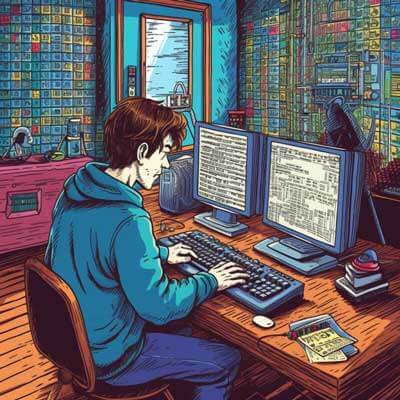
Table of Contents
What is the difference between a line break and a carriage return?
In the context of text files, a line break and a carriage return are two different characters used to indicate the end of a line.
A line break, also known as a newline character, is represented by the ASCII character code 10 (decimal) or 0x0A (hexadecimal). It is used to mark the end of a line and move the cursor to the beginning of the next line. On Unix-like systems, including Linux, a line break is represented by the character \n
.
A carriage return, also known as a CR character, is represented by the ASCII character code 13 (decimal) or 0x0D (hexadecimal). It is used to move the cursor to the beginning of the current line without advancing to the next line. On Unix-like systems, including Linux, a carriage return is represented by the character \r
.
In modern text files, a line break is typically used to mark the end of a line, while a carriage return is rarely used. However, in some legacy systems or when dealing with files created on different platforms, both characters may be present.
It's important to understand the difference between line breaks and carriage returns when working with text files, as different platforms and tools may interpret them differently.
Related Article: Displaying Images using Wget Bash Script in Linux
How do line endings differ between Windows and Linux?
Line endings, also known as newline characters, differ between Windows and Linux operating systems.
On Windows, the line ending consists of two characters: a carriage return (CR) followed by a line feed (LF). This is represented by the character sequence \r\n
. In ASCII, the carriage return is represented by the decimal value 13 (0x0D) and the line feed is represented by the decimal value 10 (0x0A). Together, they indicate the end of a line on Windows.
On Linux, Unix, and macOS, the line ending consists of a single character: a line feed (LF). This is represented by the character \n
, and in ASCII, it is represented by the decimal value 10 (0x0A). The line feed character is used to mark the end of a line on these platforms.
Because of the difference in line endings, text files created on Windows may not be correctly interpreted on Linux and vice versa. When transferring files between Windows and Linux systems, it's important to convert the line endings appropriately to ensure proper compatibility.
What is the significance of line endings in shell scripting?
In shell scripting, the significance of line endings lies in the correct interpretation of commands by the shell.
The shell, such as the Bash shell used on Linux, interprets new lines as command separators. When a shell script is executed, the shell reads the script line by line, treating each line as a separate command or a logical group of commands.
The line ending determines when the shell should consider a line as a complete command and move on to the next line. Without the proper line ending, the shell may misinterpret the script's logic or produce syntax errors.
For example, consider the following script with incorrect line endings:
#!/bin/bash echo "Hello,"; echo "World!"
In this script, the echo
commands are written on the same line without any line endings. When executed, the shell will interpret the two echo
commands as separate commands, resulting in two lines of output.
Now, let's fix the line endings:
#!/bin/bash echo "Hello," echo "World!"
With the correct line endings, the shell will interpret the script as intended, executing each command separately and producing the desired output.
Therefore, the significance of line endings in shell scripting is crucial for proper command interpretation, script execution, and script functionality.
Does the execution of a bash script change if new lines are present?
No, the execution of a bash script does not change if new lines are present or not. The presence or absence of new lines does not affect the execution of individual commands or the overall behavior of the script.
Bash scripts are interpreted line by line, and the shell treats each line as a separate command or a logical group of commands, regardless of whether new lines are present or not.
Consider the following script without new lines:
#!/bin/bash echo "Hello,"; echo "World!"
In this script, the echo
commands are written on the same line without any new lines separating them. Despite this formatting, the shell will still interpret each echo
command as a separate command and execute them sequentially.
The output of this script will be the same as the script with proper new lines:
#!/bin/bash echo "Hello," echo "World!"
In both cases, the script will produce the output:
Hello, World!
Therefore, the execution of a bash script remains unchanged whether new lines are present or not. However, the presence of new lines improves the readability and maintainability of the script, making it easier to understand and modify.
Related Article: How to SCP to a Folder in Linux: Copy Folder Remote<>Local
How do new lines affect bash scripts?
New lines, also known as line breaks, are characters used to separate lines within a text file. In bash scripts, new lines play a crucial role in defining the structure and readability of the script.
New lines are used to separate commands and make the script more readable by humans. Each line typically contains a single command or a logical group of commands. Without proper new lines, the script can become difficult to understand and maintain.
