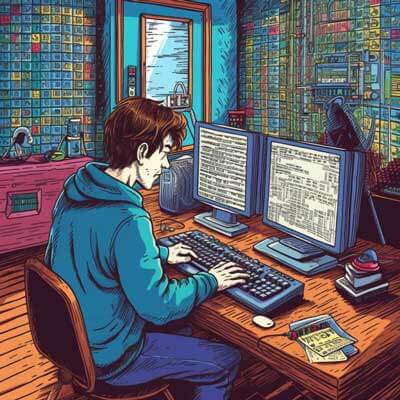
- Understanding Unicode and Character Encoding
- Internationalization Best Practices
- Handling Data Validation in a Multilingual API
- Designing a Multilingual API with FastAPI
- FastAPI’s Approach to Internationalization and Encoding
- Leveraging Pydantic Models for i18n in FastAPI
- Ensuring Proper Character Encoding in API Responses
- Challenges of Handling Multilingual Data in an API
- Encoding Requirements for Internationalization in FastAPI
- Tools and Libraries for Localization in a FastAPI Project
- Additional Resources
Understanding Unicode and Character Encoding
Unicode is a computing industry standard that provides a unique number for every character, regardless of the platform, program, or language. It allows the representation and manipulation of text in any writing system. Character encoding, on the other hand, is the process of mapping characters to numeric codes for storage or transmission.
In the context of internationalization, it is crucial to understand Unicode and character encoding to ensure proper handling of multilingual data. Unicode supports a vast range of characters, including those used in different languages, symbols, and emojis. However, when working with text in programming languages or databases, it needs to be encoded using a specific character encoding scheme, such as UTF-8 or UTF-16.
Let’s take a look at an example of encoding and decoding Unicode strings using Python:
# Encoding a Unicode string to UTF-8 unicode_string = "Hello, 世界" encoded_string = unicode_string.encode("utf-8") print(encoded_string) # b'Hello, \xe4\xb8\x96\xe7\x95\x8c' # Decoding a UTF-8 string to Unicode decoded_string = encoded_string.decode("utf-8") print(decoded_string) # Hello, 世界
In the example above, we encode a Unicode string “Hello, 世界” to UTF-8, which results in the encoded string “b’Hello, \xe4\xb8\x96\xe7\x95\x8c'”. We then decode the UTF-8 string back to Unicode to obtain the original string.
Understanding Unicode and character encoding is crucial when working with multilingual data in FastAPI, as it ensures proper handling and representation of text across different languages and character sets.
Related Article: How To Exit Python Virtualenv
Internationalization Best Practices
Internationalization (i18n) is the process of designing and developing software that can be adapted to different languages and regions without code changes. It involves separating the user interface (UI) from the application logic and storing translatable resources in external files.
When implementing internationalization in FastAPI, it is essential to follow best practices to ensure a smooth localization experience for users. Here are some best practices to consider:
1. Separate UI text from code: Avoid hardcoding UI text directly in your code. Instead, store translatable strings in external resource files, such as JSON or YAML files. This allows for easier translation and modification of text without modifying the code.
2. Use language tags: Language tags, such as “en” for English or “es” for Spanish, should be used to identify the language of the content. FastAPI supports language tags and provides built-in functions to handle language negotiation based on user preferences.
3. Provide fallbacks: If a translation for a specific language is not available, provide a fallback option to default to a more widely understood language, such as English. This ensures that users can still understand the UI, even if their preferred language is not supported.
4. Test with different languages: Make sure to thoroughly test your application with different languages to identify and fix any issues related to text rendering, layout, or character encoding. This helps ensure a consistent user experience across languages.
Handling Data Validation in a Multilingual API
Data validation is an important aspect of building a robust API, especially in a multilingual context where input data may vary in different languages. FastAPI, with its integration with Pydantic, provides a useful and flexible data validation mechanism.
Pydantic is a data validation and parsing library that allows you to define data models using Python classes. These models can then be used to validate incoming data and ensure its correctness before processing it further. Let’s take a look at an example:
from pydantic import BaseModel class User(BaseModel): username: str age: int @app.post("/users") def create_user(user: User): # Process the validated user data ...
In the example above, we define a Pydantic model called User
with two fields: username
and age
. When a POST request is made to the /users
endpoint with JSON data, FastAPI automatically validates the incoming data against the User
model. If the data doesn’t match the model’s schema, FastAPI returns a 422 Unprocessable Entity response with detailed error messages.
This data validation mechanism ensures that only valid data is processed in the API and helps prevent issues related to incorrect or malformed data. It also provides a consistent validation experience regardless of the language used in the input data.
