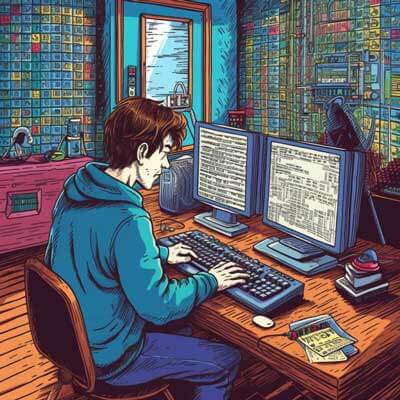
- Detecting File Copy Completion with the cp Command
- Checking if File Copy is Done with rsync
- Determining File Copy Status with the Stat Command
- Comparing Files After a Copy Completion with the cmd Command
- File Transfer Completion Commands
- Checking if File Transfer is Done with FTP
- Determining File Transfer Status with SCP or SFTP
- Comparing Files with Md5sum
- File Synchronization Completion with Rsync or Unison
- Checking if File Synchronization is Complete with rsync
- Determining File Synchronization Status
- Verifying File Synchronization Completion
- Checking for File Copy Completion in a Linux Bash Script
- Determining if a File has Finished Copying in a Linux Bash Script: Lsof command
- Verifying if a File Copy is Done in a Linux Bash Scrip: cmp command
- Detecting if a File Transfer is Complete in a Linux Bash Script: cUrl command
- Recommended Method to Check if a File Transfer is Done in a Linux Bash Script
- Command to Locate a File After The Synchronization Process
- Additional Resources
In a Linux Bash script, it is often necessary to determine if a file copy operation has completed successfully and if the origin and destination file are exactly the same. This is especially important when working with large files or when automating file transfer processes. In this article, we will explore various methods for determining file copy completion in a Linux Bash script and how to verify the status of the file copy.
Detecting File Copy Completion with the cp Command
To detect file copy completion in a Linux Bash script, we can use the cp
command. The cp
command is used to copy files and directories in Linux. By using the cp
command with the -v
(verbose) option, we can get detailed output about the file copy process, including the completion status.
Here’s an example of using the cp
command to copy a file and detect the completion status:
cp -v source_file destination_file
The -v
option will display the progress of the file copy operation, including the number of bytes copied and the percentage of completion. By monitoring the output of the cp
command, we can determine when the file copy is complete.
Related Article: How To Echo a Newline In Bash
Checking if File Copy is Done with rsync
To check if a file copy is done in a Linux Bash script, we can use the rsync
command. The rsync
command is a useful utility for synchronizing files and directories between different locations. By using the rsync
command with the --progress
option, we can get real-time progress information about the file copy operation, including the completion status.
Here’s an example of using the rsync
command to copy a file and check if the copy is done:
rsync --progress source_file destination_file
The --progress
option will display the progress of the file copy operation, including the current file being copied, the number of bytes transferred, and the percentage of completion. By monitoring the output of the rsync
command, we can determine when the file copy is complete.
Determining File Copy Status with the Stat Command
To determine the status of a file copy in a Linux Bash script, we can use the stat
command. The stat
command is used to display file or file system status in Linux. By using the stat
command with the -c
(format) option and the %s
(size) format specifier, we can get the size of the source file and the destination file. By comparing the sizes of the source file and the destination file, we can determine if the file copy is complete.
Here’s an example of using the stat
command to determine the status of a file copy:
source_size=$(stat -c "%s" source_file) destination_size=$(stat -c "%s" destination_file) if [ "$source_size" -eq "$destination_size" ]; then echo "File copy is complete" else echo "File copy is not complete" fi
The stat
command with the -c
option and the %s
format specifier will display the size of the file in bytes. By comparing the sizes of the source file and the destination file using an if statement, we can determine if the file copy is complete.
Comparing Files After a Copy Completion with the cmd Command
To verify the completion of a file copy in a Linux Bash script, we can use the cmp
command. The cmp
command is used to compare two files byte by byte in Linux. By using the cmp
command with the -s
(silent) option, we can compare the source file and the destination file. If the files are identical, the file copy is complete.
Here’s an example of using the cmp
command to verify the completion of a file copy:
cmp -s source_file destination_file if [ $? -eq 0 ]; then echo "File copy is complete" else echo "File copy is not complete" fi
The -s
option will make the cmp
command silent, meaning it will not produce any output. By checking the exit status of the cmp
command using the $?
variable, we can determine if the files are identical and therefore if the file copy is complete.
Related Article: How to Use If-Else Statements in Shell Scripts
File Transfer Completion Commands
In addition to file copy, it is also important to determine the completion of a file transfer in a Linux Bash script. File transfer can be done using various protocols such as FTP, SCP, or SFTP. The method for determining file transfer completion will depend on the specific protocol being used.
