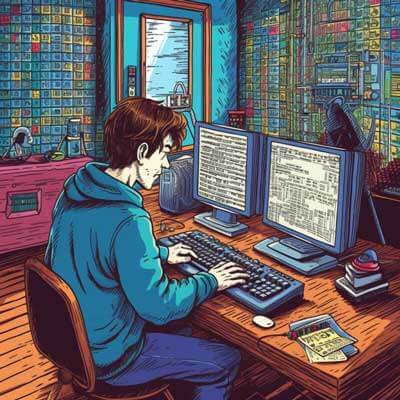
Table of Contents
Intro
In this tutorial, you will learn how to compare strings in Python, covering built-in string comparison methods, advanced comparison techniques, and tips for optimizing performance.
Related Article: How to Use Switch Statements in Python
A List of Techniques and Features for String Comparison in Python
Method | Description |
---|---|
Equality operator (==) | This compares two strings for exact match, the easiest way to see if two strings are equivalent, including case sensitivity. |
Inequality operator (!=) | This checks whether the two strings are not equal, and can be used to compare strings for inequality. |
str.lower() | This converts both strings to lowercase using the lower() method and then compares them using the equality operator (==). This allows for case-insensitive comparison. |
str.upper() | This converts both strings to uppercase using the upper() method and then compares them using the equality operator (==). This also allows for case-insensitive comparison. |
str.startswith() | This checks if one string starts with another string by using the startswith() method. It takes a substring as an argument and returns True if the original string starts with that substring, and False otherwise. |
str.endswith() | This checks if one string ends with another string by using the endswith() method. It takes a substring as an argument and returns True if the original string ends with that substring, and False otherwise. |
The "in" keyword | This checks if one string is a substring of another string by using the in keyword. It returns True if the first string is found within the second string, and False otherwise. |
str.find() | This searches for a substring in a string using the find() method. It returns the index of the first occurrence of the substring in the string, or -1 if the substring is not found. |
str.index() | This is similar to the find() method, but raises a ValueError if the substring is not found in the string instead of returning -1. |
Using regular expressions | Python's built-in re module provides powerful regular expression functionality to compare and manipulate strings based on complex patterns. |
Using external libraries | There are external libraries like difflib, fuzzywuzzy, and python-Levenshtein that provide advanced string comparison and fuzzy matching capabilities. |
Using custom comparison logic | You can implement your own custom comparison logic based on specific requirements, such as implementing algorithms like Levenshtein distance, Jaro-Winkler distance, or other string matching algorithms. |
Note: The choice of method for comparing strings in Python depends on the specific use case and requirements of your application. It's important to understand the differences and limitations of each method and choose the one that best fits your needs.
Code Examples
Here are practical examples of how string comparison operators work, using Python:
Equality (==)
The equality operator compares two strings for exact match, checking if two strings are equal, including case sensitivity. For example:
str1 = "hello" str2 = "Hello" print(str1 == str2) # False
Related Article: How to Manipulate Strings in Python and Check for Substrings
Inequality (!=)
The inequality operator compares if two strings are not equal, and can be used to compare strings for inequality. For example:
str1 = "hello" str2 = "world" print(str1 != str2) # True
Case-insensitive comparison
You can use string methods like str.lower()
or str.upper()
to convert both strings to lowercase or uppercase, respectively, and then compare them using the equality or inequality operators. For example:
str1 = "Hello" str2 = "hello" print(str1.lower() == str2.lower()) # True
Startswith (str.startswith()
)
This method checks if one string starts with another string. It takes a substring as an argument and returns True if the original string starts with that substring, and False otherwise. For example:
str1 = "Hello, world" str2 = "Hello" print(str1.startswith(str2)) # True
Endswith (str.endswith()
)
This method checks if one string ends with another string. It takes a substring as an argument and returns True if the original string ends with that substring, and False otherwise. For example:
str1 = "Hello, world" str2 = "world" print(str1.endswith(str2)) # True
Related Article: How to Match a Space in Regex Using Python
Substring check (in
keyword)
You can use the in
keyword to check if one string is a substring of another string. It returns True if the first string is found within the second string, and False otherwise. For example:
str1 = "Hello, world" str2 = "world" print(str2 in str1) # True
String search (str.find() and str.index())
These methods allow you to search for a substring in a string. The str.find()
method returns the index of the first occurrence of the substring in the string, or -1
if the substring is not found. The str.index()
method is similar, but raises a ValueError
if the substring is not found. For example:
str1 = "Hello, world" str2 = "world" print(str1.find(str2)) # 7 print(str1.index(str2)) # 7
Regular expressions
Python's built-in re module provides powerful regular expression functionality to compare and manipulate strings based on complex patterns. Regular expressions can be used for advanced string comparisons and pattern matching.
External libraries
There are external libraries like difflib, fuzzywuzzy, and python-Levenshtein that provide advanced string comparison and fuzzy matching capabilities, which can be useful for more complex string comparison tasks.
