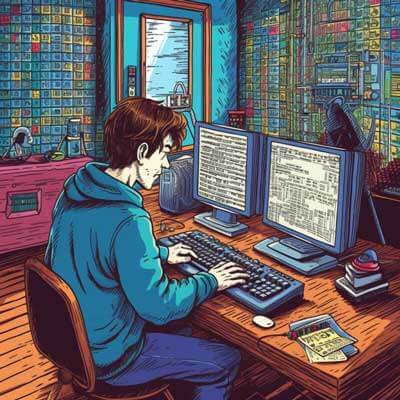
Table of Contents
Introduction to Pandas Dataframe Apply
The Pandas library is a powerful tool for data manipulation and analysis in Python. One of its most versatile functions is the apply
method, which allows you to apply a function along an axis of a DataFrame. This article will explore various examples of using apply
with Pandas DataFrame.
Related Article: How To Reset Index In A Pandas Dataframe
Dataframe Apply: Its Purpose and Role
The apply
function in Pandas DataFrame allows you to apply a function to each row or column of the DataFrame. It is particularly useful when you want to perform some operation on the entire dataset or a specific subset of the data. The apply
function helps simplify complex data transformations, aggregations, and conditional operations.
Conceptual Analysis of Dataframe Apply
When you use the apply
function, you are essentially iterating over the rows or columns of the DataFrame and applying a specified function to each element. This can be done along either axis: row-wise (axis=0) or column-wise (axis=1). The function applied can be a built-in Python function, a user-defined function, or a lambda function.
Setting Up the Coding Environment
Before diving into the examples, let's set up our coding environment. Make sure you have Python installed on your system along with the Pandas library. To install Pandas, you can use pip:
pip install pandas
Once you have Pandas installed, you can import it into your Python script or Jupyter Notebook:
import pandas as pd
Related Article: Tutorial: Django + MongoDB, ElasticSearch & Message Brokers
First Steps: Basic Use of Apply
To get started with apply
, let's first understand the basic syntax. The apply
function can be called on a DataFrame and takes a function as an argument. This function will be applied to each element of the DataFrame. Let's consider a simple example:
import pandas as pd # Create a DataFrame data = {'Name': ['John', 'Emily', 'Michael'], 'Age': [25, 30, 35]} df = pd.DataFrame(data) # Define a function to add a prefix to each name def add_prefix(name): return 'Mr. ' + name # Apply the function to the 'Name' column df['Name'] = df['Name'].apply(add_prefix) print(df)
Output:
Name Age 0 Mr. John 25 1 Mr. Emily 30 2 Mr. Michael 35
In this example, we create a DataFrame with two columns: 'Name' and 'Age'. We define a function add_prefix
that adds the prefix "Mr." to a given name. We then use apply
to apply this function to each element in the 'Name' column. As a result, each name in the 'Name' column is prefixed with "Mr.".
Use Case 1: Data Transformation with Apply
One common use case of apply
is data transformation. You can apply a function to each element in a column to transform the data in a desired way. Let's consider an example where we want to convert the values in a column to uppercase:
import pandas as pd # Create a DataFrame data = {'Name': ['John', 'Emily', 'Michael']} df = pd.DataFrame(data) # Define a function to convert a string to uppercase def convert_to_uppercase(name): return name.upper() # Apply the function to the 'Name' column df['Name'] = df['Name'].apply(convert_to_uppercase) print(df)
Output:
Name 0 JOHN 1 EMILY 2 MICHAEL
In this example, we define a function convert_to_uppercase
that converts a given string to uppercase using the upper()
method. We then use apply
to apply this function to each element in the 'Name' column, effectively converting all names to uppercase.
Use Case 2: Aggregation with Apply
Another powerful use of apply
is for aggregating data. You can apply a function to a column or row and obtain a single value as the result. Let's consider an example where we want to calculate the average age from a column:
import pandas as pd # Create a DataFrame data = {'Name': ['John', 'Emily', 'Michael'], 'Age': [25, 30, 35]} df = pd.DataFrame(data) # Define a function to calculate the average age def calculate_average_age(age_column): return age_column.mean() # Apply the function to the 'Age' column average_age = df['Age'].apply(calculate_average_age) print("Average Age:", average_age)
Output:
Average Age: 30.0
In this example, we define a function calculate_average_age
that takes an age column and calculates the mean value using the mean()
method. We then use apply
to apply this function to the 'Age' column, resulting in the average age being calculated and stored in the average_age
variable.
Use Case 3: Conditional Operations with Apply
You can also use apply
to perform conditional operations on your data. Let's consider an example where we want to categorize people based on their age:
import pandas as pd # Create a DataFrame data = {'Name': ['John', 'Emily', 'Michael'], 'Age': [25, 30, 35]} df = pd.DataFrame(data) # Define a function to categorize age groups def categorize_age(age): if age < 30: return 'Young' else: return 'Adult' # Apply the function to the 'Age' column df['Age Category'] = df['Age'].apply(categorize_age) print(df)
Output:
Name Age Age Category 0 John 25 Young 1 Emily 30 Adult 2 Michael 35 Adult
In this example, we define a function categorize_age
that checks if the age is less than 30. If it is, it returns 'Young'; otherwise, it returns 'Adult'. We then use apply
to apply this function to each element in the 'Age' column, resulting in a new column called 'Age Category' that categorizes each person based on their age.
