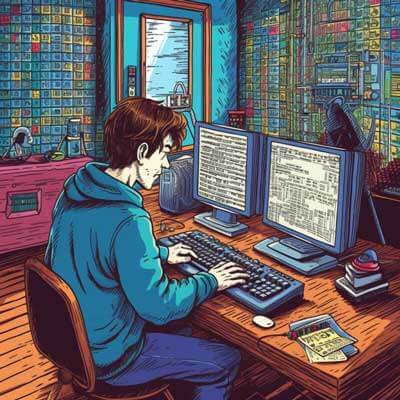
Table of Contents
Checking if a Bash Script is Running
To check if a Bash script is running, you can use various methods and techniques. In this section, we will explore some of the most commonly used approaches.
Related Article: Using SSH to Connect to a Remote Server in Linux
Method 1: Checking Process Status
One way to determine if a Bash script is running is by checking the process status using the ps
command. The ps
command displays information about active processes on your system. By filtering the output, you can easily identify if your script is currently running.
Here's an example of how you can use the ps
command to check if a Bash script named myscript.sh
is running:
ps -ef | grep myscript.sh
This command will list all the processes running on your system and filter the output to display only the ones that contain the name "myscript.sh". If the script is running, you will see the corresponding process ID (PID) and other information.
Method 2: Checking for a Running Process ID
Another way to determine if a Bash script is running is by checking for a running process ID (PID). Each running process on Linux is assigned a unique PID that can be used to identify it.
You can use the pgrep
command to search for a process by name and retrieve its PID. Here's an example of how you can use pgrep
to check if a Bash script named myscript.sh
is running:
pgrep -f myscript.sh
The -f
option allows pgrep
to match against the full argument list of the process, including the script name. If the script is running, the command will display its PID.
How to Determine the Status of a Bash Script
Once you have determined that your Bash script is running, you may want to check its status. The status of a running script can provide valuable information about its execution progress or any errors that may have occurred.
Related Article: Executing Scripts in Linux Without Bash Command Line
Method 1: Using the ps
Command
The ps
command can also be used to determine the status of a running Bash script. By combining the ps
command with the process ID (PID) of the script, you can obtain detailed information about its status.
Here's an example of how you can use the ps
command to determine the status of a running Bash script with PID 12345:
ps -p 12345 -o stat
This command will display the status of the process with PID 12345. The status can be one of several values, such as "R" for running, "S" for sleeping, "T" for stopped, or "Z" for a zombie process.
Method 2: Checking the Exit Code
Another way to determine the status of a Bash script is by checking its exit code. The exit code is a numeric value that indicates the success or failure of the script's execution. A value of 0 typically indicates success, while non-zero values indicate various types of errors.
You can check the exit code of a Bash script immediately after it has finished executing by using the special variable $?
. Here's an example:
./myscript.sh echo $?
In this example, the ./myscript.sh
command executes the script, and echo $?
displays the exit code. If the exit code is 0, the script executed successfully. Otherwise, the exit code will indicate the type of error that occurred.
Executing a Bash Script in the Foreground
When you execute a Bash script in the foreground, it means that the script will run in the current shell session, and the shell will wait for the script to finish before allowing you to enter new commands.
To execute a Bash script in the foreground, you can simply run the script using the ./
notation followed by the script name. Here's an example:
./myscript.sh
In this example, the script named myscript.sh
will be executed in the foreground. The shell will wait for the script to finish before returning control to the user.
Running a Bash Script in the Background
Running a Bash script in the background allows the script to execute independently of the current shell session. This means that you can start the script and continue working on other tasks without waiting for the script to finish.
To run a Bash script in the background, you can use the &
symbol at the end of the command. Here's an example:
./myscript.sh &
In this example, the script named myscript.sh
will be executed in the background. The shell will immediately return control to the user, allowing them to continue working on other tasks.
Related Article: Applying Patches with Bash Scripts in Linux
The Difference Between Running a Bash Script in the Background and in the Foreground
When you run a Bash script in the foreground, the script will execute in the current shell session, and the shell will wait for the script to finish before allowing you to enter new commands. This means that you cannot continue working on other tasks until the script has completed.
On the other hand, running a Bash script in the background allows the script to execute independently of the current shell session. The shell will immediately return control to the user, allowing them to continue working on other tasks while the script is running.
