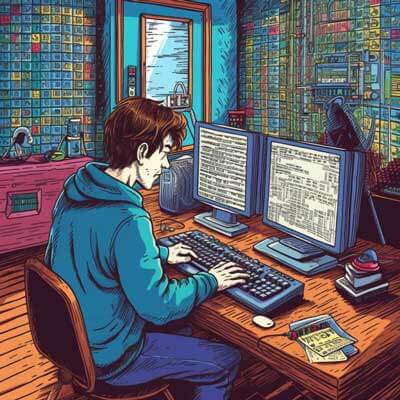
Converting bytes to a string in Python 3 is a common task when working with binary data or when dealing with file I/O operations. Python provides several methods and techniques to perform this conversion. In this answer, we will explore two common approaches to convert bytes to a string in Python 3.
Method 1: Using the decode() method
One way to convert bytes to a string in Python 3 is by using the decode()
method. This method is available on byte objects and allows you to specify the encoding to be used for the conversion.
Here’s an example that demonstrates how to use the decode()
method to convert bytes to a string:
# Define a byte object byte_data = b'Hello, World!' # Convert the byte object to a string using the decode() method string_data = byte_data.decode('utf-8') # Print the converted string print(string_data)
In the example above, we have a byte object byte_data
containing the text “Hello, World!”. We use the decode()
method with the 'utf-8'
encoding to convert the byte object to a string. The resulting string is then stored in the string_data
variable and printed.
It’s important to note that the encoding specified in the decode()
method should match the encoding of the byte object. In the example above, we assumed that the byte object was encoded using UTF-8. If the byte object has a different encoding, you should specify that encoding instead.
Related Article: How To Limit Floats To Two Decimal Points In Python
Method 2: Using the str() constructor
Another way to convert bytes to a string in Python 3 is by using the str()
constructor. This method allows you to create a string object from a byte object.
Here’s an example that demonstrates how to use the str()
constructor to convert bytes to a string:
# Define a byte object byte_data = b'Hello, World!' # Convert the byte object to a string using the str() constructor string_data = str(byte_data, 'utf-8') # Print the converted string print(string_data)
In the example above, we pass the byte object byte_data
and the 'utf-8'
encoding as arguments to the str()
constructor. The constructor then returns a string object representing the byte data. The resulting string is stored in the string_data
variable and printed.
Both the decode()
method and the str()
constructor can be used to convert bytes to a string in Python 3. The choice between the two methods depends on personal preference and the specific requirements of your code.
Best Practices
When converting bytes to a string in Python 3, it is important to consider the following best practices:
1. Specify the correct encoding: Make sure to specify the correct encoding when using the decode()
method or the str()
constructor. Using the wrong encoding can result in incorrect conversions or encoding errors.
2. Handle encoding errors: When converting bytes to a string, it is possible to encounter encoding errors, especially if the byte data is not properly encoded. To handle encoding errors, you can provide the errors
parameter to the decode()
method or the str()
constructor. This parameter allows you to specify how encoding errors should be handled.
3. Use context managers for file I/O: When working with file I/O operations that involve converting bytes to strings, it is recommended to use context managers (with
statement) to ensure that files are properly opened and closed. This helps prevent resource leaks and ensures that the file is closed even if an exception occurs.
# Example of reading bytes from a file and converting them to a string with open('data.bin', 'rb') as file: byte_data = file.read() string_data = byte_data.decode('utf-8') print(string_data)
In the example above, we use a context manager (with
statement) to open the file 'data.bin'
in binary mode ('rb'
). We then read the byte data from the file and convert it to a string using the decode()
method. Finally, we print the resulting string.
Related Article: How To Rename A File With Python