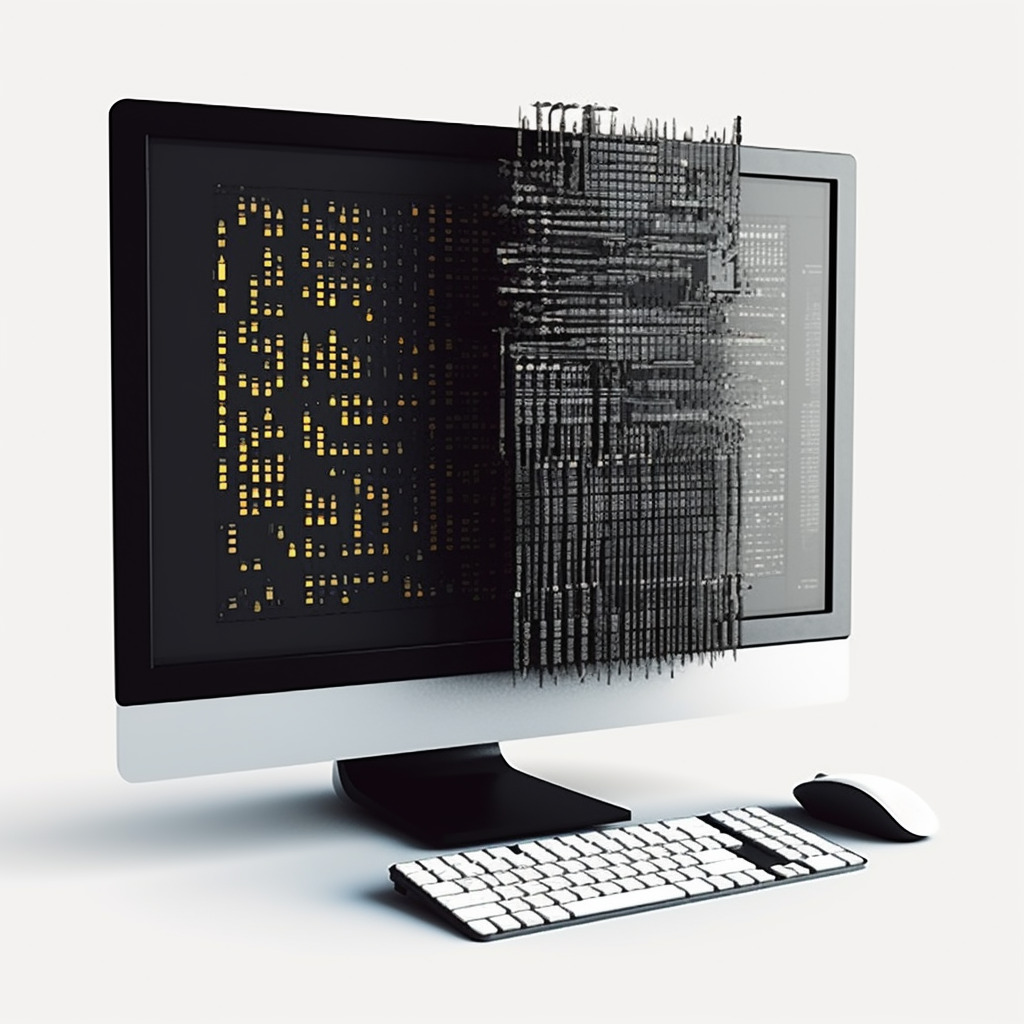
- What is Python Memory Management?
- Memory Allocation in Python
- Garbage Collection
- Memory Management Techniques
- 1. Memory Pool
- 2. Memory Fragmentation
- 3. Object Reuse
- Memory Profiling
- Memory Deallocation and Garbage Collection
- Garbage Collection in Action
- Controlling Garbage Collection
- Memory Leaks and How to Detect Them
- What is a Memory Leak?
