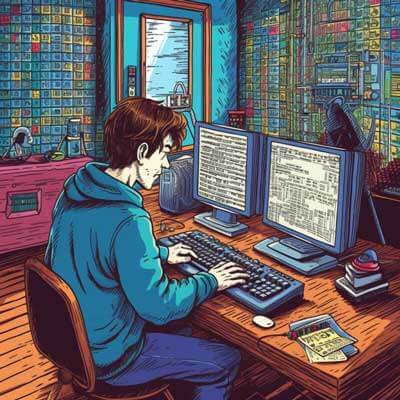
Table of Contents
Getting Started with Bash Programming
Bash (Bourne Again SHell) is a widely used command-line interpreter and scripting language for Linux and Unix systems. As a Linux sysadmin, having a solid understanding of Bash scripting is essential for automating tasks, managing systems, and troubleshooting issues. In this section, we will cover the basics of Bash programming and provide examples to help you get started.
Related Article: How to Read Text Files in Linux with Bash Scripts
Writing Your First Bash Script
To write a Bash script, create a new file with a .sh
extension. For example, let's create a script called hello.sh
that prints a simple greeting message:
#!/bin/bash echo "Hello, World!"
Save the file and make it executable using the chmod
command:
chmod +x hello.sh
Now, you can run the script by executing ./hello.sh
in the terminal. You should see the output Hello, World!
.
Variables and Data Types
Bash supports variables for storing and manipulating data. Variables in Bash are untyped, meaning you don't need to declare their data types explicitly. Here's an example that demonstrates variable usage in Bash:
#!/bin/bash name="John" age=30 echo "My name is $name and I am $age years old."
In this example, we define two variables name
and age
and assign them the values "John" and 30, respectively. The variables are then used in the echo
statement to display a message. Note the use of $
to access the variable values.
Conditional Statements
Conditional statements allow you to control the flow of your Bash script based on certain conditions. Bash provides several operators for performing conditional checks, such as -eq
(equal), -ne
(not equal), -lt
(less than), -gt
(greater than), etc. Here's an example that demonstrates the usage of conditional statements:
#!/bin/bash read -p "Enter a number: " num if [ $num -eq 0 ]; then echo "The number is zero." elif [ $num -gt 0 ]; then echo "The number is positive." else echo "The number is negative." fi
In this example, the user is prompted to enter a number. The script then checks if the number is zero, positive, or negative using the if
, elif
, and else
statements. The output message depends on the value entered by the user.
Related Article: Executing Configure Read from Bash Script in Linux
Loops
Loops allow you to iterate over a set of values or perform repetitive tasks in your Bash script. Bash provides different types of loops, such as for
, while
, and until
. Here's an example that demonstrates the usage of a for
loop:
#!/bin/bash for i in {1..5}; do echo "Iteration $i" done
In this example, the for
loop iterates over the values from 1 to 5 and displays a message for each iteration. You can customize the loop range and the statements inside the loop to suit your needs.
Functions
Functions allow you to encapsulate a set of commands into a reusable block of code. Bash functions are defined using the function
keyword or simply by their name followed by parentheses. Here's an example that demonstrates the usage of functions in Bash:
#!/bin/bash greet() { echo "Hello, $1!" } greet "John"
In this example, we define a function called greet
that takes a single argument. The function then echoes a greeting message with the argument value. Finally, we call the greet
function with the argument "John" to display the personalized greeting.
Input and Output
Bash provides various ways to handle input and output in your scripts. You can use the read
command to read input from the user, and the echo
command to display output. Here's an example that demonstrates input and output handling:
#!/bin/bash read -p "Enter your name: " name echo "Hello, $name!"
In this example, the read
command is used to prompt the user to enter their name, and the name is then displayed using the echo
command.
System Administration with Bash Scripting
As a Linux sysadmin, Bash scripting can greatly simplify your day-to-day tasks and help automate repetitive operations. In this section, we will explore how Bash scripting can be used for system administration purposes, such as managing users, performing backups, and monitoring system resources.
Related Article: How to Calculate the Sum of Inputs in Bash Scripts
Managing Users
Creating and managing user accounts is a common task for sysadmins. Bash scripting can make user management more efficient by automating the process. Here's an example that demonstrates how to create a new user using a Bash script:
#!/bin/bash read -p "Enter username: " username sudo useradd $username sudo passwd $username
In this example, the script prompts the user to enter a username. It then uses the useradd
command to create a new user and the passwd
command to set the user's password. Note the use of sudo
to run the commands with administrative privileges.
