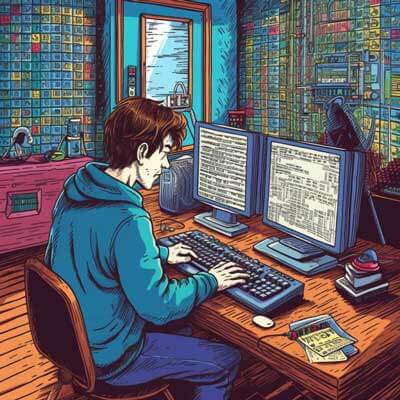
- Using Regular Expressions to Extract Numbers from a String in a Bash Script
- Ways to Use Awk to Extract Numbers from a String in a Bash Script
- Examples of Using Cut to Extract Numbers from a String in a Bash Script
- Using the tr Command to Extract Numbers from a String in a Bash Script
- The expr Command and Its Usage to Extract Numbers from a String in a Bash Script
- String Manipulation Techniques to Extract Numbers from a String in a Bash Script
- Tips for Shell Scripting to Extract Numbers from a String in a Bash Script
- Extracting Numbers from a String Using Command Line Tools in Linux
In a bash script, it is often necessary to extract specific information from strings. One common task is extracting numbers from a string. This can be useful in various scenarios, such as parsing log files, processing command output, or manipulating data. In this article, we will explore different approaches to extract numbers from strings in a bash script, focusing on Linux command-line tools.
Using Regular Expressions to Extract Numbers from a String in a Bash Script
Regular expressions are a useful tool for pattern matching and can be used to extract numbers from a string in a bash script. The grep
command is commonly used with regular expressions to search for patterns in text. Here’s an example of using grep
to extract numbers from a string:
#!/bin/bash string="The price of the product is $10.99" number=$(echo "$string" | grep -oE '[0-9]+([.][0-9]+)?') echo "Number: $number"
In this example, we use the -o
option with grep
to only output the matching part of the string. The regular expression [0-9]+([.][0-9]+)?
matches one or more digits, followed by an optional decimal point and one or more digits. The extracted number is then stored in the variable number
and printed.
Another useful command for working with regular expressions is sed
. sed
can be used to perform text transformations and substitutions, including extracting numbers from a string. Here’s an example:
#!/bin/bash string="The price of the product is $10.99" number=$(echo "$string" | sed -nE 's/.*([0-9]+([.][0-9]+)?).*/\1/p') echo "Number: $number"
In this example, we use the -n
option with sed
to suppress automatic printing and the -E
option to enable extended regular expressions. The s/.*([0-9]+([.][0-9]+)?).*/\1/p
command matches the entire string and captures the number using parentheses. The captured number is then printed using the \1
backreference.
Related Article: How To Echo a Newline In Bash
Ways to Use Awk to Extract Numbers from a String in a Bash Script
Awk is a versatile command-line tool for text processing, and it can also be used to extract numbers from a string in a bash script. Here’s an example:
#!/bin/bash string="The price of the product is $10.99" number=$(echo "$string" | awk '{ for(i=1; i<=NF; i++) if($i ~ /^[0-9]+([.][0-9]+)?$/) print $i }') echo "Number: $number"
In this example, we use awk
to iterate over each field ($i
) in the input string and check if it matches the regular expression /^[0-9]+([.][0-9]+)?$/
. If a field is a number, it is printed. The extracted number is then stored in the variable number
and printed.
Another way to use awk
to extract numbers from a string is by setting the field separator (FS
) to a regular expression that matches non-digit characters. Here’s an example:
#!/bin/bash string="The price of the product is $10.99" number=$(echo "$string" | awk 'BEGIN { FS = "[^0-9.]+" } { for(i=1; i<=NF; i++) if($i != "") print $i }') echo "Number: $number"
In this example, we use the BEGIN
block to set the field separator (FS
) to a regular expression that matches any non-digit characters. The for
loop then iterates over each field ($i
) and prints it if it is not empty. The extracted number is stored in the variable number
and printed.
Examples of Using Cut to Extract Numbers from a String in a Bash Script
The cut
command is primarily used for cutting out sections from lines of files, but it can also be used to extract numbers from a string in a bash script. Here’s an example:
#!/bin/bash string="The price of the product is $10.99" number=$(echo "$string" | cut -d'$' -f2 | cut -d' ' -f1) echo "Number: $number"
In this example, we use the -d
option with cut
to specify the delimiter as the dollar sign ($
). The -f
option is used to specify the field, in this case, the second field after the delimiter. We then use another cut
command with the space character (
) as the delimiter to extract the first field. The extracted number is stored in the variable number
and printed.