- Causes of Memory Leaks
- Detecting Memory Leaks
- Preventing Memory Leaks
- Common Memory Management Issues and Solutions
- Memory Fragmentation
- Excessive Memory Usage
- Memory Management in Data Structures
- Memory Management in Functions
- Memory Optimization Techniques
- 1. Use Generators
- 2. Use Data Structures Wisely
- 3. Use Memory Views
- 4. Use Weak References
- Real World Examples of Python Memory Management
- Advanced Memory Management Techniques
- 1. Reference Counting and Garbage Collection
- 2. Weak References
- 3. Object Pooling
- 5. External Libraries for Memory Management
- Memory Management Best Practices
- 1. Use Generators
- 2. Avoid Unnecessary Copies
- 3. Use Context Managers
- 4. Dispose of Unreferenced Objects
- 5. Use Data Structures Wisely
- 6. Profile and Optimize
What is Python Memory Management?
Python is an interpreted, high-level programming language that is widely used for a variety of applications. One of the key features of Python is its automatic memory management, which handles the allocation and deallocation of memory for your program. Understanding how Python manages memory is crucial for writing efficient and bug-free code.
In Python, memory management is handled by a private heap space. The heap is a region of memory where objects are stored and managed. Python’s memory manager takes care of allocating memory for new objects and freeing memory for objects that are no longer in use. This automatic memory management relieves the programmer from the burden of manually managing memory, as in other languages like C or C++.
Python uses a technique called reference counting to keep track of objects in memory. Each object in Python has a reference count associated with it, which is incremented whenever a new reference to the object is created, and decremented whenever a reference to the object is deleted or goes out of scope. When an object’s reference count reaches zero, it means that there are no more references to the object, and the memory occupied by the object can be freed.
Let’s consider an example to understand how reference counting works:
a = 10 # reference count of 10 is created for the integer object with value 10 b = a # reference count of the integer object is incremented to 20 c = b # reference count of the integer object is incremented to 30 del a # reference count of the integer object is decremented to 20 del b # reference count of the integer object is decremented to 10 c = None # reference count of the integer object is decremented to 0, memory is freed
While reference counting is a simple and efficient technique, it has limitations. It cannot detect reference cycles, where objects reference each other in a circular manner. To handle reference cycles, Python uses a technique called garbage collection.
Python’s garbage collector periodically checks for objects that are no longer reachable from the root of the object graph and frees the memory occupied by these objects. The garbage collector uses an algorithm called Mark and Sweep, which marks all the objects that are reachable from the root and then sweeps through the memory, freeing the memory occupied by the unmarked objects.
Python’s garbage collector is designed to be unobtrusive and efficient. It runs in the background and only kicks in when necessary. However, it’s important to note that the garbage collector can introduce some overhead, especially in applications that create and destroy a large number of objects frequently.
To learn more about Python’s memory management, refer to the official Python documentation: https://docs.python.org/3/library/gc.html.
Related Article: How to Execute a Program or System Command in Python
Memory Allocation in Python
Python manages memory allocation using a combination of techniques. It utilizes a private heap space, which is a portion of the computer’s memory dedicated to the Python interpreter. The interpreter allocates memory dynamically as needed, and deallocation is automatically handled by a garbage collector.
Garbage Collection
Garbage collection is the process of automatically reclaiming memory that is no longer in use by the program. Python uses a technique called reference counting to keep track of objects and their references. Each object has a reference count associated with it, which is incremented when a new reference to the object is created and decremented when a reference is deleted or goes out of scope.
When an object’s reference count reaches zero, it means that the object is no longer reachable and can be safely deallocated. Python’s garbage collector periodically runs to identify and collect objects with a reference count of zero.
Memory Management Techniques
Python employs several memory management techniques to optimize memory usage and improve performance.
Related Article: How to Use Python with Multiple Languages (Locale Guide)
1. Memory Pool
Python uses a memory pool to manage the allocation of small memory blocks. The memory pool consists of fixed-size blocks of memory, each of which can hold one or more Python objects. When a new object is created, Python checks if there is an available block in the memory pool that can accommodate the object’s size. If not, it requests a new block from the operating system.
The memory pool helps reduce the overhead of allocating and deallocating small memory blocks by reusing the available memory within the pool.
2. Memory Fragmentation
Memory fragmentation occurs when memory is divided into small, non-contiguous blocks, making it challenging to allocate large contiguous blocks of memory. Python uses different strategies to mitigate memory fragmentation, such as compacting memory by moving objects closer together and combining adjacent free blocks to create larger blocks.
