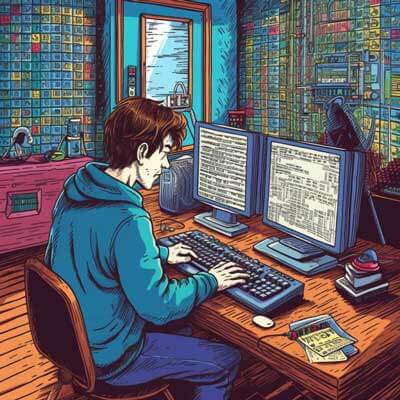
- Command Line Arguments in Bash
- Passing Arguments to a Bash Script
- Bash Script Getopt
- Handling Optional Command Line Arguments in Bash
- Bash Script Input Parameters
- Bash Script Arguments
- Bash Script Variable Assignment
- Bash Script Parameter Expansion
- Bash Script Positional Parameters
- Using Flags or Options to Pass Arguments to a Bash Script
- Limitations on the Number of Parameters in a Bash Script
- Passing Arguments to a Bash Script from a File
Command Line Arguments in Bash
Command line arguments are values or options that are passed to a command or script when it is executed. In Bash, command line arguments can be accessed inside a script using special variables. The most commonly used variables for accessing command line arguments are $0
, $1
, $2
, and so on.
– $0
: This variable stores the name of the script itself.
– $1
: This variable stores the first command line argument.
– $2
: This variable stores the second command line argument.
– And so on…
Let’s take a look at an example to understand how command line arguments work in Bash:
#!/bin/bash echo "The name of the script is: $0" echo "The first argument is: $1" echo "The second argument is: $2"
If we save this script as script.sh
and execute it with the command ./script.sh arg1 arg2
, the output will be:
The name of the script is: ./script.sh The first argument is: arg1 The second argument is: arg2
As you can see, the script is able to access the command line arguments using the special variables $0
, $1
, and $2
. This allows us to perform different actions or process different data based on the values provided.
Related Article: How To Echo a Newline In Bash
Passing Arguments to a Bash Script
In addition to accessing command line arguments using special variables, it is also possible to pass arguments to a Bash script directly when executing it. This can be done by specifying the arguments after the script name, separated by spaces.
Let’s modify our previous example to demonstrate how to pass arguments to a Bash script:
#!/bin/bash echo "The name of the script is: $0" echo "The first argument is: $1" echo "The second argument is: $2"
If we execute this script with the command ./script.sh arg1 arg2
, the output will be the same as before:
The name of the script is: ./script.sh The first argument is: arg1 The second argument is: arg2
As you can see, the script is able to access the arguments passed directly when executing it. This provides flexibility and allows for dynamic behavior based on the provided arguments.
Bash Script Getopt
The getopt
command is a useful utility in Bash that allows you to parse command line options and arguments. It provides a flexible and standardized way to handle command line options and arguments in Bash scripts.
The getopt
command uses the POSIX-style option syntax, where options are specified with a single hyphen (-
) and arguments are specified without a hyphen.
To use getopt
in a Bash script, you need to provide a list of options and their corresponding arguments. The getopt
command will then parse the command line arguments and return the options and their arguments in a specified format.
Let’s take a look at an example to understand how getopt
works in Bash:
#!/bin/bash options="f:o:h" long_options="file:,output:,help" # Parse command line options parsed_options=$(getopt -o $options -l $long_options -- "$@") # Check for errors in parsing if [ $? -ne 0 ]; then exit 1 fi # Evaluate the parsed options eval set -- "$parsed_options" # Process the options while true; do case "$1" in -f|--file) file=$2 shift 2 ;; -o|--output) output=$2 shift 2 ;; -h|--help) echo "Usage: script.sh [-f|--file <file>] [-o|--output <output>] [-h|--help]" exit 0 ;; --) shift break ;; *) echo "Invalid option: $1" exit 1 ;; esac done echo "File: $file" echo "Output: $output"
In this example, we define the options and their corresponding arguments using the variables options
and long_options
. We then use the getopt
command to parse the command line arguments and store the parsed options in the variable parsed_options
.
After parsing the options, we evaluate the parsed options using eval set -- "$parsed_options"
. This allows us to process the options using a while
loop and a case
statement.
The case
statement checks the value of $1
(the current option) and performs the corresponding action. The shift
command is used to shift the positional parameters, allowing us to access the next option and its argument.
Finally, we display the values of the options and their arguments using echo
.
This example demonstrates how getopt
can be used to handle command line options and arguments in a Bash script. It provides a standardized and flexible way to process options and allows for a more user-friendly script interface.
Handling Optional Command Line Arguments in Bash
When writing Bash scripts, it’s common to have optional command line arguments that provide additional functionality or configuration options. These optional arguments allow users to customize the behavior of the script without having to modify the script itself.
In Bash, there are several ways to handle optional command line arguments. One approach is to use the getopt
command, as demonstrated in the previous section. Another approach is to use conditional statements and variable assignment to handle the presence or absence of optional arguments.
