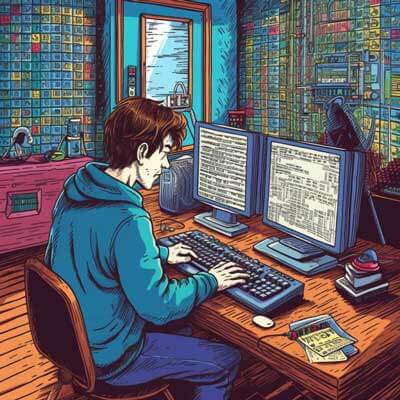
In Bash, there are several ways to echo a newline character. The newline character is represented by the escape sequence “\n”. This character is used to start a new line when displaying output in the terminal or in a file. In this guide, we will explore different methods to echo a newline in Bash.
Method 1: Using the echo command
The simplest method to echo a newline in Bash is by using the echo command with the -e option. The -e option enables the interpretation of escape sequences, including the newline character (\n). Here is an example:
echo -e "This is the first line.\nThis is the second line."
Output:
This is the first line. This is the second line.
In the above example, the \n escape sequence is used to insert a newline character between the two lines.
Related Article: How to Use If-Else Statements in Shell Scripts
Method 2: Using the printf command
Another way to echo a newline in Bash is by using the printf command. The printf command allows for more precise control over the output format compared to the echo command. To insert a newline character, you can use the \n escape sequence within the format string. Here is an example:
printf "This is the first line.\nThis is the second line.\n"
Output:
This is the first line. This is the second line.
In the above example, the \n escape sequence is used within the format string to insert a newline character at the end of each line.
Method 3: Using the $’\n’ syntax
An alternative method to echo a newline in Bash is by using the $’\n’ syntax. This syntax allows you to directly insert a newline character into a string without the need for escape sequences. Here is an example:
echo "This is the first line."$'\n'"This is the second line."
Output:
This is the first line. This is the second line.
In the above example, the $’\n’ syntax is used to insert a newline character between the two lines.
Method 4: Using a heredoc
A heredoc is a convenient way to specify multi-line strings in Bash. By using a heredoc, you can easily include newline characters in your output without the need for escape sequences. Here is an example:
cat << EOF This is the first line. This is the second line. EOF
Output:
This is the first line. This is the second line.
In the above example, the heredoc is delimited by the "EOF" marker. The newline characters are included as part of the heredoc content.
Related Article: Executing a Bash Script with Multivariables in Linux
Method 5: Using the echo command with the -n option
By default, the echo command appends a newline character to the end of the output. However, if you want to suppress the newline character, you can use the -n option. Here is an example:
echo -n "This is the first line." echo "This is the second line."
Output:
This is the first line.This is the second line.
In the above example, the -n option is used with the first echo command to suppress the newline character. The second echo command appends a newline character as usual.
Why is this question asked?
The question “How to echo a newline in Bash?” is commonly asked by Linux users and Bash script developers. Understanding how to insert newline characters is essential for formatting output in scripts, generating structured text files, or processing data. By knowing different methods to echo a newline in Bash, users can tailor their output to meet specific requirements.
Potential reasons for asking the question:
1. Formatting output: Users may have a need to format their output by inserting newline characters at specific positions. This could be to improve readability or to adhere to a certain file format.
2. Generating structured text files: Users may need to generate text files with a specific structure that requires newline characters at specific locations. This could include configuration files, log files, or data files.
3. Processing data: Users may be working with data that contains newline characters and need to manipulate or process it in a particular way. Understanding how to handle newline characters in Bash is crucial for such tasks.
Related Article: How to Manipulate Quotes & Strings in Bash Scripts
Suggestions and alternative ideas:
1. Consider using a text editor: If you need to generate a large amount of text with complex formatting, it may be more convenient to use a text editor instead of relying solely on Bash commands. Text editors provide advanced features for formatting and manipulating text.
2. Use variables for complex output: If you have a complex output with multiple lines and variables, consider using variables to store the individual lines and then concatenate them as needed. This can make the code more readable and easier to maintain.
Best practices:
1. Use the method that suits your specific requirements: Choose the method that best fits your use case. If you require precise control over the output format, consider using the printf command. If simplicity is more important, the echo command with the -e option can be sufficient.
2. Make your code readable: When using escape sequences or special characters, it is important to make your code readable. Consider adding comments or using variables to explain the purpose of the newline characters and improve code maintainability.
3. Test your output: Before using any method to echo a newline in Bash, it is recommended to test the output to ensure it meets your expectations. This can help avoid unexpected behavior or formatting issues in your scripts or files.