3. Object Reuse
Python encourages object reuse to minimize memory allocation overhead. Once an object is no longer needed, Python does not immediately deallocate it. Instead, it adds the object to a list of free objects, which can be reused for future allocations. This reduces the need for frequent allocation and deallocation of objects, improving performance.
Related Article: How to Suppress Python Warnings
Memory Profiling
To analyze and optimize memory usage in Python, you can use memory profiling tools. These tools help identify memory leaks, excessive memory consumption, and inefficient memory usage patterns.
One popular memory profiling tool for Python is memory-profiler. This tool allows you to measure the memory usage of specific functions or lines of code, helping you pinpoint areas of your code that consume excessive memory.
from memory_profiler import profile @profile def my_function(): # Code to profile my_function()
By using memory profiling tools, you can gain insights into your code’s memory usage and make necessary optimizations to improve performance.
Memory Deallocation and Garbage Collection
Python’s memory management not only involves allocating memory for new objects but also deallocating memory for objects that are no longer needed. This process is known as memory deallocation. In Python, memory deallocation is handled by the garbage collector.
Garbage Collection in Action
Let’s take a look at an example to understand how garbage collection works in Python.
def create_objects(): x = [1, 2, 3] y = [4, 5, 6] x.append(y) y.append(x) return x, y a, b = create_objects()
In this example, we create two lists x
and y
. We then append y
to x
and vice versa, creating a cycle of references between the two objects.
After the create_objects()
function returns, there are no more references to x
and y
in the program. However, the reference counts of both objects are still not zero due to the circular references.
When the garbage collector runs, it detects this cycle of references and deallocates the memory occupied by x
and y
. By reclaiming this memory, the garbage collector ensures that the program’s memory usage remains efficient.
Related Article: How to Measure Elapsed Time in Python
Controlling Garbage Collection
Python provides some control over the garbage collection process using the gc
module. You can manually control when the garbage collector runs or disable it altogether.
To disable the garbage collector:
import gc gc.disable()
To enable the garbage collector:
import gc gc.enable()
You can also manually trigger a garbage collection:
import gc gc.collect()
However, it’s generally recommended to let the garbage collector handle memory deallocation automatically. Manually triggering the garbage collector is rarely necessary and can even degrade performance in some cases.
Understanding how memory deallocation and garbage collection work in Python is essential for writing efficient and memory-safe programs. By managing memory effectively, you can avoid memory leaks and ensure optimal performance.
Memory Leaks and How to Detect Them
Memory leaks are a common issue in programming, and Python is no exception. A memory leak occurs when a program allocates memory but fails to release it, causing the program to gradually consume more and more memory until it crashes or becomes unresponsive. In this chapter, we will explore what memory leaks are, why they occur, and how to detect them in Python.
What is a Memory Leak?
A memory leak occurs when memory that is no longer needed is not properly deallocated, resulting in memory that is reserved but not used. Over time, these leaked memory blocks accumulate, leading to excessive memory usage. In Python, memory leaks commonly occur when objects are not garbage collected as expected.
Related Article: How to Execute a Curl Command Using Python
Causes of Memory Leaks
Memory leaks can be caused by various factors, including:
1. Circular references: When two or more objects reference each other, but there are no external references to these objects, they become unreachable and cannot be garbage collected.
2. Unclosed resources: If a program fails to properly close resources such as files, database connections, or network sockets, it can lead to memory leaks.
3. Large data structures: Creating and maintaining large data structures can consume a significant amount of memory. If these structures are not properly managed or deallocated, memory leaks can occur.
Detecting Memory Leaks
Detecting memory leaks can be challenging, but Python provides several tools and techniques to help identify and diagnose them. Here are a few approaches:
1. Monitoring memory usage: Python’s sys
module provides a getsizeof()
function that can be used to monitor the size of objects in memory. By periodically checking the memory usage of your program, you can identify abnormal increases in memory consumption.
import sys # Monitor memory usage object_size = sys.getsizeof(my_object)
2. Using a debugger: Debuggers such as pdb
or gdb
can help identify memory leaks by allowing you to track the allocation and deallocation of objects. By setting breakpoints and inspecting memory usage during program execution, you can pinpoint potential memory leaks.