The main difference between running a script in the background and in the foreground is the level of interactivity and the ability to multitask. Running a script in the foreground is useful when you need to closely monitor its progress or provide input during its execution. Running a script in the background is useful when you want to start a long-running task and continue working on other tasks simultaneously.
Stopping a Running Bash Script
If you need to stop a running Bash script, there are several methods you can use depending on the situation. Here are a few common approaches:
Method 1: Using Ctrl+C
If you are running a Bash script in the foreground and want to stop it, you can use the Ctrl+C keyboard shortcut. Pressing Ctrl+C will send a SIGINT signal to the script, causing it to terminate.
Here's an example:
./myscript.sh
While the script is running, you can press Ctrl+C to stop it.
Method 2: Using the kill
Command
If you need to stop a Bash script that is running in the background, you can use the kill
command to send a signal to the script's process ID (PID). The most commonly used signal to terminate a process is SIGTERM.
Here's an example of how you can use the kill
command to stop a script with PID 12345:
kill 12345
This command will send a SIGTERM signal to the process with PID 12345, causing the script to terminate.
Related Article: Executing Configure Read from Bash Script in Linux
Viewing the Output of a Running Bash Script
When a Bash script is running, it may produce output that can be useful for monitoring its progress or troubleshooting any issues. There are several ways to view the output of a running script, depending on whether the script is running in the foreground or the background.
Method 1: Viewing Output in the Foreground
If you are running a Bash script in the foreground, the output will be displayed directly in the terminal as the script executes. You can simply observe the output as it appears on the screen.
Here's an example:
./myscript.sh
As the script runs, any output it produces will be displayed in the terminal.
Method 2: Redirecting Output to a File
If you want to save the output of a running Bash script to a file for later analysis, you can redirect the output using the >
symbol. This will redirect the standard output (stdout) of the script to the specified file.
Here's an example:
./myscript.sh > output.txt
In this example, the output of the script will be redirected to a file named output.txt
. You can then open the file using a text editor to view the output.
Obtaining the Process ID of a Running Bash Script
The process ID (PID) of a running Bash script can be useful for various purposes, such as monitoring the script's execution or sending signals to the script's process. There are several methods you can use to obtain the PID of a running script.
Related Article: Using Variables in If Statements in Bash Scripts
Method 1: Using the pgrep
Command
The pgrep
command allows you to search for a process by name and retrieve its PID. You can use pgrep
to obtain the PID of a running Bash script by specifying its name as the argument.
Here's an example:
pgrep -f myscript.sh
This command will search for a process that matches the full argument list of the script, including the script name. If the script is running, the command will display its PID.
Method 2: Using the $!
Special Variable
When you run a Bash script in the background, you can obtain its PID using the $!
special variable. This variable stores the PID of the most recently executed background command.
Here's an example:
./myscript.sh & echo $!
In this example, the ./myscript.sh &
command runs the script in the background, and echo $!
displays its PID. The PID will be printed on the screen.
Using Command Line to Check if a Bash Script is Running
The command line provides various tools and techniques that you can use to check if a Bash script is running. In this section, we will explore some of the most commonly used command line methods.
Method 1: Using the ps
Command
The ps
command allows you to view information about active processes on your system. By filtering the output, you can easily determine if a Bash script is running.
Here's an example:
ps -ef | grep myscript.sh
This command will list all the processes running on your system and filter the output to display only the ones that contain the name "myscript.sh". If the script is running, you will see the corresponding process ID (PID) and other information.
Related Article: How to Extract Numbers from Strings in Bash
Method 2: Checking for a Running Process ID
You can also check if a Bash script is running by searching for its process ID (PID). Each running process on Linux is assigned a unique PID that can be used to identify it.
Here's an example:
pgrep -f myscript.sh
The -f
option allows pgrep
to match against the full argument list of the process, including the script name. If the script is running, the command will display its PID.
Checking if a Bash Script is Running in the Terminal
To check if a Bash script is running in the terminal, you can use various methods and techniques. In this section, we will explore some of the most commonly used approaches.
Method 1: Using the jobs
Command
The jobs
command allows you to view the status of jobs running in the current shell session. A job can be a command or a script that is executing in the background or the foreground.
Here's an example:
jobs
This command will display a list of jobs currently running in the terminal. If your Bash script is running, you will see its status and job number in the output.