Let’s take a look at an example to understand how to handle optional command line arguments in Bash:
#!/bin/bash # Default values file="default.txt" output="output.txt" # Process command line arguments while [[ $# -gt 0 ]]; do case "$1" in -f|--file) file=$2 shift 2 ;; -o|--output) output=$2 shift 2 ;; *) echo "Invalid option: $1" exit 1 ;; esac done echo "File: $file" echo "Output: $output"
In this example, we define default values for the optional arguments file
and output
. We then use a while
loop and a case
statement to process the command line arguments.
If the option -f
or --file
is present, we assign the value of the next argument to the variable file
using the shift
command. Similarly, if the option -o
or --output
is present, we assign the value of the next argument to the variable output
. If an invalid option is provided, an error message is displayed and the script exits.
After processing the command line arguments, we display the values of the variables file
and output
using echo
.
This approach allows us to handle optional command line arguments in a flexible and customizable way. Users can specify the optional arguments when executing the script, or the script can fall back to the default values if the arguments are not provided.
Related Article: How to Use If-Else Statements in Shell Scripts
Bash Script Input Parameters
In addition to command line arguments and arguments passed directly when executing a Bash script, it is also possible to read input parameters from the user during script execution. This can be done using the read
command, which reads a line of input from the user and stores it in a variable.
Let’s take a look at an example to understand how to read input parameters from the user in a Bash script:
#!/bin/bash echo "Enter your name:" read name echo "Enter your age:" read age echo "Hello, $name! You are $age years old."
If we execute this script, it will prompt the user to enter their name and age. After the user provides the input, the script will display a personalized message.
Enter your name: John Enter your age: 25 Hello, John! You are 25 years old.
As you can see, the read
command allows us to interact with the user during script execution and capture their input for further processing.
Bash Script Arguments
Passing arguments to a Bash script is a common requirement when writing shell scripts. Arguments can be used to control the behavior of the script, provide input data, or specify options and flags.
There are several ways to pass arguments to a Bash script, including command line arguments, arguments passed directly when executing the script, and reading input parameters from the user during script execution.
Let’s summarize the different methods for passing arguments to a Bash script:
– Command Line Arguments: These are values or options that are passed to a script when it is executed. They can be accessed inside the script using special variables such as $0
, $1
, $2
, and so on.
– Arguments Passed Directly: Arguments can also be passed directly when executing a script by specifying them after the script name, separated by spaces.
– Input Parameters: Input parameters can be read from the user during script execution using the read
command. The user’s input is stored in variables for further processing.
Bash Script Variable Assignment
In Bash scripting, variables are used to store values that can be used later in the script. Variable assignment is the process of assigning a value to a variable.
Variables in Bash are not explicitly typed, meaning you can assign any value to a variable without specifying its type. Bash automatically determines the type based on the value assigned.
To assign a value to a variable in Bash, you can use the following syntax:
variable_name=value
Let’s take a look at an example to understand how variable assignment works in Bash:
#!/bin/bash name="John" age=25 echo "Hello, $name! You are $age years old."
In this example, we assign the value “John” to the variable name
and the value 25 to the variable age
. We then use the variables in an echo
statement to display a personalized message.
Hello, John! You are 25 years old.
As you can see, variable assignment allows us to store and manipulate values in a Bash script, making it easier to work with data and perform operations based on the stored values.
Related Article: How to Manipulate Quotes & Strings in Bash Scripts
Bash Script Parameter Expansion
Parameter expansion is a feature in Bash that allows you to manipulate and expand the values of variables and parameters. It provides a way to transform or substitute variable values based on certain patterns or conditions.
There are several forms of parameter expansion in Bash, including:
– ${parameter}
: This form expands the value of the variable parameter
.
– ${parameter:-default}
: This form expands the value of the variable parameter
, but if the variable is unset or empty, it expands to the default value specified.
– ${parameter:=default}
: This form expands the value of the variable parameter
, but if the variable is unset or empty, it assigns the default value specified to the variable and expands to that value.
– ${parameter:+alternate}
: This form expands to the alternate value if the variable parameter
is set and non-empty.
– ${parameter:?error_message}
: This form displays an error message and exits the script if the variable parameter
is unset or empty.
Let’s take a look at some examples to understand how parameter expansion works in Bash:
#!/bin/bash name="John" age= echo "Name: ${name}" echo "Age: ${age:-Unknown}" echo "Alternate Age: ${age:+25}" echo "Error Age: ${age:?Age is not set.}"
In this example, we have a variable name
with the value “John” and a variable age
that is unset. We use parameter expansion to manipulate and expand the values of these variables.
Name: John Age: Unknown Alternate Age: Error Age: bash: age: Age is not set.
As you can see, parameter expansion allows us to handle unset or empty variables, provide default values, and perform conditional operations based on the values of the variables.
Bash Script Positional Parameters
Positional parameters are special variables in Bash that store the command line arguments passed to a script. They are accessed using the special variables $1
, $2
, $3
, and so on, where $1
represents the first argument, $2
represents the second argument, and so on.
