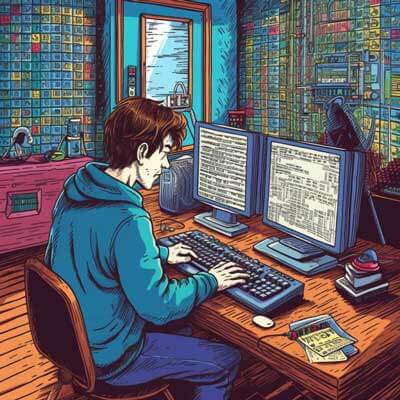
Comparing strings in Bash is a common task when working with Linux shell scripts. In Bash, you can compare strings using various operators and techniques. In this guide, we will explore different methods to compare strings in Bash and provide examples of best practices.
Method 1: Using the Equality Operator
The equality operator (==
) is used to compare two strings for equality in Bash. Here’s an example:
string1="Hello" string2="World" if [ "$string1" == "$string2" ]; then echo "The strings are equal" else echo "The strings are not equal" fi
In the above code snippet, we have two variables string1
and string2
that store the strings “Hello” and “World” respectively. The if
statement checks if string1
is equal to string2
using the equality operator (==
). If the strings are equal, it prints “The strings are equal”; otherwise, it prints “The strings are not equal”.
Related Article: How To Echo a Newline In Bash
Method 2: Using the Inequality Operator
The inequality operator (!=
) is used to check if two strings are not equal in Bash. Here’s an example:
string1="Hello" string2="World" if [ "$string1" != "$string2" ]; then echo "The strings are not equal" else echo "The strings are equal" fi
In the above code snippet, we have two variables string1
and string2
that store the strings “Hello” and “World” respectively. The if
statement checks if string1
is not equal to string2
using the inequality operator (!=
). If the strings are not equal, it prints “The strings are not equal”; otherwise, it prints “The strings are equal”.
Method 3: Using the Greater Than and Less Than Operators
In addition to equality and inequality operators, you can also use the greater than (>
) and less than (<
) operators to compare strings alphabetically in Bash. Here’s an example:
string1="Apple" string2="Banana" if [[ "$string1" > "$string2" ]]; then echo "$string1 is greater than $string2" elif [[ "$string1" < "$string2" ]]; then echo "$string1 is less than $string2" else echo "The strings are equal" fi
In the above code snippet, we have two variables string1
and string2
that store the strings “Apple” and “Banana” respectively. The if
statement compares the strings alphabetically using the greater than (>
) and less than (<
) operators. If string1
is greater than string2
, it prints “$string1 is greater than $string2”. If string1
is less than string2
, it prints “$string1 is less than $string2”. Otherwise, it prints “The strings are equal”.
Method 4: Using the -z and -n Flags
You can use the -z
flag to check if a string is empty and the -n
flag to check if a string is not empty in Bash. Here’s an example:
string="Hello" if [ -z "$string" ]; then echo "The string is empty" elif [ -n "$string" ]; then echo "The string is not empty" fi
In the above code snippet, we have a variable string
that stores the string “Hello”. The if
statement checks if string
is empty using the -z
flag. If the string is empty, it prints “The string is empty”. If the string is not empty, it prints “The string is not empty” using the -n
flag.
Related Article: How to Use If-Else Statements in Shell Scripts
Best Practices
When comparing strings in Bash, it is recommended to:
1. Always quote the strings to handle cases where the strings contain spaces or special characters. For example, use "$string"
instead of $string
.
2. Use double brackets ([[ ]]
) instead of single brackets ([ ]
) for string comparisons. Double brackets provide additional functionality and improved syntax for string comparisons.
3. Be mindful of the case sensitivity when comparing strings. By default, Bash performs case-sensitive comparisons. If you need case-insensitive comparisons, you can use the ==
operator with the nocasematch
shell option enabled.
4. Consider using regular expressions for more complex string comparisons. Bash supports pattern matching using the =~
operator. For example, you can check if a string matches a specific pattern using regular expressions.
5. Use descriptive variable names to improve code readability. Avoid using generic variable names like string1
and string2
. Instead, use meaningful names that indicate the purpose of the string.