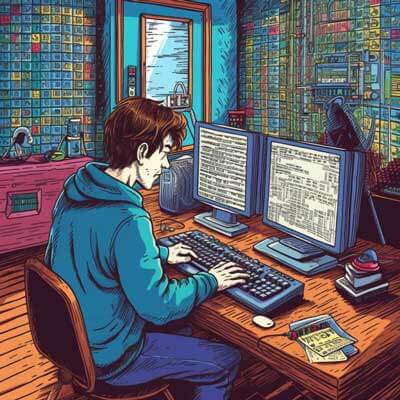
- Passing Multiple Variables to a Bash Script
- Command Line Arguments in Bash Scripts
- Parameter Passing in Bash Scripts
- Functions in Bash Scripts
- Parameter Expansion in Bash Scripts
- Calling a Script with Multiple Arguments
- Handling Parameters in Bash Scripts
- Executing a Script with Multiple Variables from Another Script
- Best Practices for Handling Variables in Bash Scripts
- Proper Variable Substitution in a Bash Script
- Additional Resources
Passing Multiple Variables to a Bash Script
Bash scripts can accept command-line arguments, which allow you to pass data to the script when executing it. These arguments can be accessed within the script using special variables.
The arguments passed to a script are stored in the variables $1
, $2
, $3
, and so on, where $1
represents the first argument, $2
represents the second argument, and so on. The variable $0
contains the name of the script itself.
Here’s an example:
#!/bin/bash echo "The first argument is: $1" echo "The second argument is: $2" echo "The third argument is: $3"
If we execute this script with the command bash script.sh foo bar baz
, the output will be:
The first argument is: foo The second argument is: bar The third argument is: baz
If fewer arguments are passed than the number of variables used, the remaining variables will be empty.
Related Article: How To Echo a Newline In Bash
Command Line Arguments in Bash Scripts
In addition to the positional parameters ($1
, $2
, etc.), Bash provides several special variables that can be used to access command line arguments and other information about the script’s execution.
– $#
: Contains the number of arguments passed to the script.
– $@
: Expands to all the arguments passed to the script as separate words.
– $*
: Expands to all the arguments passed to the script as a single word, separated by spaces.
– $?
: Contains the exit status of the last command.
– $$
: Contains the process ID of the script.
– $!
: Contains the process ID of the last background command.
Here’s an example that demonstrates the usage of these variables:
#!/bin/bash echo "Number of arguments: $#" echo "All arguments: $@" echo "Arguments as a single word: $*" echo "Exit status of the last command: $?" echo "Process ID of the script: $$" echo "Process ID of the last background command: $!"
If we execute this script with the command bash script.sh foo bar baz
, the output will be:
Number of arguments: 3 All arguments: foo bar baz Arguments as a single word: foo bar baz Exit status of the last command: 0 Process ID of the script: 12345 Process ID of the last background command:
Note that the last variable, $!
, is empty because we didn’t execute any background commands.
Parameter Passing in Bash Scripts
In Bash, you can pass parameters to a function or script using two different mechanisms: positional parameters and environment variables.
Positional parameters are variables that are automatically assigned the values of the command-line arguments. They are accessed using the $1
, $2
, etc. syntax, where $1
represents the first argument, $2
represents the second argument, and so on.
Here’s an example that demonstrates the usage of positional parameters:
#!/bin/bash function greet { echo "Hello, $1!" } greet "John"
When executing this script, the output will be:
Hello, John!
In this example, the greet
function takes one argument, which is accessed using $1
within the function.
Environment variables, on the other hand, are variables that are set in the shell environment and can be accessed by any script or program running in that environment. Environment variables are accessed using the $VAR_NAME
syntax, where VAR_NAME
is the name of the variable.
Here’s an example that demonstrates the usage of environment variables:
#!/bin/bash echo "Home directory: $HOME" echo "Current user: $USER"
When executing this script, the output will be:
Home directory: /home/john Current user: john
In this example, the $HOME
and $USER
environment variables are accessed within the script.
Functions in Bash Scripts
Functions in Bash scripts allow you to group a set of commands together and give them a name. This makes your script more modular and easier to read and maintain.
To define a function, you can use the following syntax:
function function_name { # function body }
Here’s an example that defines a function named greet
:
#!/bin/bash function greet { echo "Hello, World!" } greet
When executing this script, the output will be:
Hello, World!
In this example, the greet
function is defined to print the string “Hello, World!”. The function is then called at the end of the script.
You can pass arguments to a function by specifying them within the parentheses after the function name. The arguments can be accessed within the function using the $1
, $2
, etc. syntax.
Here’s an example that demonstrates the usage of function arguments:
#!/bin/bash function greet { echo "Hello, $1!" } greet "John"
When executing this script, the output will be:
Hello, John!
In this example, the greet
function takes one argument, which is accessed using $1
within the function.
Related Article: How to Use If-Else Statements in Shell Scripts
Parameter Expansion in Bash Scripts
Parameter expansion is a useful feature in Bash that allows you to manipulate the values of variables and perform various operations on them.
Here are some commonly used parameter expansion techniques:
– ${variable}
: Retrieves the value of the variable.
– ${variable:-default}
: Uses the value of the variable, or the default value if the variable is unset or empty.
– ${variable:=default}
: Uses the value of the variable, or assigns the default value to the variable if it is unset or empty.
– ${variable:+alternate}
: Uses the alternate value if the variable is set and not empty, otherwise uses an empty string.
– ${variable:?error_message}
: Displays an error message and exits the script if the variable is unset or empty.
– ${variable#pattern}
: Removes the shortest match of pattern
from the beginning of the variable’s value.
– ${variable##pattern}
: Removes the longest match of pattern
from the beginning of the variable’s value.