Related Article: How to Create Multiline Comments in Python
Best Practice 1: Efficient Use of Apply
When using apply
, it is important to consider efficiency. Applying a function element-wise can be slower compared to vectorized operations. To improve efficiency, you can use built-in Pandas functions that are optimized for performance. Let's consider an example where we want to calculate the length of each name in a column:
import pandas as pd # Create a DataFrame data = {'Name': ['John', 'Emily', 'Michael']} df = pd.DataFrame(data) # Calculate the length of each name df['Name Length'] = df['Name'].str.len() print(df)
Output:
Name Name Length 0 John 4 1 Emily 5 2 Michael 7
In this example, instead of using apply
to apply a custom function to calculate the length of each name, we use the built-in str.len()
function of Pandas. This function returns the length of each string in the 'Name' column, resulting in a new column called 'Name Length' with the length of each name.
Best Practice 2: Avoiding Common Mistakes with Apply
When using apply
, there are some common mistakes to avoid. One mistake is forgetting to assign the result of apply
back to the DataFrame. Let's consider an example where we want to remove the prefix "Mr." from each name:
import pandas as pd # Create a DataFrame data = {'Name': ['Mr. John', 'Mr. Emily', 'Mr. Michael']} df = pd.DataFrame(data) # Define a function to remove the prefix def remove_prefix(name): return name.replace('Mr. ', '') # Apply the function to the 'Name' column (Mistake: Missing assignment) df['Name'].apply(remove_prefix) print(df)
Output:
Name 0 Mr. John 1 Mr. Emily 2 Mr. Michael
In this example, we define a function remove_prefix
that uses the replace()
method to remove the prefix "Mr." from a given name. However, we forget to assign the result of apply
back to the 'Name' column, resulting in no changes to the DataFrame. To fix this, we need to assign the result back to the column:
df['Name'] = df['Name'].apply(remove_prefix)
Real World Example 1: Financial Analysis with Apply
To demonstrate the practical use of apply
, let's consider a real-world example of financial analysis. Suppose we have a DataFrame with stock prices for different companies over a period of time. We want to calculate the total return for each stock, given the initial and final prices. Here's an example:
import pandas as pd # Create a DataFrame with stock prices data = {'Company': ['AAPL', 'GOOG', 'MSFT'], 'Initial Price': [100, 200, 150], 'Final Price': [120, 230, 160]} df = pd.DataFrame(data) # Define a function to calculate the total return def calculate_total_return(initial_price, final_price): return ((final_price - initial_price) / initial_price) * 100 # Apply the function to the 'Initial Price' and 'Final Price' columns df['Total Return'] = df.apply(lambda row: calculate_total_return(row['Initial Price'], row['Final Price']), axis=1) print(df)
Output:
Company Initial Price Final Price Total Return 0 AAPL 100 120 20.0 1 GOOG 200 230 15.0 2 MSFT 150 160 6.666667
In this example, we create a DataFrame with stock prices for three companies: AAPL, GOOG, and MSFT. We define a function calculate_total_return
that takes the initial and final prices as arguments and calculates the total return as a percentage. We then use apply
with a lambda function to apply this function to each row of the DataFrame, calculating the total return for each stock.
Real World Example 2: Data Cleaning with Apply
Another practical use of apply
is data cleaning. Let's consider an example where we have a DataFrame with a column containing messy strings that need to be cleaned. We want to remove any special characters and convert the strings to lowercase. Here's an example:
import pandas as pd # Create a DataFrame with messy strings data = {'Text': ['Hello!', 'How are you?', 'I am fine!']} df = pd.DataFrame(data) # Define a function to clean the strings def clean_string(text): cleaned_text = ''.join(e for e in text if e.isalnum()) return cleaned_text.lower() # Apply the function to the 'Text' column df['Cleaned Text'] = df['Text'].apply(clean_string) print(df)
Output:
Text Cleaned Text 0 Hello! hello 1 How are you? howareyou 2 I am fine! iamfine
In this example, we create a DataFrame with three messy strings in the 'Text' column. We define a function clean_string
that uses a combination of the isalnum()
and lower()
methods to remove special characters and convert the strings to lowercase. We then use apply
to apply this function to each element in the 'Text' column, resulting in a new column called 'Cleaned Text' with the cleaned strings.
Related Article: How to Normalize a Numpy Array to a Unit Vector in Python
Performance Consideration 1: Apply vs. Vectorized Operations
While apply
is a powerful tool, it may not always be the most efficient option for certain operations. In general, vectorized operations provided by Pandas or NumPy tend to be faster than applying a function element-wise using apply
. Vectorized operations are optimized for performance and take advantage of underlying C or Fortran implementations. It is recommended to use vectorized operations whenever possible to improve execution speed.
