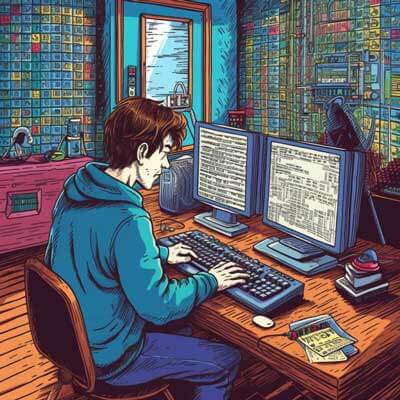
- Overview of Python Data Structures
- List – A Versatile Data Structure
- Tuple – Immutable and Ordered
- Set – Unordered Collection of Unique Elements
- Dictionary – Key-Value Pairs for Efficient Lookup
- Array – Efficient Storage and Manipulation of Homogeneous Data
- Stack – LIFO Data Structure for Last-In-First-Out Operations
- Queue – FIFO Data Structure for First-In-First-Out Operations
- Linked List – Dynamic Data Structure for Efficient Insertion and Deletion
- Heap – Efficiently Maintaining Priority Queues
- Graph – Modeling Relationships and Interconnections
- Choosing the Right Python Data Structure
- Efficient Data Structures for Large Amounts of Data
- List vs Tuple – When to Use Each
- Set vs Dictionary – Understanding the Differences
- Implementing a Stack in Python
- The Purpose of a Queue Data Structure in Python
- Implementing a Linked List in Python
- Understanding the Heap Data Structure in Python
- The Role of Graphs in Python Data Structures
- Additional Resources
Overview of Python Data Structures
Python is a versatile programming language that offers a wide range of built-in data structures to store, organize, and manipulate data. These data structures are essential tools for every Python programmer, as they provide efficient ways to manage different types of data. In this article, we will analyze the most commonly used Python data structures and explore their features, use cases, and implementation details.
Related Article: How To Convert a Dictionary To JSON In Python
List – A Versatile Data Structure
The list is one of the most commonly used data structures in Python. It is an ordered collection of elements, where each element is identified by its index. Lists in Python are mutable, which means that you can modify their elements. Lists can store elements of different data types, such as integers, strings, or even other lists.
Here is an example of creating a list in Python:
fruits = ['apple', 'banana', 'orange']
You can access elements of a list using their index:
print(fruits[0]) # Output: apple print(fruits[1]) # Output: banana print(fruits[2]) # Output: orange
Lists also support various operations, such as adding elements, removing elements, or concatenating multiple lists. Here are a few examples:
fruits.append('grape') # Adds 'grape' to the end of the list print(fruits) # Output: ['apple', 'banana', 'orange', 'grape'] fruits.remove('banana') # Removes 'banana' from the list print(fruits) # Output: ['apple', 'orange', 'grape'] numbers = [1, 2, 3] combined = fruits + numbers # Concatenates two lists print(combined) # Output: ['apple', 'orange', 'grape', 1, 2, 3]
Lists provide a flexible and versatile way to store and manipulate data in Python. They are commonly used in various applications, such as managing collections of items, implementing stacks and queues, or representing matrices and grids.
Tuple – Immutable and Ordered
A tuple is another commonly used data structure in Python. It is similar to a list, but with one key difference: tuples are immutable, which means that their elements cannot be modified once they are assigned. Tuples are often used to store related pieces of data together.
Here is an example of creating a tuple in Python:
person = ('John', 25, 'USA')
You can access elements of a tuple using their index, just like in a list:
print(person[0]) # Output: John print(person[1]) # Output: 25 print(person[2]) # Output: USA
Since tuples are immutable, you cannot modify their elements or change their size. However, you can perform operations such as concatenation or slicing:
names = ('Alice', 'Bob', 'Charlie') combined = person + names # Concatenates two tuples print(combined) # Output: ('John', 25, 'USA', 'Alice', 'Bob', 'Charlie') sliced = person[1:] # Creates a new tuple with elements from index 1 to the end print(sliced) # Output: (25, 'USA')
Tuples are useful when you want to ensure that the data remains unchanged after it is assigned. They are commonly used to represent fixed structures, such as coordinates, RGB colors, or database records.
