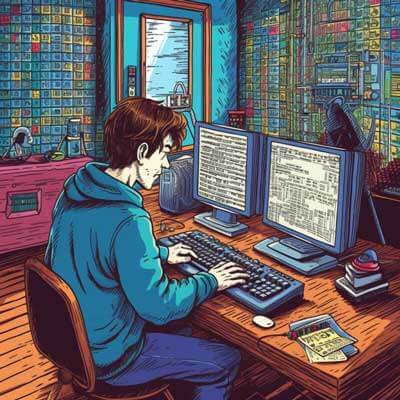
Table of Contents
The 'configure' command is a commonly used utility in Linux systems that helps in automatically configuring software source code to adapt to the specific system it is being installed on. This command is typically used when building and installing software from source code.
The 'configure' command reads a file called 'configure.ac' or 'configure.in' that contains instructions and parameters for the build process. It analyzes the system's environment and generates a 'Makefile' that can be used to compile and install the software.
To execute the 'configure' command, navigate to the source code directory and run the following command:
./configure
The 'configure' command also accepts various options and arguments that can be used to customize the build process. For example, you can specify the installation prefix, enable or disable certain features, or set environment variables. Here's an example:
./configure --prefix=/usr/local --enable-feature
Using the 'read' Command in Bash Scripts
The 'read' command in Bash allows you to read a line from standard input or a file and assign it to a variable. This command is useful when you want to prompt the user for input or read values from a file.
Here's a basic example of using the 'read' command to prompt the user for their name and store it in a variable:
#!/bin/bash echo "What is your name?" read name echo "Hello, $name!"
In this example, the 'read' command reads a line of input from the user and assigns it to the variable 'name'. The value of 'name' is then printed using the 'echo' command.
You can also use the 'read' command to read values from a file. For example, if you have a file called 'data.txt' with the following contents:
John Doe Jane Smith
You can read each line of the file and perform some action using a loop:
#!/bin/bash while read line; do echo "Hello, $line!" done < data.txt
In this example, the 'read' command is used within a 'while' loop to read each line of the file 'data.txt'. The variable 'line' is assigned the value of each line, and the 'echo' command prints a greeting for each line.
Related Article: How to Sync Local and Remote Directories with Rsync
Common Linux Commands for Scripting
When writing Bash scripts in Linux, it is important to be familiar with some common Linux commands that can be used within scripts. These commands help perform various operations like file manipulation, text processing, and system administration.
Here are a few commonly used Linux commands for scripting:
1. 'ls': List files and directories in a directory.
ls /path/to/directory
2. 'cp': Copy files and directories.
cp source_file destination_file
3. 'mv': Move or rename files and directories.
mv old_name new_name
4. 'rm': Remove files and directories.
rm file_name
5. 'grep': Search for a specific pattern in files.
grep pattern file_name
6. 'sed': Stream editor for text manipulation.
sed 's/find/replace/' file_name
7. 'awk': Text processing and pattern matching.
awk '{print $1}' file_name
These are just a few examples of the many useful Linux commands available for scripting. By combining these commands with other Bash features like loops and conditionals, you can create useful scripts to automate various tasks.
Executing a Bash Script in Linux
To execute a Bash script in Linux, you need to ensure that the script has execute permissions. This can be done using the 'chmod' command.
Here's an example of how to give execute permissions to a script called 'script.sh':
chmod +x script.sh
Once the script has execute permissions, you can run it by specifying the script's name or path:
./script.sh
In this example, the './' prefix is used to indicate that the script is located in the current directory. If the script is located in a different directory, you can specify the full path to the script.
Understanding File Permissions in Linux
In Linux, file permissions determine who can read, write, and execute a file. Understanding file permissions is essential when working with Bash scripts, as you may need to modify permissions to execute or edit scripts.
File permissions are represented by three sets of permissions: user, group, and others. Each set consists of three characters: read (r), write (w), and execute (x).
To view the permissions of a file, you can use the 'ls' command with the '-l' option:
ls -l file_name
The output will display the permissions for the file in the following format:
-rwxr-xr-x 1 user group 1024 Jan 1 00:00 file_name
The first character represents the file type ('-' for a regular file). The next three characters represent the user permissions, followed by the group permissions, and finally, the permissions for others.
To modify file permissions, you can use the 'chmod' command. Here are some examples:
chmod +x script.sh # Add execute permission to the script chmod -w file.txt # Remove write permission from the file chmod 644 file.txt # Set read and write permissions for the user, and read permissions for others
Related Article: How to Filter Strings in Bash Scripts
Utilizing Variables in Bash Scripts
Variables in Bash scripts are used to store and manipulate data. They can be assigned values and accessed throughout the script.
To assign a value to a variable, use the following syntax:
variable_name=value
Here's an example:
name="John Doe"
To access the value of a variable, prefix the variable name with a '$' sign:
echo $name
In this example, the value of the 'name' variable is printed using the 'echo' command.
Variables can also be used in command substitution, where the output of a command is assigned to a variable:
current_date=$(date +%Y-%m-%d) echo "Today's date is $current_date"
In this example, the 'date' command is executed and its output is assigned to the 'current_date' variable. The variable is then used in the 'echo' command to display the current date.
Differences Between a Terminal and Command Prompt
A terminal and a command prompt are both interfaces used to interact with a computer's operating system. While they serve similar purposes, there are some key differences between the two.
A terminal is a program that provides a text-based interface to execute commands. It is commonly used in Unix-based systems like Linux and macOS. The terminal allows users to run various commands and access the full range of system functionality.
On the other hand, a command prompt is a similar interface, but it is specific to the Windows operating system. It provides a command-line interface (CLI) to execute commands and perform system operations.
One major difference between a terminal and a command prompt is the underlying operating system. A terminal is typically used in Unix-based systems, while a command prompt is used in Windows. This means that the commands and syntax used in each interface may differ.
Another difference is the shell or command interpreter used. In a terminal, the default shell is often Bash, which is a popular shell in Unix-based systems. In a command prompt, the default shell is usually CMD or PowerShell.
Calling a Bash Script from Another Bash Script
In Bash scripting, you can call one Bash script from another Bash script to modularize your code and reuse common functionality. This can be done using the following syntax:
./script2.sh
In this example, the script called 'script2.sh' is executed by specifying its name and using the './' prefix to indicate that it is located in the current directory. If the script is located in a different directory, you can specify the full path to the script.
When calling a Bash script from another script, you can also pass arguments to the called script. These arguments can be accessed within the script using special variables:
./script2.sh arg1 arg2
In the called script, you can access the arguments using the '$1', '$2', etc., syntax:
#!/bin/bash echo "Argument 1: $1" echo "Argument 2: $2"
In this example, the arguments 'arg1' and 'arg2' are passed to 'script2.sh'. The script then prints the values of the arguments using the 'echo' command.
Calling Bash scripts from other Bash scripts is useful for creating modular and reusable code, allowing you to organize and manage your scripts more efficiently.
Additional Resources
- Using Variables in Bash Script