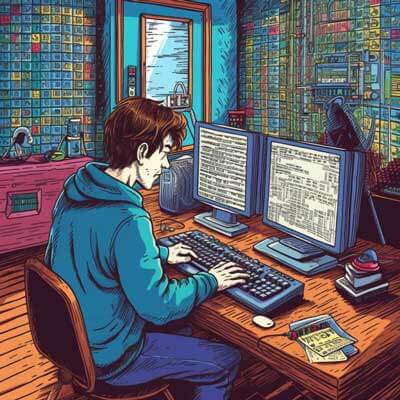
- Popular Data Structures for Storing Binary Files
- Reading Binary Data from a File
- Libraries for Reading Binary Data in Python
- Techniques for Reading Binary Data Structures
- Converting Binary Data into Structured Data
- Recommended Approach for Reading Binary Data into Data Structures
- Example of Reading Binary Data into a Data Structure
- Performance Considerations when Reading Binary Data Structures
- Advantages of Storing Data Structures in Binary Files
- Additional Resources
Popular Data Structures for Storing Binary Files
When working with binary files in Python, it is important to understand the popular data structures used for storing such files. These data structures define the organization and layout of the binary data within the file. Some commonly used data structures for storing binary files include:
1. Arrays: Arrays are a contiguous block of memory that store a fixed number of elements of the same data type. They are often used for storing homogeneous data, such as integers or floating-point numbers.
import array # Create an array of integers arr = array.array('i', [1, 2, 3, 4, 5]) # Access elements of the array print(arr[0]) # Output: 1 print(arr[2]) # Output: 3
2. Structs: Structs are used to pack and unpack binary data in a specific format. They allow you to define the layout of the binary data using format strings. Structs are useful when you need to read or write binary data with a specific structure.
import struct # Pack binary data into a struct packed_data = struct.pack('iif', 1, 2, 3.14) # Unpack binary data from a struct unpacked_data = struct.unpack('iif', packed_data) print(unpacked_data) # Output: (1, 2, 3.140000104904175)
3. Bitfields: Bitfields are used to store multiple Boolean values in a single byte. They allow you to pack multiple Boolean flags into a compact binary representation.
import ctypes # Define a bitfield structure class Flags(ctypes.LittleEndianStructure): _fields_ = [ ('flag1', ctypes.c_uint8, 1), ('flag2', ctypes.c_uint8, 1), ('flag3', ctypes.c_uint8, 1), ('flag4', ctypes.c_uint8, 1), ('reserved', ctypes.c_uint8, 4), ] # Create an instance of the bitfield flags = Flags() # Set the flag values flags.flag1 = 1 flags.flag2 = 0 flags.flag3 = 1 flags.flag4 = 1 # Access the flag values print(flags.flag1) # Output: 1 print(flags.flag2) # Output: 0 print(flags.flag3) # Output: 1 print(flags.flag4) # Output: 1
Related Article: How To Convert a Dictionary To JSON In Python
Reading Binary Data from a File
To read binary data from a file in Python, you can use the built-in open()
function with the appropriate file mode. By default, the open()
function opens a file in text mode, which is not suitable for reading binary data. To open a file in binary mode, you need to specify the 'rb'
mode.
# Open a binary file in read mode with open('binary_file.bin', 'rb') as file: # Read binary data from the file data = file.read() # Process the binary data # ...
Once you have read the binary data from the file, you can process it according to the specific data structure used to store the data.
Libraries for Reading Binary Data in Python
Python provides several libraries for reading binary data, each with its own advantages and use cases. Some popular libraries for reading binary data in Python include:
1. struct
: The struct
module is a built-in Python module that provides functions for packing and unpacking binary data. It allows you to define the layout of the binary data using format strings and provides functions for converting between binary data and Python data types.
import struct # Pack binary data into a struct packed_data = struct.pack('iif', 1, 2, 3.14) # Unpack binary data from a struct unpacked_data = struct.unpack('iif', packed_data) print(unpacked_data) # Output: (1, 2, 3.140000104904175)
2. array
: The array
module provides a high-performance array object that can be used to store and manipulate homogeneous data efficiently. It supports a wide range of data types and provides functions for reading and writing binary data from and to files.
import array # Create an array of integers arr = array.array('i', [1, 2, 3, 4, 5]) # Write the array to a binary file with open('array.bin', 'wb') as file: arr.tofile(file) # Read the array from the binary file with open('array.bin', 'rb') as file: arr.fromfile(file, len(arr)) print(arr) # Output: array('i', [1, 2, 3, 4, 5])
3. numpy
: The numpy
library provides a useful array object called ndarray
that can be used to store and manipulate n-dimensional arrays efficiently. It supports a wide range of data types and provides functions for reading and writing binary data from and to files.