Performing Backups
Regularly backing up important data is crucial for system administrators. Bash scripting can be used to automate the backup process and ensure data integrity. Here's an example that demonstrates how to create a simple backup script using Bash:
#!/bin/bash backup_dir="/path/to/backup" source_dir="/path/to/source" timestamp=$(date +%Y%m%d%H%M%S) backup_file="backup_$timestamp.tar.gz" tar -czf $backup_dir/$backup_file $source_dir
In this example, the script defines the backup directory (backup_dir
) and the source directory (source_dir
). It then creates a timestamp for the backup file name using the date
command. Finally, it uses the tar
command to create a compressed archive of the source directory and saves it to the backup directory.
Monitoring System Resources
Monitoring system resources is essential for maintaining the performance and stability of Linux servers. Bash scripting can be used to gather system information and generate reports for analysis. Here's an example that demonstrates how to monitor CPU usage using a Bash script:
#!/bin/bash cpu_usage=$(top -b -n 1 | grep "Cpu(s)" | awk '{print $2}') echo "CPU Usage: $cpu_usage%"
In this example, the script uses the top
command with the -b
flag to run in batch mode and the -n 1
flag to get a single snapshot of system information. It then uses grep
and awk
to extract the CPU usage percentage from the top
output. The script finally displays the CPU usage percentage.
Exploring Scripting Languages for Automation
While Bash scripting is a useful tool for system administration, it's not the only scripting language available. In this section, we will explore other scripting languages commonly used for automation and compare them to Bash scripting.
Related Article: Displaying Memory Usage in Bash Scripts on Linux
Python
Python is a versatile and easy-to-learn programming language that is widely used for automation and scripting. It provides a rich set of libraries and supports various operating systems, making it an excellent choice for sysadmins. Here's an example that demonstrates how to create a simple Python script:
#!/usr/bin/env python name = input("Enter your name: ") print("Hello, " + name + "!")
In this example, the Python script prompts the user to enter their name and then displays a personalized greeting message.
Perl
Perl is another popular scripting language known for its text processing capabilities and useful regular expression support. It is often used for system administration tasks, such as log parsing and data manipulation. Here's an example that demonstrates how to create a simple Perl script:
#!/usr/bin/env perl print "Enter your name: "; $name = <STDIN>; chomp $name; print "Hello, $name!\n";
In this example, the Perl script prompts the user to enter their name, reads the input from STDIN
, removes the newline character using chomp
, and then displays a personalized greeting message.
Understanding the Linux Operating System
As a Linux sysadmin, having a deep understanding of the Linux operating system is crucial for effective system administration. In this section, we will cover the fundamental concepts and components of Linux that are relevant to Bash scripting.
Filesystem Hierarchy
The Linux filesystem follows a hierarchical structure, with the root directory (/
) at the top. Understanding the filesystem hierarchy is important for navigating and managing files and directories in Bash scripts. Here's an overview of some important directories in the Linux filesystem:
- /bin
: Contains essential user binaries (executable programs).
- /sbin
: Contains essential system binaries.
- /usr
: Contains user-installable programs and data.
- /etc
: Contains system configuration files.
- /var
: Contains variable data, such as logs, spool files, and temporary files.
- /home
: Contains user home directories.
- /root
: Home directory for the root user.
- /tmp
: Contains temporary files.
Related Article: Using a Watchdog Process to Trigger Bash Scripts in Linux
Processes and Services
Processes are running instances of programs in Linux. Understanding how processes work and how to manage them is essential for system administrators. Bash scripting can be used to control processes and manage services. Here are some important commands for working with processes in Bash scripts:
- ps
: Lists running processes.
- kill
: Terminates a process.
- service
: Controls system services.
- systemctl
: Controls systemd services (available on newer Linux distributions).
Permissions and Ownership
Linux uses a robust permission system to control access to files and directories. Understanding permissions and ownership is crucial for managing security and access control in Bash scripts. Here's an overview of the permission system in Linux:
- Read (r
): Allows reading files or viewing directory contents.
- Write (w
): Allows modifying files or creating new files in a directory.
