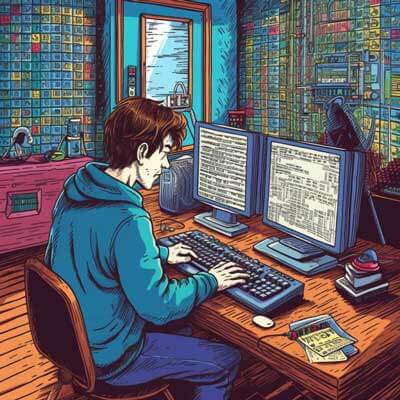
- Code Snippet: Comparing Array Sizes Using a Loop
- Code Snippet: Comparing Array Sizes Using the ‘wc’ Command
- Code Snippet: Comparing Array Sizes Using the ‘expr’ Command
- Code Snippet: Comparing Array Sizes Using the ‘test’ Command
- Code Snippet: Comparing Array Sizes Using the ‘if’ Statement
- Code Snippet: Comparing Array Sizes Using the ‘[[ … ]]’ Construct
- Code Snippet: Comparing Array Sizes Using the ‘declare’ Command
- Code Snippet: Comparing Array Sizes Using the ‘for’ Loop
- Code Snippet: Comparing Array Sizes Using the ‘let’ Command
- Code Snippet: Comparing Array Sizes Using the ‘sort’ Command
- What is the Best Way to Compare the Length of Arrays in Bash Scripting?
- How Do I Check if Two Arrays in Bash Have the Same Size?
- Is There a Command in Bash to Compare the Size of Arrays?
- Can I Compare the Dimensions of Arrays in Bash Scripting?
- What is the Syntax to Compare the Sizes of Arrays in Bash Scripting?
- Are There Any Built-in Functions in Bash to Compare Array Lengths?
- How Can I Determine if One Array is Larger Than Another in Bash Scripting?
- What is the Recommended Approach to Compare Array Sizes in Bash Scripting?
Array manipulation is a common task in bash scripting, and sometimes it becomes necessary to compare the sizes of arrays. Whether you want to check if two arrays have the same length or determine if one array is larger than another, there are several approaches you can take to achieve this in bash scripting on Linux. In this article, we will explore different methods to compare array sizes and provide code snippets for each approach.
Code Snippet: Comparing Array Sizes Using a Loop
To compare the sizes of arrays using a loop in bash scripting, you can iterate over each array and count the number of elements. Here’s an example code snippet that demonstrates this approach:
array1=("apple" "banana" "cherry") array2=("apple" "banana" "cherry" "date") count1=0 for element in "${array1[@]}"; do count1=$((count1 + 1)) done count2=0 for element in "${array2[@]}"; do count2=$((count2 + 1)) done if [ $count1 -eq $count2 ]; then echo "Array sizes are equal" else echo "Array sizes are not equal" fi
In this code snippet, we use two arrays, array1
and array2
, and iterate over each array using a loop. We increment a counter variable, count1
and count2
, for each element in the respective arrays. Finally, we compare the counts using an if
statement and output the result.
Related Article: How To Echo a Newline In Bash
Code Snippet: Comparing Array Sizes Using the ‘wc’ Command
Another method to compare the sizes of arrays in bash scripting is by utilizing the wc
command to count the lines of output from the arrays. Here’s an example code snippet that demonstrates this approach:
array1=("apple" "banana" "cherry") array2=("apple" "banana" "cherry" "date") count1=$(printf "%s\n" "${array1[@]}" | wc -l) count2=$(printf "%s\n" "${array2[@]}" | wc -l) if [ $count1 -eq $count2 ]; then echo "Array sizes are equal" else echo "Array sizes are not equal" fi
In this code snippet, we use the printf
command to print each element of the arrays on separate lines. We then pipe the output to the wc
command, which counts the lines and outputs the total count. Finally, we compare the counts using an if
statement and output the result.