import numpy as np # Create a numpy array of integers arr = np.array([1, 2, 3, 4, 5], dtype=np.int32) # Save the array to a binary file np.save('numpy_array.npy', arr) # Load the array from the binary file loaded_arr = np.load('numpy_array.npy') print(loaded_arr) # Output: [1 2 3 4 5]
Techniques for Reading Binary Data Structures
When reading binary data structures in Python, there are several techniques you can use depending on the specific data structure and its layout. Some common techniques include:
1. Using the struct
module: The struct
module provides functions for packing and unpacking binary data according to a specified format. You can use the struct.pack()
function to pack Python data into binary data and the struct.unpack()
function to unpack binary data into Python data.
import struct # Pack binary data into a struct packed_data = struct.pack('iif', 1, 2, 3.14) # Unpack binary data from a struct unpacked_data = struct.unpack('iif', packed_data) print(unpacked_data) # Output: (1, 2, 3.140000104904175)
2. Using the array
module: The array
module provides a high-performance array object that can be used to store and manipulate homogeneous data efficiently. You can use the array.fromfile()
function to read binary data from a file into an array and the array.tofile()
function to write an array to a binary file.
import array # Create an array of integers arr = array.array('i', [1, 2, 3, 4, 5]) # Write the array to a binary file with open('array.bin', 'wb') as file: arr.tofile(file) # Read the array from the binary file with open('array.bin', 'rb') as file: arr.fromfile(file, len(arr)) print(arr) # Output: array('i', [1, 2, 3, 4, 5])
3. Using the numpy
library: The numpy
library provides a useful array object called ndarray
that can be used to store and manipulate n-dimensional arrays efficiently. You can use the numpy.fromfile()
function to read binary data from a file into an array and the numpy.tofile()
function to write an array to a binary file.
import numpy as np # Create a numpy array of integers arr = np.array([1, 2, 3, 4, 5], dtype=np.int32) # Save the array to a binary file np.save('numpy_array.npy', arr) # Load the array from the binary file loaded_arr = np.load('numpy_array.npy') print(loaded_arr) # Output: [1 2 3 4 5]
Related Article: How to Sort a Dictionary by Key in Python
Converting Binary Data into Structured Data
When working with binary data structures in Python, it is often necessary to convert the binary data into structured data that can be easily manipulated and processed. This can be done using various techniques, depending on the specific data structure and its layout.
One common technique is to use the struct
module to unpack the binary data into a tuple or a named tuple. The struct.unpack()
function can be used to unpack binary data according to a specified format string. The format string specifies the layout of the binary data and the data types of the fields.
import struct # Define a struct format string format_string = 'iif' # Create a binary data string binary_data = struct.pack(format_string, 1, 2, 3.14) # Unpack the binary data into a tuple unpacked_data = struct.unpack(format_string, binary_data) print(unpacked_data) # Output: (1, 2, 3.140000104904175)
Another technique is to use the array
module to read the binary data into an array and then convert the array into a list or another data structure. The array.fromfile()
function can be used to read binary data from a file into an array.
import array # Create an array of integers arr = array.array('i') # Read binary data from a file into the array with open('binary_file.bin', 'rb') as file: arr.fromfile(file, 5) # Convert the array into a list data_list = list(arr) print(data_list) # Output: [1, 2, 3, 4, 5]
You can also use the numpy
library to read the binary data into a numpy array and then manipulate the array using its useful array operations.
import numpy as np # Read binary data from a file into a numpy array arr = np.fromfile('binary_file.bin', dtype=np.int32) print(arr) # Output: [1 2 3 4 5]
Recommended Approach for Reading Binary Data into Data Structures
When reading binary data into data structures in Python, the recommended approach depends on the specific requirements and constraints of the application. However, a general recommended approach is to use the struct
module for reading binary data structures.
The struct
module provides functions for packing and unpacking binary data according to a specified format string. By defining the layout of the binary data using a format string, you can easily unpack the binary data into a structured representation that can be easily manipulated and processed.
Here is an example of reading binary data into a structured representation using the struct
module:
import struct # Define a struct format string format_string = 'iif' # Read binary data from a file with open('binary_file.bin', 'rb') as file: binary_data = file.read() # Unpack the binary data into a structured representation unpacked_data = struct.unpack_from(format_string, binary_data) print(unpacked_data) # Output: (1, 2, 3.140000104904175)
This approach allows you to easily handle different data types and structures by simply changing the format string. It provides a flexible and efficient way to read binary data into data structures in Python.