Method 2: Checking the Exit Code
Another way to determine if a Bash script is running in the terminal is by checking its exit code. The exit code is a numeric value that indicates the success or failure of the script's execution. A value of 0 typically indicates success, while non-zero values indicate various types of errors.
You can check the exit code of a Bash script immediately after it has finished executing by using the special variable $?
. Here's an example:
./myscript.sh echo $?
In this example, the ./myscript.sh
command executes the script, and echo $?
displays the exit code. If the exit code is 0, the script executed successfully. Otherwise, the exit code will indicate the type of error that occurred.
Related Article: Exploring Local Scope of Bash Variables in Linux Scripts
Determining if a Bash Script is Running in the Background
To determine if a Bash script is running in the background, you can use various methods and techniques. In this section, we will explore some of the most commonly used approaches.
Method 1: Using the jobs
Command
The jobs
command allows you to view the status of jobs running in the current shell session. A job can be a command or a script that is executing in the background or the foreground.
Here's an example:
jobs
This command will display a list of jobs currently running in the terminal. If your Bash script is running in the background, you will see its status and job number in the output.
Method 2: Checking the Process ID
Another way to determine if a Bash script is running in the background is by checking its process ID (PID). When you run a script in the background, it is assigned a unique PID that can be used to identify it.
Here's an example:
pgrep -f myscript.sh
The -f
option allows pgrep
to match against the full argument list of the process, including the script name. If the script is running in the background, the command will display its PID.
Checking the Status of a Bash Script Using Command Line
To check the status of a Bash script using the command line, you can use various methods and techniques. In this section, we will explore some of the most commonly used approaches.
Related Article: Troubleshooting: Unable to Save Bash Scripts in Vi on Linux
Method 1: Using the ps
Command
The ps
command allows you to view information about active processes on your system. By filtering the output, you can easily determine the status of a Bash script.
Here's an example:
ps -p 12345 -o stat
This command will display the status of the process with PID 12345. The status can be one of several values, such as "R" for running, "S" for sleeping, "T" for stopped, or "Z" for a zombie process.
Method 2: Checking the Exit Code
Another way to determine the status of a Bash script is by checking its exit code. The exit code is a numeric value that indicates the success or failure of the script's execution. A value of 0 typically indicates success, while non-zero values indicate various types of errors.
You can check the exit code of a Bash script immediately after it has finished executing by using the special variable $?
. Here's an example:
./myscript.sh echo $?
In this example, the ./myscript.sh
command executes the script, and echo $?
displays the exit code. If the exit code is 0, the script executed successfully. Otherwise, the exit code will indicate the type of error that occurred.
Understanding the Difference Between Running a Bash Script in the Background and in the Foreground on Linux
The difference between running a Bash script in the background and in the foreground on Linux is the same as the difference between running a Bash script in the background and in the foreground in general.
When you run a Bash script in the foreground on Linux, the script will execute in the current shell session, and the shell will wait for the script to finish before allowing you to enter new commands. This means that you cannot continue working on other tasks until the script has completed.
On the other hand, running a Bash script in the background on Linux allows the script to execute independently of the current shell session. The shell will immediately return control to the user, allowing them to continue working on other tasks while the script is running.
The main difference between running a script in the background and in the foreground is the level of interactivity and the ability to multitask. Running a script in the foreground is useful when you need to closely monitor its progress or provide input during its execution. Running a script in the background is useful when you want to start a long-running task and continue working on other tasks simultaneously.
Stopping a Running Bash Script on Linux
To stop a running Bash script on Linux, you can use the same methods as stopping a running Bash script in general. There are several approaches you can take depending on the situation.
Related Article: Setting up an Intrusion Detection System on Linux
Method 1: Using Ctrl+C
If you are running a Bash script in the foreground on Linux and want to stop it, you can use the Ctrl+C keyboard shortcut. Pressing Ctrl+C will send a SIGINT signal to the script, causing it to terminate.
Here's an example:
./myscript.sh
While the script is running, you can press Ctrl+C to stop it.
Method 2: Using the kill
Command
If you need to stop a Bash script that is running in the background on Linux, you can use the kill
command to send a signal to the script's process ID (PID). The most commonly used signal to terminate a process is SIGTERM.
Here's an example of how you can use the kill
command to stop a script with PID 12345 on Linux:
kill 12345
This command will send a SIGTERM signal to the process with PID 12345, causing the script to terminate.
Additional Resources
- Linux Journal - Monitoring running processes in Linux