Another way to use cut
to extract numbers from a string is by specifying a character range using the -c
option. Here’s an example:
#!/bin/bash string="The price of the product is $10.99" number=$(echo "$string" | cut -c19-24) echo "Number: $number"
In this example, we use the -c
option with cut
to specify the character range from the 19th to the 24th characters. This range corresponds to the number 10.99
in the input string. The extracted number is stored in the variable number
and printed.
Using the tr Command to Extract Numbers from a String in a Bash Script
The tr
command is primarily used for translating or deleting characters, but it can also be used to extract numbers from a string in a bash script. Here’s an example:
#!/bin/bash string="The price of the product is $10.99" number=$(echo "$string" | tr -cd '[:digit:].') echo "Number: $number"
In this example, we use the -cd
option with tr
to delete all characters except digits ([:digit:]
) and the decimal point (.
). The extracted number is stored in the variable number
and printed.
Related Article: How to Use If-Else Statements in Shell Scripts
The expr Command and Its Usage to Extract Numbers from a String in a Bash Script
The expr
command is primarily used for evaluating expressions, but it can also be used to extract numbers from a string in a bash script. Here’s an example:
#!/bin/bash string="The price of the product is $10.99" number=$(expr "$string" : '.*\([$][0-9]\{1,\}\.[0-9]\{2,\}\)') echo "Number: $number"
In this example, we use the expr
command with the :
operator to match the regular expression .*\([$][0-9]\{1,\}\.[0-9]\{2,\}\)
. This regular expression matches a dollar sign ($
) followed by one or more digits and a decimal point, followed by two or more digits. The matched number is then stored in the variable number
and printed.
String Manipulation Techniques to Extract Numbers from a String in a Bash Script
In addition to using command-line tools, there are also string manipulation techniques that can be used to extract numbers from a string in a bash script. Here’s an example:
#!/bin/bash string="The price of the product is $10.99" number="${string//[!0-9.]}" number="${number%.}" number="${number%.*}" echo "Number: $number"
In this example, we use parameter expansion to remove all non-digit and non-decimal point characters from the string (${string//[!0-9.]}
). We then use ${number%.}
to remove any trailing decimal point and ${number%.*}
to remove any fractional part. The extracted number is stored in the variable number
and printed.
Tips for Shell Scripting to Extract Numbers from a String in a Bash Script
When working with shell scripting to extract numbers from a string in a bash script, here are some tips to keep in mind:
1. Use appropriate command-line tools: There are various command-line tools available in Linux that are specifically designed for text processing. Choose the tool that best fits your requirements and use it accordingly.
2. Understand regular expressions: Regular expressions are useful tools for pattern matching. Familiarize yourself with regular expression syntax and use it effectively to extract numbers from strings.
3. Handle edge cases: Consider edge cases where the string might not contain a number or might contain multiple numbers. Make sure your script handles these cases gracefully.
4. Test your script: Before using your script in a production environment, thoroughly test it with different input strings to ensure it works as expected.
Related Article: Executing a Bash Script with Multivariables in Linux
Extracting Numbers from a String Using Command Line Tools in Linux
In this section, we will summarize the different command-line tools and techniques discussed in this article for extracting numbers from a string in a bash script:
– Using regular expressions with grep
or sed
: Regular expressions provide a flexible way to match and extract numbers from strings. Use the -o
option with grep
or the s
command with sed
to extract the matched part of the string.
– Using awk
: awk
is a useful tool for text processing and can be used to extract numbers by iterating over each field in the input string and checking if it matches a regular expression.
– Using cut
: cut
is primarily used for cutting out sections from lines of files, but it can also be used to extract numbers by specifying the delimiter or character range that surrounds the number.
– Using the tr
command: The tr
command can be used to delete all characters except digits and the decimal point, effectively extracting the number from the string.
– Using the expr
command: The expr
command can be used with regular expressions to extract numbers from strings. Match the string against a regular expression and use the matched part as the extracted number.
– Using string manipulation techniques: Parameter expansion and string manipulation can be used to remove non-digit and non-decimal point characters from the string, effectively extracting the number.