3. Profiling tools: Python offers profiling tools like cProfile
and memory_profiler
that can be used to analyze the memory usage of your program. These tools provide detailed reports on memory consumption, helping you identify areas where memory leaks may be occurring.
Preventing Memory Leaks
To prevent memory leaks in your Python programs, consider the following best practices:
1. Properly manage resources: Always close files, database connections, and network sockets when you are done using them. Use context managers (with
statement) to ensure resources are properly closed, even in the event of exceptions.
2. Avoid circular references: Be mindful of creating circular references between objects. If you find yourself in a situation where circular references are unavoidable, consider using weak references or breaking the circular reference manually.
3. Use garbage collection strategies: Python’s garbage collector automatically reclaims memory from objects that are no longer reachable. However, you can also manually trigger garbage collection using the gc
module’s collect()
function.
import gc # Trigger garbage collection gc.collect()
Related Article: How to Parse a YAML File in Python
Common Memory Management Issues and Solutions
Python’s automatic memory management system does a great job of handling memory allocation and deallocation for most situations. However, there are some common memory management issues that can arise, and it’s important to be aware of them and know how to solve them when they do occur.
Memory Fragmentation
Another common memory management issue is memory fragmentation. Memory fragmentation occurs when memory becomes divided into small, non-contiguous blocks, making it difficult to allocate larger blocks of memory.
This can happen when objects are allocated and deallocated in a non-linear fashion, resulting in fragmented memory. As a result, it may be challenging to allocate a large block of memory even if there is enough free memory available.
One solution to memory fragmentation is to use memory pools. A memory pool is a pre-allocated block of memory that can be divided into smaller fixed-size blocks. By using memory pools, you can allocate and deallocate objects from the pool, ensuring that memory remains contiguous and reducing the likelihood of fragmentation.
Python provides the ctypes
module, which allows you to work with memory pools. Here’s an example of how to use a memory pool:
import ctypes block_size = 1024 num_blocks = 10 # Create a memory pool pool = ctypes.create_string_buffer(block_size * num_blocks) # Allocate a block of memory from the pool block = ctypes.cast(pool, ctypes.POINTER(ctypes.c_char)) # Use the allocated memory block[0] = b'A' block[1] = b'B' # Deallocate the memory ctypes.memset(block, 0, block_size)
In this example, a memory pool is created using the create_string_buffer
function from the ctypes
module. The cast
function is then used to allocate a block of memory from the pool. The allocated memory can then be used as needed, and it can be deallocated using the memset
function.
Excessive Memory Usage
Sometimes, your Python program may use more memory than expected. This can happen due to inefficient memory usage or the creation of unnecessary objects.
One common cause of excessive memory usage is creating unnecessary copies of objects. For example, if you have a large list and you create a new list by slicing it, a new copy of the list will be created in memory. This can quickly consume a significant amount of memory if done repeatedly.
To avoid excessive memory usage, it’s important to be mindful of object creation and copying. Whenever possible, try to work with objects in place instead of creating new copies. This can often be achieved by using built-in functions or methods that operate on the original object directly.
For example, instead of creating a new list by slicing, you can use the extend
method to add elements to an existing list:
my_list = [1, 2, 3] my_list.extend([4, 5, 6])
In this example, the extend
method is used to add elements to the my_list
object directly, avoiding the creation of a new list.
By being mindful of object creation and copying, you can help reduce memory usage and improve the performance of your Python programs.
Overall, understanding common memory management issues and their solutions is crucial to writing efficient and reliable Python code. By being aware of memory leaks, memory fragmentation, and excessive memory usage, you can avoid potential pitfalls and ensure that your programs make the most efficient use of memory.
Related Article: How to Automatically Create a Requirements.txt in Python
Memory Management in Data Structures
In previous chapters, we have explored how Python manages memory and the different strategies it uses for memory allocation and deallocation. In this chapter, we will dive deeper into memory management specifically related to data structures in Python.
