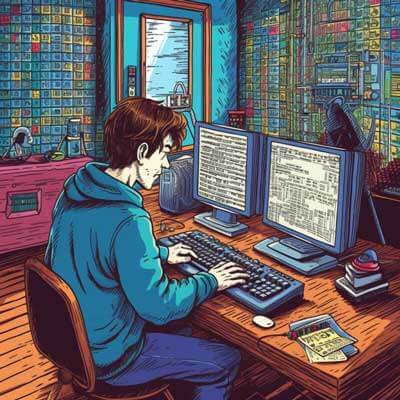
Table of Contents
Avoiding numbers in bash script names
While it is possible to include numbers in bash script names, it is generally recommended to avoid doing so. Including numbers in script names can make them less descriptive and harder to understand. It can also lead to confusion and make it more difficult to maintain and update the scripts. Instead, it is best to use meaningful and descriptive names that accurately reflect the purpose or functionality of the script.
For example, consider the following bash script:
#!/bin/bash # This script calculates the average of a list of numbers numbers=(1 2 3 4 5) sum=0 count=0 for number in "${numbers[@]}"; do sum=$((sum + number)) count=$((count + 1)) done average=$((sum / count)) echo "The average is: $average"
In this example, the script calculates the average of a list of numbers. The script name "calculate_average.sh" clearly indicates its purpose, making it easier for other users to understand and use the script.
Related Article: How to Fix Openssl Error: Self Signed Certificate in Certificate Chain on Linux
How to name scripts with numbers in bash
While it is generally recommended to avoid using numbers in bash script names, there may be cases where you need to include numbers for specific purposes. When naming scripts with numbers in bash, there are a few guidelines to consider. Here is how you can name scripts with numbers in bash:
1. Use descriptive words: Include descriptive words along with the numbers in your script name. This can help make the script name more meaningful and descriptive. For example, instead of "script1.sh", you can use "backup_v1.sh".
2. Separate words with underscores or hyphens: To improve readability, separate words in your script name using underscores or hyphens. For example, instead of "script1.sh", you can use "backup_v1.sh".
3. Avoid leading numbers: Avoid starting your script name with a number. Instead, include the number within the script name to maintain readability. For example, use "backup_v1.sh" instead of "1backup.sh".
4. Be consistent: If you have multiple versions or iterations of a script, consider using a consistent naming pattern. This can make it easier to identify and manage different versions of the script. For example, you can use "backup_v1.sh", "backup_v2.sh", and so on.
Here is an example of a bash script named "backup_v1.sh" that includes a number in the script name:
#!/bin/bash # This script performs version 1 of the backup process source_dir="/path/to/source" destination_dir="/path/to/destination" # Backup files cp -R "$source_dir" "$destination_dir"
In this example, the script name "backup_v1.sh" includes the number "1" along with a descriptive word. This helps differentiate different versions of the script and makes it easier to manage and maintain.
Using alphanumeric characters in bash script names
Bash script names can indeed include alphanumeric characters. Alphanumeric characters refer to the combination of alphabetic letters (uppercase or lowercase) and numbers. This allows for a wide range of possibilities when naming bash scripts.
Using alphanumeric characters in bash script names can make them more descriptive and easier to understand. It also follows the convention of using alphanumeric characters in Linux filenames. This consistency helps differentiate bash scripts from other types of files and improves the overall readability and maintainability of your scripts.
Here is an example of a bash script named "count_files.sh" that uses alphanumeric characters:
#!/bin/bash # This script counts the number of files in a directory directory="/path/to/directory" file_count=$(ls -l "$directory" | grep -c "^-") echo "Number of files in $directory: $file_count"
In this example, the script name "count_files.sh" is descriptive and uses alphanumeric characters. This makes it easier for other users to understand the purpose of the script and improves the overall readability.
Alternative to using numbers in bash script names
If you want to avoid using numbers in bash script names, there are several alternatives you can consider. These alternatives can help make your script names more descriptive and easier to understand. Here are some alternatives to using numbers in bash script names:
1. Use descriptive words: Instead of using numbers, use descriptive words that accurately reflect the purpose or functionality of the script. For example, instead of "script2.sh", you can use "backup_files.sh".
2. Include action or verb: Include an action or verb in your script name to indicate what the script does. This can make the script name more meaningful and descriptive. For example, instead of "2script.sh", you can use "install_package.sh".