- Execute (x
): Allows executing files or accessing directories.
Permissions are assigned to three categories of users: the owner of the file or directory, the group associated with the file or directory, and other users. Bash provides commands like chmod
and chown
to modify permissions and ownership in scripts.
Linux Administration using Bash Scripting
Bash scripting is a useful tool for automating Linux administration tasks. In this section, we will explore some common use cases where Bash scripting can be applied to simplify and streamline system administration.
Managing System Updates
Keeping Linux systems up to date with the latest security patches and software updates is crucial for maintaining system security and stability. Bash scripting can be used to automate the process of checking for and applying system updates. Here's an example of a Bash script that updates a Debian-based system:
#!/bin/bash sudo apt update sudo apt upgrade -y
In this example, the script uses the apt
package manager to update the system's package lists and upgrade installed packages. The -y
flag is used to automatically answer "yes" to any prompts during the upgrade process.
Related Article: Securing MySQL Access through Bash Scripts in Linux
Monitoring System Resources
Monitoring system resources is essential for identifying performance bottlenecks and ensuring optimal system operation. Bash scripting can be used to collect and analyze system resource data. Here's an example of a Bash script that monitors CPU and memory usage:
#!/bin/bash cpu_usage=$(top -b -n 1 | grep "Cpu(s)" | awk '{print $2}') memory_usage=$(free -m | awk '/Mem/ {print $3}') echo "CPU Usage: $cpu_usage%" echo "Memory Usage: $memory_usage MB"
In this example, the script uses the top
command to get CPU usage information and the free
command to get memory usage information. The script then displays the CPU and memory usage in the output.
Automating File Backups
Regularly backing up critical files and directories is crucial for data protection and disaster recovery. Bash scripting can be used to automate the process of creating backups and storing them in a remote location. Here's an example of a Bash script that creates a backup of a directory and uploads it to a remote server using rsync
:
#!/bin/bash source_dir="/path/to/source" backup_dir="/path/to/backup" remote_server="user@remote-server:/path/to/backup" timestamp=$(date +%Y%m%d%H%M%S) backup_file="backup_$timestamp.tar.gz" tar -czf $backup_dir/$backup_file $source_dir rsync -avz $backup_dir/$backup_file $remote_server
In this example, the script uses the tar
command to create a compressed archive of the source directory. It then uses rsync
to synchronize the backup file with a remote server. Replace user@remote-server:/path/to/backup
with the appropriate remote server details.
Essential Command Line Tools for SysAdmins
As a Linux sysadmin, being proficient in command line tools is essential for efficient system administration. In this section, we will cover some essential command line tools that are commonly used by sysadmins and can be integrated into Bash scripts.
grep
The grep
command is a useful tool for searching text patterns in files or command output. It supports regular expressions and various options for customizing search behavior. Here's an example that demonstrates how to use grep
to search for a specific pattern in a file:
grep "pattern" file.txt
In this example, replace "pattern" with the desired text pattern and "file.txt" with the name of the file to search in.
Related Article: How to Choose the Preferred Bash Shebang in Linux
sed
The sed
command is a stream editor that can perform various text transformations and substitutions. It is often used for automated editing and processing of text files. Here's an example that demonstrates how to use sed
to replace a specific string in a file:
sed -i 's/old/new/g' file.txt
In this example, replace "old" with the string to be replaced, "new" with the replacement string, and "file.txt" with the name of the file to modify. The -i
option is used to edit the file in-place.
awk
The awk
command is a versatile tool for processing text data and generating reports. It provides a useful scripting language for extracting and manipulating data. Here's an example that demonstrates how to use awk
to print specific columns from a file:
awk '{print $1, $3}' file.txt
In this example, replace "file.txt" with the name of the file to process. The awk
command will print the first and third columns of each line in the file.
curl
The curl
command is a versatile tool for making HTTP requests from the command line. It supports various protocols and options for sending and receiving data. Here's an example that demonstrates how to use curl
to retrieve the content of a web page:
curl https://example.com
In this example, replace "https://example.com" with the URL of the web page to retrieve. The curl
command will display the content of the web page in the terminal.
jq
The jq
command is a lightweight and flexible JSON processor for the command line. It provides a simple and useful syntax for querying and manipulating JSON data. Here's an example that demonstrates how to use jq
to extract a specific value from a JSON file:
jq '.key' file.json
In this example, replace ".key" with the desired JSON key path and "file.json" with the name of the JSON file to process. The jq
command will extract the corresponding value from the JSON data.
