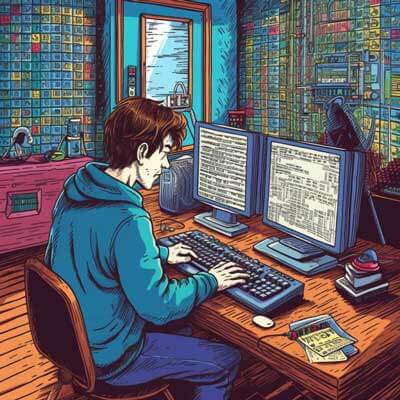
- Using Regular Expressions for Pattern Matching in Bash
- String Manipulation Techniques in Bash
- Filtering Text Using the Grep Command
- Manipulating Strings with the Sed Command
- Advanced String Manipulation with the Awk Command
- Filtering Text in Bash
- Implementing String Substitution in Bash
- Matching Patterns with Wildcards in Bash
- String Manipulation Using Bash Scripts
- Common Techniques for Pattern Matching in Bash Scripts
- Using Regular Expressions to Filter Strings in Bash
- Comparing Grep, Sed, and Awk for Text Filtering in Linux
- Built-in Functions and Commands for String Manipulation in Bash
- Examples of String Substitution in Bash
- Advanced Techniques for Pattern Matching in Bash
Using Regular Expressions for Pattern Matching in Bash
Regular expressions are a useful tool for pattern matching in Bash scripting. They allow you to define complex patterns and search for matches within strings. In Bash, you can use the =~
operator to match a string against a regular expression. Here’s an example:
#!/bin/bash string="Hello, world!" if [[ $string =~ ^Hello ]]; then echo "The string starts with 'Hello'" fi
In this example, we use the =~
operator to match the string against the regular expression ^Hello
, which matches any string that starts with “Hello”. If the match is successful, we print a message indicating that the string starts with “Hello”.
Regular expressions provide a wide range of pattern matching capabilities, such as matching specific characters, ranges of characters, or even complex patterns. They are a useful tool for filtering strings based on specific patterns in Bash scripting.
Related Article: How To Echo a Newline In Bash
String Manipulation Techniques in Bash
Bash provides various string manipulation techniques that allow you to modify and manipulate strings. These techniques include substring extraction, string concatenation, and string length calculation.
Here are a few examples:
#!/bin/bash string="Hello, world!" # Extracting a substring substring=${string:0:5} echo "Substring: $substring" # Output: "Hello" # Concatenating strings greeting="Hi" name="John" message="$greeting, $name!" echo "Message: $message" # Output: "Hi, John!" # Calculating string length length=${#string} echo "Length: $length" # Output: 13
In this example, we extract a substring from the original string using the ${string:start:length}
syntax. We concatenate two strings using the "$string1$string2"
syntax. Finally, we calculate the length of a string using the ${#string}
syntax.
These string manipulation techniques provide flexibility when working with strings in Bash scripting.
Filtering Text Using the Grep Command
The grep
command is a useful tool for filtering text based on patterns. It allows you to search for lines that match a specific pattern and display those lines. Here’s an example:
#!/bin/bash # Filtering lines containing the word "apple" grep "apple" fruits.txt
In this example, we use the grep
command to filter lines in the “fruits.txt” file that contain the word “apple”. The command will display all the lines that match the pattern.
The grep
command provides various options and flags that allow you to customize the search and filtering process. It supports regular expressions and can be used in combination with other commands to perform complex text filtering operations.
Manipulating Strings with the Sed Command
The sed
command is another useful tool for string manipulation in Bash scripting. It allows you to perform various operations on strings, such as substitution, deletion, and insertion. Here’s an example:
#!/bin/bash # Replacing "apple" with "orange" in a string string="I like apple pie." new_string=$(echo $string | sed 's/apple/orange/') echo "New string: $new_string" # Output: "I like orange pie."
In this example, we use the sed
command to replace the word “apple” with “orange” in a string. The s/pattern/replacement/
syntax is used to specify the pattern to match and the replacement string.
The sed
command provides a wide range of options and flags that allow you to customize the string manipulation process. It can be combined with other commands to perform complex string manipulation operations.
Related Article: How to Use If-Else Statements in Shell Scripts
Advanced String Manipulation with the Awk Command
The awk
command is a versatile tool for advanced string manipulation in Bash scripting. It allows you to process text files line by line and perform various operations on each line. Here’s an example:
#!/bin/bash # Extracting the second field from a CSV file awk -F',' '{print $2}' data.csv
In this example, we use the awk
command to extract the second field from a CSV file. The -F','
option is used to specify the field separator as a comma. The {print $2}
expression is used to print the second field of each line.