Checking if File Transfer is Done with FTP
To check if a file transfer is done in a Linux Bash script using the FTP protocol, we can use the ftp
command. The ftp
command is a standard command-line FTP client in Linux. By using the ftp
command with the -nv
(no verbose) option and the -i
(no interactive) option, we can execute FTP commands non-interactively and suppress verbose output. By monitoring the output of the ftp
command, we can determine when the file transfer is complete.
Here’s an example of using the ftp
command to check if a file transfer is done:
ftp -nv <<EOF open ftp.example.com user username password get source_file destination_file bye EOF
The ftp
command with the -nv
option and the -i
option will execute the FTP commands non-interactively and suppress verbose output. By using a here document (<<EOF
) to provide the FTP commands, we can automate the file transfer. By monitoring the output of the ftp
command, we can determine when the file transfer is complete.
Determining File Transfer Status with SCP or SFTP
To determine the status of a file transfer in a Linux Bash script using the SCP or SFTP protocol, we can use the ssh
command. The ssh
command is a standard command-line SSH client in Linux. By using the ssh
command with the -q
(quiet) option and the -o
(option) option, we can execute SSH commands non-interactively and suppress verbose output. By monitoring the output of the ssh
command, we can determine when the file transfer is complete.
Here’s an example of using the ssh
command to determine the status of a file transfer using the SCP or SFTP protocol:
ssh -q -oBatchMode=yes server "ls -l destination_file" if [ $? -eq 0 ]; then echo "File transfer is complete" else echo "File transfer is not complete" fi
The ssh
command with the -q
option and the -o
option will execute the SSH command non-interactively and suppress verbose output. By executing a remote command using the ssh
command and checking the exit status of the ssh
command using the $?
variable, we can determine if the file transfer is complete.
Related Article: Executing a Bash Script with Multivariables in Linux
Comparing Files with Md5sum
To verify the completion of a file transfer in a Linux Bash script using the SCP or SFTP protocol, we can use the md5sum
command. The md5sum
command is used to calculate and check MD5 checksums in Linux. By using the md5sum
command to calculate the MD5 checksum of the source file and the destination file, we can compare the checksums to verify the completion of the file transfer and thus their integrity. This command will ensure that the file contents are exactly the same.
Here’s an example of using the md5sum
command to verify the completion of a file transfer using the SCP or SFTP protocol:
source_checksum=$(md5sum source_file | awk '{print $1}') destination_checksum=$(md5sum destination_file | awk '{print $1}') if [ "$source_checksum" == "$destination_checksum" ]; then echo "File transfer is complete" else echo "File transfer is not complete" fi
The md5sum
command is used to calculate the MD5 checksum of a file. By using the awk
command to extract the checksum from the output of the md5sum
command and comparing the checksums using an if statement, we can verify the completion of the file transfer.
File Synchronization Completion with Rsync or Unison
In addition to file copy and file transfer, it is also important to determine the completion of file synchronization in a Linux Bash script. File synchronization can be done using various tools such as rsync
or unison
. The method for determining file synchronization completion will depend on the specific tool being used.
Checking if File Synchronization is Complete with rsync
To check if file synchronization is complete in a Linux Bash script using the rsync
command, we can use the rsync
command with the --stats
option. The --stats
option will display statistics about the file synchronization process, including the number of files transferred, the size of the transferred files, and the time taken for the synchronization. By monitoring the output of the rsync
command, we can determine when the file synchronization is complete.
Here’s an example of using the rsync
command to check if file synchronization is complete:
rsync --stats source_directory/ destination_directory/
The rsync
command with the --stats
option will display statistics about the file synchronization process. By monitoring the output of the rsync
command, we can determine when the file synchronization is complete.
Related Article: How to Manipulate Quotes & Strings in Bash Scripts
Determining File Synchronization Status
To determine the status of file synchronization in a Linux Bash script using the unison
command, we can use the unison
command with the -auto
option and the -batch
option. The -auto
option will automatically synchronize files and the -batch
option will execute unison
in batch mode, meaning it will not prompt for any input. By monitoring the exit status of the unison
command, we can determine if the file synchronization is complete.
Here’s an example of using the unison
command to determine the status of file synchronization:
unison -auto -batch source_directory/ destination_directory/ if [ $? -eq 0 ]; then echo "File synchronization is complete" else echo "File synchronization is not complete" fi
The unison
command with the -auto
option and the -batch
option will automatically synchronize files and execute unison
in batch mode. By checking the exit status of the unison
command using the $?
variable, we can determine if the file synchronization is complete.
Verifying File Synchronization Completion
To verify the completion of file synchronization in a Linux Bash script using the rsync
command, we can use the rsync
command with the --dry-run
option. The --dry-run
option will simulate the file synchronization process without actually transferring any files. By monitoring the output of the rsync
command, we can determine if the file synchronization is complete.
