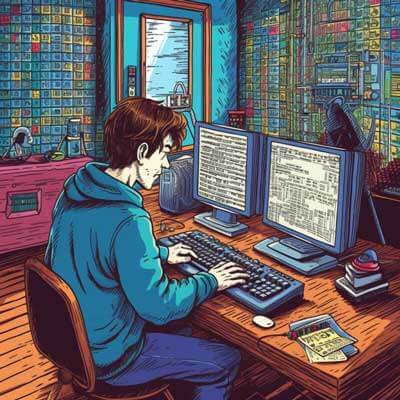
Table of Contents
Here are some useful Python code snippets we hope might come handy for your next project.
Finding the Maximum Value in a List
Finding the maximum value in a list is a common task in Python. You can use the built-in max()
function to accomplish this. The max()
function takes an iterable as an argument and returns the maximum value from that iterable.
Here's an example that demonstrates how to find the maximum value in a list:
numbers = [5, 2, 9, 1, 7] max_value = max(numbers) print(max_value) # Output: 9
In this example, we have a list of numbers [5, 2, 9, 1, 7]
. We use the max()
function to find the maximum value in the list and assign it to the variable max_value
. Finally, we print the max_value
, which outputs 9
.
You can also find the maximum value in a list of strings by using the max()
function. In this case, the maximum value will be the string that comes last alphabetically.
fruits = ['apple', 'banana', 'cherry', 'date'] max_fruit = max(fruits) print(max_fruit) # Output: cherry
In this example, the max()
function returns the string 'cherry'
, which comes last alphabetically in the list of fruits.
Note that if you have a list of objects that are not numbers or strings, the max()
function will return the object with the highest value according to its implementation of the comparison operators.
Related Article: How to Use a Foreach Function in Python 3
Reversing a String
To reverse a string in Python, you can use slicing. Slicing allows you to extract a portion of a string by specifying the start and end indices. By specifying a negative step value, you can reverse the order of the characters in the string.
Here's an example that demonstrates how to reverse a string using slicing:
text = "Hello, World!" reversed_text = text[::-1] print(reversed_text) # Output: "!dlroW ,olleH"
In this example, we have a string text
with the value "Hello, World!"
. We use slicing with a step value of -1
to reverse the characters in the string and assign the result to the variable reversed_text
. Finally, we print the reversed_text
, which outputs "!dlroW ,olleH"
.
You can also reverse a string by converting it to a list, using the reverse()
method, and then converting it back to a string.
text = "Hello, World!" reversed_text = ''.join(reversed(list(text))) print(reversed_text) # Output: "!dlroW ,olleH"
In this example, we convert the string text
to a list of characters using the list()
function. We then use the reversed()
function to reverse the order of the characters in the list. Finally, we join the reversed list of characters back into a string using the join()
method and assign the result to the variable reversed_text
. The output is the same as before: "!dlroW ,olleH"
.
Counting the Occurrence of a Character in a String
To count the occurrence of a character in a string in Python, you can use the count()
method. The count()
method takes a character as an argument and returns the number of times that character appears in the string.
Here's an example that demonstrates how to count the occurrence of a character in a string:
text = "Hello, World!" count = text.count('o') print(count) # Output: 2
In this example, we have a string text
with the value "Hello, World!"
. We use the count()
method with the character 'o'
as the argument to count the number of times 'o'
appears in the string. The result is assigned to the variable count
, and we print the count
, which outputs 2
.
You can also count the occurrence of a substring in a string by using the count()
method with the substring as the argument.
text = "Hello, World!" count = text.count('llo') print(count) # Output: 1
In this example, the count()
method returns 1
because the substring 'llo'
appears only once in the string.
Note that the count()
method is case-sensitive. If you want to count the occurrence of a character or substring regardless of case, you can convert the string and the character or substring to lowercase or uppercase using the lower()
or upper()
method before calling the count()
method.
Checking if a String is a Palindrome
A palindrome is a word, phrase, number, or sequence of characters that reads the same backward as forward. To check if a string is a palindrome in Python, you can compare the string with its reverse.
Here's an example that demonstrates how to check if a string is a palindrome:
def is_palindrome(string): reversed_string = string[::-1] return string == reversed_string text = "radar" is_palindrome = is_palindrome(text) print(is_palindrome) # Output: True
In this example, we define a function is_palindrome()
that takes a string as an argument. The function uses slicing to reverse the string and assigns the reversed string to the variable reversed_string
. The function then compares the original string with the reversed string and returns True
if they are equal, indicating that the string is a palindrome.