The awk
command provides a useful scripting language that allows you to perform complex string manipulation operations. It supports variables, conditionals, loops, and other programming constructs, making it a versatile tool for advanced text processing.
Filtering Text in Bash
In addition to external commands like grep
, sed
, and awk
, Bash itself provides built-in mechanisms for filtering text based on patterns. These mechanisms include pattern matching operators and string manipulation functions.
Here are a few examples:
#!/bin/bash string="Hello, world!" # Pattern matching with wildcard characters if [[ $string == *world* ]]; then echo "The string contains 'world'" fi # Pattern matching with regular expressions if [[ $string =~ ^Hello ]]; then echo "The string starts with 'Hello'" fi # String manipulation with parameter expansion substring=${string:0:5} echo "Substring: $substring" # Output: "Hello"
In this example, we use the ==
operator with wildcard characters to match a pattern in the string. We also use the =~
operator with regular expressions to match a pattern. Finally, we use parameter expansion to extract a substring from the string.
These built-in mechanisms in Bash provide convenient ways to filter text based on patterns without relying on external commands.
Implementing String Substitution in Bash
String substitution is a common operation in Bash scripting that allows you to replace occurrences of a pattern with a replacement string. Bash provides various mechanisms for implementing string substitution, including parameter expansion and the sed
command.
Here are a few examples:
#!/bin/bash string="Hello, world!" # Using parameter expansion for substitution new_string=${string/world/John} echo "New string: $new_string" # Output: "Hello, John!" # Using the sed command for substitution new_string=$(echo $string | sed 's/world/John/') echo "New string: $new_string" # Output: "Hello, John!"
In this example, we use parameter expansion to substitute the word “world” with “John” in the original string. We also use the sed
command to perform the same substitution.
These mechanisms provide flexibility when implementing string substitution in Bash scripting, allowing you to customize the replacement process based on your specific requirements.
Related Article: Executing a Bash Script with Multivariables in Linux
Matching Patterns with Wildcards in Bash
Wildcards are special characters that allow you to match patterns in Bash scripting. They are commonly used in file and string matching operations. Bash provides several wildcards, including the asterisk (*
) and question mark (?
).
Here are a few examples:
#!/bin/bash # Matching files with an extension for file in *.txt; do echo "Processing file: $file" done # Matching strings with a pattern string="Hello, world!" if [[ $string == *world* ]]; then echo "The string contains 'world'" fi
In this example, we use the asterisk wildcard to match files with a specific extension in a directory. We also use the asterisk wildcard to match a pattern in a string.
Wildcards provide a convenient way to match patterns in Bash scripting, allowing you to perform file and string matching operations efficiently.
String Manipulation Using Bash Scripts
Bash scripts provide a useful and flexible environment for performing string manipulation operations. With the various string manipulation techniques and commands available in Bash, you can easily develop scripts to process and manipulate strings.
Here’s an example of a Bash script that performs string manipulation operations:
#!/bin/bash # Extracting a substring from a string substring() { local string=$1 local start=$2 local length=$3 echo "${string:start:length}" } # Concatenating two strings concatenate() { local string1=$1 local string2=$2 echo "$string1$string2" } # Calculating the length of a string length() { local string=$1 echo "${#string}" } # Main script string="Hello, world!" substring=$(substring "$string" 0 5) echo "Substring: $substring" # Output: "Hello" new_string=$(concatenate "Hi" "John") echo "New string: $new_string" # Output: "HiJohn" length=$(length "$string") echo "Length: $length" # Output: 13
In this script, we define functions for extracting a substring, concatenating strings, and calculating the length of a string. We then use these functions to perform string manipulation operations on the original string.
Bash scripts provide a convenient way to automate string manipulation tasks, allowing you to process and manipulate strings efficiently.
Common Techniques for Pattern Matching in Bash Scripts
Pattern matching is a common task in Bash scripting, and there are several techniques you can use to match patterns in your scripts. These techniques include wildcard characters, regular expressions, and string manipulation functions.
Here’s a summary of the common techniques for pattern matching in Bash scripts:
– Wildcard characters: Use the asterisk (*
) and question mark (?
) wildcards to match patterns in file and string matching operations.
– Regular expressions: Use the =~
operator with regular expressions to match patterns in strings. Regular expressions provide a useful and flexible way to define complex patterns.
– String manipulation functions: Use string manipulation functions like substring extraction, string concatenation, and length calculation to match patterns within strings.