Set – Unordered Collection of Unique Elements
A set is a data structure in Python that represents an unordered collection of unique elements. Unlike lists or tuples, sets do not preserve the order of elements and do not allow duplicate values. Sets are useful when you need to store a collection of items without any particular order or when you want to eliminate duplicate values from a sequence.
Here is an example of creating a set in Python:
fruits = {'apple', 'banana', 'orange'}
You can perform various operations on sets, such as adding elements, removing elements, or checking for membership:
fruits.add('grape') # Adds 'grape' to the set print(fruits) # Output: {'apple', 'banana', 'orange', 'grape'} fruits.remove('banana') # Removes 'banana' from the set print(fruits) # Output: {'apple', 'orange', 'grape'} print('apple' in fruits) # Output: True print('watermelon' in fruits) # Output: False
Sets also support operations like union, intersection, and difference. For example:
fruits1 = {'apple', 'banana', 'orange'} fruits2 = {'orange', 'grape', 'watermelon'} union = fruits1 | fruits2 # Creates a new set with elements from both sets print(union) # Output: {'apple', 'banana', 'orange', 'grape', 'watermelon'} intersection = fruits1 & fruits2 # Creates a new set with common elements print(intersection) # Output: {'orange'} difference = fruits1 - fruits2 # Creates a new set with elements in fruits1 but not in fruits2 print(difference) # Output: {'apple', 'banana'}
Sets are commonly used in situations where you need to perform operations like checking for duplicates or finding common elements between multiple sets. They are also useful for tasks like counting unique items or filtering out duplicates from a sequence.
Related Article: How to Sort a Dictionary by Key in Python
Dictionary – Key-Value Pairs for Efficient Lookup
A dictionary is a highly versatile data structure in Python that allows you to store and retrieve values based on unique keys. It is an unordered collection of key-value pairs, where each key is associated with a corresponding value. Dictionaries are commonly used when you need to access or update values based on their unique identifiers.
Here is an example of creating a dictionary in Python:
person = {'name': 'John', 'age': 25, 'country': 'USA'}
You can access values in a dictionary by providing the corresponding key:
print(person['name']) # Output: John print(person['age']) # Output: 25 print(person['country']) # Output: USA
Dictionaries also support various operations, such as adding new key-value pairs, updating values, or removing items:
person['occupation'] = 'Engineer' # Adds a new key-value pair to the dictionary print(person) # Output: {'name': 'John', 'age': 25, 'country': 'USA', 'occupation': 'Engineer'} person['age'] = 26 # Updates the value associated with the 'age' key print(person) # Output: {'name': 'John', 'age': 26, 'country': 'USA', 'occupation': 'Engineer'} del person['country'] # Removes the key-value pair with the key 'country' print(person) # Output: {'name': 'John', 'age': 26, 'occupation': 'Engineer'}
Dictionaries provide a fast and efficient way to store and retrieve data based on unique keys. They are commonly used in scenarios where you need to associate values with specific identifiers, such as storing user information, configuring settings, or maintaining a mapping between two sets of values.
Array – Efficient Storage and Manipulation of Homogeneous Data
An array is a data structure in Python that allows you to store and manipulate a fixed number of elements of the same data type. Unlike lists, arrays are designed for homogeneous data, meaning that all elements must have the same type. Arrays provide efficient storage and manipulation of data, as they offer direct access to individual elements based on their index.
To use arrays in Python, you need to import the array
module:
import array
Here is an example of creating an array in Python:
numbers = array.array('i', [1, 2, 3, 4, 5])
In this example, we create an array of integers ('i'
) and initialize it with a sequence of numbers.
You can access elements of an array using their index, just like in a list:
print(numbers[0]) # Output: 1 print(numbers[1]) # Output: 2 print(numbers[2]) # Output: 3
Arrays also support various operations, such as adding elements, removing elements, or modifying values:
numbers.append(6) # Adds a new element to the end of the array print(numbers) # Output: array('i', [1, 2, 3, 4, 5, 6]) numbers.insert(0, 0) # Inserts a new element at index 0 print(numbers) # Output: array('i', [0, 1, 2, 3, 4, 5, 6]) numbers[3] = 999 # Modifies the value at index 3 print(numbers) # Output: array('i', [0, 1, 2, 999, 4, 5, 6]) numbers.remove(4) # Removes the element with the value 4 print(numbers) # Output: array('i', [0, 1, 2, 999, 5, 6])
Arrays are commonly used when you need to store a large number of elements of the same data type and require efficient access and manipulation. They are particularly useful in scenarios where memory efficiency and performance are critical, such as scientific computing, numerical analysis, or handling large datasets.