Performance Consideration 2: Improving Speed with Apply
If you find that apply
is necessary for your specific use case, there are a few techniques you can employ to improve its speed. One technique is to use the numba
library, which provides just-in-time (JIT) compilation for Python functions. JIT compilation can significantly speed up the execution of apply
by converting the Python code to machine code at runtime. Another technique is to parallelize the apply
operation using the dask
library, which allows for distributed computing and can leverage multiple CPU cores to process the data in parallel.
Advanced Technique 1: Using Applymap and Apply with Difference
In addition to apply
, Pandas provides two other similar functions: applymap
and map
. While apply
operates on a DataFrame or Series, applymap
works element-wise on a DataFrame, and map
works element-wise on a Series. Here's an example of using applymap
and apply
with the difference
function:
import pandas as pd # Create a DataFrame data = {'A': [1, 2, 3], 'B': [4, 5, 6]} df = pd.DataFrame(data) # Apply the 'difference' function element-wise using applymap df_difference_applymap = df.applymap(lambda x: x - 1) # Apply the 'difference' function element-wise using apply df_difference_apply = df.apply(lambda x: x.apply(lambda y: y - 1)) print("Applymap:") print(df_difference_applymap) print("Apply:") print(df_difference_apply)
Output:
Applymap: A B 0 0 3 1 1 4 2 2 5 Apply: A B 0 0 3 1 1 4 2 2 5
In this example, we create a DataFrame with two columns: 'A' and 'B'. We use applymap
to apply a lambda function that subtracts 1 from each element of the DataFrame. We also use apply
with a nested lambda function to achieve the same result. Both methods produce the same output.
Advanced Technique 2: Apply with Lambda Functions
Lambda functions can be particularly useful when working with apply
. They allow you to define a function inline without the need for a separate function definition. Here's an example:
import pandas as pd # Create a DataFrame data = {'Name': ['John Doe', 'Jane Smith', 'Michael Johnson']} df = pd.DataFrame(data) # Apply a lambda function to extract the last name df['Last Name'] = df['Name'].apply(lambda name: name.split()[-1]) print(df)
Output:
Name Last Name 0 John Doe Doe 1 Jane Smith Smith 2 Michael Johnson Johnson
In this example, we create a DataFrame with a 'Name' column. We use apply
with a lambda function to extract the last name from each full name by splitting the string and selecting the last element. The result is stored in a new column called 'Last Name'.
Related Article: How to Export a Python Data Frame to SQL Files
Code Snippet 1: Basic Use of Apply
import pandas as pd # Create a DataFrame data = {'Name': ['John', 'Emily', 'Michael'], 'Age': [25, 30, 35]} df = pd.DataFrame(data) # Define a function to add a prefix to each name def add_prefix(name): return 'Mr. ' + name # Apply the function to the 'Name' column df['Name'] = df['Name'].apply(add_prefix) print(df)
Code Snippet 2: Apply with Aggregation
import pandas as pd # Create a DataFrame data = {'Name': ['John', 'Emily', 'Michael'], 'Age': [25, 30, 35]} df = pd.DataFrame(data) # Define a function to calculate the average age def calculate_average_age(age_column): return age_column.mean() # Apply the function to the 'Age' column average_age = df['Age'].apply(calculate_average_age) print("Average Age:", average_age)
Code Snippet 3: Apply with Conditional Operations
import pandas as pd # Create a DataFrame data = {'Name': ['John', 'Emily', 'Michael'], 'Age': [25, 30, 35]} df = pd.DataFrame(data) # Define a function to categorize age groups def categorize_age(age): if age < 30: return 'Young' else: return 'Adult' # Apply the function to the 'Age' column df['Age Category'] = df['Age'].apply(categorize_age) print(df)
Code Snippet 4: Apply with Lambda Functions
import pandas as pd # Create a DataFrame data = {'Name': ['John', 'Emily', 'Michael']} df = pd.DataFrame(data) # Apply a lambda function to convert each name to uppercase df['Name'] = df['Name'].apply(lambda name: name.upper()) print(df)
Related Article: How to Remove a Key from a Python Dictionary
Code Snippet 5: Use of Applymap
import pandas as pd # Create a DataFrame data = {'A': [1, 2, 3], 'B': [4, 5, 6]} df = pd.DataFrame(data) # Apply a lambda function element-wise using applymap df = df.applymap(lambda x: x - 1) print(df)
Error Handling: Common Errors and Solutions
When using apply
, you may encounter some common errors. One common error is when the function you apply expects a different number of arguments than what is provided. Make sure the function you apply matches the expected number of arguments for each element. Another common error is when the function you apply is not compatible with the data type of the elements in the column. Ensure that your function can handle the data types present in the column. Additionally, be mindful of null or missing values in your data, as they can cause errors when applying functions. Use appropriate methods such as fillna()
or conditional statements to handle missing values before applying functions.
These are just a few common errors you may encounter when using apply
. Always review any error messages and consult the documentation or community resources for further assistance in resolving specific issues you encounter.