Here’s an example of using the rsync
command to verify the completion of file synchronization:
rsync --dry-run source_directory/ destination_directory/ if [ $? -eq 0 ]; then echo "File synchronization is complete" else echo "File synchronization is not complete" fi
The rsync
command with the --dry-run
option will simulate the file synchronization process. By checking the exit status of the rsync
command using the $?
variable, we can determine if the file synchronization is complete.
Checking for File Copy Completion in a Linux Bash Script
To check for file copy completion in a Linux Bash script, we can use the cp
command with the -u
(update) option. The -u
option will only copy the source file to the destination file if the source file is newer than the destination file or if the destination file does not exist. By checking the exit status of the cp
command, we can determine if the file copy is complete.
Here’s an example of checking for file copy completion using the cp
command:
cp -u source_file destination_file if [ $? -eq 0 ]; then echo "File copy is complete" else echo "File copy is not complete" fi
The cp
command with the -u
option will only copy the source file to the destination file if the source file is newer than the destination file or if the destination file does not exist. By checking the exit status of the cp
command using the $?
variable, we can determine if the file copy is complete.
Related Article: How to Import JSON from a Bash Script on Linux
Determining if a File has Finished Copying in a Linux Bash Script: Lsof command
To determine if a file has finished copying in a Linux Bash script, we can use the lsof
command. The lsof
command is used to list open files in Linux. By using the lsof
command with the -r
(repeat) option and the -F
(format) option, we can continuously check if the file is still open by another process. If the file is no longer open, it means that the file has finished copying.
Here’s an example of determining if a file has finished copying using the lsof
command:
while lsof -r 1 -F p source_file; do sleep 1 done echo "File has finished copying"
The lsof
command with the -r
option and the -F
option will continuously check if the file is open by another process. By using a while loop and the sleep
command to pause the script for 1 second between checks, we can determine if the file has finished copying.
Verifying if a File Copy is Done in a Linux Bash Scrip: cmp command
To verify if a file copy is done in a Linux Bash script, we can use the cmp
command to compare the source file and the destination file. If the files are identical, it means that the file copy is done.
Here’s an example of verifying if a file copy is done using the cmp
command:
cmp -s source_file destination_file if [ $? -eq 0 ]; then echo "File copy is done" else echo "File copy is not done" fi
The cmp
command with the -s
option will compare the source file and the destination file. By checking the exit status of the cmp
command using the $?
variable, we can determine if the files are identical and therefore if the file copy is done.
Detecting if a File Transfer is Complete in a Linux Bash Script: cUrl command
To detect if a file transfer is complete in a Linux Bash script, we can use the curl
command. The curl
command is a versatile command-line tool for transferring data with URLs. By using the curl
command with the -O
(remote output) option and the -s
(silent) option, we can download a file from a remote server without displaying any progress information. By checking the exit status of the curl
command, we can determine if the file transfer is complete.
Here’s an example of detecting if a file transfer is complete using the curl
command:
curl -O -s source_url if [ $? -eq 0 ]; then echo "File transfer is complete" else echo "File transfer is not complete" fi
The curl
command with the -O
option and the -s
option will download the file from the specified URL without displaying any progress information. By checking the exit status of the curl
command using the $?
variable, we can determine if the file transfer is complete.
Related Article: Appending Dates to Filenames in Bash Scripts
Recommended Method to Check if a File Transfer is Done in a Linux Bash Script
The recommended method to check if a file transfer is done in a Linux Bash script is to use the wget
command. The wget
command is a useful command-line tool for retrieving files from the web. By using the wget
command with the --spider
option and the -q
(quiet) option, we can check if the file exists on the remote server without actually downloading the file. By checking the exit status of the wget
command, we can determine if the file transfer is done.
Here’s an example of using the wget
command to check if a file transfer is done:
wget --spider -q source_url if [ $? -eq 0 ]; then echo "File transfer is done" else echo "File transfer is not done" fi
The wget
command with the --spider
option and the -q
option will check if the file exists on the remote server without actually downloading the file. By checking the exit status of the wget
command using the $?
variable, we can determine if the file transfer is done.
Command to Locate a File After The Synchronization Process
To verify the completion of a file synchronization in a Linux Bash script, we can use the find
command. The find
command is used to search for files and directories in Linux. By using the find
command with the -type f
(file type) option and the -newer
(newer than) option, we can search for files that are newer than a specified reference file. By checking if there are no files that are newer than the reference file, we can verify the completion of the file synchronization.
Here’s an example of using the find
command to verify the completion of a file synchronization:
find destination_directory/ -type f -newer reference_file | grep -q . if [ $? -eq 1 ]; then echo "File synchronization is complete" else echo "File synchronization is not complete" fi
The find
command with the -type f
option and the -newer
option will search for files that are newer than the reference file. By using the grep
command to check if there are any files found and checking the exit status of the grep
command using the $?
variable, we can verify the completion of the file synchronization.
Additional Resources
– Checking progress of a file copy using the status parameter in rsync