We test the function by passing the string "radar"
as an argument and assign the result to the variable is_palindrome
. Finally, we print the is_palindrome
, which outputs True
.
Note that the comparison is case-sensitive. If you want to ignore case when checking for palindromes, you can convert the string to lowercase using the lower()
method before comparing.
def is_palindrome(string): reversed_string = string.lower()[::-1] return string.lower() == reversed_string text = "Racecar" is_palindrome = is_palindrome(text) print(is_palindrome) # Output: True
In this example, we convert both the original string and the reversed string to lowercase before comparing them. This ensures that the function returns True
even if the string has different capitalization.
Related Article: How to Plot a Histogram in Python Using Matplotlib with List Data
Sorting a List in Ascending Order
Sorting a list in ascending order is a common operation in Python. You can use the sorted()
function to sort a list in ascending order.
Here's an example that demonstrates how to sort a list in ascending order:
numbers = [5, 2, 9, 1, 7] sorted_numbers = sorted(numbers) print(sorted_numbers) # Output: [1, 2, 5, 7, 9]
In this example, we have a list of numbers [5, 2, 9, 1, 7]
. We use the sorted()
function to sort the numbers in ascending order and assign the sorted list to the variable sorted_numbers
. Finally, we print the sorted_numbers
, which outputs [1, 2, 5, 7, 9]
.
You can also sort a list of strings in ascending order using the sorted()
function. In this case, the strings will be sorted alphabetically.
fruits = ['apple', 'banana', 'cherry', 'date'] sorted_fruits = sorted(fruits) print(sorted_fruits) # Output: ['apple', 'banana', 'cherry', 'date']
In this example, the sorted()
function returns a sorted list of fruits ['apple', 'banana', 'cherry', 'date']
, which is sorted alphabetically.
Note that the sorted()
function creates a new sorted list and does not modify the original list. If you want to sort the original list in-place, you can use the sort()
method.
numbers = [5, 2, 9, 1, 7] numbers.sort() print(numbers) # Output: [1, 2, 5, 7, 9]
In this example, we use the sort()
method instead of the sorted()
function to sort the numbers list in-place. The output is the same as before: [1, 2, 5, 7, 9]
.
Removing Duplicates from a List
Removing duplicates from a list is a common task in Python. You can use the set()
function or a list comprehension to remove duplicates from a list.
Here's an example that demonstrates how to remove duplicates from a list using the set()
function:
numbers = [2, 5, 2, 1, 7, 5, 9] unique_numbers = list(set(numbers)) print(unique_numbers) # Output: [1, 2, 5, 7, 9]
In this example, we have a list of numbers [2, 5, 2, 1, 7, 5, 9]
. We use the set()
function to create a set from the list, which automatically removes duplicates. We then convert the set back to a list using the list()
function and assign the result to the variable unique_numbers
. Finally, we print the unique_numbers
, which outputs [1, 2, 5, 7, 9]
.
Note that the order of the elements may change when using the set()
function, as sets are unordered collections. If you need to preserve the order of the elements, you can use a list comprehension instead.
numbers = [2, 5, 2, 1, 7, 5, 9] unique_numbers = [x for i, x in enumerate(numbers) if x not in numbers[:i]] print(unique_numbers) # Output: [2, 5, 1, 7, 9]
In this example, we use a list comprehension to iterate over the elements of the numbers
list. We check if each element is not in the preceding elements of the list using slicing. If the element is not in the preceding elements, it is added to the unique_numbers
list. The output is [2, 5, 1, 7, 9]
, which preserves the order of the elements.
Calculating the Average of Numbers in a List
Calculating the average of numbers in a list is a common mathematical operation. You can use the sum()
function and the len()
function to calculate the average.
Here's an example that demonstrates how to calculate the average of numbers in a list:
numbers = [5, 2, 9, 1, 7] average = sum(numbers) / len(numbers) print(average) # Output: 4.8
In this example, we have a list of numbers [5, 2, 9, 1, 7]
. We use the sum()
function to calculate the sum of the numbers and the len()
function to get the number of elements in the list. We then divide the sum by the length to calculate the average and assign it to the variable average
. Finally, we print the average
, which outputs 4.8
.
Note that the average is a floating-point value. If you want to round the average to a specific number of decimal places, you can use the round()
function.
numbers = [5, 2, 9, 1, 7] average = round(sum(numbers) / len(numbers), 2) print(average) # Output: 4.8
In this example, we use the round()
function to round the average to two decimal places. The output is the same as before: 4.8
.
