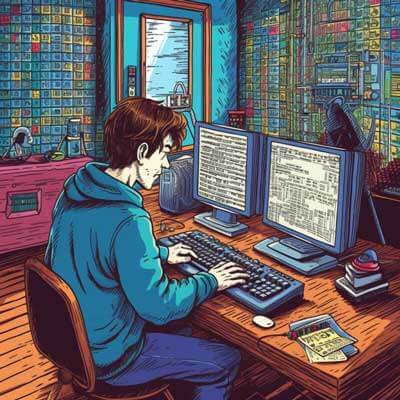
Table of Contents
In this section, we will explore some of the common string manipulation techniques in Bash.
Bash Script Quotes
In Bash scripts, quotes are used to define strings and protect them from being split into multiple words. There are three types of quotes in Bash: single quotes (''), double quotes ("") and backticks.
Single quotes are used to define literal strings, where the characters inside the quotes are treated as-is and no variable substitution or command substitution takes place. For example:
name='John' echo 'Hello, $name' # Output: Hello, $name
Double quotes, on the other hand, allow for variable substitution and command substitution. Variables inside double quotes are expanded to their values, and commands enclosed in $(...) or ...
are executed and replaced with their output. For example:
name='John' echo "Hello, $name" # Output: Hello, John echo "Today is $(date)" # Output: Today is [current date]
Backticks () are an older form of command substitution, but the recommended syntax is $(...). They are used to execute a command and replace it with its output. For example:
echo "The current directory is: `pwd`" # Output: The current directory is: [current directory]
Related Article: How to Limi Argument Inputs in Bash Scripts
Bash Remove Quotes from String
There may be situations where you need to remove quotes from a string in a Bash script. This can be done using various string manipulation techniques.
One way to remove quotes from a string is by using the sed
command. The sed
command is a useful stream editor that can perform various operations on text, including removing characters. Here's an example:
string='"Hello, World!"' string=$(echo $string | sed 's/"//g') echo $string # Output: Hello, World!
In this example, the sed
command is used to remove all occurrences of the double quote character (") from the string.
Another way to remove quotes from a string is by using parameter expansion. Bash provides various parameter expansion operators that can be used to manipulate strings. One such operator is the ${var//pattern/replacement}
syntax, which replaces all occurrences of a pattern with a replacement. Here's an example:
string='"Hello, World!"' string=${string//\"/} echo $string # Output: Hello, World!
In this example, the //\"/
pattern is used to match all occurrences of the double quote character (") and replace them with an empty string.
Bash Add Quotes to String
If you need to add quotes to a string in a Bash script, you can use string concatenation or parameter expansion.
String concatenation can be done using the +
operator. Here's an example:
name='John' greeting='Hello, ' + $name + '!' echo $greeting # Output: Hello, John!
In this example, the +
operator is used to concatenate the strings 'Hello, '
and $name
, and then concatenate the result with the string '!'
.
Parameter expansion can also be used to add quotes to a string. You can use the ${var/pattern/replacement}
syntax to replace a pattern with a replacement. Here's an example:
name='John' greeting='Hello, ${name}!' greeting=${greeting/\${name}/$name} echo $greeting # Output: Hello, John!
In this example, the /\${name}/$name
pattern is used to match the ${name}
pattern and replace it with the value of the $name
variable.
Bash String Concatenation
String concatenation is a common string manipulation operation in Bash. It allows you to combine multiple strings into a single string.
In Bash, you can perform string concatenation using the +
operator or the +=
operator.
Here's an example using the +
operator:
name='John' greeting='Hello, ' + $name + '!' echo $greeting # Output: Hello, John!
In this example, the +
operator is used to concatenate the strings 'Hello, '
, $name
, and '!'
.
Here's an example using the +=
operator:
name='John' greeting='Hello, ' greeting+=${name} greeting+='!' echo $greeting # Output: Hello, John!
In this example, the +=
operator is used to append the value of the $name
variable to the greeting
string, and then append the string '!'
to the greeting
string.