Stack – LIFO Data Structure for Last-In-First-Out Operations
A stack is a specialized data structure in Python that follows the Last-In-First-Out (LIFO) principle. It is a collection of elements where the last element added is the first one to be removed. Stacks are commonly used in situations where you need to keep track of the order of items and perform operations like pushing (adding) or popping (removing) elements.
In Python, you can implement a stack using a list. Here is an example of creating a stack and performing basic operations:
stack = [] stack.append('apple') # Pushes 'apple' onto the stack stack.append('banana') # Pushes 'banana' onto the stack stack.append('orange') # Pushes 'orange' onto the stack print(stack) # Output: ['apple', 'banana', 'orange'] top = stack.pop() # Pops the top element from the stack print(top) # Output: orange print(stack) # Output: ['apple', 'banana']
In this example, we use the append()
method to push elements onto the stack and the pop()
method to remove the top element. The last element added (‘orange’) is the first one to be removed.
Stacks are widely used in various applications, such as expression evaluation, backtracking algorithms, depth-first search, or managing function calls in programming languages. They provide a simple and intuitive way to handle items in a specific order and can be easily implemented using other data structures like lists or linked lists.
Related Article: How to Remove a Key from a Python Dictionary
Queue – FIFO Data Structure for First-In-First-Out Operations
A queue is another specialized data structure in Python that follows the First-In-First-Out (FIFO) principle. It is a collection of elements where the first element added is the first one to be removed. Queues are commonly used when you need to maintain the order of items and perform operations like enqueueing (adding) or dequeuing (removing) elements.
In Python, you can implement a queue using a list. However, since adding or removing elements from the beginning of a list is inefficient, it is recommended to use the collections.deque
class, which provides efficient operations for both ends of the queue.
Here is an example of creating a queue using collections.deque
and performing basic operations:
from collections import deque queue = deque() queue.append('apple') # Enqueues 'apple' at the end of the queue queue.append('banana') # Enqueues 'banana' at the end of the queue queue.append('orange') # Enqueues 'orange' at the end of the queue print(queue) # Output: deque(['apple', 'banana', 'orange']) front = queue.popleft() # Dequeues the front element from the queue print(front) # Output: apple print(queue) # Output: deque(['banana', 'orange'])
In this example, we use the append()
method to enqueue elements at the end of the queue and the popleft()
method to dequeue the front element. The first element added (‘apple’) is the first one to be removed.
Queues are widely used in various applications, such as process scheduling, event handling, breadth-first search, or implementing message queues. They provide a reliable way to manage items in a specific order and can be efficiently implemented using the collections.deque
class or other specialized data structures like linked lists.
Linked List – Dynamic Data Structure for Efficient Insertion and Deletion
A linked list is a dynamic data structure in Python that consists of a sequence of nodes, where each node contains a value and a reference to the next node. Linked lists are particularly useful when you need to efficiently insert or delete elements at arbitrary positions, as they provide constant-time operations for these operations.
In Python, you can implement a linked list by defining a Node
class and a LinkedList
class that manages the nodes. Here is an example of creating a linked list and performing basic operations:
class Node: def __init__(self, value): self.value = value self.next = None class LinkedList: def __init__(self): self.head = None def insert(self, value): new_node = Node(value) if self.head is None: self.head = new_node else: current = self.head while current.next: current = current.next current.next = new_node def delete(self, value): if self.head is None: return if self.head.value == value: self.head = self.head.next return current = self.head while current.next: if current.next.value == value: current.next = current.next.next return current = current.next def display(self): current = self.head while current: print(current.value, end=' ') current = current.next print() linked_list = LinkedList() linked_list.insert('apple') linked_list.insert('banana') linked_list.insert('orange') linked_list.display() # Output: apple banana orange linked_list.delete('banana') linked_list.display() # Output: apple orange
In this example, we define a Node
class that represents a single node in the linked list, and a LinkedList
class that manages the nodes. The insert()
method inserts a new node at the end of the list, the delete()
method removes a node with a specific value, and the display()
method prints the values of all nodes in the list.