Example of Reading Binary Data into a Data Structure
Let’s consider an example where we have a binary file that stores information about employees in a company. Each employee record is a fixed-length binary structure that contains fields such as employee ID, name, age, and salary. We can use the struct
module to read the binary data into a structured representation.
import struct # Define the struct format string for an employee record format_string = 'i20sii' # Read binary data from the file with open('employees.bin', 'rb') as file: binary_data = file.read() # Calculate the number of records in the file num_records = len(binary_data) // struct.calcsize(format_string) # Unpack the binary data into structured representations employees = [] for i in range(num_records): offset = i * struct.calcsize(format_string) record = struct.unpack_from(format_string, binary_data, offset) employees.append(record) # Process the structured representations for employee in employees: employee_id, name, age, salary = employee print(f"Employee ID: {employee_id}, Name: {name.decode().strip()}, Age: {age}, Salary: {salary}")
In this example, we first define the struct format string for an employee record. The format string specifies the layout of the binary data, with each field represented by a format specifier. We then read the binary data from the file and calculate the number of records in the file.
Using a loop, we unpack each employee record from the binary data using the struct.unpack_from()
function. The unpack_from()
function allows us to unpack a structured representation from a specific offset within the binary data. We append each record to a list of employees.
Finally, we process the structured representations by iterating over the list of employees. We extract the individual fields from each employee record and print them out.
Related Article: How to Remove a Key from a Python Dictionary
Performance Considerations when Reading Binary Data Structures
When reading binary data structures in Python, performance considerations are important to ensure efficient and optimal processing. Here are some performance considerations to keep in mind:
1. Minimize I/O operations: Reading binary data from a file involves I/O operations, which can be slow. Minimize the number of I/O operations by reading larger chunks of data at once instead of reading small chunks multiple times.
2. Use buffered I/O: Buffered I/O can significantly improve performance by reducing the number of system calls and minimizing the overhead of I/O operations. Use buffered I/O for reading binary data from files by opening the file in binary mode and using the read()
function to read larger chunks of data.
# Open a binary file in read mode with buffered I/O with open('binary_file.bin', 'rb', buffering=4096) as file: # Read binary data from the file data = file.read(4096) # Process the binary data # ...
3. Preallocate memory: When reading binary data into data structures such as arrays or numpy arrays, preallocate the memory for the data structure to avoid unnecessary resizing and copying of data.
import numpy as np # Preallocate memory for a numpy array arr = np.empty(1000000, dtype=np.int32) # Read binary data from a file into the array with open('binary_file.bin', 'rb') as file: file.readinto(arr) # Process the array # ...
4. Use efficient data structures: Choose the most efficient data structure for your specific use case. For example, if you need to perform numerical computations on the binary data, consider using numpy arrays, which provide efficient and optimized operations for numerical data.
import numpy as np # Read binary data from a file into a numpy array arr = np.fromfile('binary_file.bin', dtype=np.float64) # Perform numerical computations on the array result = np.mean(arr) print(result)
These performance considerations can help improve the efficiency and speed of reading binary data structures in Python.
Advantages of Storing Data Structures in Binary Files
Storing data structures in binary files has several advantages over other storage formats. Some of the advantages include:
1. Efficiency: Binary files are more space-efficient compared to text-based formats like CSV or JSON. Binary files store data in a raw, compact format, without the overhead of metadata and formatting characters. This makes binary files ideal for storing large datasets or complex data structures.
2. Performance: Reading and writing binary files is generally faster compared to text-based formats. Binary files require less parsing and conversion, resulting in faster I/O operations. This is particularly important when working with large datasets or when performance is a critical factor.
3. Compatibility: Binary files can be easily read and written by programs written in different programming languages. Since binary files store data in a raw format, they can be interpreted and processed by any program that understands the underlying data structure.
4. Security: Binary files can provide a higher level of security compared to text-based formats. Since binary files store data in a raw format, it is more difficult for unauthorized users to tamper with or modify the data. This can be important when working with sensitive data or when data integrity is critical.
5. Flexibility: Binary files allow for more flexibility in terms of data organization and structure. Unlike text-based formats that have predefined fields and formatting rules, binary files can be customized to fit specific data structures and requirements. This makes binary files suitable for a wide range of applications and use cases.
Additional Resources
– Reading and Writing Binary Files in Python
– Python struct – Working with Binary Data
– Python File Handling