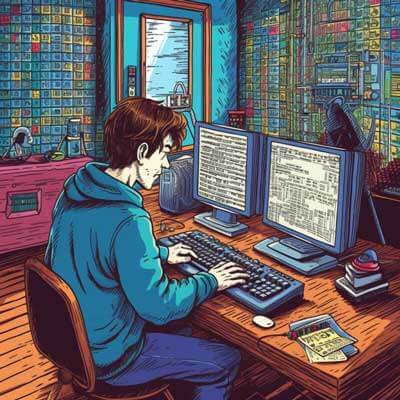
Table of Contents
What are bash scripts?
Bash scripts, also known as shell scripts, are a collection of commands that are executed in a sequence. These scripts are written in the Bash (Bourne Again SHell) language and are primarily used in Linux and Unix operating systems. Bash scripts allow users to automate repetitive tasks, execute commands with specific parameters, and perform various system operations.
Here is an example of a simple bash script that prints "Hello, World!":
#!/bin/bash echo "Hello, World!"
In this script, the first line specifies the interpreter to be used, which is bash in this case. The echo
command is then used to print the message "Hello, World!" to the console.
Related Article: How to Use Multiple If Statements in Bash Scripts
What is zsh?
Zsh, short for Z Shell, is a useful command-line shell that is designed for interactive use. It is an extended version of the Bourne shell (sh) with additional features and improvements. Zsh provides advanced tab completion, better history management, and a more customizable user interface compared to bash.
Zsh is highly extensible and offers a wide range of plugins and themes that enhance the user experience. It supports scripting and can execute shell scripts written in bash, making it a popular choice among Linux users.
How does zsh differ from bash?
Zsh and bash are both Unix shell languages, but they have some differences in terms of features and syntax. Here are a few key differences between zsh and bash:
1. Tab Completion: Zsh provides more advanced tab completion capabilities compared to bash. It can automatically complete command names, file paths, and even arguments based on the context.
2. History Management: Zsh has better history management features, allowing users to search and navigate through their command history more efficiently. It also supports shared command history among multiple shell sessions.
3. Spelling Correction: Zsh has a built-in spelling correction feature that can automatically correct mistyped commands or file names.
4. Customization: Zsh offers more customization options, allowing users to configure their shell environment to their liking. It supports themes, plugins, and various settings that can be adjusted to improve productivity.
Can bash scripts run on zsh?
Yes, zsh is compatible with bash scripts and can execute them without any modifications. This means that if you have existing bash scripts, you can run them using zsh without any issues.
To run a bash script with zsh, you can simply invoke the zsh interpreter and pass the bash script as an argument. Here's an example:
zsh myscript.sh
In this example, the bash script "myscript.sh" is executed using the zsh interpreter.
Related Article: Integrating a Bash Script into a Makefile in Linux
Are bash and zsh compatible?
Yes, bash and zsh are compatible with each other. Zsh is designed to be a superset of the Bourne shell (sh), which means that it supports all the features and syntax of the Bourne shell, including bash.
Bash scripts can be executed using the zsh interpreter without any modifications. This compatibility allows users to switch between bash and zsh without worrying about script compatibility.
Can I use zsh as my default shell in Linux?
Yes, you can use zsh as your default shell in Linux. Here's how you can set zsh as your default shell:
1. Install zsh: If zsh is not already installed on your system, you can install it using the package manager for your Linux distribution. For example, on Ubuntu, you can use the following command:
sudo apt-get install zsh
2. Change the default shell: Once zsh is installed, you can change your default shell by running the following command:
chsh -s $(which zsh)
This command will update the default shell for your user account to zsh.
3. Log out and log in again: After changing the default shell, you need to log out and log in again for the changes to take effect. Once you log back in, zsh will be your default shell.
How do I write a bash script?
To write a bash script, you can use any text editor to create a plain text file with a .sh
extension. Here's a basic example of a bash script that prints "Hello, World!":
#!/bin/bash echo "Hello, World!"
In this script, the first line specifies the interpreter to be used, which is bash in this case. The echo
command is then used to print the message "Hello, World!" to the console.
You can save this script to a file, such as hello.sh
, and make it executable using the following command:
chmod +x hello.sh
After making the script executable, you can run it by executing the following command:
./hello.sh
This will execute the script and display the output "Hello, World!".
What are the advantages of using zsh over bash?
Zsh offers several advantages over bash, making it a popular choice among Linux users. Here are some advantages of using zsh:
1. Advanced Tab Completion: Zsh provides more advanced tab completion capabilities compared to bash. It can autocomplete command names, file paths, and even arguments based on the context. This can significantly speed up command-line navigation and reduce typing.
2. Better History Management: Zsh has better history management features, allowing users to search and navigate through their command history more efficiently. It supports searching, filtering, and sharing command history among multiple shell sessions.
3. Spelling Correction: Zsh has a built-in spelling correction feature that can automatically correct mistyped commands or file names. This can help prevent errors caused by typos and save time spent on debugging.
4. Customization Options: Zsh offers more customization options, allowing users to configure their shell environment to their liking. It supports themes, plugins, and various settings that can be adjusted to improve productivity. This level of customization allows users to tailor their shell experience to their specific needs.
5. Community Support: Zsh has a vibrant community and a large number of plugins and themes available. This means that users can easily enhance their shell experience by installing community-contributed extensions. The community also provides active support and regular updates to ensure a stable and feature-rich shell environment.
Related Article: How to Check If a File Does Not Exist in Bash
Can zsh execute bash commands?
Yes, zsh can execute bash commands without any issues. Zsh is designed to be a superset of the Bourne shell (sh), which includes bash. This means that zsh supports all the features and syntax of bash, making it compatible with bash commands.
For example, if you have a bash command that you want to execute using zsh, you can simply run the command in a zsh shell. Zsh will interpret and execute the command just like bash would.
Here's an example of executing a bash command in zsh:
zsh -c "echo Hello, World!"
In this example, the -c
option is used to specify the bash command to be executed, which is echo Hello, World!
. Zsh will execute the command and display the output "Hello, World!".