Linked lists are commonly used in various scenarios, such as implementing stacks, queues, or hash tables, or when you need efficient insertion and deletion of elements at arbitrary positions. They provide a flexible and dynamic way to manage data and are particularly useful in situations where the size of the data changes frequently.
Heap – Efficiently Maintaining Priority Queues
A heap is a specialized tree-based data structure in Python that allows you to efficiently maintain a priority queue. A priority queue is a collection of elements where each element is assigned a priority and the element with the highest priority is always the first one to be removed. Heaps provide efficient operations for adding elements, removing the highest-priority element, and updating the priorities.
In Python, you can implement a heap using the heapq
module, which provides functions for heap operations. Here is an example of creating a heap and performing basic operations:
import heapq heap = [] heapq.heappush(heap, 5) # Adds 5 to the heap heapq.heappush(heap, 3) # Adds 3 to the heap heapq.heappush(heap, 7) # Adds 7 to the heap print(heap) # Output: [3, 5, 7] highest_priority = heapq.heappop(heap) # Removes and returns the highest-priority element print(highest_priority) # Output: 3 print(heap) # Output: [5, 7]
In this example, we use the heappush()
function to add elements to the heap and the heappop()
function to remove the highest-priority element. The element with the lowest value is considered to have the highest priority.
Heaps are commonly used in various applications, such as sorting algorithms (e.g., heapsort), graph algorithms (e.g., Dijkstra’s algorithm), or scheduling tasks based on priorities. They provide an efficient way to manage elements with priorities and can be easily implemented using the heapq
module or other specialized data structures like binary heaps.
Related Article: How to Remove an Element from a List by Index in Python
Graph – Modeling Relationships and Interconnections
A graph is a versatile data structure in Python that allows you to model relationships and interconnections between objects. It consists of a set of vertices (nodes) and a set of edges that connect the vertices. Graphs are widely used in various applications, such as social networks, routing algorithms, or data analysis.
In Python, you can implement a graph using various approaches, such as an adjacency matrix or an adjacency list. Here is an example of creating a graph using an adjacency list and performing basic operations:
class Graph: def __init__(self): self.vertices = {} def add_vertex(self, vertex): self.vertices[vertex] = [] def add_edge(self, source, destination): if source in self.vertices and destination in self.vertices: self.vertices[source].append(destination) self.vertices[destination].append(source) def display(self): for vertex, neighbors in self.vertices.items(): print(f"{vertex}: {neighbors}") graph = Graph() graph.add_vertex('A') graph.add_vertex('B') graph.add_vertex('C') graph.add_vertex('D') graph.add_edge('A', 'B') graph.add_edge('B', 'C') graph.add_edge('C', 'D') graph.display()
In this example, we define a Graph
class that represents a graph using an adjacency list. The add_vertex()
method adds a new vertex to the graph, the add_edge()
method adds a new edge between two vertices, and the display()
method prints the vertices and their neighbors.
Graphs provide a useful way to model and analyze relationships between objects. They are commonly used in various domains, such as social networks, network routing, recommendation systems, or data visualization. Graph algorithms can be applied to solve complex problems and provide valuable insights into the structure and behavior of interconnected data.
Choosing the Right Python Data Structure
When working on a Python project, it is crucial to choose the right data structure based on the requirements and constraints of the problem at hand. Each data structure has its own strengths and weaknesses, and understanding their characteristics can help you make informed decisions.
Here are some factors to consider when choosing a Python data structure:
– Data Type: Determine the type of data you need to store. For example, if you need to store a collection of elements with no particular order, a set might be a good choice. If you need to store key-value pairs, a dictionary would be more suitable.
– Access and Manipulation: Assess the operations you will perform on the data structure. If you need to frequently access or modify elements at arbitrary positions, a linked list or an array might be more efficient than a list.
– Ordering: Consider whether the order of elements is important. If you need to maintain the order, a list or a tuple would be a good choice. If the order is not important or duplicates should be eliminated, a set or a dictionary might be more appropriate.
– Memory and Performance: Evaluate the memory usage and performance requirements of your application. For large collections of homogeneous data, an array can provide better memory efficiency and faster access than a list. For efficient insertion and deletion of elements, a linked list can outperform other data structures.
