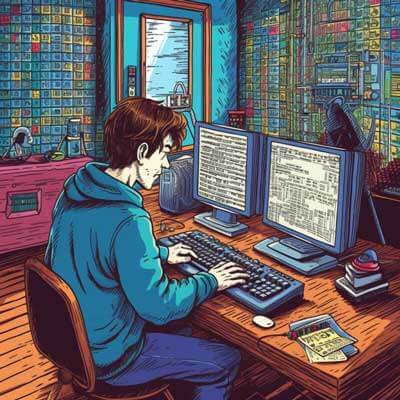
Table of Contents
Basic syntax and usage of switch case statements
In many programming languages, such as C or Java, the switch case statement is a powerful tool for executing different blocks of code based on the value of a variable. However, Python does not have a built-in switch case statement like these languages. Despite this, there are several ways to achieve similar functionality in Python.
One common approach is to use if-elif-else statements to create a switch-like structure. Here is an example:
def switch_case(argument): if argument == 1: return "One" elif argument == 2: return "Two" elif argument == 3: return "Three" else: return "Invalid input"
In this example, the function switch_case
takes an argument
and uses if-elif-else statements to check its value. Depending on the value, a different string is returned. If the argument
does not match any of the specific cases, the last else statement is executed.
Another way to achieve switch-like behavior in Python is by using a dictionary. Here is an example:
def switch_case(argument): switch = { 1: "One", 2: "Two", 3: "Three" } return switch.get(argument, "Invalid input")
In this example, a dictionary called switch
is defined with keys representing the different cases and values representing the corresponding outputs. The switch.get(argument, "Invalid input")
statement retrieves the value associated with the argument
key. If the argument
key is not found in the dictionary, it returns the default value "Invalid input".
It is important to note that the dictionary approach can be more efficient than the if-elif-else approach when dealing with a large number of cases, as dictionary lookups are generally faster than multiple if-elif-else checks.
Although not a native feature of Python, these methods provide a way to achieve switch case-like functionality in Python. It is worth noting that the if-elif-else approach is more readable and easier to understand for most developers, while the dictionary approach offers better performance for larger switch structures.
Related Article: How To Exit/Deactivate a Python Virtualenv
Using if-elif-else statements
One way to emulate a switch case statement in Python is by using a series of if-elif-else statements. Here's an example:
def switch_case_example(value): if value == 1: print("This is case 1") elif value == 2: print("This is case 2") elif value == 3: print("This is case 3") else: print("This is the default case") # Testing the switch_case_example function switch_case_example(2) # Output: This is case 2 switch_case_example(4) # Output: This is the default case
In this example, the switch_case_example
function takes a value as input and uses if-elif-else statements to check against different cases. If the value matches any of the cases, the corresponding code block is executed. If none of the cases match, the default case is executed.
Using dictionaries
Another way to implement a switch case statement in Python is by using dictionaries. Here's an example:
def switch_case_example(value): cases = { 1: "This is case 1", 2: "This is case 2", 3: "This is case 3", } default = "This is the default case" result = cases.get(value, default) print(result) # Testing the switch_case_example function switch_case_example(2) # Output: This is case 2 switch_case_example(4) # Output: This is the default case
In this example, the switch_case_example
function uses a dictionary to map the different cases to their corresponding code blocks. The get
method of the dictionary is used to retrieve the value associated with the input value
. If the input value is not found in the dictionary, the default case is returned.
Using dictionaries can be more concise and easier to read, especially when dealing with a large number of cases.
Using switch case statements for simple decision-making
One popular approach is to use dictionaries to create a mapping between conditions and their corresponding actions. Let's take a look at an example:
def handle_case_1(): print("Handling case 1") def handle_case_2(): print("Handling case 2") def handle_case_3(): print("Handling case 3") def handle_default(): print("Handling default case") # Create a dictionary mapping conditions to functions switch_case = { 1: handle_case_1, 2: handle_case_2, 3: handle_case_3, } # Get the condition input from the user condition = int(input("Enter a condition: ")) # Use the dictionary to determine the corresponding action action = switch_case.get(condition, handle_default) # Execute the action action()
In this example, we define several functions that handle different cases. We then create a dictionary called switch_case
that maps the conditions to their corresponding functions. We use the get()
method of the dictionary to retrieve the appropriate function based on the condition input by the user. If the condition does not match any keys in the dictionary, we provide a default function to handle that case.