3. Use underscores or hyphens: To separate words in your script name, you can use underscores or hyphens. This improves readability and makes the script name more descriptive. For example, instead of "script2.sh", you can use "install-package.sh".
4. Follow naming conventions: Follow the naming conventions and guidelines discussed earlier to ensure consistency and readability in your script names.
Here is an example of a bash script named "backup_files.sh" that uses an alternative to using numbers:
#!/bin/bash # This script backs up files from a source directory to a destination directory source_dir="/path/to/source" destination_dir="/path/to/destination" # Backup files cp -R "$source_dir" "$destination_dir"
In this example, the script name "backup_files.sh" is descriptive and avoids using numbers. This makes it easier for other users to understand and use the script.
Related Article: Secure File Transfer with SFTP: A Linux Tutorial
Bash script naming conventions
When it comes to naming conventions for bash scripts, there are a few guidelines to keep in mind. Bash scripts are commonly used in the Linux environment for automating tasks and running commands. Here are some naming conventions to follow:
1. Use lowercase letters: It is recommended to use lowercase letters for bash script names. This is a widely accepted convention in the Linux community and helps to differentiate bash scripts from other types of files.
2. Use underscores or hyphens: To separate words in a bash script name, you can use either underscores or hyphens. For example, "my_script.sh" or "my-script.sh". This improves readability and makes the script name more descriptive.
3. Avoid special characters: It is best to avoid using special characters such as spaces, symbols, or punctuation marks in bash script names. This can cause issues when running the script or referencing it in other scripts.
4. Be descriptive: Choose a meaningful and descriptive name for your bash script. This helps other users understand the purpose of the script and makes it easier to maintain and update in the future.
Here is an example of a bash script named "hello_world.sh":
#!/bin/bash echo "Hello, World!"
In this example, the script name clearly indicates its purpose, which is to print "Hello, World!" to the console.
Best practices for naming bash scripts
When it comes to naming bash scripts, there are a few best practices to consider. These practices can help improve the readability, maintainability, and compatibility of your scripts. Here are some best practices for naming bash scripts:
1. Be descriptive: Choose a descriptive name that accurately reflects the purpose or functionality of the script. This makes it easier for other users to understand and use your script.
2. Use lowercase letters: Use lowercase letters for script names to maintain consistency with Linux conventions. This helps differentiate bash scripts from other types of files.
3. Separate words with underscores or hyphens: To improve readability, separate words in script names using underscores or hyphens. For example, "backup_files.sh" or "install-package.sh".
4. Avoid special characters: Avoid using special characters, symbols, or spaces in script names. This can cause issues when running the script or referencing it in other scripts.
5. Follow language-specific conventions: Bash scripting has its own conventions and guidelines. Familiarize yourself with these conventions and follow them to ensure compatibility and readability.
6. Use meaningful variable names: In your script, use meaningful variable names to improve readability and maintainability. This helps other users understand the purpose of the script and makes it easier to debug and modify.
Here is an example of a bash script named "backup_files.sh" that follows these best practices:
#!/bin/bash # This script backs up files from a source directory to a destination directory source_dir="/path/to/source" destination_dir="/path/to/destination" # Backup files cp -R "$source_dir" "$destination_dir"
In this example, the script name "backup_files.sh" is descriptive and follows the best practices for naming bash scripts.
Naming conventions for executable scripts
When it comes to naming conventions for executable scripts, there are a few guidelines to follow. These conventions help ensure compatibility and ease of use. Here are some naming conventions for executable scripts:
1. Use lowercase letters: It is recommended to use lowercase letters for executable script names. This is a widely accepted convention in the Linux community and helps differentiate executable scripts from other types of files.
2. Use underscores or hyphens: To separate words in an executable script name, you can use either underscores or hyphens. For example, "my_script.sh" or "my-script.sh". This improves readability and makes the script name more descriptive.
3. Include a file extension: Executable scripts typically have a file extension, such as ".sh" for bash scripts. This helps identify the file type and the script's purpose.
4. Follow platform-specific conventions: Different operating systems may have their own naming conventions for executable scripts. It is important to follow these conventions to ensure compatibility and ease of use.
Here is an example of an executable script named "hello_world.sh" that follows these naming conventions:
#!/bin/bash echo "Hello, World!"
In this example, the script name "hello_world.sh" is lowercase, uses underscores to separate words, and includes the file extension ".sh". This makes the script name more descriptive and follows the naming conventions for executable scripts.
Additional Resources
- Bash Scripting Tutorial