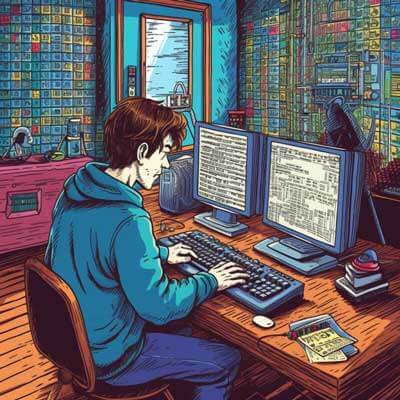
Table of Contents
Determining the success of a Bash script is an essential part of shell scripting. It allows you to check if the script executed successfully or encountered any errors during execution. In this article, we will explore various techniques to determine the success of a Bash script in Linux.
Bash Script Success Check
One of the common ways to determine the success of a Bash script is to check its exit status. Every command executed in a Bash script returns an exit status code. By convention, an exit status code of 0 indicates success, while any non-zero exit status code indicates an error.
To check the exit status of a previous command or script, you can use the special variable $?
. This variable holds the exit status of the last executed command.
Here's an example of checking the exit status of a command in a Bash script:
#!/bin/bash ls /path/to/nonexistent/file if [[ $? -eq 0 ]]; then echo "Command succeeded" else echo "Command failed" fi
In this example, the ls
command tries to list a non-existent file. Since the command fails and returns a non-zero exit status code, the script will output "Command failed".
Related Article: How to Import JSON from a Bash Script on Linux
Check if Bash Script Succeeded
To determine if a Bash script as a whole succeeded or failed, you can use the exit status of the script itself. By convention, you can use the exit
command to explicitly set the exit status of the script. If no exit status is specified, the script will inherit the exit status of the last command executed.
Here's an example of checking the exit status of a Bash script:
#!/bin/bash # Some commands and logic if [[ $? -eq 0 ]]; then echo "Script succeeded" else echo "Script failed" fi
In this example, the script checks the exit status using $?
and outputs "Script succeeded" if the exit status is 0, indicating success.
Bash Script Exit Code
The exit code of a Bash script represents the status of its execution. A successful execution typically has an exit code of 0, while a non-zero exit code indicates an error or failure.
To set the exit code of a Bash script, you can use the exit
command followed by the desired exit code. For example, to explicitly set the exit code to 1, you can use:
#!/bin/bash # Some commands and logic exit 1
In this example, the script sets the exit code to 1, indicating a failure.
Determine if Bash Script Completed Successfully
To determine if a Bash script completed successfully, you can check the exit code using conditional statements. If the exit code is 0, the script is considered to have completed successfully.
Here's an example of checking if a Bash script completed successfully:
#!/bin/bash # Some commands and logic exit_code=$? if [[ $exit_code -eq 0 ]]; then echo "Script completed successfully" else echo "Script failed with exit code $exit_code" fi
In this example, the script checks the exit code using the $?
variable and outputs "Script completed successfully" if the exit code is 0.
Related Article: How to Read Text Files in Linux with Bash Scripts
Check Bash Script Execution Result
Another way to determine the success of a Bash script is to use the if
statement to check the execution result of a specific command or a block of commands.
Here's an example of checking the execution result of a command in a Bash script:
#!/bin/bash # Some commands and logic if command; then echo "Command succeeded" else echo "Command failed" fi
In this example, the if
statement checks the execution result of the command
and outputs "Command succeeded" if it succeeds.
You can also use the if
statement to check the execution result of a block of commands enclosed in curly braces:
#!/bin/bash # Some commands and logic if { command1; command2; } then echo "Commands succeeded" else echo "Commands failed" fi
In this example, the if
statement checks the execution result of command1
and command2
and outputs "Commands succeeded" if both commands succeed.
Bash Script Error Handling
Error handling is an important aspect of Bash scripting. It allows you to handle and recover from errors that may occur during script execution. There are several techniques and best practices for error handling in Bash scripts.
Bash Script Status Check
To perform error handling in a Bash script, you can use conditional statements to check the exit status of commands and take appropriate actions based on the result.