Another approach to achieve switch case-like functionality is to use if-elif-else statements. While this approach is more verbose, it can be useful for more complex decision-making scenarios. Here's an example:
def handle_case_1(): print("Handling case 1") def handle_case_2(): print("Handling case 2") def handle_case_3(): print("Handling case 3") def handle_default(): print("Handling default case") # Get the condition input from the user condition = int(input("Enter a condition: ")) # Use if-elif-else statements to determine the corresponding action if condition == 1: handle_case_1() elif condition == 2: handle_case_2() elif condition == 3: handle_case_3() else: handle_default()
In this example, we define the same set of functions to handle different cases. We take the condition input from the user and use if-elif-else statements to determine the appropriate action based on the condition.
Both the dictionary and if-elif-else approaches provide flexibility and readability in handling multiple conditions in Python. Choose the approach that best suits your needs and the complexity of your decision-making logic.
We will explore more advanced techniques for handling complex decision-making scenarios using external libraries and design patterns.
Related Article: How To Check If a File Exists In Python
Understanding the limitations of switch case statements in Python
Python does not provide a direct syntax for switch case statements. In languages like C or Java, you can use the switch keyword followed by cases and break statements. Here's an example of a switch case statement in C:
switch (expression) { case value1: // code to be executed if expression matches value1 break; case value2: // code to be executed if expression matches value2 break; default: // code to be executed if expression doesn't match any value }
In Python, you would typically use if-elif-else statements to achieve similar functionality:
if expression == value1: # code to be executed if expression matches value1 elif expression == value2: # code to be executed if expression matches value2 else: # code to be executed if expression doesn't match any value
Another limitation of switch case statements in Python is that they can only be used with equality comparisons. In other words, you can only check if a value is equal to another value. This means you cannot use complex conditions or perform range checks like you can in other languages.
For example, if you want to check if a number is between 1 and 10, you cannot do it directly with a switch case statement. Instead, you would need to use a combination of if statements:
if number >= 1 and number <= 10: # code to be executed if number is between 1 and 10 else: # code to be executed if number is outside the range
It's important to note that although switch case statements are not available in Python, there are alternative approaches that can be used to achieve similar functionality. One common approach is to use a dictionary to map values to functions. Here's an example:
def case1(): # code to be executed if expression matches value1 def case2(): # code to be executed if expression matches value2 def default_case(): # code to be executed if expression doesn't match any value switch = { value1: case1, value2: case2 } switch.get(expression, default_case)()
In this approach, we define different functions for each case and use a dictionary to map the values to the corresponding functions. We then use the get()
method to retrieve the appropriate function based on the expression value and call it.
Using a third-party library
If you prefer a more structured and formal switch case syntax, you can consider using a third-party library like switchcase
. This library provides a switch case construct similar to those found in other programming languages. Here's an example:
from switchcase import switch def switch_case_example(argument): with switch(argument) as s: s.case(1, lambda: print("You selected option 1")) s.case(2, lambda: print("You selected option 2")) s.case(3, lambda: print("You selected option 3")) s.default(lambda: print("Invalid option")) switch_case_example(1) # Output: You selected option 1
In this example, we import the switch
class from the switchcase
library and use it as a context manager. Inside the context, we define the cases using the case()
method and their corresponding actions as lambda functions. The default()
method is used to define the action for cases that do not match any of the specified cases.
Using a third-party library can make the code more readable and maintainable, especially when dealing with complex switch case scenarios.
Using classes and objects for advanced switch case functionality
You can achieve similar functionality using classes and objects. This approach allows you to create more advanced and customizable switch case statements.
To implement switch case functionality using classes and objects, you can define a class that represents the switch statement. Inside the class, you can define methods for each case and a default method for handling cases that are not explicitly defined.
Here's an example of how you can create a switch case using classes and objects:
class SwitchCase: def case1(self): print("This is case 1") def case2(self): print("This is case 2") def case3(self): print("This is case 3") def default(self): print("This is the default case") # Creating an instance of the SwitchCase class switch = SwitchCase() # Using the switch case value = 2 if value == 1: switch.case1() elif value == 2: switch.case2() elif value == 3: switch.case3() else: switch.default()
In this example, we define a class called SwitchCase
with methods case1()
, case2()
, and case3()
representing different cases. We also define a default()
method that will be called if none of the cases match the given value.
To use the switch case functionality, we create an instance of the SwitchCase
class and then use if-elif-else statements to check the value and call the corresponding method.
By using classes and objects, you can easily extend and modify the switch case functionality. You can add additional cases by defining new methods in the class, and you can customize the behavior of each case by adding parameters to the methods or storing data in instance variables.
Using classes and objects for switch case functionality provides a more flexible and object-oriented approach compared to traditional switch case statements. It allows you to encapsulate the logic for each case within methods, making the code more modular and easier to maintain.
You can learn more about classes and objects in Python in the official Python documentation: https://docs.python.org/3/tutorial/classes.html.