Positional parameters are useful for writing scripts that need to process a variable number of arguments or perform actions based on the specific arguments provided.
Let’s take a look at an example to understand how positional parameters work in Bash:
#!/bin/bash echo "The name of the script is: $0" echo "The first argument is: $1" echo "The second argument is: $2" echo "All arguments are: $@"
If we save this script as script.sh
and execute it with the command ./script.sh arg1 arg2
, the output will be:
The name of the script is: ./script.sh The first argument is: arg1 The second argument is: arg2 All arguments are: arg1 arg2
As you can see, the script is able to access the positional parameters using the special variables $0
, $1
, and $2
. The special variable $@
represents all the positional parameters.
Positional parameters allow us to write flexible scripts that can process a variable number of arguments and perform different actions based on the specific arguments provided.
Using Flags or Options to Pass Arguments to a Bash Script
Flags or options are a common way to pass arguments to a Bash script. Flags are single-letter options preceded by a hyphen (-
), while options are longer, more descriptive options preceded by two hyphens (--
).
In Bash, flags and options can be handled using the getopt
command, as demonstrated earlier. However, if you prefer a more lightweight approach, you can use conditional statements and variable assignment to process flags and options.
Let’s take a look at an example to understand how to use flags and options to pass arguments to a Bash script:
#!/bin/bash # Default values verbose=false output="output.txt" # Process command line arguments while [[ $# -gt 0 ]]; do case "$1" in -v|--verbose) verbose=true shift ;; -o|--output) output=$2 shift 2 ;; *) echo "Invalid option: $1" exit 1 ;; esac done if [ "$verbose" = true ]; then echo "Verbose mode enabled" fi echo "Output: $output"
In this example, we define default values for the flags verbose
and output
. We then use a while
loop and a case
statement to process the command line arguments.
If the flag -v
or --verbose
is present, we set the variable verbose
to true
using the shift
command. If the flag -o
or --output
is present, we assign the value of the next argument to the variable output
using the shift
command.
After processing the command line arguments, we use a conditional statement to check the value of verbose
and display a message if it is true
. We also display the value of the variable output
using echo
.
This approach allows us to use flags and options to pass arguments to a Bash script, providing more control and flexibility to the user. Users can enable or disable certain features or specify custom values for configuration options when executing the script.
Related Article: How to Import JSON from a Bash Script on Linux
Limitations on the Number of Parameters in a Bash Script
In Bash, there is a limitation on the number of parameters that can be passed to a script. This limitation is imposed by the operating system and is typically determined by the maximum size of the command line buffer.
The maximum number of parameters that can be passed to a Bash script varies depending on the operating system and its configuration. On most modern systems, the maximum number of parameters is large enough to accommodate the needs of most scripts.
However, it’s important to keep in mind that passing a large number of parameters to a script can have an impact on the performance and efficiency of the script. Handling a large number of parameters may require additional processing time and memory resources.
If you find yourself needing to pass a large number of parameters to a script, it may be a sign that it’s time to reconsider your approach and consider alternative methods for passing and processing data. For example, you could store the data in a file and read it from the script, or use environment variables to pass the data.
It’s also worth noting that passing a large number of parameters can make the script harder to read and maintain. If possible, try to group related parameters together or use options and flags to provide a more concise and user-friendly interface.
Overall, while there is a limitation on the number of parameters that can be passed to a Bash script, it is rarely a practical constraint for most scripts. It’s important to consider the impact on performance and readability when designing scripts that require a large number of parameters.
Passing Arguments to a Bash Script from a File
In addition to passing arguments to a Bash script through the command line, it is also possible to pass arguments from a file. This can be useful when you have a large number of arguments or when you want to specify complex arguments that are difficult to pass directly on the command line.
To pass arguments from a file to a Bash script, you can use input redirection (<
) to read the file as input to the script. Inside the script, you can read the arguments using the read
command.
Let’s take a look at an example to understand how to pass arguments from a file to a Bash script:
#!/bin/bash # Read arguments from file while IFS= read -r line; do arguments+=("$line") done < arguments.txt # Process the arguments for argument in "${arguments[@]}"; do echo "Argument: $argument" done
In this example, we have a file called arguments.txt
that contains a list of arguments, with each argument on a separate line. We use input redirection (<
) to read the file as input to the script.
Inside the script, we use a while
loop and the read
command to read each line from the file and store it in the array arguments
. The -r
option is used with read
to preserve backslashes in the input.
After reading the arguments, we use a for
loop to iterate over the elements of the arguments
array and display each argument using echo
.
If the arguments.txt
file contains the following lines:
arg1 arg2 arg3
When we execute the script, the output will be:
Argument: arg1 Argument: arg2 Argument: arg3
As you can see, passing arguments from a file to a Bash script provides a flexible and convenient way to handle a large number of arguments or complex arguments that are difficult to pass directly on the command line. It allows for better organization and can simplify the execution of the script.