– Complexity: Analyze the time and space complexity of the operations you will perform. Some data structures, like dictionaries or sets, provide constant-time operations for common operations like membership checking or element insertion. Others, like arrays or linked lists, have linear-time complexity for certain operations.
Efficient Data Structures for Large Amounts of Data
When working with large amounts of data in Python, it is important to choose data structures that can efficiently handle the size and complexity of the data. Using the right data structures can significantly improve the performance and memory usage of your code.
Here are some efficient data structures for managing large amounts of data in Python:
– Arrays: Arrays are efficient for storing large collections of homogeneous data, such as numerical values or large strings. They provide direct access to individual elements based on their index and have a small memory overhead compared to lists.
– Dictionaries: Dictionaries are efficient for storing and retrieving data based on unique keys. They offer constant-time operations for common operations like element lookup, insertion, or deletion. Dictionaries are particularly useful when you need to handle large datasets or perform fast key-based lookups.
– Sets: Sets are efficient for handling large collections of unique elements. They provide constant-time operations for membership checking, insertion, and deletion. Sets are useful for tasks like removing duplicates from a sequence, counting unique items, or filtering out irrelevant data.
– Linked Lists: Linked lists are efficient for handling large datasets that require frequent insertion or deletion of elements at arbitrary positions. They provide constant-time operations for these operations, as they only require updating a few references. Linked lists are particularly useful when the size of the data changes frequently.
– Heaps: Heaps are efficient for maintaining priority queues, especially when you need to handle large amounts of data with varying priorities. They offer logarithmic-time operations for adding elements, removing the highest-priority element, or updating priorities. Heaps are commonly used in scenarios like sorting algorithms, task scheduling, or event handling.
Related Article: How to Solve a Key Error in Python
List vs Tuple – When to Use Each
Both lists and tuples are commonly used data structures in Python, but they have different characteristics and use cases. Understanding the differences between lists and tuples can help you choose the most appropriate data structure for your needs.
Here are some factors to consider when deciding between a list and a tuple:
– Mutability: Lists are mutable, which means that you can modify their elements after they are created. Tuples, on the other hand, are immutable, which means that their elements cannot be modified once they are assigned. If you need to modify the elements of a collection, use a list. If you want to ensure that the data remains unchanged, use a tuple.
– Ordering: Lists preserve the order of elements, while tuples also preserve the order but provide additional guarantees. Since tuples are immutable, they guarantee that the order of elements remains the same throughout the program. If the order of elements is important and should not change, use a tuple. If the order can change or elements can be modified, use a list.
– Use Cases: Lists are commonly used for dynamic collections of elements that can be modified, such as managing collections of items, implementing stacks or queues, or representing matrices and grids. Tuples are often used for fixed structures or sequences, such as coordinates, RGB colors, or database records. Tuples are also useful when you want to ensure that the data remains unchanged after it is assigned.
– Performance: Lists and tuples have similar performance characteristics, but tuples are generally slightly faster and have a smaller memory footprint than lists. If performance is critical or memory usage is a concern, consider using tuples instead of lists.
Set vs Dictionary – Understanding the Differences
Sets and dictionaries are both useful data structures in Python, but they have different characteristics and use cases. Understanding the differences between sets and dictionaries can help you choose the most appropriate data structure for your needs.
Here are some key differences between sets and dictionaries:
– Keys: Dictionaries store values with corresponding keys, while sets store only values. Each element in a dictionary is associated with a unique key, which allows for efficient lookup and retrieval. Sets, on the other hand, store only unique values and do not require keys.
– Uniqueness: Dictionaries can contain duplicate values, as long as the keys are unique. Sets, on the other hand, only store unique values and automatically eliminate duplicates. If you need to store a collection of unique values or remove duplicates from a sequence, use a set.
– Ordering: Dictionaries do not preserve the order of elements, as they are based on key-value pairs. Sets also do not preserve the order of elements, as they are designed for efficient membership checking and do not rely on indexing. If the order of elements is important, use a list or a tuple instead.
– Performance: Dictionaries provide efficient lookup and retrieval of values based on keys, thanks to their underlying hash table implementation. Sets also provide efficient membership checking, insertion, and deletion of values, thanks to their hash set implementation. Both dictionaries and sets have similar performance characteristics and are often faster than lists for certain operations.
