating-point arithmetic using bc
, you can use the syntax:
result=$(echo "3.14 + 2.71" | bc) echo $result
In this example, we use the echo
command to pipe the expression "3.14 + 2.71"
to bc
. The result of the calculation is stored in the variable result
, and we print it using echo
.
The output of this code will be 5.85
, which is the sum of 3.14
and 2.71
.
Method 2: Using the awk Command
Another way to perform floating-point arithmetic in Bash scripting is by using the awk
command. awk
is a useful text processing tool that can also handle arithmetic operations.
To perform floating-point arithmetic using awk
, you can use the syntax:
result=$(awk 'BEGIN {printf "%.2f", 3.14 + 2.71}') echo $result
In this example, we use awk
with the BEGIN
statement to perform the calculation 3.14 + 2.71
. We use the printf
function to format the result with a precision of 2 decimal places (%.2f
). The result is stored in the variable result
, and we print it using echo
.
The output of this code will also be 5.85
, which is the sum of 3.14
and 2.71
.
Formatting Numbers in Bash Scripting
When working with numbers in Bash scripting, you may often need to format them for better readability or presentation. Bash provides several built-in functions and techniques to format numbers. Let's explore some of them.
Method 1: Using the printf Command
The printf
command, in addition to rounding numbers, can also be used to format numbers with leading zeros or a specific width.
To format a number with leading zeros, you can use the syntax:
number=7 formatted=$(printf "%03d" $number) echo $formatted
In this example, we have a variable number
with the value 7
. We use printf
with the format %03d
, where %d
indicates a decimal number and 03
specifies the width of the number with leading zeros. The formatted number is stored in the variable formatted
, and we print it using echo
.
The output of this code will be 007
, which is the formatted version of the number 7
with leading zeros.
Related Article: How to Compare Strings in Bash: A Simple Guide
Method 2: Using the awk Command
The awk
command can also be used to format numbers in Bash scripting. By combining awk
with the printf
function, you can achieve various number formatting options.
To format a number with a specific width and decimal places using awk
, you can use the syntax:
number=3.14 formatted=$(awk 'BEGIN {printf "%-6.2f", '$number'}') echo $formatted
In this example, we set the variable number
to 3.14
. We use awk
with the BEGIN
statement to perform the formatting. Inside BEGIN
, we use printf
with the format %6.2f
, where %f
indicates a floating-point number, 6
specifies the width, and 2
specifies the number of decimal places. The formatted number is stored in the variable formatted
, and we print it using echo
.
The output of this code will be 3.14
, which is the formatted version of the number 3.14
with a width of 6 and 2 decimal places.
How to Round a Number to 2 Decimal Places in Bash Scripting
Rounding a number to 2 decimal places in Bash scripting can be achieved using the techniques we discussed earlier. Here's a step-by-step guide on how to round a number to 2 decimal places.
Step 1: Assign the number you want to round to a variable:
number=3.14159
Step 2: Use the printf
command to round the number to 2 decimal places:
rounded=$(printf "%.2f" $number)
Step 3: Print the rounded number:
echo $rounded
Putting it all together, here's a complete example:
number=3.14159 rounded=$(printf "%.2f" $number) echo $rounded
The output of this code will be 3.14
, which is the rounded value of 3.14159
to 2 decimal places.
Best Practices for Floating Point Arithmetic in Bash Scripting
Performing floating-point arithmetic in Bash scripting requires some considerations to ensure accuracy and avoid common pitfalls. Here are some best practices to follow when working with floating-point numbers in Bash.
1. Use Tools Designed for Floating-Point Arithmetic
Bash scripting primarily supports integer arithmetic, so it's recommended to use external tools such as bc
or awk
for floating-point calculations. These tools provide more accurate results and better precision for floating-point operations.
Related Article: Terminate Bash Script Loop via Keyboard Interrupt in Linux
2. Be Mindful of Floating-Point Precision
Floating-point numbers have limited precision, which can lead to rounding errors and unexpected results. It's important to be aware of the precision limitations and adjust your calculations accordingly. For example, you may need to round the final result or use a higher precision when necessary.
3. Be Cautious with Equality Comparisons
Due to the precision limitations of floating-point numbers, direct equality comparisons may not yield the expected results. Instead of comparing two floating-point numbers directly, it's recommended to use a range or a tolerance to account for small differences.
4. Use Specific Formatting Options
When displaying or outputting floating-point numbers, consider using specific formatting options to improve readability. The printf
command, as mentioned earlier, allows you to control the precision, width, and other formatting aspects of numbers.
Built-in Functions for Formatting Numbers with Decimal Places in Bash Scripting
While Bash scripting doesn't have built-in functions specifically for formatting numbers with decimal places, you can utilize external tools like bc
or awk
to achieve the desired formatting.
Here's an example of how you can format a number with 2 decimal places using the bc
command:
number=3.14159 formatted=$(echo "scale=2; $number" | bc) echo $formatted
In this example, we set the variable number
to 3.14159
. We use the echo
command to pipe the expression "scale=2; $number"
to bc
. The scale=2
sets the decimal precision to 2 places. The formatted number is stored in the variable formatted
, and we print it using echo
.
The output of this code will be 3.14
, which is the formatted value of 3.14159
with 2 decimal places.
While Bash doesn't provide native functions for formatting decimal places, using external tools like bc
or awk
can help you achieve the desired formatting requirements.