Related Article: How to Concatenate String Variables in Bash
Step-by-Step Scripting Tutorials
In this section, we will provide step-by-step tutorials to guide you through the process of creating useful Bash scripts for common system administration tasks.
Creating a User Management Script
In this tutorial, we will create a Bash script that simplifies user management tasks, such as creating, deleting, and modifying user accounts. The script will prompt the user for input and execute the corresponding actions. Here's the step-by-step guide:
Step 1: Create a new file called user_management.sh
:
touch user_management.sh
Step 2: Open the file in a text editor and add the shebang line at the beginning:
#!/bin/bash
Step 3: Define a function for creating a new user:
create_user() { read -p "Enter username: " username sudo useradd $username echo "User $username created." }
Step 4: Define a function for deleting an existing user:
delete_user() { read -p "Enter username: " username sudo userdel $username echo "User $username deleted." }
Step 5: Define a function for modifying an existing user's password:
change_password() { read -p "Enter username: " username sudo passwd $username echo "Password changed for user $username." }
Step 6: Define a function for displaying a menu and executing the selected action:
show_menu() { echo "User Management Script" echo "1. Create User" echo "2. Delete User" echo "3. Change Password" read -p "Enter your choice: " choice case $choice in 1) create_user ;; 2) delete_user ;; 3) change_password ;; *) echo "Invalid choice." ;; esac }
Step 7: Call the show_menu
function to start the script:
show_menu
Step 8: Save the file and make it executable:
chmod +x user_management.sh
Step 9: Run the script:
./user_management.sh
The script will display a menu with options to create, delete, or change the password of a user. Enter the corresponding number to execute the selected action.
Creating a Backup Script
In this tutorial, we will create a Bash script that automates the process of creating backups of specified directories and files. The script will compress the backup data and store it in a designated backup directory. Here's the step-by-step guide:
Step 1: Create a new file called backup.sh
:
touch backup.sh
Step 2: Open the file in a text editor and add the shebang line at the beginning:
#!/bin/bash
Step 3: Define the backup directory and source directory variables at the beginning of the script:
backup_dir="/path/to/backup" source_dir="/path/to/source"
Step 4: Create a timestamp variable to include in the backup file name:
timestamp=$(date +%Y%m%d%H%M%S)
Step 5: Define a function for creating the backup:
create_backup() { backup_file="backup_$timestamp.tar.gz" tar -czf $backup_dir/$backup_file $source_dir echo "Backup created: $backup_file" }
Step 6: Call the create_backup
function to start the backup process:
create_backup
Step 7: Save the file and make it executable:
chmod +x backup.sh
Step 8: Run the script:
./backup.sh
The script will create a compressed backup file with the current timestamp in the specified backup directory. Adjust the backup_dir
and source_dir
variables to match your setup.
Creating Shell Scripts in Linux
In this section, we will cover the process of creating shell scripts in Linux. Shell scripts are text files containing a series of commands that are executed by the shell interpreter. They provide a convenient way to automate tasks and perform complex operations. Here's a step-by-step guide to creating a shell script:
Step 1: Open a text editor of your choice. You can use any text editor available on your Linux system, such as Nano, Vim, or Emacs.
Step 2: Create a new file with a .sh
extension. For example, to create a script called myscript.sh
, use the following command:
touch myscript.sh
Step 3: Add the shebang line at the beginning of the file. The shebang line specifies the interpreter that should be used to execute the script. For Bash scripts, the shebang line should be #!/bin/bash
.
#!/bin/bash
Step 4: Write your script by adding commands and statements to the file. You can include any valid Bash command or construct in your script. Here's an example of a simple script that displays a greeting message:
#!/bin/bash echo "Hello, World!"
Step 5: Save the file and exit the text editor.
Step 6: Make the script executable using the chmod
command. This step is necessary to allow the script to be executed as a program. Use the following command to make the script executable:
chmod +x myscript.sh
Step 7: Run the script by executing its filename. For example, to run the myscript.sh
script, use the following command:
./myscript.sh
The script will be executed by the Bash interpreter, and the output will be displayed in the terminal.