– Use Cases: Dictionaries are commonly used when you need to associate values with unique keys or perform efficient lookups based on keys. They are useful for tasks like storing user information, configuring settings, or maintaining a mapping between two sets of values. Sets are commonly used when you need to store a collection of unique values or perform operations like membership checking, intersection, or difference.
Implementing a Stack in Python
A stack is a commonly used data structure that follows the Last-In-First-Out (LIFO) principle. It is a collection of elements where the last element added is the first one to be removed. Stacks can be easily implemented in Python using lists.
Here is an example of implementing a stack in Python:
class Stack: def __init__(self): self.stack = [] def is_empty(self): return len(self.stack) == 0 def push(self, item): self.stack.append(item) def pop(self): if self.is_empty(): return None return self.stack.pop() def peek(self): if self.is_empty(): return None return self.stack[-1] def size(self): return len(self.stack)
In this example, we define a Stack
class that uses a list to store the elements. The is_empty()
method checks if the stack is empty, the push()
method adds an item to the stack, the pop()
method removes and returns the top item, the peek()
method returns the top item without removing it, and the size()
method returns the size of the stack.
Here is an example of using the Stack
class:
stack = Stack() stack.push('apple') stack.push('banana') stack.push('orange') print(stack.peek()) # Output: orange print(stack.pop()) # Output: orange print(stack.size()) # Output: 2
In this example, we create a Stack
object, push three items onto the stack, and perform various operations like peeking, popping, and getting the size of the stack.
Stacks are widely used in various applications, such as expression evaluation, backtracking algorithms, depth-first search, or managing function calls in programming languages. They provide a simple and intuitive way to handle items in a specific order and can be easily implemented using other data structures like lists or linked lists.
Related Article: How to Check If Something Is Not In A Python List
The Purpose of a Queue Data Structure in Python
A queue is a commonly used data structure that follows the First-In-First-Out (FIFO) principle. It is a collection of elements where the first element added is the first one to be removed. Queues can be easily implemented in Python using lists or the collections.deque
class.
The purpose of a queue data structure in Python is to maintain the order of items and perform operations like enqueueing (adding) or dequeuing (removing) elements. Queues are commonly used in scenarios where you need to process items in the same order they were added or handle tasks based on their arrival time.
Here is an example of implementing a queue in Python using the collections.deque
class:
from collections import deque class Queue: def __init__(self): self.queue = deque() def is_empty(self): return len(self.queue) == 0 def enqueue(self, item): self.queue.append(item) def dequeue(self): if self.is_empty(): return None return self.queue.popleft() def peek(self): if self.is_empty(): return None return self.queue[0] def size(self): return len(self.queue)
In this example, we define a Queue
class that uses a collections.deque
object to store the elements. The is_empty()
method checks if the queue is empty, the enqueue()
method adds an item to the end of the queue, the dequeue()
method removes and returns the front item, the peek()
method returns the front item without removing it, and the size()
method returns the size of the queue.
Here is an example of using the Queue
class:
queue = Queue() queue.enqueue('apple') queue.enqueue('banana') queue.enqueue('orange') print(queue.peek()) # Output: apple print(queue.dequeue()) # Output: apple print(queue.size()) # Output: 2
In this example, we create a Queue
object, enqueue three items into the queue, and perform various operations like peeking, dequeuing, and getting the size of the queue.
Queues are widely used in various applications, such as process scheduling, event handling, breadth-first search, or implementing message queues. They provide a reliable way to manage items in a specific order and can be efficiently implemented using lists or the collections.deque
class.
Implementing a Linked List in Python
A linked list is a dynamic data structure that consists of a sequence of nodes, where each node contains a value and a reference to the next node. Linked lists can be easily implemented in Python using classes.
Here is an example of implementing a singly linked list in Python:
class Node: def __init__(self, value): self.value = value self.next = None class LinkedList: def __init__(self): self.head = None def is_empty(self): return self.head is None def insert_at_beginning(self, value): new_node = Node(value) new_node.next = self.head self.head = new_node def insert_at_end(self, value): new_node = Node(value) if self.is_empty(): self.head = new_node else: current = self.head while current.next: current = current.next current.next = new_node def delete(self, value): if self.is_empty(): return if self.head.value == value: self.head = self.head.next return current = self.head while current.next: if current.next.value == value: current.next = current.next.next return current = current.next def display(self): current = self.head while current: print(current.value, end=' ') current = current.next print()
In this example, we define a Node
class that represents a single node in the linked list and a LinkedList
class that manages the nodes. The is_empty()
method checks if the list is empty, the insert_at_beginning()
method inserts a new node at the beginning of the list, the insert_at_end()
method inserts a new node at the end of the list, the delete()
method removes a node with a specific value, and the display()
method prints the values of all nodes in the list.