Related Article: How to Use Pandas Groupby for Group Statistics in Python
Custom comparison logic
In some cases, you may need to implement your own custom comparison logic based on specific requirements, such as implementing algorithms like Levenshtein distance, Jaro-Winkler distance, or other string matching algorithms.
Greater than comparison types
There are many python comparison operators, such as <, <=, >, >=, ==, and !=. These operators allow you to check if one string is greater than, less than, equal to, or not equal to another string.
Here's an example of how you can check if one string is greater than another in Python:
# Example of string comparison in Python # Define two strings string1 = "apple" string2 = "banana" # Compare the strings using the '>' operator if string1 > string2: print("string1 is greater than string2") else: print("string1 is not greater than string2")
In this example, the >
operator is used to compare string1 and string2 lexicographically, which means that the strings are compared character by character based on their Unicode values. If string1
is lexicographically greater than string2
, the condition in the if
statement will be True, and the corresponding message will be printed. Otherwise, the else
block will be executed.
Note that string comparison in Python is case-sensitive, which means that uppercase letters are considered greater than lowercase letters. If you want to perform case-insensitive string comparison, you can convert the strings to lowercase or uppercase using the lower()
or upper()
string methods before performing the comparison.
Unicode
You can also check if strings are equivalent using unicodedata:
# -*- coding: utf-8 -*- # String comparison using unicode in Python # Example strings with unicode characters string1 = "Café" string2 = "Cafe\u0301" # Method 1: Using the unicode normalization method import unicodedata # Normalize strings using NFKC normalization form normalized_string1 = unicodedata.normalize("NFKC", string1) normalized_string2 = unicodedata.normalize("NFKC", string2) # Compare normalized strings if normalized_string1 == normalized_string2: print("Method 1: Strings are equal") else: print("Method 1: Strings are not equal") # Method 2: Using the unicode collation method import locale # Set locale to a UTF-8 supported locale locale.setlocale(locale.LC_ALL, 'en_US.UTF-8') # Compare strings using unicode collation if locale.strcoll(string1, string2) == 0: print("Method 2: Strings are equal") else: print("Method 2: Strings are not equal")
In this example, we have two strings string1 and string2 that contain the word "Café", but string2 uses a different representation with a combining acute accent character (\u0301
). We then use two different methods to compare these strings in Python using unicode.
Method 1 uses the unicodedata module and the normalize()
function with the NFKC (Normalization Form KC) normalization form to normalize the strings before comparison. This method ensures that the strings are represented in a canonical form that considers compatibility, composition, and decomposition of unicode characters.
Method 2 uses the locale module to set the locale to a UTF-8 supported locale and then uses the strcoll()
function to compare the strings using unicode collation. This method takes into account the language-specific rules for string comparison, such as sorting and collation, based on the locale settings.
Common Use Cases
String comparisons are frequently used in various practical applications.
Related Article: How to Fix Indentation Errors in Python
Real-World Scenarios
1. User Input Validation: Compare user input against predefined values.
2. Search Operations: Check for substrings within larger strings.
# file: user_input_validation.py user_input = "yes" if user_input.lower() == "yes": print("User agreed") else: print("User disagreed") # file: search_operations.py text = "The quick brown fox jumps over the lazy dog" word = "fox" if word in text: print(f"'{word}' found in text") else: print(f"'{word}' not found in text")
Common Use Cases
1. Configuration Parsing: Compare and process configuration values.
2. Data Cleaning: Normalize and compare data from different sources.
# file: configuration_parsing.py config_value = "true" if config_value.lower() in ["true", "yes", "1"]: enable_feature = True else: enable_feature = False print("Feature enabled:", enable_feature) # file: data_cleaning.py data = ["Apple", "banana", "Cherry", "apple"] normalized_data = [item.lower() for item in data] print(normalized_data) # ['apple', 'banana', 'cherry', 'apple']
Secure String Comparisons
Security is paramount when comparing sensitive strings, such as passwords.
Preventing Timing Attacks
To prevent timing attacks, use constant-time comparison functions.
# file: secure_comparison.py import hmac def secure_compare(a, b): return hmac.compare_digest(a, b) password = "secure_password" user_input = "secure_password" print(secure_compare(password, user_input)) # True
Related Article: How To Convert a Dictionary To JSON In Python
Secure String Comparisons
1. Use Hashing: Hash strings before comparing to ensure security.
2. Avoid Leaking Information: Ensure comparison functions do not reveal details about the strings.
# file: hashing_example.py import hashlib def hash_string(s): return hashlib.sha256(s.encode()).hexdigest() password_hash = hash_string("secure_password") user_input_hash = hash_string("secure_password") print(secure_compare(password_hash, user_input_hash)) # True
Benchmarking String Comparison Methods
When comparing strings in Python, performance can vary significantly based on the method used. Here, we benchmark different string comparison methods to identify which ones are the most efficient.