Related Article: How to Handle New Lines in Bash Scripts
Commonly Used Bash Commands
As a Linux sysadmin, there are several commonly used Bash commands that you should be familiar with. These commands are essential for managing and troubleshooting Linux systems. In this section, we will cover some of the most frequently used Bash commands and provide examples of their usage.
ls
The ls
command is used to list directory contents. It provides information about files and directories in a specified directory or the current directory if no directory is specified. Here are some examples of using the ls
command:
ls # List files and directories in the current directory ls /path/to/directory # List files and directories in a specified directory ls -l # List files and directories in long format ls -a # List all files and directories, including hidden ones
cd
The cd
command is used to change the current directory. It allows you to navigate the filesystem and switch between directories. Here are some examples of using the cd
command:
cd # Change to the home directory cd /path/to/directory # Change to a specified directory cd .. # Change to the parent directory cd - # Change to the previous directory
pwd
The pwd
command is used to print the current working directory. It displays the full path of the current directory. Here's an example of using the pwd
command:
pwd # Print the current working directory
Related Article: How to Compare Two Files in Bash Script
mkdir
The mkdir
command is used to create directories. It allows you to create one or more directories with the specified names. Here's an example of using the mkdir
command:
mkdir directory # Create a directory with the specified name mkdir -p /path/to/dir # Create a directory and its parent directories if they don't exist
rm
The rm
command is used to remove files and directories. It allows you to delete one or more files or directories permanently. Here are some examples of using the rm
command:
rm file # Remove a file rm -r directory # Remove a directory and its contents recursively rm -rf directory # Remove a directory and its contents forcefully
cp
The cp
command is used to copy files and directories. It allows you to duplicate files or directories to a specified location. Here are some examples of using the cp
command:
cp file destination # Copy a file to the specified destination cp -r directory destination # Copy a directory and its contents to the specified destination
mv
The mv
command is used to move or rename files and directories. It allows you to change the location or name of a file or directory. Here are some examples of using the mv
command:
mv file destination # Move a file to the specified destination mv file newname # Rename a file to the specified new name
Related Article: Troubleshooting: Unable to Run Bash Script as Root in Linux
grep
The grep
command is used to search for patterns in files or command output. It allows you to filter and extract lines that match a specified pattern. Here are some examples of using the grep
command:
grep pattern file # Search for lines that match the specified pattern in a file command | grep pattern # Search for lines that match the specified pattern in the output of a command
chmod
The chmod
command is used to change the permissions of files and directories. It allows you to control access to files and directories for different users. Here's an example of using the chmod
command:
chmod +x script.sh # Add executable permission to a script file chmod 644 file # Set read and write permission for the owner, and read permission for others on a file
chown
The chown
command is used to change the ownership of files and directories. It allows you to change the user and group ownership of a file or directory. Here's an example of using the chown
command:
chown user:group file # Change the owner and group of a file
Automating Repetitive Tasks in Linux
As a Linux sysadmin, you often encounter repetitive tasks that can be time-consuming and error-prone if done manually. Bash scripting provides a useful tool for automating these tasks, saving you time and reducing the risk of human errors. In this section, we will explore some common repetitive tasks that can be automated using Bash scripts.
Related Article: How To Recursively Grep Directories And Subdirectories
Log Rotation
Log files can grow rapidly and consume a significant amount of disk space over time. To prevent this, log rotation is often implemented to compress and archive old log files while keeping the most recent logs. Bash scripting can be used to automate log rotation tasks, such as compressing and archiving logs based on certain criteria, like file size or age. Here's an example of a Bash script that performs log rotation:
#!/bin/bash log_dir="/var/log" archive_dir="/var/log/archive" max_size=10000 for log_file in $log_dir/*.log; do if [ -f "$log_file" ] && [ $(wc -c <"$log_file") -gt $max_size ]; then archive_file="$archive_dir/$(basename "$log_file").$(date +%Y%m%d%H%M%S).gz" gzip -c "$log_file" > "$archive_file" echo "Log file $log_file rotated and archived as $archive_file" > "$log_file" fi done
In this example, the script iterates over all log files in the specified directory ($log_dir
). It checks the file size using the wc
command and compresses the file using gzip
if it exceeds the specified maximum size ($max_size
). The compressed file is then saved in the archive directory ($archive_dir
) with the current timestamp. Finally, the script empties the original log file using the redirection operator (>
).