Designing a Multilingual API with FastAPI
Designing a multilingual API involves considering various aspects, such as language negotiation, localization of error messages, and handling multilingual data. FastAPI provides features and tools that make it easier to design and develop multilingual APIs.
Language negotiation, or content negotiation, refers to the process of determining the language preference of the client and serving the appropriate language version of the API response. FastAPI supports language negotiation out of the box through the accept_language
parameter in path operations. Let’s see an example:
from fastapi import FastAPI from fastapi import Request, Depends app = FastAPI() @app.get("/greeting") async def greet(request: Request, accept_language: str = Depends(get_accept_language)): if accept_language.startswith("es"): return {"message": "¡Hola!"} else: return {"message": "Hello!"}
In the example above, we define a GET endpoint /greeting
that greets the user based on their language preference. The accept_language
parameter is automatically populated with the client’s language preference based on the Accept-Language
header in the request. If the language preference starts with “es” (Spanish), the API responds with a Spanish greeting; otherwise, it responds with an English greeting.
To localize error messages, FastAPI provides a translation mechanism through the HTTPException
class. You can subclass the HTTPException
class and override its detail
attribute with a localized error message. Here’s an example:
from fastapi import HTTPException class CustomException(HTTPException): def __init__(self, status_code: int, detail: str = None, headers: dict = None): if detail is None: if status_code == 404: detail = "Recurso no encontrado" elif status_code == 500: detail = "Error interno del servidor" super().__init__(status_code, detail, headers)
In the example above, we define a custom exception class CustomException
that subclasses HTTPException
. We override the detail
attribute with localized error messages for specific status codes (e.g., 404 and 500).
These are just a few examples of how FastAPI can be used to design a multilingual API. FastAPI’s flexibility and integration with Pydantic make it a useful framework for developing APIs that can handle different languages and localization requirements.
Related Article: How to Integrate Python with MySQL for Database Queries
FastAPI’s Approach to Internationalization and Encoding
FastAPI takes a pragmatic approach to internationalization and encoding, providing developers with the necessary tools and flexibility to handle multilingual data and character encoding issues.
FastAPI leverages the power of Pydantic models for data validation, making it easy to define and enforce data schemas for incoming requests. Pydantic supports various types, including string types that can handle Unicode characters. By using Pydantic models, you can ensure that your API handles multilingual data correctly and consistently.
FastAPI also provides built-in support for language negotiation through the accept_language
parameter in path operations. This allows you to serve localized responses based on the client’s language preference without additional code complexity. FastAPI automatically parses the Accept-Language
header and provides the language preference as a parameter to your API endpoint.
To handle character encoding, FastAPI relies on the underlying Python ecosystem and the handling of strings as Unicode by default. By working with Unicode strings and using proper encoding and decoding mechanisms, you can ensure that your API handles different character sets and languages correctly.
FastAPI also supports the use of external libraries and tools for localization and internationalization. This allows you to leverage existing libraries, such as gettext, for translating UI text and supporting multiple languages in your API.
Overall, FastAPI’s approach to internationalization and encoding provides developers with the flexibility and tools needed to build robust and multilingual APIs.
Leveraging Pydantic Models for i18n in FastAPI
Pydantic models are a useful tool for handling internationalization (i18n) in FastAPI. By leveraging Pydantic models, you can easily define data schemas that support multilingual data and ensure proper data validation.
To handle i18n in FastAPI using Pydantic models, you can define fields with string types that support Unicode characters. Pydantic supports various string types, such as str
, constr
, and conbytes
, which can handle Unicode characters and different encodings. Here’s an example:
from pydantic import BaseModel class Product(BaseModel): name: str description: str
In the example above, we define a Pydantic model called Product
with name
and description
fields. Both fields are defined as str
types, which can handle Unicode characters. This allows you to store and process multilingual data in your API.
When validating incoming data against a Pydantic model, FastAPI automatically handles the i18n aspects, such as character encoding and language-specific validation rules. This ensures that your API can handle different languages and character sets correctly.
Ensuring Proper Character Encoding in API Responses
Proper character encoding is crucial when building APIs that support different languages and character sets. FastAPI, with its integration with the underlying Python ecosystem, ensures that API responses are encoded correctly based on the client’s language preference.
FastAPI follows the UTF-8 encoding by default, which is considered the standard encoding for the web and supports a wide range of characters. When returning API responses, FastAPI automatically encodes the response content using UTF-8, ensuring that characters from different languages are properly represented.
