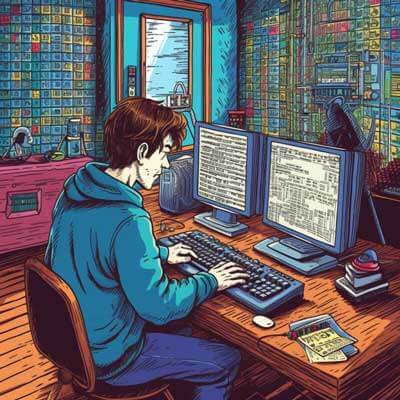
Table of Contents
What is the keyboard interrupt signal in Linux?
In Linux, the keyboard interrupt signal is a signal sent to a process when the user presses the CTRL+C key combination. By default, when a process receives the keyboard interrupt signal, it terminates and returns control to the command prompt.
The keyboard interrupt signal has a signal number of 2 and is represented by the SIGINT
constant. It is one of the many signals defined by the Linux operating system to communicate with processes and manage their execution.
Related Article: Making Bash Scripts Executable with Chmod in Linux
How do I stop a loop in a shell script?
To stop a loop in a shell script, you can utilize the keyboard interrupt signal (SIGINT
) by trapping it within the script. By trapping the keyboard interrupt signal, you can define a custom action to be executed when the user presses CTRL+C, allowing you to gracefully stop the loop.
Here's an example of how to stop a loop using the keyboard interrupt signal in a bash script:
#!/bin/bash # Define a function to handle the keyboard interrupt signal function handle_interrupt { echo "Loop interrupted. Exiting..." exit 0 } # Trap the keyboard interrupt signal and associate it with the handle_interrupt function trap handle_interrupt SIGINT # Perform some operations within the loop while true; do # Perform some operations # Continue with the loop done
In this example, the script defines a function called handle_interrupt
that displays a message and exits when the keyboard interrupt signal is received. The trap
command is used to trap the SIGINT
signal and associate it with the handle_interrupt
function. This ensures that when the user presses CTRL+C, the handle_interrupt
function is executed and the loop is gracefully stopped.
What is the equivalent of CTRL+C in a bash script?
The equivalent of CTRL+C in a bash script is the keyboard interrupt signal (SIGINT
). When the user presses CTRL+C, the keyboard interrupt signal is sent to the running script, causing it to terminate and return control to the command prompt.
You can capture and handle the keyboard interrupt signal in a bash script using the trap
command. By defining a custom action to be executed when the keyboard interrupt signal is received, you can mimic the behavior of CTRL+C within your script.
How to break out of a loop in a bash script?
To break out of a loop in a bash script, you can use the break
statement. The break
statement is used to exit a loop prematurely, regardless of the loop condition. When the break
statement is encountered, the program control immediately exits the loop and continues with the next statement after the loop.
Here's an example of how to break out of a loop in a bash script:
#!/bin/bash # Perform some operations within the loop while true; do # Perform some operations # Check if the loop should be broken if [ condition ]; then break fi # Continue with the loop done # Continue with the script
In this example, the script enters a loop using the while true
statement. Within the loop, some operations are performed, and then a condition is checked. If the condition is true, indicating that the loop should be broken, the break
statement is executed, and the loop is exited. The program control then continues with the next statement after the loop.
Related Article: How to Limi Argument Inputs in Bash Scripts
How can I end a bash script with a keyboard interrupt?
To end a bash script with a keyboard interrupt, you can capture and handle the keyboard interrupt signal (SIGINT
) within the script. By defining a custom action to be executed when the keyboard interrupt signal is received, you can gracefully terminate the script when the user presses CTRL+C.
Here's an example of how to end a bash script with a keyboard interrupt:
#!/bin/bash # Define a function to handle the keyboard interrupt signal function handle_interrupt { echo "Script interrupted. Exiting..." exit 0 } # Trap the keyboard interrupt signal and associate it with the handle_interrupt function trap handle_interrupt SIGINT # Perform some operations # Continue with the script
In this example, the script defines a function called handle_interrupt
that displays a message and exits when the keyboard interrupt signal is received. The trap
command is used to trap the SIGINT
signal and associate it with the handle_interrupt
function. This ensures that when the user presses CTRL+C, the handle_interrupt
function is executed, and the script is gracefully terminated.
What command can I use to stop a bash script?
To stop a bash script, you can use the exit
command. The exit
command is used to terminate a script and return a specified exit code to the calling process. By convention, an exit code of 0 indicates success, while any non-zero exit code indicates an error or failure.
Here's an example of how to use the exit
command to stop a bash script:
#!/bin/bash # Perform some operations # Check if an error occurred if [ condition ]; then exit 1 fi # Continue with the script
In this example, the script performs some operations, and then checks a condition. If the condition is true, indicating an error occurred, the script exits with an exit code of 1. This signals to the calling process that the script encountered an error and should be stopped.
How do I gracefully exit a bash script?
To gracefully exit a bash script, you can use the exit
command to terminate the script and return a specified exit code to the calling process. By convention, an exit code of 0 indicates success, while any non-zero exit code indicates an error or failure.
Here's an example of how to gracefully exit a bash script:
#!/bin/bash # Perform some operations # Check if an error occurred if [ condition ]; then echo "An error occurred" exit 1 fi # Cleanup resources # Exit the script exit 0
In this example, the script performs some operations, and then checks a condition. If the condition is true, indicating an error occurred, the script displays an error message and exits with an exit code of 1. This signals to the calling process that the script encountered an error. After that, any necessary cleanup operations are performed, and the script exits with an exit code of 0 to indicate successful termination.
Is there a way to terminate a bash script with a keyboard shortcut?
Yes, you can terminate a bash script with a keyboard shortcut by pressing CTRL+C. When you press CTRL+C, the keyboard interrupt signal (SIGINT
) is sent to the running script, causing it to terminate and return control to the command prompt.
Related Article: How to Use Mkdir Only If a Directory Doesn't Exist in Linux
How do I exit a bash script?
To exit a bash script, you can use the exit
command. The exit
command is used to terminate a script and return a specified exit code to the calling process. By convention, an exit code of 0 indicates success, while any non-zero exit code indicates an error or failure.
Here's an example of how to use the exit
command in a bash script:
#!/bin/bash # Perform some operations # Check if an error occurred if [ $? -ne 0 ]; then echo "An error occurred" exit 1 fi # Continue with the script
In this example, the script performs some operations, and then checks the exit code of the previous command using the $?
variable. If the exit code is not equal to 0, indicating an error occurred, the script displays an error message and exits with an exit code of 1.
How can I terminate a loop in a bash script?
To terminate a loop in a bash script, you can use the break
statement. The break
statement is used to exit a loop prematurely, regardless of the loop condition. When the break
statement is encountered, the program control immediately exits the loop and continues with the next statement after the loop.
Here's an example of how to use the break
statement in a bash script:
#!/bin/bash while true; do # Perform some operations # Check if the loop should be terminated if [ condition ]; then break fi # Continue with the loop done
In this example, the script enters an infinite loop using the while true
statement. Within the loop, some operations are performed, and then a condition is checked. If the condition is true, indicating that the loop should be terminated, the break
statement is executed, and the loop is exited.
Additional Resources
- Bash Scripting Tutorial - Loops