Converting a String to Title Case
Title case is a capitalization scheme where the first letter of each word is capitalized. To convert a string to title case in Python, you can use the title()
method.
Here's an example that demonstrates how to convert a string to title case:
text = "hello, world!" title_case_text = text.title() print(title_case_text) # Output: "Hello, World!"
In this example, we have a string text
with the value "hello, world!"
. We use the title()
method to convert the string to title case and assign the result to the variable title_case_text
. Finally, we print the title_case_text
, which outputs "Hello, World!"
.
The title()
method capitalizes the first letter of each word in the string and converts the rest of the letters to lowercase. If you have a string with all lowercase letters and you want to capitalize the first letter of each word, you can use the capitalize()
method.
text = "hello, world!" capitalized_text = ' '.join(word.capitalize() for word in text.split()) print(capitalized_text) # Output: "Hello, World!"
In this example, we use a list comprehension to split the string into words using the split()
method. We then capitalize the first letter of each word using the capitalize()
method and join the capitalized words back into a string using the join()
method with a space as the separator. The output is the same as before: "Hello, World!"
.
Related Article: Deploying Flask Web Apps: From WSGI to Kubernetes
Checking if a List is Empty
To check if a list is empty in Python, you can use the not
operator and the bool()
function. The bool()
function returns False
if the list is empty and True
otherwise.
Here's an example that demonstrates how to check if a list is empty:
empty_list = [] if not bool(empty_list): print("The list is empty.") else: print("The list is not empty.")
In this example, we have an empty list empty_list
. We use the bool()
function to convert the list to a boolean value. Since the list is empty, the bool()
function returns False
, and the not
operator negates the result to True
. Therefore, the code inside the if
statement is executed, and "The list is empty."
is printed.
If you have a list with elements, the bool()
function will return True
, indicating that the list is not empty.
non_empty_list = [1, 2, 3] if not bool(non_empty_list): print("The list is empty.") else: print("The list is not empty.")
In this example, the bool()
function returns True
because the list non_empty_list
has elements. Therefore, the code inside the else
statement is executed, and "The list is not empty."
is printed.
Note that the if
statement can be simplified by directly evaluating the list in a boolean context.
empty_list = [] if not empty_list: print("The list is empty.") else: print("The list is not empty.")
In this simplified example, the empty list empty_list
is directly evaluated in a boolean context. Since the list is empty, the condition not empty_list
evaluates to True
, and the code inside the if
statement is executed, resulting in the same output as before: "The list is empty."
.
Finding the Index of an Element in a List
To find the index of an element in a list in Python, you can use the index()
method. The index()
method takes an element as an argument and returns the index of the first occurrence of that element in the list.
Here's an example that demonstrates how to find the index of an element in a list:
fruits = ['apple', 'banana', 'cherry', 'date'] index = fruits.index('banana') print(index) # Output: 1
In this example, we have a list of fruits ['apple', 'banana', 'cherry', 'date']
. We use the index()
method with the element 'banana'
as the argument to find its index in the list. The result is assigned to the variable index
, and we print the index
, which outputs 1
.
If the element is not present in the list, the index()
method raises a ValueError
. To avoid this, you can check if the element is in the list before calling the index()
method.
fruits = ['apple', 'banana', 'cherry', 'date'] if 'banana' in fruits: index = fruits.index('banana') print(index) # Output: 1 else: print("The element is not in the list.")
In this example, we check if the element 'banana'
is in the list fruits
using the in
operator. Since 'banana'
is present in the list, the code inside the if
statement is executed, and the index is printed as before.
Note that the index()
method returns the index of the first occurrence of the element. If the element appears multiple times in the list, only the index of the first occurrence is returned. If you need to find the indices of all occurrences, you can use a list comprehension.
fruits = ['apple', 'banana', 'cherry', 'banana'] indices = [i for i, x in enumerate(fruits) if x == 'banana'] print(indices) # Output: [1, 3]
In this example, we use a list comprehension to iterate over the elements of the fruits
list and their indices using the enumerate()
function. We check if each element is equal to 'banana'
and add the index to the indices
list if it is. The output is [1, 3]
, which are the indices of the occurrences of 'banana'
in the list.
Creating a Dictionary from Two Lists
To create a dictionary from two lists in Python, you can use the zip()
function and the dictionary comprehension. The zip()
function combines the elements of the two lists into pairs, and the dictionary comprehension creates key-value pairs from those pairs.