Related Article: Can a Running Bash Script be Edited in Linux?
Bash String Substitution
String substitution is a useful feature in Bash that allows you to replace parts of a string with another string.
In Bash, you can perform string substitution using parameter expansion. The ${var/pattern/replacement}
syntax is used to replace the first occurrence of a pattern with a replacement, and the ${var//pattern/replacement}
syntax is used to replace all occurrences of a pattern with a replacement.
Here's an example using the ${var/pattern/replacement}
syntax:
string='Hello, world!' string=${string/world/John} echo $string # Output: Hello, John!
In this example, the /world/John
pattern is used to match the first occurrence of the string 'world'
and replace it with the string 'John'
.
Here's an example using the ${var//pattern/replacement}
syntax:
string='Hello, world!' string=${string//o/0} echo $string # Output: Hell0, w0rld!
In this example, the /o/0
pattern is used to match all occurrences of the letter 'o'
and replace them with the number '0'
.
Bash String Variables
In Bash, string variables are used to store and manipulate strings. A string variable is defined by assigning a value to a variable name.
Here's an example of defining and using a string variable:
name='John' echo $name # Output: John
In this example, the $name
variable is defined and assigned the value 'John'
. The value of the $name
variable is then printed using the echo
command.
String variables can also be concatenated and manipulated using various string manipulation techniques. Refer to the previous sections for more information on string manipulation in Bash.
Bash String Operations
Bash provides various string operations that can be used to manipulate and compare strings. Some of the common string operations in Bash include string concatenation, string substitution, string length, string comparison, and string extraction.
Refer to the previous sections for detailed examples and explanations of these string operations.
Bash String Comparison
String comparison is a common operation in Bash scripts. It allows you to compare two strings and perform different actions based on the result of the comparison.
In Bash, you can use the ==
operator to perform string comparison. Here's an example:
name='John' if [ $name == 'John' ]; then echo "Hello, John!" else echo "Hello, stranger!" fi
In this example, the $name
variable is compared with the string 'John'
using the ==
operator. If the two strings are equal, the first branch of the if
statement is executed and the message "Hello, John!" is printed. Otherwise, the second branch of the if
statement is executed and the message "Hello, stranger!" is printed.
It's worth noting that Bash performs lexicographical comparison by default, which means that uppercase letters are considered smaller than lowercase letters. If you want to perform case-insensitive comparison, you can use the -eq
operator instead. For example:
name='John' if [ $name -eq 'john' ]; then echo "Hello, John!" else echo "Hello, stranger!" fi
In this example, the -eq
operator is used to perform case-insensitive comparison between the $name
variable and the string 'john'
.
Related Article: How to Use Multithreading in Bash Scripts on Linux
How to Extract a Substring in Bash?
In Bash, you can extract a substring from a string using parameter expansion. The ${var:start:length}
syntax is used to extract a substring starting from a specific position and with a specific length.
Here's an example:
string='Hello, world!' substring=${string:7:5} echo $substring # Output: world
In this example, the ${string:7:5}
parameter expansion is used to extract a substring starting from the 7th character of the $string
variable and with a length of 5 characters.
How to Check if a String Contains a Substring in Bash?
In Bash, you can check if a string contains a substring using the [[ string =~ substring ]]
syntax. This syntax allows you to use regular expressions to match substrings.
Here's an example:
string='Hello, world!' if [[ $string =~ world ]]; then echo "Substring found!" else echo "Substring not found!" fi
In this example, the [[ $string =~ world ]]
expression is used to check if the $string
variable contains the substring 'world'
. If the substring is found, the message "Substring found!" is printed. Otherwise, the message "Substring not found!" is printed.
How to Convert a String to Lowercase in Bash?
In Bash, you can convert a string to lowercase using parameter expansion and the ${var,,}
syntax.
Here's an example:
string='Hello, World!' lowercase=${string,,} echo $lowercase # Output: hello, world!
