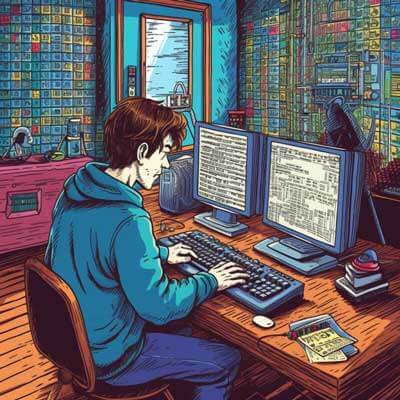
Table of Contents
To declare a function in bash, you need to use the function
keyword followed by the name of the function and a set of parentheses. The function name should follow the same naming conventions as variables in bash, which means it can contain letters, numbers, and underscores, but it cannot start with a number.
Here is an example of how to declare a function in bash:
function greet() { echo "Hello, World!" }
In this example, we declare a function named greet
that simply echoes the string "Hello, World!".
What is the syntax for defining a function in bash?
The syntax for defining a function in bash is similar to declaring a function, but it includes a set of curly braces {}
that enclose the body of the function. The body of the function consists of a series of commands that will be executed when the function is called.
Here is an example of how to define a function in bash:
function greet() { echo "Hello, $1!" }
In this example, we define a function named greet
that takes one argument. The argument is referenced using the $1
syntax within the function body. When the function is called, the value of the argument will be substituted in place of $1
.
Related Article: How to Use Mkdir Only If a Directory Doesn't Exist in Linux
How do you call a function in a bash script?
To call a function in a bash script, you simply use the name of the function followed by a set of parentheses. If the function takes arguments, you can pass them within the parentheses.
Here is an example of how to call a function in bash:
function greet() { echo "Hello, $1!" } greet "John"
In this example, we define a function named greet
that takes one argument. We then call the greet
function passing the argument "John". The function will be executed, and the output will be "Hello, John!".
How can functions enhance a bash script?
Functions can enhance a bash script in several ways:
- Code reuse: Functions allow you to define reusable blocks of code that can be called multiple times from different parts of the script. This promotes modularity and reduces code duplication.
- Modularity: By breaking down a script into smaller functions, you can organize the code into logical units, making it easier to understand and maintain.
- Abstraction: Functions provide a way to abstract complex operations into a single, high-level command. This makes the script more readable and easier to use.
- Error handling: Functions can be used to encapsulate error handling logic, making it easier to handle and recover from errors in a consistent manner.
- Testing: Functions can be individually tested, which makes it easier to debug and verify the correctness of the code.
In addition to these benefits, functions can also improve code readability, promote code reuse, and make the script more modular and maintainable.
Code Snippet: Function declaration in bash
function greet() { echo "Hello, World!" }
This code snippet demonstrates how to declare a function named greet
that simply echoes the string "Hello, World!".
Related Article: How to Use the Ls Command in Linux
Code Snippet: Function definition in bash
function greet() { echo "Hello, $1!" }
This code snippet demonstrates how to define a function named greet
that takes one argument and echoes a personalized greeting using the value of the argument.
Code Snippet: Function call in bash
function greet() { echo "Hello, $1!" } greet "John"
This code snippet demonstrates how to call the greet
function and pass the argument "John". The function will be executed, and the output will be "Hello, John!".
Additional Resources
- Bash Functions