Here is an example of using the LinkedList
class:
linked_list = LinkedList() linked_list.insert_at_beginning('apple') linked_list.insert_at_end('banana') linked_list.insert_at_end('orange') linked_list.display() # Output: apple banana orange linked_list.delete('banana') linked_list.display() # Output: apple orange
In this example, we create a LinkedList
object, insert three nodes into the list, and perform various operations like deleting nodes and displaying the values of all nodes.
Linked lists are widely used in various scenarios, such as implementing stacks, queues, or hash tables, or when you need efficient insertion and deletion of elements at arbitrary positions. They provide a flexible and dynamic way to manage data and are particularly useful in situations where the size of the data changes frequently.
Understanding the Heap Data Structure in Python
A heap is a specialized tree-based data structure that allows you to efficiently maintain a priority queue. A priority queue is a collection of elements where each element is assigned a priority, and the element with the highest priority is always the first one to be removed. Heaps provide efficient operations for adding elements, removing the highest-priority element, and updating the priorities.
In Python, you can implement a heap using the heapq
module, which provides functions for heap operations. The heapq
module uses a binary heap, which is a complete binary tree that satisfies the heap property: for every node, the value of the parent is greater (or smaller) than the values of its children.
Here is an example of using the heapq
module to create and manipulate a heap in Python:
import heapq heap = [] heapq.heappush(heap, 5) # Adds 5 to the heap heapq.heappush(heap, 3) # Adds 3 to the heap heapq.heappush(heap, 7) # Adds 7 to the heap print(heap) # Output: [3, 5, 7] highest_priority = heapq.heappop(heap) # Removes and returns the highest-priority element print(highest_priority) # Output: 3 print(heap) # Output: [5, 7]
In this example, we use the heapq.heappush()
function to add elements to the heap and the heapq.heappop()
function to remove the highest-priority element. The element with the lowest value is considered to have the highest priority.
Heaps are commonly used in various applications, such as sorting algorithms (e.g., heapsort), graph algorithms (e.g., Dijkstra’s algorithm), or scheduling tasks based on priorities. They provide an efficient way to manage elements with priorities and can be easily implemented using the heapq
module or other specialized data structures like binary heaps.
Related Article: How to Read a File Line by Line into a List in Python
The Role of Graphs in Python Data Structures
A graph is a versatile data structure that allows you to model relationships and interconnections between objects. It consists of a set of vertices (nodes) and a set of edges that connect the vertices. Graphs are widely used in various applications, such as social networks, routing algorithms, or data analysis.
In Python, you can implement a graph using various approaches, such as an adjacency matrix or an adjacency list. The choice of implementation depends on the characteristics of the graph and the operations you need to perform.
Here is an example of using an adjacency list to represent a graph in Python:
class Graph: def __init__(self): self.vertices = {} def add_vertex(self, vertex): self.vertices[vertex] = [] def add_edge(self, source, destination): if source in self.vertices and destination in self.vertices: self.vertices[source].append(destination) self.vertices[destination].append(source) def display(self): for vertex, neighbors in self.vertices.items(): print(f"{vertex}: {neighbors}") graph = Graph() graph.add_vertex('A') graph.add_vertex('B') graph.add_vertex('C') graph.add_vertex('D') graph.add_edge('A', 'B') graph.add_edge('B', 'C') graph.add_edge('C', 'D') graph.display()
In this example, we define a Graph
class that represents a graph using an adjacency list. The add_vertex()
method adds a new vertex to the graph, the add_edge()
method adds a new edge between two vertices, and the display()
method prints the vertices and their neighbors.
Here is the output of the display()
method:
A: ['B'] B: ['A', 'C'] C: ['B', 'D'] D: ['C']
Graphs provide a useful way to model and analyze relationships between objects. They are commonly used in various domains, such as social networks, network routing, recommendation systems, or data visualization. Graph algorithms can be applied to solve complex problems and provide valuable insights into the structure and behavior of interconnected data.