Related Article: How to Manipulate Quotes & Strings in Bash Scripts
Using Regular Expressions to Filter Strings in Bash
Regular expressions are a useful tool for filtering strings based on specific patterns in Bash scripting. They allow you to define complex patterns and search for matches within strings. By using regular expressions, you can easily filter and extract strings that match specific patterns in your scripts.
Here’s an example that uses regular expressions to filter strings:
#!/bin/bash # Filtering strings that contain a digit strings=("Hello, world!" "Hi, there!" "12345") for string in "${strings[@]}"; do if [[ $string =~ [0-9] ]]; then echo "Matched string: $string" fi done
In this example, we define an array of strings and use a loop to iterate over each string. We then use the =~
operator with the regular expression [0-9]
to match strings that contain a digit. If a match is found, we print the matched string.
Regular expressions provide a flexible and useful way to filter strings based on specific patterns in Bash scripts, allowing you to perform complex filtering operations efficiently.
Comparing Grep, Sed, and Awk for Text Filtering in Linux
When it comes to text filtering in Linux, several tools are commonly used, including grep
, sed
, and awk
. While all three tools are useful and provide text filtering capabilities, they have different strengths and use cases.
– grep
: grep
is a command-line tool that is primarily used for searching and filtering text based on patterns. It supports regular expressions and provides a wide range of options and flags for customizing the search. grep
is a versatile tool for basic text filtering operations.
– sed
: sed
is a stream editor that is commonly used for text manipulation and transformation. It allows you to perform various operations on text files, such as substitution, deletion, and insertion. sed
supports regular expressions and provides a scripting language that allows you to perform complex text processing operations. sed
is a useful tool for advanced text filtering and manipulation.
– awk
: awk
is a versatile tool for text processing and analysis. It allows you to process text files line by line and perform various operations on each line. awk
provides a useful scripting language that supports variables, conditionals, loops, and other programming constructs. It is commonly used for advanced text filtering, data extraction, and report generation.
Built-in Functions and Commands for String Manipulation in Bash
Bash provides several built-in functions and commands that allow you to manipulate strings efficiently. These functions and commands include parameter expansion, string slicing, and string length calculation.
Here are a few examples:
– Parameter expansion: Bash provides various parameter expansion techniques, such as ${parameter}
, ${parameter-default}
, and ${parameter//pattern/replacement}
, that allow you to manipulate strings in different ways.
– String slicing: Bash allows you to extract substrings from strings using the ${string:start:length}
syntax. This allows you to extract a portion of a string based on the starting position and length.
– String length calculation: Bash provides the ${#string}
syntax to calculate the length of a string. This allows you to determine the number of characters in a string.
These built-in functions and commands provide convenient and efficient ways to manipulate strings in Bash scripting. By leveraging these features, you can easily develop scripts that perform complex string manipulation operations.
Related Article: How to Import JSON from a Bash Script on Linux
Examples of String Substitution in Bash
String substitution is a common operation in Bash scripting that allows you to replace occurrences of a pattern with a replacement string. Bash provides various mechanisms for implementing string substitution, including parameter expansion and the sed
command.
Here are a few examples:
#!/bin/bash string="Hello, world!" # Using parameter expansion for substitution new_string=${string/world/John} echo "New string: $new_string" # Output: "Hello, John!" # Using the sed command for substitution new_string=$(echo $string | sed 's/world/John/') echo "New string: $new_string" # Output: "Hello, John!"
In this example, we use parameter expansion to substitute the word “world” with “John” in the original string. We also use the sed
command to perform the same substitution.
These mechanisms provide flexibility when implementing string substitution in Bash scripting, allowing you to customize the replacement process based on your specific requirements.
Advanced Techniques for Pattern Matching in Bash
Pattern matching is a fundamental aspect of Bash scripting, and there are advanced techniques you can use to perform complex pattern matching operations. These techniques include regular expressions, nested conditionals, and advanced string manipulation functions.
Here’s an example that demonstrates advanced pattern matching in Bash:
#!/bin/bash # Matching strings with complex patterns strings=("Hello, world!" "Hi, there!" "Hello, everyone!") for string in "${strings[@]}"; do if [[ $string =~ ^(Hello|Hi),.* ]]; then echo "Matched string: $string" fi done
In this example, we define an array of strings and use a loop to iterate over each string. We then use the =~
operator with a regular expression to match strings that start with “Hello” or “Hi” followed by any characters. If a match is found, we print the matched string.
These advanced techniques provide useful capabilities for performing complex pattern matching in Bash scripts, allowing you to filter and process strings based on intricate patterns.