Real world examples of switch case usage in Python
Switch statements are not natively available in Python. However, there are several ways to achieve similar functionality using different coding techniques. We will explore some real-world examples of how switch case statements can be implemented in Python.
Related Article: How to Convert a String to Boolean in Python
Example 1: Grade Calculator
Suppose we want to create a program that calculates the grade based on a student's score. Here's how we can achieve this using a dictionary:
def calculate_grade(score): grade_dict = { 90: "A", 80: "B", 70: "C", 60: "D", 50: "F" } for key in grade_dict.keys(): if score >= key: return grade_dict[key] else: return "Invalid score" score = 85 grade = calculate_grade(score) print(f"The grade for a score of {score} is {grade}")
In this example, we use a dictionary to map the score range to the corresponding grade. We iterate over the keys of the dictionary in descending order and return the grade when we find a key that is less than or equal to the given score. If the score is invalid (i.e., below 50), we return "Invalid score".
Example 2: Menu Selection
Let's say we want to create a program that performs different actions based on the user's menu selection. Here's one way to implement this using functions:
def action1(): print("Performing Action 1") def action2(): print("Performing Action 2") def action3(): print("Performing Action 3") def default_action(): print("Invalid selection") def switch_case(selection): actions = { 1: action1, 2: action2, 3: action3 } actions.get(selection, default_action)() user_selection = 2 switch_case(user_selection)
In this example, we define separate functions for each action. We use a dictionary to map the user's selection to the corresponding function. If the user selects an option that is not in the dictionary, the default_action
function is called.
Example 3: Handling Multiple Conditions
Sometimes, we might need to handle multiple conditions in our code. We can achieve this using a combination of if-elif-else statements. Here's an example:
def perform_action(condition): if condition == "A": print("Performing action for condition A") elif condition == "B": print("Performing action for condition B") elif condition == "C": print("Performing action for condition C") else: print("Invalid condition") condition = "B" perform_action(condition)
In this example, we use if-elif-else statements to check the condition and perform the corresponding action. If none of the conditions match, the else
block is executed.
Best practices for using switch case statements in Python
Related Article: How to Handle Cookies and Cookie Outputs in Python
Using if-elif-else statements
The most common approach to mimic switch case behavior in Python is by using if-elif-else statements. Each case is represented by an elif statement, and the else statement acts as the default case. Here is an example:
def switch_case_example(argument): if argument == 1: # Case 1 print("Case 1") elif argument == 2: # Case 2 print("Case 2") elif argument == 3: # Case 3 print("Case 3") else: # Default case print("Default case") switch_case_example(2) # Output: Case 2
This approach is straightforward and readable, but it can become cumbersome if there are many cases to handle.
Using dictionaries
Another approach is to use dictionaries to map case values to corresponding functions or actions. This can be especially useful when the cases involve complex actions or when there are a large number of cases. Here is an example:
def case_one(): print("Case 1") def case_two(): print("Case 2") def case_three(): print("Case 3") def default_case(): print("Default case") switch_dict = { 1: case_one, 2: case_two, 3: case_three, } argument = 2 switch_dict.get(argument, default_case)() # Output: Case 2
In this example, we define separate functions for each case, and then create a dictionary where the keys represent the case values and the values are the corresponding functions. We use the .get()
method to retrieve the function based on the argument, and if the key is not found, the default case function is executed.
Using third-party libraries
There are also third-party libraries available that provide switch case functionality in Python. One popular library is the switch-case
library, which allows you to write switch case statements similar to other programming languages. You can install it using pip:
pip install switch-case
Here is an example of how to use the switch-case
library:
from switch_case import switch argument = 2 with switch(argument) as case: if case(1): print("Case 1") if case(2): print("Case 2") if case(3): print("Case 3") if case.default: print("Default case")
This library provides a more concise syntax for writing switch case statements, making the code easier to read and maintain.
Using switch case statements with different data types
Python developers often use if-elif-else statements as a substitute for switch case statements. This approach allows you to check different conditions and execute corresponding blocks of code. However, if you have a large number of conditions, this can result in lengthy and repetitive code. In such cases, using a dictionary can provide a more concise and efficient solution.
Let's explore how to use switch case statements with different data types in Python.
Related Article: FastAPI Enterprise Basics: SSO, RBAC, and Auditing
Switch case with integers
To implement a switch case statement with integers, we can use a dictionary with integer keys and function values. Each function represents a case or condition. Here's an example:
def case_one(): print("You selected case one.") def case_two(): print("You selected case two.") def case_default(): print("Invalid selection.") switch = { 1: case_one, 2: case_two, } user_choice = 2 switch.get(user_choice, case_default)()
In this example, we define three functions: case_one()
, case_two()
, and case_default()
. The switch
dictionary maps integer keys (1
and 2
) to their respective functions. We use the get()
method to retrieve the function based on the user_choice
variable, and if the key is not found, we execute the case_default()
function.
