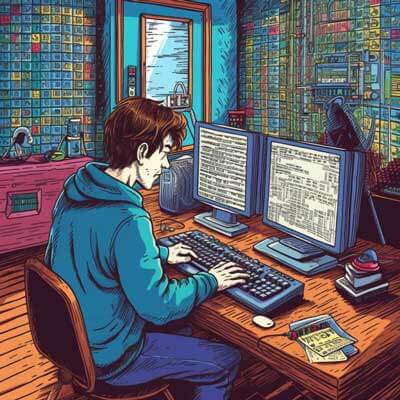
- Comparing Two Files Using the diff Command
- Comparing Files with the cmp Command
- Comparing Files Using the comm Command
- Comparing Files with the File Comparison Operators
- Comparing Files Using Awk
- Comparing Files with diffstat
- Comparing Files Using Vimdiff
- Comparing Files with Meld
- Comparing Files Using Git Diff
- Comparing Files Using Kdiff3
- Comparing Files with Diffuse
Comparing Two Files Using the diff Command
The diff
command is a useful tool in Linux that allows you to compare two files line by line. It displays the differences between the files in a user-friendly format, making it easy to identify changes. Here’s how you can use the diff
command to compare two files:
$ diff file1.txt file2.txt
This command will compare file1.txt
and file2.txt
and display the differences between them. If there are no differences, the command will not produce any output.
You can also use the -q
option to get a brief summary of whether the files are different or not:
$ diff -q file1.txt file2.txt
If the files are different, the command will display the names of the files. If the files are identical, the command will not produce any output.
Related Article: How To Echo a Newline In Bash
Comparing Files with the cmp Command
The cmp
command is another useful tool for comparing two files in Bash script. Unlike the diff
command, which displays the differences between the files, the cmp
command compares the binary contents of the files byte by byte. Here’s how you can use the cmp
command:
$ cmp file1.txt file2.txt
If the files are different, the command will display the byte and line numbers where the first difference occurs. If the files are identical, the command will not produce any output.
You can also use the -s
option to get a brief summary of whether the files are different or not:
$ cmp -s file1.txt file2.txt
If the files are different, the command will exit with a non-zero status. If the files are identical, the command will exit with a zero status.
Comparing Files Using the comm Command
The comm
command is another useful tool for comparing two sorted files in Bash script. It compares the contents of two files line by line and displays the lines that are unique to each file, as well as the lines that are common to both files. Here’s how you can use the comm
command:
$ comm file1.txt file2.txt
This command will display three columns: the lines unique to file1.txt
, the lines unique to file2.txt
, and the lines common to both files.
You can also use the -1
, -2
, or -3
options to suppress the output of certain columns:
$ comm -1 file1.txt file2.txt # Suppress lines unique to file1.txt $ comm -2 file1.txt file2.txt # Suppress lines unique to file2.txt $ comm -3 file1.txt file2.txt # Suppress lines common to both files
Comparing Files with the File Comparison Operators
In Bash script, you can also use the file comparison operators to compare two files. The operators allow you to check if two files are identical, if one file is newer than the other, or if one file is older than the other. Here are the file comparison operators:
– -eq
: True if the files have the same inode number and device number.
– -nt
: True if the first file is newer than the second file.
– -ot
: True if the first file is older than the second file.
Here’s an example of how you can use the file comparison operators:
if [ file1.txt -eq file2.txt ]; then echo "The files are identical" fi if [ file1.txt -nt file2.txt ]; then echo "file1.txt is newer than file2.txt" fi if [ file1.txt -ot file2.txt ]; then echo "file1.txt is older than file2.txt" fi
Related Article: How to Use If-Else Statements in Shell Scripts
Comparing Files Using Awk
Awk is a useful text processing tool in Linux that can also be used to compare two files. You can use Awk to compare the contents of two files line by line and perform custom actions based on the comparison result. Here’s an example of how you can use Awk to compare two files:
$ awk 'NR==FNR{a[$0];next} !($0 in a)' file1.txt file2.txt
This command will compare the contents of file1.txt
and file2.txt
and print the lines that are unique to file2.txt
. If you want to print the lines that are unique to file1.txt
, you can simply swap the file names.