Here’s an example of returning a Unicode string in a FastAPI response:
from fastapi import FastAPI app = FastAPI() @app.get("/greeting") async def greet(): return {"message": "Hello, 世界"}
In the example above, the /greeting
endpoint returns a JSON response with a Unicode string “Hello, 世界”. FastAPI automatically encodes the response content using UTF-8, ensuring that the Unicode characters are properly represented in the response.
FastAPI’s approach to character encoding in API responses ensures that your API can handle different languages and character sets correctly, providing a seamless experience for users across the globe.
Related Article: 16 Amazing Python Libraries You Can Use Now
Challenges of Handling Multilingual Data in an API
Handling multilingual data in an API can present various challenges, including character encoding issues, data validation, and translation of UI text. FastAPI provides features and tools to help overcome these challenges and build robust multilingual APIs.
One challenge is ensuring proper character encoding and decoding of multilingual data. Different languages and character sets require specific encoding schemes to represent and transmit text correctly. FastAPI relies on the underlying Python ecosystem, which supports Unicode strings by default and uses UTF-8 encoding, one of the most widely used encoding schemes. By working with Unicode strings and using proper encoding and decoding mechanisms, you can ensure that your API can handle multilingual data correctly.
Data validation is another challenge when handling multilingual data in an API. Validating data in different languages requires considering language-specific validation rules and potential issues related to text rendering and layout. FastAPI, with its integration with Pydantic, provides a useful data validation mechanism that supports multilingual data. By defining Pydantic models and validating incoming data against these models, you can ensure that only valid data is processed in your API.
Translation of UI text is yet another challenge when building multilingual APIs. FastAPI allows you to separate UI text from code and provides support for localization through external libraries, such as gettext. By storing translatable resources in external files and using localization libraries, you can easily translate UI text and support multiple languages in your API.
Overcoming the challenges of handling multilingual data in an API requires careful consideration of character encoding, data validation, and translation. FastAPI’s features and integrations with Pydantic and external libraries make it easier to handle these challenges and build robust multilingual APIs.
Encoding Requirements for Internationalization in FastAPI
When implementing internationalization (i18n) in FastAPI, it is important to ensure proper encoding of text to support different languages and character sets. FastAPI follows the UTF-8 encoding by default, which is widely supported and can handle a wide range of characters.
To meet the encoding requirements for i18n in FastAPI, you should adhere to the following guidelines:
1. Use Unicode strings: Use Unicode strings to represent text in your API. By default, FastAPI handles Unicode strings and uses UTF-8 encoding to ensure proper representation of characters from different languages.
2. Store data in a compatible encoding: If you need to store data in a database or external system, make sure to use a compatible encoding, such as UTF-8. This ensures that the data can be properly encoded and decoded when retrieved.
3. Use proper encoding and decoding mechanisms: When working with text in your API, use proper encoding and decoding mechanisms to ensure consistent and accurate representation of characters. FastAPI relies on the underlying Python ecosystem, which handles encoding and decoding of strings using UTF-8 by default.
4. Test with different languages and character sets: To ensure that your API can handle different languages and character sets correctly, test it with various languages and character sets. This helps identify and fix any issues related to character encoding, rendering, or layout.
Tools and Libraries for Localization in a FastAPI Project
FastAPI provides flexibility and integration with external tools and libraries for localization in your project. These tools and libraries can help you handle translation of UI text, support multiple languages, and provide a seamless localization experience for your users.
Here are some popular tools and libraries that can be used for localization in a FastAPI project:
1. gettext: gettext is a widely used library for managing multilingual text in software applications. It provides functions for translating UI text based on the user’s language preference. FastAPI supports gettext integration, allowing you to easily translate UI text and support multiple languages.
2. Babel: Babel is a library that provides internationalization and localization support for Python applications. It includes features such as date and time formatting, number formatting, and pluralization. Babel can be used in conjunction with FastAPI to handle various localization tasks.
3. Flask-Babel: Flask-Babel is an extension for the Flask web framework that integrates Babel for internationalization and localization. While FastAPI is not based on Flask, you can still leverage Flask-Babel for localization in a FastAPI project by using it alongside FastAPI.
4. Polyglot: Polyglot is a library that provides a simple and lightweight approach to internationalization and localization. It supports various backend storage systems, including JSON and YAML files, and allows for easy translation of UI text. Polyglot can be used in a FastAPI project to handle localization requirements.
These are just a few examples of the tools and libraries available for localization in a FastAPI project. By leveraging these tools and libraries, you can ensure a seamless localization experience for your users and support multiple languages in your API.
Related Article: Database Query Optimization in Django: Boosting Performance for Your Web Apps
Additional Resources
– Handling language-specific data in FastAPI using Pydantic models
– Best practices for handling character encoding issues in Python
– Difference between encoding and character set