Data Backup
Regularly backing up critical data is crucial for disaster recovery and ensuring data integrity. Bash scripting can be used to automate data backup tasks, such as creating backups of specified directories and files, and transferring them to remote storage or cloud services. Here's an example of a Bash script that performs data backup:
#!/bin/bash source_dir="/path/to/source" backup_dir="/path/to/backup" remote_server="user@remote-server:/path/to/backup" timestamp=$(date +%Y%m%d%H%M%S) backup_file="backup_$timestamp.tar.gz" tar -czf $backup_dir/$backup_file $source_dir rsync -avz $backup_dir/$backup_file $remote_server
In this example, the script creates a compressed backup file using the tar
command and saves it in the specified backup directory ($backup_dir
). It then uses rsync
to synchronize the backup file with a remote server ($remote_server
). Adjust the source directory ($source_dir
), backup directory ($backup_dir
), and remote server details ($remote_server
) to match your setup.
System Health Monitoring
Monitoring system health is essential for identifying and resolving issues before they impact system performance or availability. Bash scripting can be used to automate system health monitoring tasks, such as checking CPU and memory usage, monitoring disk space, and tracking network connectivity. Here's an example of a Bash script that performs system health monitoring:
#!/bin/bash cpu_threshold=80 memory_threshold=80 disk_threshold=90 check_cpu_usage() { cpu_usage=$(top -b -n 1 | grep "Cpu(s)" | awk '{print $2}') if (( $(echo "$cpu_usage > $cpu_threshold" | bc -l) )); then echo "CPU usage is above the threshold: $cpu_usage%" fi } check_memory_usage() { memory_usage=$(free -m | awk '/Mem/ {print $3}') if (( $(echo "$memory_usage > $memory_threshold" | bc -l) )); then echo "Memory usage is above the threshold: $memory_usage MB" fi } check_disk_usage() { disk_usage=$(df -h / | awk '/\// {print $5}' | sed 's/%//') if (( $(echo "$disk_usage > $disk_threshold" | bc -l) )); then echo "Disk usage is above the threshold: $disk_usage%" fi } check_network_connectivity() { ping -c 1 google.com > /dev/null if [ $? -ne 0 ]; then echo "Network connectivity issue detected" fi } check_cpu_usage check_memory_usage check_disk_usage check_network_connectivity
In this example, the script defines functions to check CPU usage (check_cpu_usage
), memory usage (check_memory_usage
), disk usage (check_disk_usage
), and network connectivity (check_network_connectivity
). Each function uses appropriate commands to gather relevant system information and compares it against the specified thresholds. If the usage exceeds the threshold, an appropriate message is displayed.
Advantages of Bash Scripting for System Administration
Bash scripting offers numerous advantages for system administration tasks. It provides a flexible and efficient way to automate repetitive tasks, manage system configurations, and troubleshoot issues. Here are some key advantages of Bash scripting for system administration:
Related Article: How To Split A String On A Delimiter In Bash
Automation
Bash scripting allows sysadmins to automate repetitive tasks, such as user management, log rotation, data backup, and system monitoring. By writing scripts, sysadmins can save time and reduce the risk of human errors.
Customization
Bash scripting provides the flexibility to customize and tailor scripts according to specific system requirements. Sysadmins can write scripts that perform complex operations, process data, and integrate with other tools and systems.
Script Reusability
Bash scripts can be reused across different systems and environments, saving sysadmins the effort of rewriting scripts for similar tasks. Scripts can be easily shared and modified to suit different scenarios.
Rapid Prototyping
Bash scripting allows sysadmins to quickly prototype solutions and test ideas. The interpreted nature of Bash enables rapid development and iteration, making it an ideal choice for ad-hoc scripting tasks.
Related Article: How to Manipulate Quotes & Strings in Bash Scripts
Integration with Command Line Tools
Bash scripts can leverage existing command line tools and utilities to perform complex operations. Sysadmins can combine multiple commands, pipe output between them, and use command line options and arguments to achieve desired results.
Troubleshooting
Bash scripting can be a valuable tool for troubleshooting system issues. Sysadmins can write scripts to collect diagnostic information, analyze logs, and perform system checks to identify and resolve problems.
Portability
Bash scripting is supported on various Unix-like systems, including Linux, macOS, and BSD. Scripts written in Bash can be executed across different platforms with minimal modifications, providing portability and compatibility.
