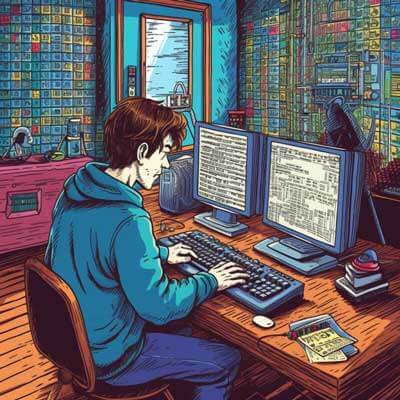
Table of Contents
When incorporating the md5sum command in bash scripts, it is important to properly quote the file paths to ensure that spaces and special characters are handled correctly. Failure to do so can lead to unexpected behavior and errors.
Let's consider an example where we want to calculate the MD5 checksum of a file with a space in its name, "my file.txt". If we do not quote the file path correctly, we may encounter issues. For example:
file_path="my file.txt" md5sum $file_path
This will result in an error, as the shell will interpret "my" and "file.txt" as separate arguments to the md5sum command. To fix this, we need to properly quote the file path using double quotes:
file_path="my file.txt" md5sum "$file_path"
Now, the md5sum command will correctly interpret the file path as a single argument, and the MD5 checksum will be calculated without any errors.
It is important to note that in certain cases, such as when using variables or command substitution, backticks or double quotes may be required instead of single quotes. This depends on the specific requirements of your bash script.
Best Practices for Quoting in Bash Scripts with md5sum
To ensure proper quoting in bash scripts that involve the md5sum command, it is recommended to follow these best practices:
1. Always quote file paths: When passing file paths to the md5sum command, make sure to quote them properly to handle spaces and special characters correctly. Double quotes are generally the preferred choice, as they allow for variable expansion and command substitution.
2. Use variable substitution cautiously: When using variables in bash scripts, be mindful of how they are quoted. If you need the variable's value to be expanded, make sure to enclose it within double quotes. However, if you want the variable to be treated as a literal string, use single quotes instead.
3. Be aware of command substitution: When using command substitution in bash scripts, it is generally recommended to use the $(command) syntax instead of backticks. This ensures better readability and avoids potential issues with nested quotes.
Related Article: How to Run Bash Scripts in the Zsh Shell
Ensuring Proper Quoting in Bash Scripts with md5sum
To ensure proper quoting in bash scripts that involve the md5sum command, you can follow these guidelines:
1. Always quote file paths using double quotes: This ensures that file paths with spaces or special characters are handled correctly. For example:
file_path="my file.txt" md5sum "$file_path"
2. Use single quotes for literal strings: If you want to pass a string as a literal value without any variable expansion or command substitution, use single quotes. For example:
echo 'Hello World'
3. Be mindful of variable expansion: When using variables in bash scripts, enclose them within double quotes to ensure their values are expanded. For example:
name="John" echo "Hello $name"
4. Avoid nested quotes: If you need to use quotes within quotes, be cautious to avoid conflicts. For example:
echo "She said, 'Hello World'"
Potential Issues of Not Using Quotes with md5sum in Bash Scripts
Not using quotes properly when incorporating the md5sum command in bash scripts can lead to several potential issues. Some of these issues include:
1. Incorrect file path interpretation: Without proper quoting, file paths with spaces or special characters may be interpreted incorrectly by the md5sum command. This can result in errors or unexpected behavior.
2. Variable expansion issues: If variables are not properly quoted, their values may not be expanded correctly, leading to incorrect arguments being passed to the md5sum command.
3. Command substitution problems: If command substitution is not properly quoted, the output of the command may not be substituted correctly, leading to unexpected results.
4. Security vulnerabilities: Improper quoting of file paths can potentially introduce security vulnerabilities, especially when dealing with user input or untrusted data. It is important to validate and sanitize file paths to prevent command injection attacks.
To avoid these potential issues, it is crucial to always quote file paths, variables, and command substitutions appropriately when working with the md5sum command in bash scripts.
Alternatives to Adding Quotes when Using md5sum in Bash Scripts
While quoting is the recommended approach for handling file paths and other arguments in bash scripts with the md5sum command, there are alternative techniques that can be used in certain scenarios. These alternatives include:
1. Using escape characters: In some cases, where only a single special character needs to be included in a string, escape characters can be used instead of quotes. For example:
file_path=my\ file.txt md5sum $file_path
2. Storing file paths in an array: If you have multiple file paths that need to be processed by the md5sum command, you can store them in an array and iterate over the array elements. This avoids the need for individual quoting of each file path. For example:
file_paths=("file1.txt" "file2.txt" "file3.txt") for file_path in "${file_paths[@]}"; do md5sum "$file_path" done
3. Using a glob pattern: If you have a collection of files that need to be processed by the md5sum command, you can use a glob pattern to match the files instead of quoting each file path individually. For example:
md5sum *.txt
It is important to note that these alternatives may not be suitable in all scenarios and should be used with caution. Quoting is generally the recommended approach for ensuring proper handling of file paths and arguments in bash scripts.
Related Article: How to Fix Openssl Error: Self Signed Certificate in Certificate Chain on Linux
Double Quotes vs. Single Quotes/h3>
Both double quotes and single quotes can be used to incorporate quotes when working with the md5sum command. However, they have different behaviors and should be used based on the specific requirements of your script.
Double quotes allow for variable expansion and command substitution. This means that variables enclosed within double quotes will be evaluated and replaced with their values. For example:
name="John" echo "Hello $name"
This will output: Hello John
Single quotes, on the other hand, treat everything within them as a literal string. This means that variables and command substitutions are not expanded inside single quotes. For example:
name="John" echo 'Hello $name'
This will output: Hello $name
When working with file paths or any other argument that requires variable expansion or command substitution, double quotes should be used. Single quotes are useful when you want to pass a string as a literal value without any expansion.