Here's an example that demonstrates how to create a dictionary from two lists:
keys = ['a', 'b', 'c'] values = [1, 2, 3] dictionary = {k: v for k, v in zip(keys, values)} print(dictionary) # Output: {'a': 1, 'b': 2, 'c': 3}
In this example, we have two lists keys
and values
with the values ['a', 'b', 'c']
and [1, 2, 3]
, respectively. We use the zip()
function to combine the elements of the two lists into pairs. The dictionary comprehension {k: v for k, v in zip(keys, values)}
creates key-value pairs from the pairs, resulting in the dictionary {'a': 1, 'b': 2, 'c': 3}
. Finally, we print the dictionary
.
If the two lists have different lengths, the resulting dictionary will only contain key-value pairs up to the length of the shorter list.
keys = ['a', 'b', 'c'] values = [1, 2] dictionary = {k: v for k, v in zip(keys, values)} print(dictionary) # Output: {'a': 1, 'b': 2}
In this example, the values
list has only two elements, so the resulting dictionary contains only two key-value pairs.
If you have more than two lists, you can use the zip()
function with multiple lists and the dictionary comprehension to create a dictionary with multiple values per key.
keys = ['a', 'b', 'c'] values1 = [1, 2, 3] values2 = ['x', 'y', 'z'] dictionary = {k: (v1, v2) for k, v1, v2 in zip(keys, values1, values2)} print(dictionary) # Output: {'a': (1, 'x'), 'b': (2, 'y'), 'c': (3, 'z')}
In this example, we have three lists keys
, values1
, and values2
. We use the zip()
function to combine the elements of the three lists into triplets. The dictionary comprehension {k: (v1, v2) for k, v1, v2 in zip(keys, values1, values2)}
creates key-value pairs from the triplets, resulting in a dictionary with multiple values per key.
Reading a Text File
To read the contents of a text file in Python, you can use the built-in open()
function with the file mode set to 'r'
(read). The open()
function returns a file object, and you can use the read()
method of the file object to read the contents as a string.
Here's an example that demonstrates how to read the contents of a text file:
with open('example.txt', 'r') as file: contents = file.read() print(contents)
In this example, we use the open()
function to open the file 'example.txt'
in read mode. We use a with
statement to ensure that the file is automatically closed after reading. We call the read()
method of the file object to read the contents of the file as a string, and we assign the result to the variable contents
. Finally, we print the contents
.
Note that you need to provide the correct file path or filename for the open()
function to work. If the file is not in the same directory as your Python script, you need to specify the relative or absolute path to the file.
If you want to read the contents of a text file line by line instead of as a single string, you can use the readlines()
method of the file object. The readlines()
method returns a list of strings, where each string represents a line in the file.
with open('example.txt', 'r') as file: lines = file.readlines() for line in lines: print(line)
In this example, we use the readlines()
method to read the contents of the file line by line. We assign the list of lines to the variable lines
, and we iterate over the lines using a for
loop. Inside the loop, we print each line.
Related Article: How to Implement a Python Progress Bar
Writing to a Text File
To write content to a text file in Python, you can use the built-in open()
function with the file mode set to 'w'
(write). The open()
function returns a file object, and you can use the write()
method of the file object to write content to the file.
Here's an example that demonstrates how to write content to a text file:
with open('example.txt', 'w') as file: file.write('Hello, World!')
In this example, we use the open()
function to open the file 'example.txt'
in write mode. We use a with
statement to ensure that the file is automatically closed after writing. We call the write()
method of the file object to write the string 'Hello, World!'
to the file.
Note that if the file already exists, the 'w'
mode will overwrite the existing content. If you want to append content to an existing file or create a new file if it doesn't exist, you can use the 'a'
(append) mode or the 'x'
(exclusive creation) mode, respectively.
If you want to write multiple lines to a text file, you can use the write()
method multiple times, separating the lines with newline characters ('\n'
).
with open('example.txt', 'w') as file: file.write('Line 1\n') file.write('Line 2\n') file.write('Line 3\n')
In this example, we use the write()
method three times to write three lines to the file. Each line is followed by a newline character ('\n'
), which creates a new line in the file.
If you have a list of strings and you want to write each string as a line to a text file, you can use the writelines()
method of the file object. The writelines()
method takes an iterable of strings as an argument and writes each string as a line to the file.
lines = ['Line 1\n', 'Line 2\n', 'Line 3\n'] with open('example.txt', 'w') as file: file.writelines(lines)
In this example, we have a list of lines ['Line 1\n', 'Line 2\n', 'Line 3\n']
. We use the writelines()
method to write each line to the file. The output is the same as before: a text file with three lines.