Python provides a wide range of built-in data structures such as lists, tuples, dictionaries, and sets. Each data structure has its own memory management characteristics, which we’ll discuss in this chapter.
Lists:
Lists in Python are dynamic arrays that can grow or shrink as needed. When a list needs to grow beyond its current capacity, Python will allocate a new block of memory, typically larger than the previous one, and copy the existing elements to the new memory location. This process is known as resizing.
Let’s consider an example:
numbers = [1, 2, 3] # Memory allocated for [1, 2, 3] numbers.append(4) # Memory reallocated for [1, 2, 3, 4]
In the above example, when we call the append()
method, Python resizes the memory allocated for the numbers
list to accommodate the new element.
Tuples:
Unlike lists, tuples are immutable, meaning they cannot be modified once created. As a result, memory management for tuples is simpler compared to lists. When a tuple is created, Python allocates a fixed block of memory for it, depending on the size and number of elements.
point = (3, 4) # Memory allocated for (3, 4)
In the above example, the memory is allocated for the tuple (3, 4)
and remains unchanged throughout the program’s execution.
Dictionaries:
Dictionaries in Python are implemented as hash tables, which require dynamic memory allocation. When a dictionary is created, Python allocates memory for the initial number of elements. If the dictionary grows beyond its capacity, Python will resize the memory and rehash the existing elements to the new memory location.
student = {'name': 'John', 'age': 20} # Memory allocated for {'name': 'John', 'age': 20} student['grade'] = 'A' # Memory reallocated for {'name': 'John', 'age': 20, 'grade': 'A'}
In the above example, when we add a new key-value pair to the student
dictionary, Python resizes the memory allocated for the dictionary to accommodate the new element.
Sets:
Sets in Python are similar to dictionaries in terms of memory management. When a set is created, Python allocates memory for the initial number of elements. If the set grows beyond its capacity, Python will resize the memory and rehash the existing elements to the new memory location.
fruits = {'apple', 'banana'} # Memory allocated for {'apple', 'banana'} fruits.add('orange') # Memory reallocated for {'apple', 'banana', 'orange'}
In the above example, when we add a new element to the fruits
set, Python resizes the memory allocated for the set to accommodate the new element.
Understanding how memory management works in different data structures is essential for writing efficient and memory-friendly Python code. By being aware of the memory behavior of various data structures, you can make informed decisions and optimize your code accordingly.
In the next chapter, we will explore memory management considerations when working with objects in Python.
References:
– Python Documentation: https://docs.python.org/3/c-api/memory.html
Memory Management in Functions
In Python, functions play a crucial role in code organization and reusability. When a function is called, memory is allocated to store its variables and execution code. Understanding how memory is managed within functions can help optimize performance and avoid memory-related issues.
Local Variables and the Stack
When a function is called, a new frame is created on top of the call stack to store its local variables. The call stack is a data structure that keeps track of function calls and their respective frames. Each frame contains the local variables and the return address, which is used to resume execution after the function finishes.
Local variables are created and stored within the function’s frame. These variables are only accessible within the function’s scope and are automatically deallocated when the function returns. This automatic deallocation is done by the Python interpreter, which uses a strategy called garbage collection.
Memory Management with Immutable Objects
Immutable objects, such as numbers and strings, are stored in a different area of memory called the heap. The heap is a region where objects that are not deallocated immediately are stored. Python uses a reference counting mechanism to keep track of the number of references to an object.
When an immutable object is passed as an argument to a function, a new reference is created within the function’s frame. This reference points to the same memory location as the original object. However, any modifications made to the object within the function do not affect the original object outside the function.
For example, consider the following code snippet:
def modify_string(s): s += " World" my_string = "Hello" modify_string(my_string) print(my_string) # Output: Hello
In this example, the modify_string
function takes a string as an argument and appends ” World” to it. However, the original string my_string
remains unchanged because the +=
operator creates a new string object within the function’s frame.
Memory Management with Mutable Objects
Mutable objects, such as lists and dictionaries, behave differently when passed as arguments to a function. Unlike immutable objects, changes made to mutable objects within a function can affect the original object outside the function.
