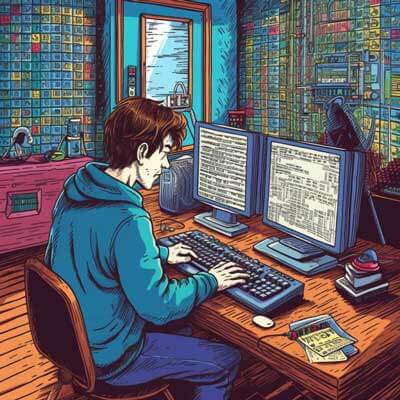
- Basic Date Formatting Options in Bash
- Advanced Date Formatting Options in Bash
- Manipulating Dates in Bash Scripts
- Displaying the Current Date and Time in a Bash Script
- Extracting Specific Components from a Date in Bash
- Converting Date Formats in Bash
- Automatically Updating the Date in a Bash Script
- Understanding the Date Command in Linux
- Using the Date Command in Bash Scripts
- Additional Resources
Basic Date Formatting Options in Bash
When working with dates in Bash scripts, it’s important to be able to format them in a way that is easily readable and understandable. Bash provides various options for basic date formatting. Here are a few examples:
1. Displaying the current date:
#!/bin/bash current_date=$(date +"%Y-%m-%d") echo "Current date: $current_date"
In this example, the date
command is used with the +%Y-%m-%d
format option to display the current date in the format “YYYY-MM-DD”.
2. Displaying the current time:
#!/bin/bash current_time=$(date +"%H:%M:%S") echo "Current time: $current_time"
In this example, the date
command is used with the +%H:%M:%S
format option to display the current time in the format “HH:MM:SS”.
Related Article: How To Echo a Newline In Bash
Advanced Date Formatting Options in Bash
In addition to the basic formatting options, Bash also provides advanced options for formatting dates. Here are a few examples:
1. Displaying the current date and time with milliseconds:
#!/bin/bash current_datetime=$(date +"%Y-%m-%d %H:%M:%S.%3N") echo "Current date and time: $current_datetime"
In this example, the date
command is used with the +%Y-%m-%d %H:%M:%S.%3N
format option to display the current date and time with milliseconds.
2. Displaying the current date in a custom format:
#!/bin/bash current_date=$(date +"Today is %A, %B %d, %Y") echo "$current_date"
In this example, the date
command is used with a custom format option to display the current date in a more human-readable format, including the day of the week, month, and year.
Manipulating Dates in Bash Scripts
Bash provides various tools and functions for manipulating dates in scripts. Here are a few examples:
1. Adding or subtracting days from a given date:
#!/bin/bash given_date="2022-01-01" days_to_add=7 new_date=$(date -d "$given_date + $days_to_add days" +"%Y-%m-%d") echo "New date: $new_date"
In this example, the date
command is used with the -d
option to add 7 days to a given date and display the new date.
2. Calculating the difference between two dates:
#!/bin/bash start_date="2022-01-01" end_date="2022-01-07" date_diff=$(($(date -d "$end_date" +%s) - $(date -d "$start_date" +%s))) days_diff=$((date_diff / 86400)) echo "Number of days between $start_date and $end_date: $days_diff"
In this example, the date
command is used to calculate the difference in seconds between two dates, and then the result is converted to days.
Displaying the Current Date and Time in a Bash Script
To display the current date and time in a Bash script, you can use the date
command with the appropriate format options. Here’s an example:
#!/bin/bash current_datetime=$(date +"%Y-%m-%d %H:%M:%S") echo "Current date and time: $current_datetime"
In this example, the date
command is used with the +%Y-%m-%d %H:%M:%S
format option to display the current date and time in the format “YYYY-MM-DD HH:MM:SS”.
Related Article: How to Use If-Else Statements in Shell Scripts
Extracting Specific Components from a Date in Bash
If you need to extract specific components from a date in Bash, you can use the date
command with the appropriate format options and command substitution. Here are a few examples:
1. Extracting the year from a date:
#!/bin/bash given_date="2022-01-01" year=$(date -d "$given_date" +"%Y") echo "Year: $year"
In this example, the date
command is used with the +%Y
format option to extract the year from a given date.
2. Extracting the month from a date:
#!/bin/bash given_date="2022-01-01" month=$(date -d "$given_date" +"%m") echo "Month: $month"
In this example, the date
command is used with the +%m
format option to extract the month from a given date.
Converting Date Formats in Bash
To convert date formats in Bash, you can use the date
command with the appropriate format options. Here are a few examples:
1. Converting a date from one format to another:
#!/bin/bash given_date="2022-01-01" converted_date=$(date -d "$given_date" +"%d/%m/%Y") echo "Converted date: $converted_date"
In this example, the date
command is used with the +%d/%m/%Y
format option to convert a date from the format “YYYY-MM-DD” to the format “DD/MM/YYYY”.
2. Converting a date to a different timezone:
#!/bin/bash given_date="2022-01-01" converted_date=$(TZ="America/New_York" date -d "$given_date" +"%Y-%m-%d %H:%M:%S %Z") echo "Converted date: $converted_date"
In this example, the date
command is used with the -d
option to convert a date to a different timezone (in this case, America/New_York).
Automatically Updating the Date in a Bash Script
To automatically update the date in a Bash script, you can use the date
command with command substitution. Here’s an example:
#!/bin/bash current_date=$(date +"%Y-%m-%d") sed -i "s/DATE_TO_BE_UPDATED/$current_date/g" script.sh
In this example, the date
command is used to get the current date and store it in the current_date
variable. Then, the sed
command is used to replace the placeholder “DATE_TO_BE_UPDATED” in the script.sh file with the current date.
Related Article: Executing a Bash Script with Multivariables in Linux
Understanding the Date Command in Linux
The date
command in Linux is used to display or set the system date and time. It provides various options for formatting and manipulating dates. Here are a few important options:
-d, –date=STRING: Display the date and time specified by STRING.
+%FORMAT: Display the date and time in the specified format.
%Y: Year (e.g., 2022)
%m: Month (e.g., 01)
%d: Day of the month (e.g., 01)
%H: Hour (e.g., 12)
%M: Minute (e.g., 30)
%S: Second (e.g., 45)
Using the Date Command in Bash Scripts
The date
command can be used in Bash scripts to display or manipulate dates. Here are a few examples:
1. Displaying the current date:
#!/bin/bash current_date=$(date +"%Y-%m-%d") echo "Current date: $current_date"
In this example, the date
command is used with the +%Y-%m-%d
format option to display the current date.
2. Adding or subtracting days from a given date:
#!/bin/bash given_date="2022-01-01" days_to_add=7 new_date=$(date -d "$given_date + $days_to_add days" +"%Y-%m-%d") echo "New date: $new_date"
In this example, the date
command is used with the -d
option to add 7 days to a given date and display the new date.