Code Snippet: Comparing Array Sizes Using the ‘expr’ Command
You can also compare the sizes of arrays in bash scripting using the expr
command to perform arithmetic operations and compare the lengths. Here’s an example code snippet that demonstrates this approach:
array1=("apple" "banana" "cherry") array2=("apple" "banana" "cherry" "date") count1=$(expr ${#array1[@]}) count2=$(expr ${#array2[@]}) if [ $count1 -eq $count2 ]; then echo "Array sizes are equal" else echo "Array sizes are not equal" fi
In this code snippet, we use the ${#array[@]}
syntax to get the length of each array. The expr
command evaluates the arithmetic expression and assigns the result to the count1
and count2
variables. Finally, we compare the counts using an if
statement and output the result.
Code Snippet: Comparing Array Sizes Using the ‘test’ Command
The test
command, also known as [
, can be used to compare the sizes of arrays in bash scripting. Here’s an example code snippet that demonstrates this approach:
array1=("apple" "banana" "cherry") array2=("apple" "banana" "cherry" "date") if [ ${#array1[@]} -eq ${#array2[@]} ]; then echo "Array sizes are equal" else echo "Array sizes are not equal" fi
In this code snippet, we use the ${#array[@]}
syntax to get the length of each array. The [
command compares the counts using the -eq
operator and outputs the result based on the condition.
Related Article: How to Use If-Else Statements in Shell Scripts
Code Snippet: Comparing Array Sizes Using the ‘if’ Statement
The if
statement in bash scripting can be used to compare the sizes of arrays. Here’s an example code snippet that demonstrates this approach:
array1=("apple" "banana" "cherry") array2=("apple" "banana" "cherry" "date") if [ ${#array1[@]} -eq ${#array2[@]} ]; then echo "Array sizes are equal" else echo "Array sizes are not equal" fi
In this code snippet, we use the ${#array[@]}
syntax to get the length of each array. The if
statement compares the counts using the -eq
operator and outputs the result based on the condition.
Code Snippet: Comparing Array Sizes Using the ‘[[ … ]]’ Construct
The [[ ... ]]
construct in bash scripting provides more advanced conditional expressions and can be used to compare the sizes of arrays. Here’s an example code snippet that demonstrates this approach:
array1=("apple" "banana" "cherry") array2=("apple" "banana" "cherry" "date") if [[ ${#array1[@]} -eq ${#array2[@]} ]]; then echo "Array sizes are equal" else echo "Array sizes are not equal" fi
In this code snippet, we use the ${#array[@]}
syntax to get the length of each array. The [[ ... ]]
construct compares the counts using the -eq
operator and outputs the result based on the condition.
Code Snippet: Comparing Array Sizes Using the ‘declare’ Command
The declare
command in bash scripting can be used to compare the sizes of arrays by assigning the lengths to variables. Here’s an example code snippet that demonstrates this approach:
array1=("apple" "banana" "cherry") array2=("apple" "banana" "cherry" "date") declare -i count1=${#array1[@]} declare -i count2=${#array2[@]} if [ $count1 -eq $count2 ]; then echo "Array sizes are equal" else echo "Array sizes are not equal" fi
In this code snippet, we use the ${#array[@]}
syntax to get the length of each array. The declare -i
command assigns the lengths to the count1
and count2
variables as integers. Finally, we compare the counts using an if
statement and output the result.
Related Article: Executing a Bash Script with Multivariables in Linux
Code Snippet: Comparing Array Sizes Using the ‘for’ Loop
You can also compare the sizes of arrays in bash scripting using a for
loop to iterate over the arrays and count the elements. Here’s an example code snippet that demonstrates this approach:
array1=("apple" "banana" "cherry") array2=("apple" "banana" "cherry" "date") count1=0 for element in "${array1[@]}"; do count1=$((count1 + 1)) done count2=0 for element in "${array2[@]}"; do count2=$((count2 + 1)) done if [ $count1 -eq $count2 ]; then echo "Array sizes are equal" else echo "Array sizes are not equal" fi
In this code snippet, we use two arrays, array1
and array2
, and iterate over each array using a for
loop. We increment a counter variable, count1
and count2
, for each element in the respective arrays. Finally, we compare the counts using an if
statement and output the result.