When a mutable object is passed as an argument, the function receives a reference to the object. Any modifications made to the object within the function directly affect the original object. This is because the reference points to the same memory location.
Consider the following code snippet:
def append_to_list(lst): lst.append(4) my_list = [1, 2, 3] append_to_list(my_list) print(my_list) # Output: [1, 2, 3, 4]
In this example, the append_to_list
function takes a list as an argument and appends the number 4 to it. The original list my_list
is modified because the function operates directly on the referenced object.
Memory Management and Variable Scope
Variable scope refers to the visibility and lifetime of a variable within a program. In Python, variables defined within a function are considered local variables and are only accessible within that function’s scope.
When a function is called, memory is allocated for its local variables. This memory is deallocated when the function returns. Local variables cannot be accessed outside the function’s scope, and attempting to do so will result in a NameError
.
Consider the following code snippet:
def calculate_sum(a, b): result = a + b return result print(result) # Output: NameError: name 'result' is not defined
In this example, the variable result
is defined within the calculate_sum
function. Trying to access result
outside the function’s scope raises a NameError
because the variable is not defined at that point.
Understanding how memory is managed within functions is essential for writing efficient and bug-free code. By knowing how variables are allocated and deallocated, you can optimize memory usage and prevent memory leaks.
Memory Optimization Techniques
Python provides several techniques to optimize memory usage in your programs. These techniques can help reduce the memory footprint of your application and improve its performance. In this chapter, we will explore some of the commonly used memory optimization techniques in Python.
Related Article: How to Pretty Print a JSON File in Python (Human Readable)
1. Use Generators
Generators are a powerful feature in Python that can help save memory by producing values on the fly, instead of storing them all in memory at once. By using the yield
statement, you can create a generator function that generates values one at a time, as they are needed. This can be particularly useful when dealing with large datasets or infinite sequences.
Here’s an example of a generator function that generates an infinite sequence of Fibonacci numbers:
def fibonacci(): a, b = 0, 1 while True: yield a a, b = b, a + b fib_gen = fibonacci() for i in range(10): print(next(fib_gen))
This code will print the first 10 Fibonacci numbers without storing them all in memory at once.
2. Use Data Structures Wisely
Choosing the right data structure can greatly impact memory usage. For example, if you need to store a large number of key-value pairs, using a dictionary (dict
) can be more memory-efficient compared to a list of tuples.
Similarly, if you have a sequence of integers that are within a small range, using an array (array.array
) instead of a list can save memory by storing the values more compactly.
Consider the following code that stores a large list of integers:
numbers = [1, 2, 3, ...] # large list of integers
If the range of numbers is known to be small, you can use an array to reduce memory usage:
import array numbers = array.array('i', [1, 2, 3, ...]) # large array of integers
3. Use Memory Views
Memory views (memoryview
) provide a way to access the internal memory of an object without making a copy. This can be useful when working with large data structures, such as NumPy arrays or binary files.
By using memory views, you can avoid unnecessary memory duplication and improve the performance of your code. Here’s an example that demonstrates how to use a memory view to access a NumPy array:
import numpy as np data = np.array([1, 2, 3, 4, 5]) view = memoryview(data) # Access elements using the memory view for i in range(len(data)): print(view[i])
Related Article: How to Use Regex to Match Any Character in Python
4. Use Weak References
In some cases, you may need to hold references to objects without preventing them from being garbage collected. This can be achieved using weak references (weakref
).
Weak references allow you to maintain a reference to an object that does not count towards its reference count. This can be useful when dealing with cyclic references or caches where objects may be removed from memory when not in use.
Here’s an example that demonstrates the use of weak references:
import weakref class MyClass: def __init__(self, name): self.name = name obj = MyClass("example") ref = weakref.ref(obj) print(ref().name) # Access the referenced object
In this example, the object obj
is accessible through the weak reference ref()
, but it can still be garbage collected if there are no strong references to it.
These are just a few of the memory optimization techniques available in Python. By applying these techniques judiciously, you can optimize memory usage and improve the performance of your Python programs.
Real World Examples of Python Memory Management
Python’s memory management plays a crucial role in ensuring efficient memory usage and preventing memory leaks. In this chapter, we will explore some real-world examples that illustrate how Python handles memory management.