You can also use Awk to perform more complex comparisons, such as comparing specific columns or using regular expressions. Awk provides a rich set of built-in functions and operators that can be used to manipulate and compare text.
Comparing Files with diffstat
Diffstat is a tool that takes the output of the diff
command and produces a summary of the differences between two files. It displays the number of added, modified, and deleted lines, making it easy to get a quick overview of the changes. Here’s how you can use diffstat to compare two files:
$ diff file1.txt file2.txt | diffstat
This command will compare file1.txt
and file2.txt
using the diff
command and display a summary of the differences using diffstat.
Diffstat is a useful tool for quickly identifying the nature and extent of the changes between two files.
Comparing Files Using Vimdiff
Vimdiff is a command-line tool that allows you to compare two files in a side-by-side view using the Vim text editor. It provides a useful and flexible interface for comparing and merging files. Here’s how you can use Vimdiff to compare two files:
$ vimdiff file1.txt file2.txt
This command will open the files file1.txt
and file2.txt
in Vimdiff. The differences between the files will be highlighted, making it easy to navigate and understand the changes.
Vimdiff provides a wide range of commands and options for navigating, merging, and resolving conflicts between files. It is a great tool for working with multiple versions of a file.
Related Article: How to Manipulate Quotes & Strings in Bash Scripts
Comparing Files with Meld
Meld is a graphical tool that allows you to compare and merge files in Linux. It provides a user-friendly interface for visualizing and resolving differences between files. Here’s how you can use Meld to compare two files:
$ meld file1.txt file2.txt
This command will open the files file1.txt
and file2.txt
in Meld. The differences between the files will be displayed in a side-by-side view, making it easy to compare and understand the changes.
Meld provides a range of features for merging and resolving conflicts between files. It is a useful tool for managing changes in your codebase.
Comparing Files Using Git Diff
If your files are in a Git repository, you can use the git diff
command to compare the changes between two versions of a file. This command compares the changes between the working directory and the specified Git commit or branch. Here’s how you can use git diff
to compare two files:
$ git diff commit1 commit2 file.txt
This command will compare the changes between commit1
and commit2
for the file file.txt
. The differences between the versions will be displayed in the unified diff format.
You can also use the git difftool
command to open a visual diff tool, such as Meld or Vimdiff, to compare the changes between two versions of a file.
Comparing Files Using Kdiff3
Kdiff3 is an open-source tool that provides a graphical interface for comparing and merging files. It supports a wide range of file formats and provides advanced features for comparing and synchronizing files. Here’s how you can use Kdiff3 to compare two files:
1. Open Kdiff3.
2. Click on the “File” menu and select “Compare” to open the comparison dialog.
3. Select the two files you want to compare.
4. Click on the “Compare” button.
Kdiff3 will open the files in a side-by-side view, highlighting the differences between them. You can navigate through the differences and merge the changes as needed.
Kdiff3 is a popular tool for comparing and merging files, especially among Linux users. It provides a simple and intuitive interface for managing changes in your codebase.
Related Article: How to Import JSON from a Bash Script on Linux
Comparing Files with Diffuse
Diffuse is an open-source graphical tool for comparing and merging files. It provides a user-friendly interface for visualizing and resolving differences between files. Here’s how you can use Diffuse to compare two files:
1. Open Diffuse.
2. Click on the “File” menu and select “Open” to open the first file.
3. Click on the “File” menu again and select “Open” to open the second file.
4. Diffuse will open the files in a side-by-side view, highlighting the differences between them.
Diffuse provides a range of features for merging and resolving conflicts between files. It is a useful tool for managing changes in your codebase.