Here's an example of error handling in a Bash script using conditional statements:
#!/bin/bash if command1; then echo "Command 1 succeeded" else echo "Command 1 failed" exit 1 fi if command2; then echo "Command 2 succeeded" else echo "Command 2 failed" exit 1 fi # Continue with the rest of the script
In this example, the script checks the exit status of command1
and command2
using conditional statements. If any of the commands fail, the script outputs an error message and exits with a non-zero exit status code.
Validate Bash Script Success
To validate the success of a Bash script, you can use assertions or assertions-like statements to check if certain conditions are met during script execution. If the conditions are not met, you can raise an error or exit the script with a non-zero exit status code.
Here's an example of validating the success of a Bash script using assertions:
#!/bin/bash # Some commands and logic assert_command_succeeded() { if ! "$@"; then echo "Assertion failed: $*" exit 1 fi } # Validate that the required dependencies are installed assert_command_succeeded command1 --version assert_command_succeeded command2 --version # Continue with the rest of the script
In this example, the script defines a function assert_command_succeeded
that checks if a command succeeds. If the command fails, the function outputs an error message and exits with a non-zero exit status code. The script then uses the assert_command_succeeded
function to validate the success of the required dependencies.
Related Article: Troubleshooting: Unable to Save Bash Scripts in Vi on Linux
Bash Script Exit Status
The exit status of a Bash script is an important indicator of its success or failure. By convention, an exit status of 0 indicates success, while any non-zero exit status indicates an error or failure.
To set the exit status of a Bash script, you can use the exit
command followed by the desired exit status code.
Here's an example of setting the exit status of a Bash script:
#!/bin/bash # Some commands and logic exit 0
In this example, the script explicitly sets the exit status to 0, indicating success.
Check the Return Code of a Bash Script
The return code of a Bash script is equivalent to its exit status. It represents the status of the script's execution. A return code of 0 indicates success, while a non-zero return code indicates an error or failure.
To check the return code of a Bash script, you can use the $?
variable, which holds the exit status of the last executed command.
Here's an example of checking the return code of a Bash script:
#!/bin/bash # Some commands and logic exit_code=$? if [[ $exit_code -eq 0 ]]; then echo "Script completed successfully" else echo "Script failed with return code $exit_code" fi
In this example, the script checks the return code using the $?
variable and outputs "Script completed successfully" if the return code is 0.
How to Determine if a Bash Script Failed or Succeeded?
To determine if a Bash script failed or succeeded, you can check its exit status, return code, or use conditional statements to check the execution result of specific commands.
Here's an example of determining if a Bash script failed or succeeded using the exit status:
#!/bin/bash # Some commands and logic if [[ $? -eq 0 ]]; then echo "Script succeeded" else echo "Script failed" fi
In this example, the script checks the exit status using $?
and outputs "Script succeeded" if the exit status is 0.
You can also determine if a Bash script failed or succeeded using the return code:
#!/bin/bash # Some commands and logic exit_code=$? if [[ $exit_code -eq 0 ]]; then echo "Script succeeded" else echo "Script failed with return code $exit_code" fi
In this example, the script checks the return code using the $?
variable and outputs "Script succeeded" if the return code is 0.
Additionally, you can determine if a specific command or a block of commands succeeded or failed using conditional statements:
#!/bin/bash # Some commands and logic if command; then echo "Command succeeded" else echo "Command failed" fi
In this example, the script uses the if
statement to check the execution result of the command
and outputs "Command succeeded" if it succeeds.
Recommended Way to Handle Errors in a Bash Script
Handling errors in a Bash script is important to ensure the script behaves as expected and provides meaningful feedback to users. There are several recommended ways to handle errors in a Bash script.
One common approach is to use conditional statements to check the exit status or return code of commands and take appropriate actions based on the result. For example:
#!/bin/bash # Some commands and logic if command1; then echo "Command 1 succeeded" else echo "Command 1 failed" exit 1 fi if command2; then echo "Command 2 succeeded" else echo "Command 2 failed" exit 1 fi # Continue with the rest of the script
In this example, the script checks the exit status of command1
and command2
using conditional statements. If any of the commands fail, the script outputs an error message and exits with a non-zero exit status code.