Example 1: Memory Deallocation with Garbage Collection
Python utilizes a garbage collector to automatically deallocate memory that is no longer in use. Let’s consider the following code snippet:
class MyClass: def __init__(self, name): self.name = name obj1 = MyClass("Object 1") obj2 = MyClass("Object 2") obj3 = obj1
In this example, we create three instances of the MyClass
class. obj1
and obj2
are independent objects, while obj3
is a reference to obj1
. If we delete obj1
using del obj1
, the memory occupied by obj1
will not be immediately deallocated. This is because obj3
still holds a reference to the same memory location. However, if we delete both obj1
and obj3
using del obj1, obj3
, the memory will be deallocated.
Python’s garbage collector periodically checks for objects that are no longer referenced and frees the associated memory. This process ensures that memory is efficiently managed, avoiding memory leaks and unnecessary memory consumption.
Example 2: Memory Management with Large Data Structures
Python’s memory management becomes especially important when dealing with large data structures. Consider the following code snippet:
data = [1] * 10000000
In this example, we create a list data
with 10 million elements, each initialized to the value 1. This list consumes a significant amount of memory. If we no longer need this list, we can free up the memory by simply assigning data
to a new empty list:
data = []
This operation will remove the reference to the large list, allowing the garbage collector to deallocate the memory. It’s crucial to be mindful of memory usage when working with large data structures to prevent memory exhaustion.
Example 3: Managing Memory with Context Managers
Python provides context managers as a convenient way to manage resources, including memory. The with
statement is commonly used to create a context manager. Let’s consider an example with file handling:
with open("data.txt", "r") as file: contents = file.read() # Perform operations on the contents # The file is automatically closed outside the context manager
In this example, the open()
function returns a file object, which is automatically closed when the with
block is exited. This ensures that system resources, including memory, are properly managed and released.
By utilizing context managers, you can avoid resource leaks and ensure efficient memory management while working with various types of resources.
These real-world examples demonstrate how Python’s memory management mechanisms, such as garbage collection and context managers, contribute to efficient memory usage and prevent memory leaks. Understanding these concepts is essential for writing robust and memory-efficient Python code.
Advanced Memory Management Techniques
In previous chapters, we covered the basics of Python memory management and explored some common techniques to optimize memory usage. In this chapter, we will delve into more advanced memory management techniques to further enhance the performance of your Python programs.
Related Article: How to Download a File Over HTTP in Python
1. Reference Counting and Garbage Collection
Python uses a combination of reference counting and garbage collection to manage memory. Reference counting keeps track of the number of references to an object, while the garbage collector periodically checks for unreferenced objects and frees up their memory.
While reference counting is efficient for most cases, it can’t handle cyclic references, where objects reference each other in a cycle. To deal with cyclic references, Python uses a garbage collector that employs the concept of generations. The garbage collector divides objects into different generations based on their age and collects them at different intervals.
By understanding how reference counting and garbage collection work together, you can write more memory-efficient code and avoid memory leaks.
2. Weak References
In some cases, you may want to maintain a reference to an object without preventing it from being garbage collected. This is where weak references come in handy. Weak references allow you to refer to an object without increasing its reference count, thus allowing it to be garbage collected.
Python provides the weakref
module, which allows you to create weak references to objects. Here’s an example of using weak references:
import weakref class MyClass: pass obj = MyClass() weak_ref = weakref.ref(obj) # Access the object through the weak reference print(weak_ref()) # The object is still alive, so it can be accessed del obj # The object has been garbage collected, so the weak reference returns None print(weak_ref())
3. Object Pooling
Object pooling is a technique that involves reusing objects instead of creating new ones. This can be useful in situations where creating new objects is expensive or memory-intensive, such as when dealing with large data sets or network connections.
Python provides the multiprocessing.Pool
class, which allows you to create a pool of worker processes. These processes can be used to perform parallel computations or handle multiple requests simultaneously, without the overhead of creating new processes each time.