Overall, Bash scripting empowers sysadmins with a useful toolset to streamline system administration tasks, increase productivity, and maintain system stability and security.
Passing Arguments to a Bash Script
Bash scripts can accept arguments from the command line, allowing sysadmins to provide inputs and customize script behavior. In this section, we will explore how to pass arguments to a Bash script and access them within the script.
To pass arguments to a Bash script, arguments are specified after the script name when executing the script. The arguments are then accessible within the script using special variables.
Here's an example of a Bash script that accepts two arguments and displays them:
#!/bin/bash echo "First argument: $1" echo "Second argument: $2"
In this example, the script uses the special variables $1
and $2
to access the first and second arguments, respectively. $1
represents the first argument, $2
represents the second argument, and so on. Note that the script name itself is considered as $0
.
To pass arguments to the script, invoke the script with the desired arguments separated by spaces:
./script.sh arg1 arg2
In this example, the script is executed with two arguments: arg1
and arg2
. The script will display the values of the arguments in the output.
Bash also provides other special variables to access command line arguments, such as $@
(all arguments), $#
(number of arguments), and $*
(all arguments as a single string). These variables can be useful for handling variable numbers of arguments or iterating over all arguments in a loop.
Here's an example of a Bash script that demonstrates the usage of these special variables:
#!/bin/bash echo "Number of arguments: $#" echo "All arguments: $@" echo "All arguments as a single string: $*" for arg in "$@"; do echo "Argument: $arg" done
In this example, the script displays the number of arguments using $#
, all arguments using $@
, and all arguments as a single string using $*
. It then iterates over all arguments using a for
loop and displays each argument individually.
Related Article: How to Check If a File Does Not Exist in Bash
Difference Between Shell Script and Programming Language
Shell scripting and programming languages are both used for writing scripts and automating tasks, but they have distinct characteristics and purposes. In this section, we will explore the difference between shell scripting and programming languages.
Shell Scripting
Shell scripting refers to writing scripts using a shell interpreter, such as Bash, to automate command line tasks and system administration operations. Shell scripts are typically written for specific operating systems or shells and rely on the shell's built-in commands and utilities.
Shell scripts are primarily used for executing system commands, managing files and directories, and performing system administration tasks. They are well-suited for automating repetitive tasks, processing text-based data, and integrating with command line tools.
Shell scripting is often used in Linux and Unix environments, where the shell is the primary interface for interacting with the operating system. Shell scripts are interpreted at runtime and executed line by line by the shell interpreter.
Programming Languages
Programming languages, on the other hand, are more general-purpose and can be used for a wide range of applications, including web development, software engineering, data analysis, and artificial intelligence. Programming languages provide a rich set of features, such as data types, control structures, functions, and libraries, to facilitate complex computations and application development.
Programming languages are designed to be more expressive and flexible, allowing developers to create sophisticated software systems. They often provide features like object-oriented programming, modularization, and support for various data structures and algorithms.
Programming languages are typically compiled or interpreted by dedicated compilers or interpreters, which convert the source code into machine-readable instructions or execute it in a virtual machine.
Key Differences
The key differences between shell scripting and programming languages are:
- Scope: Shell scripting is focused on system administration tasks and command line operations, whereas programming languages have a broader scope and can be used for various applications and domains.
- Syntax: Shell scripting uses a simplified syntax, often resembling the command line syntax, while programming languages have more complex syntax with strict rules and conventions.
- Features: Shell scripting relies on the built-in commands and utilities of the shell, whereas programming languages provide a wide range of features, such as data types, control structures, functions, and libraries.
- Performance: Shell scripts are typically slower than compiled programming languages due to their interpreted nature. Programming languages, especially compiled ones, can offer better performance for computationally intensive tasks.
- Portability: Shell scripts are often specific to a particular shell or operating system, while programming languages can be more portable and run on various platforms with minimal modifications.
Related Article: Making Bash Scripts Executable with Chmod in Linux
Best Practices for Writing Bash Scripts
Writing clean, reliable, and maintainable Bash scripts is essential for effective system administration. In this section, we will cover some best practices and guidelines to follow when writing Bash scripts.
Use a Descriptive Shebang Line
Begin your script with a shebang line that specifies the interpreter to be used. Use #!/bin/bash
for Bash scripts or #!/usr/bin/env bash
for improved portability.