– ${variable%pattern}
: Removes the shortest match of pattern
from the end of the variable’s value.
– ${variable%%pattern}
: Removes the longest match of pattern
from the end of the variable’s value.
– ${variable/pattern/replacement}
: Replaces the first match of pattern
with replacement
in the variable’s value.
– ${variable//pattern/replacement}
: Replaces all matches of pattern
with replacement
in the variable’s value.
Here’s an example that demonstrates the usage of parameter expansion:
#!/bin/bash name="John" echo "Hello, ${name:-Anonymous}!"
When executing this script, the output will be:
Hello, John!
In this example, we use the ${name:-Anonymous}
parameter expansion to check if the name
variable is unset or empty. If it is, the default value “Anonymous” is used instead.
Calling a Script with Multiple Arguments
To call a script with multiple arguments, you simply separate the arguments with spaces when executing the script.
Suppose we have a Bash script named script.sh
with the following content:
#!/bin/bash echo "The first argument is: $1" echo "The second argument is: $2" echo "The third argument is: $3"
To call this script with multiple arguments, you can run the following command:
$ bash script.sh foo bar baz
The output will be:
The first argument is: foo The second argument is: bar The third argument is: baz
In this example, we pass three arguments (foo
, bar
, baz
) to the script, which are then accessed using $1
, $2
, $3
.
Handling Parameters in Bash Scripts
When working with parameters in Bash scripts, it is important to handle edge cases such as checking for the presence or absence of certain arguments, handling default values, and validating the input.
Here’s an example that demonstrates how to handle parameters in a Bash script:
#!/bin/bash if [[ -z $1 ]]; then echo "No argument provided." exit 1 fi filename="$1.txt" echo "Creating file: $filename" touch "$filename"
In this example, we check if the first argument is empty using the -z
conditional expression. If it is empty, we print an error message and exit the script with a non-zero exit code. Otherwise, we create a new file with the name provided as the first argument.
This is just a basic example, but in real-world scenarios, you may need to handle more complex parameter validations and error handling.
Related Article: How to Manipulate Quotes & Strings in Bash Scripts
Executing a Script with Multiple Variables from Another Script
In Bash, you can execute a script with multiple variables from another script by passing the values to the script as command-line arguments.
Suppose we have a Bash script named script.sh
with the following content:
#!/bin/bash echo "The first variable is: $1" echo "The second variable is: $2" echo "The third variable is: $3"
To execute this script with multiple variables from another script, you can use the following syntax:
#!/bin/bash variable1="foo" variable2="bar" variable3="baz" bash script.sh "$variable1" "$variable2" "$variable3"
When executing the script, the output will be:
The first variable is: foo The second variable is: bar The third variable is: baz
In this example, we define three variables (variable1
, variable2
, variable3
) in the calling script and pass their values as command-line arguments to the script.sh
script.
Best Practices for Handling Variables in Bash Scripts
When working with variables in Bash scripts, it is important to follow some best practices to ensure your scripts are reliable, maintainable, and secure.
1. Use descriptive variable names: Choose meaningful names for your variables that accurately describe their purpose or content. This makes your code more readable and easier to understand.
2. Use proper variable scoping: Declare your variables in the appropriate scope to prevent unintended side effects. Local variables should be declared within functions, while global variables should be declared outside of any function.
3. Initialize variables before use: Always initialize your variables before using them to avoid unexpected behavior. Uninitialized variables can lead to errors or produce incorrect results.
4. Quote variable expansions: When expanding variables, always wrap them in double quotes ("$variable"
) to prevent word splitting and pathname expansion. This ensures that the variable is treated as a single entity and preserves any special characters.
5. Avoid using global variables unless necessary: Global variables can introduce complexity and make your code more error-prone. Whenever possible, use local variables within functions to encapsulate data and prevent unintended changes.
6. Validate and sanitize user input: When accepting user input as variables, validate and sanitize the input to prevent security vulnerabilities such as code injection or unexpected behavior.
7. Use variable substitution and parameter expansion: Take advantage of Bash’s useful features for manipulating variables, such as parameter expansion, to simplify your code and make it more efficient.
8. Document your variables: Include comments or documentation to explain the purpose and usage of your variables. This helps other developers (including yourself) understand the code and its intended behavior.
Proper Variable Substitution in a Bash Script
In Bash, proper variable substitution is important to ensure that the correct value is substituted and that any special characters or spaces within the value are handled correctly.
To substitute a variable in Bash, you can use the $variable
syntax. However, there are cases where you need to use special syntax for proper substitution.
Here are some common scenarios and how to handle them:
– When substituting a variable within a string, it is recommended to enclose the variable name in curly braces to avoid ambiguity. For example:
#!/bin/bash name="John" echo "Hello, ${name}!"
– When using variables within command substitutions (enclosing a command within $()
), it is important to quote the command substitution to preserve any whitespace or special characters. For example:
#!/bin/bash files=$(ls *.txt) echo "Files: $files"
– When performing arithmetic operations with variables, you can use the $(( ))
syntax. This ensures that the result of the arithmetic operation is substituted correctly. For example:
#!/bin/bash num1=10 num2=5 result=$((num1 + num2)) echo "Result: $result"
– When substituting variables in a command that requires quoting or escaping, it is recommended to use quotes or backslashes appropriately. For example:
#!/bin/bash path="/path/with spaces" echo "Path: \"$path\""
These are just a few examples of proper variable substitution in Bash. It is important to understand the specific requirements and syntax of each scenario to ensure correct and reliable substitution.
Related Article: How to Import JSON from a Bash Script on Linux
Additional Resources
– Passing Multiple Arguments to a Bash Script
– Command Line Arguments in Bash Script with Multiple Variables