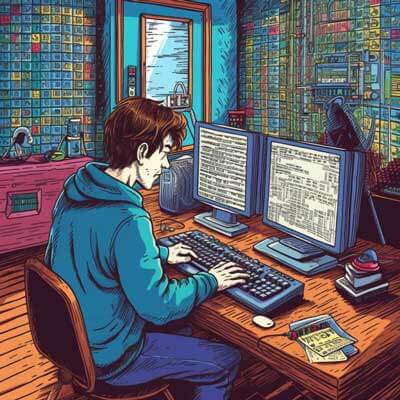
Introduction
In Python, there is no direct equivalent to the foreach loop found in some other programming languages. However, there are several ways to achieve similar functionality in Python. In this answer, we will explore two possible approaches to implementing a foreach equivalent in Python.
Related Article: How To Limit Floats To Two Decimal Points In Python
Method 1: Using a for loop
One way to achieve foreach-like behavior in Python is by using a for loop. The for loop in Python is designed to iterate over a sequence of elements, such as a list, tuple, or string. Here’s an example:
fruits = ['apple', 'banana', 'orange'] for fruit in fruits: print(fruit)
Output:
apple banana orange
In this example, the for loop iterates over each element in the fruits
list and assigns it to the variable fruit
. The print statement then displays each fruit on a new line.
Method 2: Using the built-in iter()
and next()
functions
Another way to achieve foreach-like behavior in Python is by using the built-in iter()
and next()
functions. The iter()
function returns an iterator object, which can be used to iterate over elements. The next()
function is used to retrieve the next element from the iterator. Here’s an example:
fruits = ['apple', 'banana', 'orange'] fruits_iter = iter(fruits) while True: try: fruit = next(fruits_iter) print(fruit) except StopIteration: break
Output:
apple banana orange
In this example, we first create an iterator object using the iter()
function and assign it to the variable fruits_iter
. Then, we use a while loop to continuously retrieve the next element from the iterator using the next()
function until a StopIteration
exception is raised, indicating that there are no more elements to iterate over.
Alternative Ideas
While the two methods described above are the most common ways to implement a foreach equivalent in Python, there are other alternative approaches that can be used depending on the specific requirements of your code. These alternatives include:
1. Using list comprehensions: List comprehensions provide a concise way to create lists based on existing lists. They can also be used to iterate over elements and perform operations on them. Here’s an example:
fruits = ['apple', 'banana', 'orange'] fruits_uppercase = [fruit.upper() for fruit in fruits] print(fruits_uppercase)
Output:
['APPLE', 'BANANA', 'ORANGE']
In this example, we use a list comprehension to iterate over each element in the fruits
list and convert it to uppercase using the upper()
method.
2. Using the map()
function: The map()
function is a built-in function in Python that applies a given function to each element of an iterable and returns an iterator of the results. Here’s an example:
fruits = ['apple', 'banana', 'orange'] def print_fruit(fruit): print(fruit) list(map(print_fruit, fruits))
Output:
apple banana orange
In this example, we define a function print_fruit()
that takes a single argument and prints it. We then use the map()
function to apply this function to each element in the fruits
list.
Related Article: How To Rename A File With Python
Best Practices
When implementing a foreach equivalent in Python, it is important to follow some best practices to ensure clean and readable code:
1. Use descriptive variable names: Instead of using generic variable names like item
or element
, choose more meaningful names that reflect the purpose of the iteration.
2. Choose the appropriate method based on the context: Depending on the specific requirements of your code, one method may be more suitable than the other. Consider factors such as code readability, performance, and compatibility with other parts of your code.
3. Consider using list comprehensions or the map()
function for more complex operations: If you need to perform more complex operations on each element, such as transforming or filtering the data, list comprehensions or the map()
function can provide a more concise and expressive solution.