Enable Error Handling
Use set -euo pipefail
at the beginning of your script to enable strict error handling. This ensures that any command failure or undefined variable will cause the script to exit immediately.
Use Meaningful Variable Names
Choose descriptive variable names that reflect the purpose and content of the data they represent. Avoid using single-letter variable names or ambiguous names.
Related Article: How to Check If a File Copy is Completed with a Bash Script
Quote Variables
Always quote variables to avoid word splitting and globbing issues. Use double quotes ("$variable"
) unless you specifically need to preserve literal values or allow globbing ('${variable}'
).
Indentation and Formatting
Use consistent indentation and formatting to improve readability. Indent your code with spaces (not tabs) and choose a consistent number of spaces for each level of indentation (e.g., 4 spaces).
Comment your Code
Include comments to explain the purpose and functionality of your code. Commenting helps other developers (including your future self) understand your script and troubleshoot issues.
Handle User Input Gracefully
Validate and sanitize user input to prevent unexpected behavior or security vulnerabilities. Use conditional statements and input validation techniques to handle user input gracefully.
Related Article: Using Variables in If Statements in Bash Scripts
Avoid Hardcoding Paths
Avoid hardcoding absolute paths in your script, as they may vary between systems. Use variables or environment variables to store and reference paths, allowing for greater flexibility.
Use Functions for Reusability
Encapsulate reusable portions of your code into functions to improve modularity and reusability. Functions help keep your script organized and make it easier to maintain and update.
Use Exit Codes
Use exit codes (exit 0
for success, exit 1
for failure) to indicate the outcome of your script. This allows other scripts or processes to handle the result appropriately.
Testing and Debugging
Test your script thoroughly before deploying it in a production environment. Use debugging techniques, such as echoing variables or using the set -x
option, to troubleshoot issues.
Related Article: Tutorial on Traceroute in Linux
Document Dependencies
Include a section in your script's documentation that lists any dependencies or prerequisites required for the script to run. This helps other sysadmins understand and prepare the required environment.
Useful Command Line Tools for SysAdmins
As a Linux sysadmin, having a good set of command line tools in your toolbox is essential for efficient system administration. In this section, we will explore some useful command line tools that can help you manage and troubleshoot Linux systems.
htop
htop
is an interactive process viewer that provides real-time monitoring of system resources. It displays a color-coded and user-friendly interface, making it easier to identify resource-intensive processes and troubleshoot performance issues. To install htop
, use the package manager of your Linux distribution (e.g., sudo apt install htop
for Debian-based systems).
tmux
tmux
is a terminal multiplexer that allows you to create and manage multiple terminal sessions within a single window. It provides features like session management, window splitting, and session sharing, making it easier to organize and work with multiple terminal sessions simultaneously. To install tmux
, use the package manager of your Linux distribution (e.g., sudo apt install tmux
for Debian-based systems).
Related Article: Creating Incremental Backups with Bash Scripts in Linux
ncdu
ncdu
(NCurses Disk Usage) is a disk usage analyzer that provides a simple and intuitive interface for analyzing disk space usage. It allows you to navigate through directories, view disk usage statistics, and identify space-consuming files and directories. To install ncdu
, use the package manager of your Linux distribution (e.g., sudo apt install ncdu
for Debian-based systems).
rsync
rsync
is a useful file synchronization and transfer tool that can be used to efficiently copy and synchronize files between different systems. It supports various options for controlling the copying process, preserving file attributes, and synchronizing remote directories. To install rsync
, use the package manager of your Linux distribution (e.g., sudo apt install rsync
for Debian-based systems).
grep
grep
is a versatile tool for searching and filtering text patterns in files or command output. It supports regular expressions and various options for customizing search behavior. grep
is invaluable for analyzing log files, searching for specific patterns, and extracting information. It is available by default on most Linux distributions.
awk
awk
is a useful text processing and pattern scanning tool that provides a simple and expressive language for manipulating structured text data. It allows you to extract and manipulate columns, perform calculations, and generate reports. awk
is a great tool for processing log files, analyzing data, and transforming text. It is available by default on most Linux distributions.
Related Article: Tutorial: Functions in Bash Scripts on Linux
Additional Resources
- Writing Your First Shell Script