Here’s an example of using multiprocessing.Pool
to parallelize a computation:
from multiprocessing import Pool def square(x): return x ** 2 if __name__ == '__main__': with Pool() as pool: result = pool.map(square, [1, 2, 3, 4, 5]) print(result)
In this example, the Pool
class manages a pool of worker processes, allowing us to distribute the computation of squaring numbers across multiple processes.
Related Article: How to Unzip Files in Python
5. External Libraries for Memory Management
Python has a rich ecosystem of external libraries that can help with advanced memory management. Some popular libraries include pympler
, which provides tools for tracking memory usage and object sizes, and objgraph
, which allows you to visualize object relationships and identify memory leaks.
These libraries can be valuable resources for profiling your code and optimizing memory usage in complex applications.
In this chapter, we explored advanced memory management techniques such as reference counting and garbage collection, weak references, object pooling, memory profiling, and external libraries. By applying these techniques, you can further optimize the memory usage of your Python programs and improve their performance.
Memory Management Best Practices
Understanding how Python manages memory is essential for writing efficient and optimized code. In this chapter, we will explore some best practices for memory management in Python.
1. Use Generators
Generators are a great way to save memory when dealing with large datasets or infinite sequences. Unlike lists or arrays, generators do not store the entire sequence in memory. Instead, they generate values on-the-fly, allowing you to iterate over them without storing them all at once.
Consider the following example where we generate a sequence of Fibonacci numbers using a generator function:
def fibonacci(): a, b = 0, 1 while True: yield a a, b = b, a + b # Usage fib_gen = fibonacci() for i in range(10): print(next(fib_gen))
Using a generator in this case allows us to generate Fibonacci numbers indefinitely without consuming excessive memory.
Related Article: How to Use the And/Or Operator in Python Regular Expressions
2. Avoid Unnecessary Copies
Python creates a new object when a variable is assigned a new value. However, sometimes we may unintentionally create unnecessary copies of objects, leading to increased memory usage.
For example, consider the following code:
def process_data(data): # Unnecessary copy data_copy = data[:] # Process data_copy
In this example, data_copy = data[:]
creates a copy of the entire data
list. If the data
list is large, this can lead to unnecessary memory consumption. Instead, we can directly process the data
list without creating a copy:
def process_data(data): # Process data
By avoiding unnecessary copies, we can significantly reduce memory usage.
3. Use Context Managers
Python provides context managers, which allow us to manage resources efficiently, including memory. Context managers ensure that resources are properly released, even if exceptions occur.
Consider the following example where we open a file and read its contents:
with open('data.txt', 'r') as file: data = file.read() # Process data
In this example, the open()
function returns a file object, and the with
statement automatically closes the file after we are done. This ensures that the file is properly closed, preventing any potential memory leaks.
4. Dispose of Unreferenced Objects
Python’s garbage collector automatically reclaims memory from objects that are no longer referenced. However, sometimes we may need to explicitly dispose of objects to free up memory.
The del
statement can be used to remove references to objects and trigger the garbage collector. For example:
data = [1, 2, 3, 4, 5] # Process data del data
In this example, del data
removes the reference to the data
list, allowing the garbage collector to reclaim the memory.
Related Article: How to Get Logical Xor of Two Variables in Python
5. Use Data Structures Wisely
Choosing the right data structure can have a significant impact on memory usage. For example, using a dictionary instead of a list for large collections of key-value pairs can reduce memory consumption.
Additionally, consider using data structures provided by libraries like NumPy or Pandas when dealing with large numeric datasets. These libraries are optimized for memory efficiency and provide various data structures specifically designed for data analysis.
By selecting appropriate data structures, we can minimize memory usage and improve the performance of our code.
6. Profile and Optimize
Lastly, profiling your code and optimizing memory usage can help identify and resolve potential memory bottlenecks. Python provides several profiling tools, such as cProfile
and memory_profiler
, which can help identify memory-intensive operations.
Once identified, you can optimize memory usage by applying the previously mentioned best practices, such as using generators, avoiding unnecessary copies, and disposing of unreferenced objects.
By continuously profiling and optimizing your code, you can ensure efficient memory management and improve the overall performance of your Python applications.
In this chapter, we explored some best practices for memory management in Python. By following these practices, you can write more memory-efficient code and optimize the performance of your applications.