For example, consider the following bash script without new lines:
#!/bin/bash echo "Hello,"; echo "World!"
In this script, the echo
commands are written on the same line without any new lines separating them. While this script is valid and will produce the same output as the previous example, it is harder to read and understand.
Using new lines to separate the commands improves the readability of the script:
#!/bin/bash echo "Hello," echo "World!"
In this updated script, each command is written on a separate line, making it easier to follow and modify.
What is the importance of new lines in bash scripts?
New lines are important in bash scripts for several reasons:
1. Readability: New lines enhance the readability of the script by providing visual separation between commands. They make the script easier to understand and maintain, especially when dealing with complex logic or long commands.
2. Modularity: New lines allow for the logical grouping of commands. Each line can represent a specific action or a step in a larger process. This modular structure improves the script's organization and makes it easier to modify or debug individual commands.
3. Error handling: New lines help in error handling and troubleshooting. When a script encounters an error, the line number where the error occurred is often provided in the error message. With properly formatted new lines, it becomes easier to locate and fix errors in the script.
4. Version control: New lines play a crucial role in version control systems, such as Git. By separating commands onto different lines, it becomes easier to track changes made to the script over time. Version control systems often highlight changes at the line level, making it easier to review and merge changes made by multiple contributors.
Overall, new lines improve the maintainability, readability, and modularity of bash scripts, leading to more efficient development and debugging processes.
How does Linux handle new lines in bash scripts?
Linux, as well as other Unix-like operating systems, treats new lines in bash scripts as command separators. When a bash script is executed, the shell reads the script line by line, treating each line as a separate command or a logical group of commands.
The shell interprets new lines as the end of a command and executes each command sequentially. It waits for a command to complete before moving on to the next line. This behavior allows for the proper execution of the script and ensures that each command is executed in the correct order.
It's important to note that Linux treats new lines as command separators, regardless of whether they are written explicitly or as a result of word wrapping. For example, the following script is interpreted the same way as the previous example:
#!/bin/bash echo "Hello," echo "World!"
Even if the text editor or terminal wraps the lines due to limited space, the shell still treats each line as a separate command.
What are some common issues with new lines in bash scripts?
While new lines are essential for the structure and readability of bash scripts, they can also introduce potential issues if not used correctly. Some common issues with new lines in bash scripts include:
1. Syntax errors: If new lines are not used properly, it can lead to syntax errors in the script. For example, forgetting to terminate a command with a new line or adding an extra new line where it shouldn't be can result in a syntax error when executing the script.
2. Misinterpretation of commands: In some cases, improper use of new lines can cause the shell to misinterpret commands. For example, if a command is split across multiple lines without using proper line continuation techniques, the shell may treat each line as a separate command, resulting in unexpected behavior.
3. Word wrapping issues: When editing bash scripts in text editors, word wrapping can sometimes cause issues with new lines. If the editor automatically wraps lines without considering the command structure, it can break the script's logic and result in syntax errors or unexpected behavior.
To avoid these issues, it's important to follow best practices when using new lines in bash scripts. This includes properly terminating commands with new lines, using line continuation techniques when needed, and ensuring that word wrapping in text editors does not interfere with the script's structure.
Related Article: How to Use Getopts in Bash: A Practical Example
How can I remove new lines from a text file in Linux?
In some cases, you may want to remove new lines from a text file in Linux. This can be useful when processing files line by line or when you want to concatenate multiple lines into a single line.
There are several ways to remove new lines from a text file in Linux, depending on the specific requirements. Here are two common approaches:
1. Using the tr
command: The tr
command is used to translate or delete characters in a text stream. By specifying the new line character as the character to be deleted, you can remove all new lines from a file. Here's an example:
$ cat file.txt Line 1 Line 2 Line 3 $ tr -d '\n' < file.txt Line 1Line 2Line 3
In this example, the cat
command is used to display the contents of the file "file.txt". The tr
command with the -d
option is then used to delete the new line character (\n
) from the input stream, which is redirected from the file. The result is a single line without any new lines.
2. Using the sed
command: The sed
command is a useful stream editor that can perform various text transformations. By using the sed
command with a specific pattern, you can remove new lines from a file. Here's an example:
$ cat file.txt Line 1 Line 2 Line 3 $ sed ':a;N;$!ba;s/\n//g' file.txt Line 1Line 2Line 3
In this example, the cat
command is used to display the contents of the file "file.txt". The sed
command is then used with a specific pattern (:a;N;$!ba;s/\n//g
) to remove all new lines from the input stream. The result is a single line without any new lines.
These are just two examples of removing new lines from a text file in Linux. Depending on the specific requirements and the tools available, there may be other approaches to achieve the same result.
Additional Resources
- Bash Scripting Tutorial for Beginners