Another approach is to use assertions or assertions-like statements to validate the success of specific commands or conditions. If the assertions fail, the script can raise an error or exit with a non-zero exit status code. For example:
#!/bin/bash assert_command_succeeded() { if ! "$@"; then echo "Assertion failed: $*" exit 1 fi } # Validate that the required dependencies are installed assert_command_succeeded command1 --version assert_command_succeeded command2 --version # Continue with the rest of the script
In this example, the script defines a function assert_command_succeeded
that checks if a command succeeds. If the command fails, the function outputs an error message and exits with a non-zero exit status code. The script then uses the assert_command_succeeded
function to validate the success of the required dependencies.
It is also recommended to provide informative error messages and handle exceptions gracefully. This can be achieved by using conditional statements, error handling functions, and logging mechanisms.
Related Article: How To Recursively Grep Directories And Subdirectories
Validate the Success of a Bash Script
To validate the success of a Bash script, you can use assertions or assertions-like statements to check if certain conditions are met during script execution. If the conditions are not met, you can raise an error or exit the script with a non-zero exit status code.
Here's an example of validating the success of a Bash script using assertions:
#!/bin/bash # Some commands and logic assert_command_succeeded() { if ! "$@"; then echo "Assertion failed: $*" exit 1 fi } # Validate that the required dependencies are installed assert_command_succeeded command1 --version assert_command_succeeded command2 --version # Continue with the rest of the script
In this example, the script defines a function assert_command_succeeded
that checks if a command succeeds. If the command fails, the function outputs an error message and exits with a non-zero exit status code. The script then uses the assert_command_succeeded
function to validate the success of the required dependencies.
Built-in Functions in Bash to Check if a Script Was Successful
Bash provides built-in functions that can be used to check if a script was successful. These functions allow you to handle errors, check the exit status or return code, and perform various checks during script execution.
One such built-in function is set -e
, which enables the shell's exit-on-error option. When this option is enabled, the shell will exit immediately if any command in the script fails.
Here's an example of using the set -e
option to check if a script was successful:
#!/bin/bash set -e # Some commands and logic echo "Script executed successfully"
In this example, the set -e
option is used to enable the exit-on-error option. If any command in the script fails, the script will exit immediately.
Another built-in function is trap
, which allows you to execute a command or script when a specific signal is received. This can be used to handle errors and perform cleanup actions in case of failure.
Here's an example of using the trap
function to check if a script was successful:
#!/bin/bash # Some commands and logic on_error() { echo "Script failed" exit 1 } trap on_error ERR # Continue with the rest of the script
In this example, the trap
function is used to call the on_error
function when an error occurs. The on_error
function outputs an error message and exits with a non-zero exit status code.
These are just a few examples of the built-in functions in Bash that can be used to check if a script was successful. There are many other functions and techniques available that can help you handle errors and validate the success of your Bash scripts.
Best Practices for Checking the Success of a Bash Script
When it comes to checking the success of a Bash script, there are several best practices you can follow to ensure your scripts are robust and reliable. These best practices include using proper error handling, validating dependencies, logging errors, and providing meaningful feedback to users.
Here are some best practices for checking the success of a Bash script:
1. Use conditional statements or the if
statement to check the exit status or return code of commands and take appropriate actions based on the result.
2. Validate the success of required dependencies before executing the main logic of your script. This can be done using assertions or assertions-like statements to check if the dependencies are installed and accessible.
3. Implement proper error handling by using conditional statements, error handling functions, or the set -e
option to handle errors and failures gracefully.
4. Log errors and provide informative error messages to help with troubleshooting and debugging. You can redirect error output to a log file or use a logging framework to handle error logging.
5. Validate the success of specific commands or conditions using assertions or assertions-like statements. If the assertions fail, raise an error or exit the script with a non-zero exit status code.
6. Test your Bash scripts thoroughly to ensure they handle errors and failures correctly. Use test cases that cover different scenarios and edge cases to verify the behavior of your scripts.
Additional Resources
- Exit status or return code of a successful bash script