Finding the Length of a String
To find the length of a string in Python, you can use the built-in len()
function. The len()
function returns the number of characters in the string, including spaces and punctuation.
Here's an example that demonstrates how to find the length of a string:
text = "Hello, World!" length = len(text) print(length) # Output: 13
In this example, we have a string text
with the value "Hello, World!"
. We use the len()
function to find the length of the string and assign it to the variable length
. Finally, we print the length
, which outputs 13
.
Note that the len()
function counts each character in the string, including spaces and punctuation. If you want to exclude leading and trailing spaces from the length calculation, you can use the strip()
method.
text = " Hello, World! " length = len(text.strip()) print(length) # Output: 13
In this example, we use the strip()
method to remove leading and trailing spaces from the string before calculating its length. The output is the same as before: 13
.
If you have a list of strings and you want to find the length of each string, you can use a list comprehension.
strings = ['apple', 'banana', 'cherry'] lengths = [len(s) for s in strings] print(lengths) # Output: [5, 6, 6]
In this example, we use a list comprehension to iterate over the strings in the strings
list and calculate the length of each string using the len()
function. The output is a list of lengths [5, 6, 6]
, which correspond to the lengths of the strings.
Replacing Substrings in a String
To replace substrings in a string in Python, you can use the replace()
method. The replace()
method takes two arguments: the substring to be replaced and the replacement substring.
Here's an example that demonstrates how to replace substrings in a string:
text = "Hello, World!" new_text = text.replace('Hello', 'Hi') print(new_text) # Output: "Hi, World!"
In this example, we have a string text
with the value "Hello, World!"
. We use the replace()
method to replace the substring 'Hello'
with the substring 'Hi'
and assign the result to the variable new_text
. Finally, we print the new_text
, which outputs "Hi, World!"
.
Note that the replace()
method replaces all occurrences of the substring. If you only want to replace the first occurrence, you can specify the count
parameter.
text = "Hello, World!" new_text = text.replace('o', '0', 1) print(new_text) # Output: "Hell0, World!"
In this example, we specify 1
as the count
parameter, which limits the number of replacements to 1
. Therefore, only the first occurrence of the substring 'o'
is replaced with the character '0'
.
If you have multiple substrings to replace, you can chain multiple replace()
method calls.
text = "Hello, World!" new_text = text.replace('Hello', 'Hi').replace('World', 'Universe') print(new_text) # Output: "Hi, Universe!"
In this example, we chain two replace()
method calls to replace the substrings 'Hello'
and 'World'
with the substrings 'Hi'
and 'Universe'
, respectively. The output is "Hi, Universe!"
.
Checking if a String Contains Only Digits
To check if a string contains only digits in Python, you can use the isdigit()
method. The isdigit()
method returns True
if all characters in the string are digits, and False
otherwise.
Here's an example that demonstrates how to check if a string contains only digits:
text = "12345" if text.isdigit(): print("The string contains only digits.") else: print("The string does not contain only digits.")
In this example, we have a string text
with the value "12345"
. We use the isdigit()
method to check if all characters in the string are digits. Since all characters in the string are digits, the condition text.isdigit()
evaluates to True
, and the code inside the if
statement is executed, resulting in the message "The string contains only digits."
being printed.
If the string contains non-digit characters, the isdigit()
method returns False
.
text = "12345a" if text.isdigit(): print("The string contains only digits.") else: print("The string does not contain only digits.")
In this example, the string text
contains the character 'a'
, which is not a digit. Therefore, the condition text.isdigit()
evaluates to False
, and the code inside the else
statement is executed, resulting in the message "The string does not contain only digits."
being printed.
Note that the isdigit()
method only returns True
if all characters in the string are digits. If the string contains spaces or other non-digit characters, the method will return False
. If you want to check if the string contains at least one digit, you can use the isnumeric()
method instead.
Related Article: Python Set Intersection Tutorial
Splitting a String into a List of Words
To split a string into a list of words in Python, you can use the split()
method. The split()
method splits a string into a list of words based on whitespace characters by default.
Here's an example that demonstrates how to split a string into a list of words:
text = "Hello, World!" words = text.split() print(words) # Output: ['Hello,', 'World!']
In this example, we have a string text
with the value "Hello, World!"
. We use the split()
method to split the string into words and assign the result to the variable words
. The split()
method splits the string based on whitespace characters, resulting in a list of words ['Hello,', 'World!']