Code Snippet: Comparing Array Sizes Using the ‘let’ Command
The let
command in bash scripting can be used to compare the sizes of arrays by performing arithmetic operations and comparing the lengths. Here’s an example code snippet that demonstrates this approach:
array1=("apple" "banana" "cherry") array2=("apple" "banana" "cherry" "date") let count1=${#array1[@]} let count2=${#array2[@]} if [ $count1 -eq $count2 ]; then echo "Array sizes are equal" else echo "Array sizes are not equal" fi
In this code snippet, we use the ${#array[@]}
syntax to get the length of each array. The let
command performs the arithmetic operations and assigns the lengths to the count1
and count2
variables. Finally, we compare the counts using an if
statement and output the result.
Code Snippet: Comparing Array Sizes Using the ‘sort’ Command
The sort
command in bash scripting can be used to compare the sizes of arrays indirectly by sorting the arrays and checking if they are identical. Here’s an example code snippet that demonstrates this approach:
array1=("apple" "banana" "cherry") array2=("apple" "banana" "cherry" "date") sorted_array1=($(printf '%s\n' "${array1[@]}" | sort)) sorted_array2=($(printf '%s\n' "${array2[@]}" | sort)) if [ "${sorted_array1[*]}" == "${sorted_array2[*]}" ]; then echo "Array sizes are equal" else echo "Array sizes are not equal" fi
In this code snippet, we use the printf
command to print each element of the arrays on separate lines. We then pipe the output to the sort
command, which sorts the lines alphabetically. Finally, we compare the sorted arrays using an if
statement and output the result.
Related Article: How to Manipulate Quotes & Strings in Bash Scripts
What is the Best Way to Compare the Length of Arrays in Bash Scripting?
The best way to compare the length of arrays in bash scripting depends on the specific requirements of your script. Different methods have their advantages and disadvantages, such as performance, readability, and complexity. It’s important to choose the approach that suits your needs and maintains the integrity of your script. In the following sections, we will present various techniques and provide code snippets to compare array sizes, allowing you to decide which method is best for your use case.
How Do I Check if Two Arrays in Bash Have the Same Size?
To check if two arrays in bash have the same size, you can compare the lengths of the arrays using conditional statements or command substitution. By comparing the count of elements in each array, you can determine if they have the same size.
Is There a Command in Bash to Compare the Size of Arrays?
Bash does not have a built-in command specifically designed to compare the size of arrays. However, you can utilize other commands and constructs to achieve this functionality. For example, you can use the wc
command to count the lines of output from the arrays and compare them. Alternatively, you can use the expr
command to perform arithmetic operations and compare the lengths of arrays.
Related Article: How to Import JSON from a Bash Script on Linux
Can I Compare the Dimensions of Arrays in Bash Scripting?
In bash scripting, arrays are one-dimensional, meaning they can only have a single dimension. Therefore, you cannot directly compare the dimensions of arrays in bash scripting. If you need to work with multi-dimensional arrays, you may consider using other programming languages or data structures that support such functionality.
What is the Syntax to Compare the Sizes of Arrays in Bash Scripting?
The syntax to compare the sizes of arrays in bash scripting depends on the specific method you choose to use. The general approach involves comparing the counts or lengths of the arrays using conditional statements, command substitution, or arithmetic operations.
Are There Any Built-in Functions in Bash to Compare Array Lengths?
Bash does not provide built-in functions specifically for comparing array lengths. However, you can use various commands and constructs to achieve the desired functionality. For instance, you can utilize loops, conditional statements, command substitution, and arithmetic operations to compare the lengths of arrays.
Related Article: Appending Dates to Filenames in Bash Scripts
How Can I Determine if One Array is Larger Than Another in Bash Scripting?
To determine if one array is larger than another in bash scripting, you can compare the lengths of the arrays using conditional statements or command substitution. By comparing the count of elements in each array, you can determine if one is larger than the other.
What is the Recommended Approach to Compare Array Sizes in Bash Scripting?
The recommended approach to compare array sizes in bash scripting depends on the specific requirements of your script and the complexity of your arrays. If you are working with small arrays, using a loop to count the elements may be sufficient. However, if you are dealing with large arrays, it may be more efficient to use commands like wc
or expr
to compare the sizes. It’s important to consider factors such as performance, readability, and maintainability when choosing the approach that best suits your needs.