When we run this code with user_choice
set to 2
, the output will be:
You selected case two.
This approach allows us to easily add or remove cases by modifying the dictionary, making our code more readable and maintainable.
Switch case with strings
To implement a switch case statement with strings, we can use a similar approach as before, but with string keys instead. Here's an example:
def case_one(): print("You selected case one.") def case_two(): print("You selected case two.") def case_default(): print("Invalid selection.") switch = { "one": case_one, "two": case_two, } user_choice = "two" switch.get(user_choice, case_default)()
In this example, we define the same functions as before, but now the switch
dictionary maps string keys ("one"
and "two"
) to their respective functions. We use the get()
method to retrieve the function based on the user_choice
variable, and if the key is not found, we execute the case_default()
function.
When we run this code with user_choice
set to "two"
, the output will be the same as before:
You selected case two.
Using dictionaries in this way allows us to handle different cases efficiently, without the need for lengthy if-elif-else statements.
Switch case with other data types
The switch case approach shown above can be extended to handle other data types, such as floats, booleans, or even custom objects. However, it's important to note that the keys in the dictionary must match the data types being used.
For example, if you want to handle float values, you would use float keys in the dictionary. Similarly, if you want to handle boolean values, you would use boolean keys in the dictionary.
Here's an example of a switch case statement with boolean values:
def case_true(): print("You selected True.") def case_false(): print("You selected False.") def case_default(): print("Invalid selection.") switch = { True: case_true, False: case_false, } user_choice = True switch.get(user_choice, case_default)()
In this example, we define three functions: case_true()
, case_false()
, and case_default()
. The switch
dictionary maps boolean keys (True
and False
) to their respective functions. We use the get()
method to retrieve the function based on the user_choice
variable, and if the key is not found, we execute the case_default()
function.
When we run this code with user_choice
set to True
, the output will be:
You selected True.
As you can see, by using dictionaries, we can implement switch case-like behavior in Python for different data types.
Common mistakes to avoid when using switch case statements
Switch case statements can be a powerful tool when it comes to writing clean and concise code in Python. However, there are some common mistakes that developers make when using switch case statements. We will discuss these mistakes and how to avoid them.
1. Not using a proper switch case construct
Many developers try to emulate a switch case using if-elif-else statements, which can make the code lengthy and less readable. Instead, a better approach is to use a dictionary with functions as values, and then call the function based on the input value.
def option1(): print("Option 1 selected.") def option2(): print("Option 2 selected.") def option3(): print("Option 3 selected.") # Create a dictionary with function names as values switch_case = { 1: option1, 2: option2, 3: option3, } # Call the function based on the input value input_value = 2 switch_case.get(input_value, lambda: print("Invalid option."))()
2. Forgetting to handle default cases
When using switch case statements, it is important to handle all possible cases, including the default case. Forgetting to handle the default case can lead to unexpected behavior or errors in your code. To handle the default case, you can use the get()
method of the dictionary and provide a default function to be called.
def option1(): print("Option 1 selected.") def option2(): print("Option 2 selected.") def option3(): print("Option 3 selected.") def default_option(): print("Invalid option.") # Create a dictionary with function names as values switch_case = { 1: option1, 2: option2, 3: option3, } # Call the function based on the input value input_value = 4 switch_case.get(input_value, default_option)()
3. Using switch case for non-integer values
Switch case statements are typically used with integers or enumerated types. Attempting to use switch case with non-integer values can lead to errors or unexpected behavior. If you need to handle non-integer values, it is recommended to use if-elif-else statements instead.
def option1(): print("Option 1 selected.") def option2(): print("Option 2 selected.") def option3(): print("Option 3 selected.") def default_option(): print("Invalid option.") # Using if-elif-else statements for non-integer values input_value = "option2" if input_value == "option1": option1() elif input_value == "option2": option2() elif input_value == "option3": option3() else: default_option()
When using switch case statements in Python, it is important to avoid these common mistakes. By using a proper switch case construct, handling default cases, and using switch case only for integer values, you can write cleaner and more maintainable code.
4. Performance considerations:
When optimizing switch case statements in Python, it's important to consider the performance implications of each approach. In general, using if-elif-else statements or a dictionary mapping should have similar performance characteristics. However, using a third-party library like py-switch
may introduce some overhead due to the additional function calls and context management.
If performance is a critical concern, you may consider using a simple if-elif-else chain instead of a switch case construct. In some cases, this can be more efficient than using a switch-like approach.