. Finally, we print the words
.
Note that the split()
method treats consecutive whitespace characters as a single delimiter and removes leading and trailing whitespace. If you want to split the string based on a specific delimiter, you can specify it as the argument of the split()
method.
text = "Hello, World!" words = text.split(',') print(words) # Output: ['Hello', ' World!']
In this example, we use ','
as the argument of the split()
method. The split()
method splits the string at each occurrence of ','
, resulting in a list of words ['Hello', ' World!']
.
If you want to split the string into characters instead of words, you can pass an empty string ''
as the argument of the split()
method.
text = "Hello, World!" characters = text.split('') print(characters) # Output: ['H', 'e', 'l', 'l', 'o', ',', ' ', 'W', 'o', 'r', 'l', 'd', '!']
In this example, we pass ''
as the argument of the split()
method. The split()
method splits the string at each character, resulting in a list of characters ['H', 'e', 'l', 'l', 'o', ',', ' ', 'W', 'o', 'r', 'l', 'd', '!']
.
Checking if a List Contains a Specific Element
To check if a list contains a specific element in Python, you can use the in
operator. The in
operator returns True
if the element is present in the list, and False
otherwise.
Here's an example that demonstrates how to check if a list contains a specific element:
fruits = ['apple', 'banana', 'cherry'] if 'banana' in fruits: print("The list contains 'banana'.") else: print("The list does not contain 'banana'.")
In this example, we have a list of fruits ['apple', 'banana', 'cherry']
. We use the in
operator to check if the string 'banana'
is present in the list. Since 'banana'
is present, the condition 'banana' in fruits
evaluates to True
, and the code inside the if
statement is executed, resulting in the message "The list contains 'banana'."
being printed.
If the element is not present in the list, the in
operator returns False
.
fruits = ['apple', 'banana', 'cherry'] if 'orange' in fruits: print("The list contains 'orange'.") else: print("The list does not contain 'orange'.")
In this example, the string 'orange'
is not present in the list fruits
. Therefore, the condition 'orange' in fruits
evaluates to False
, and the code inside the else
statement is executed, resulting in the message "The list does not contain 'orange'."
being printed.
Note that the in
operator performs a linear search through the list to check if the element is present. If you have a large list and need to perform multiple checks, you may consider converting the list to a set for faster membership tests.
fruits = ['apple', 'banana', 'cherry'] fruits_set = set(fruits) if 'banana' in fruits_set: print("The list contains 'banana'.") else: print("The list does not contain 'banana'.")
In this example, we convert the list fruits
to a set fruits_set
using the set()
function. We then use the in
operator to check if 'banana'
is present in the set. The output is the same as before: "The list contains 'banana'."
.
Removing an Element from a List by Value
To remove an element from a list by value in Python, you can use the remove()
method. The remove()
method takes an element as an argument and removes the first occurrence of that element from the list.
Here's an example that demonstrates how to remove an element from a list by value:
fruits = ['apple', 'banana', 'cherry'] fruits.remove('banana') print(fruits) # Output: ['apple', 'cherry']
In this example, we have a list of fruits ['apple', 'banana', 'cherry']
. We use the remove()
method with the string 'banana'
as the argument to remove the first occurrence of 'banana'
from the list. The resulting list is ['apple', 'cherry']
, and we print the fruits
.
If the element is not present in the list, the remove()
method raises a ValueError
. To avoid this, you can check if the element is in the list before calling the remove()
method.
fruits = ['apple', 'banana', 'cherry'] if 'banana' in fruits: fruits.remove('banana') print(fruits) # Output: ['apple', 'cherry'] else: print("The element is not in the list.")
In this example, we check if the string 'banana'
is in the list fruits
using the in
operator. Since 'banana'
is present in the list, the code inside the if
statement is executed, the first occurrence of 'banana'
is removed from the list using the remove()
method, and the resulting list ['apple', 'cherry']
is printed.
Note that the remove()
method only removes the first occurrence of the element. If the element appears multiple times in the list, only the first occurrence is removed. If you need to remove all occurrences, you can use a list comprehension.
fruits = ['apple', 'banana', 'cherry', 'banana'] fruits = [x for x in fruits if x != 'banana'] print(fruits) # Output: ['apple', 'cherry']
In this example, we use a list comprehension to iterate over the elements of the fruits
list and filter out the elements that are equal to 'banana'
. The resulting list ['apple', 'cherry']
does not contain any occurrences of 'banana'
.