In this example, the ${string,,}
parameter expansion is used to convert the $string
variable to lowercase.
How to Split a String into an Array in Bash?
In Bash, you can split a string into an array using the read
command and the -a
option.
Here's an example:
string='Hello, World!' read -a array <<< $string echo ${array[0]} # Output: Hello, echo ${array[1]} # Output: World!
In this example, the <<< $string
syntax is used to pass the $string
variable as input to the read
command. The -a
option is used to store the words of the string in the array
variable. The elements of the array can then be accessed using the ${array[index]}
syntax.
Related Article: How To Find All Files With Text On Linux
How to Replace All Occurrences of a Substring in a String in Bash?
In Bash, you can replace all occurrences of a substring in a string using parameter expansion and the ${var//pattern/replacement}
syntax.
Here's an example:
string='Hello, world!' new_string=${string//o/0} echo $new_string # Output: Hell0, w0rld!
In this example, the ${string//o/0}
parameter expansion is used to replace all occurrences of the letter 'o'
in the $string
variable with the number '0'
.
How to Trim Leading and Trailing Whitespace from a String in Bash?
In Bash, you can trim leading and trailing whitespace from a string using parameter expansion and the ${var#pattern}
and ${var%pattern}
syntaxes.
Here's an example:
string=' Hello, world! ' trimmed_string=${string##*( )} trimmed_string=${trimmed_string%%*( )} echo $trimmed_string # Output: Hello, world!
In this example, the ${string##*( )}
parameter expansion is used to trim leading whitespace from the $string
variable, and the ${trimmed_string%%*( )}
parameter expansion is used to trim trailing whitespace from the $trimmed_string
variable.
How to Count the Number of Characters in a String in Bash?
In Bash, you can count the number of characters in a string using parameter expansion and the ${#var}
syntax.
Here's an example:
string='Hello, world!' count=${#string} echo $count # Output: 13
In this example, the ${#string}
parameter expansion is used to count the number of characters in the $string
variable.
How to Reverse a String in Bash?
In Bash, you can reverse a string using parameter expansion and the ${var:offset:length}
syntax.
Here's an example:
string='Hello, world!' reversed_string="" for (( i=${#string}-1; i>=0; i-- )); do reversed_string+=${string:i:1} done echo $reversed_string # Output: !dlrow ,olleH
In this example, a loop is used to iterate over each character of the $string
variable in reverse order. The ${string:i:1}
parameter expansion is used to extract each character, and the +=
operator is used to append it to the reversed_string
variable.
Related Article: Parent Variable Accessibility in Bash Scripts
How to Find the Index of a Substring in a String in Bash?
In Bash, you can find the index of a substring in a string using parameter expansion and the ${var#pattern}
syntax.
Here's an example:
string='Hello, world!' substring='world' index=${string%%$substring*} echo ${#index} # Output: 7
In this example, the ${string%%$substring*}
parameter expansion is used to remove the substring $substring
and everything after it from the $string
variable, leaving only the characters before the substring. The ${#index}
parameter expansion is then used to count the number of characters in the $index
variable, which represents the index of the substring.
How to Compare Two Strings in Bash?
In Bash, you can compare two strings using the ==
operator or the [[ string == pattern ]]
syntax.
Here's an example using the ==
operator:
string1='Hello, world!' string2='Hello, John!' if [ $string1 == $string2 ]; then echo "Equal" else echo "Not equal" fi
In this example, the $string1
variable is compared with the $string2
variable using the ==
operator. If the two strings are equal, the message "Equal" is printed. Otherwise, the message "Not equal" is printed.
Here's an example using the [[ string == pattern ]]
syntax:
string1='Hello, world!' if [[ $string1 == Hello* ]]; then echo "Starts with Hello" else echo "Does not start with Hello" fi
In this example, the [[ $string1 == Hello* ]]
expression is used to check if the $string1
variable starts with the string 'Hello'
. If the condition is true, the message "Starts with Hello" is printed. Otherwise, the message "Does not start with Hello" is printed.