# file: benchmarking_string_comparison.py import time def benchmark(method, a, b, iterations=100000): start = time.time() for _ in range(iterations): method(a, b) end = time.time() return end - start # Methods to compare def equality_comparison(a, b): return a == b def case_insensitive_comparison(a, b): return a.lower() == b.lower() def substring_check(a, b): return a in b # Test strings a = "Hello, World!" b = "hello, world!" # Benchmarking print("Equality comparison:", benchmark(equality_comparison, a, b)) print("Case insensitive comparison:", benchmark(case_insensitive_comparison, a, b)) print("Substring check:", benchmark(substring_check, a, b))
Optimizing for Speed
Optimizing string comparison for speed involves selecting the right method and minimizing overhead.
1. Use Equality Comparison (==
): For exact matches, the equality operator is the fastest.
2. Avoid Unnecessary Conversions: Minimize operations like .lower()
unless needed.
3. Leverage String Interning: Python interns short strings, making comparisons faster.
Example of using string interning:
# file: string_interning.py import sys a = sys.intern("Hello, World!") b = sys.intern("Hello, World!") print(a == b) # True print(a is b) # True, due to interning
Comparing Strings in Different Languages
Handling string comparison in different languages involves considering locale-specific rules.
Use locale-aware comparison functions for accurate results.
# file: locale_comparison.py import locale locale.setlocale(locale.LC_COLLATE, 'de_DE.UTF-8') a = "straße" b = "strasse" print(locale.strcoll(a, b)) # Locale-aware comparison
Related Article: How to Check for an Empty String in Python
Handling Locale-Specific Comparisons
Ensure the correct locale is set for accurate comparisons.
# file: locale_specific_comparison.py import locale def compare_strings(a, b, locale_name='en_US.UTF-8'): locale.setlocale(locale.LC_COLLATE, locale_name) return locale.strcoll(a, b) a = "café" b = "cafe" print(compare_strings(a, b, 'fr_FR.UTF-8')) # Locale-specific comparison
String Normalization
String normalization is important for ensuring consistent and accurate string comparisons, especially when dealing with characters that can be represented in multiple ways. The unicodedata module provides the functionality to normalize strings.
Pre-processing Techniques
Normalize strings to a standard form before comparing.
# file: string_normalization.py import unicodedata def normalize_string(s): return unicodedata.normalize('NFC', s) a = "café" b = "cafe\u0301" # 'e' + combining acute accent print(a == b) # False print(normalize_string(a) == normalize_string(b)) # True
Normalization Methods
1. NFC (Normalization Form C): Composes characters into a single code point.
2. NFD (Normalization Form D): Decomposes characters into multiple code points.
# file: normalization_methods.py import unicodedata a = "café" b = "cafe\u0301" # 'e' + combining acute accent print(unicodedata.normalize('NFC', a) == unicodedata.normalize('NFC', b)) # True print(unicodedata.normalize('NFD', a) == unicodedata.normalize('NFD', b)) # True
Related Article: How to Convert String to Bytes in Python 3
Phonetic Algorithms: Soundex and Metaphone
Phonetic algorithms are useful for comparing strings that sound similar but may be spelled differently. Two popular phonetic algorithms are Soundex and Metaphone.
Soundex Algorithm
The Soundex algorithm encodes strings into a phonetic representation based on their pronunciation. It was originally developed for English words but can be adapted for other languages.
# file: soundex.py def soundex(name): soundex_code = "" codes = {"BFPV": "1", "CGJKQSXZ": "2", "DT": "3", "L": "4", "MN": "5", "R": "6"} name = name.upper() # Retain the first letter soundex_code += name[0] # Replace consonants with digits for char in name[1:]: for key in codes: if char in key: code = codes[key] if code != soundex_code[-1]: # Avoid duplicate codes soundex_code += code # Remove vowels, H, W, Y and append zeros to make the length 4 soundex_code = soundex_code.replace("A", "").replace("E", "").replace("I", "").replace("O", "").replace("U", "").replace("H", "").replace("W", "").replace("Y", "") soundex_code = (soundex_code + "000")[:4] return soundex_code print(soundex("Robert")) # R163 print(soundex("Rupert")) # R163 print(soundex("Rubin")) # R150
Metaphone Algorithm
The Metaphone algorithm improves upon Soundex by providing more accurate phonetic encoding. It is more complex and handles more variations in pronunciation.
# file: metaphone.py import metaphone as mp def metaphone_encoding(name): return mp.doublemetaphone(name) print(metaphone_encoding("Robert")) # ('RBRT', '') print(metaphone_encoding("Rupert")) # ('RPRT', '') print(metaphone_encoding("Rubin")) # ('RPN', 'RBN')
The use of phonetic algorithms can be particularly useful in applications such as searching and matching names in databases where spelling variations may exist.
Advanced Comparison Techniques
Here are some advanced techniques that can be useful in your next project:
Related Article: How to Export a Python Data Frame to SQL Files
Fuzzy String Matching
Fuzzy string matching is a technique used to compare strings that are similar but not exactly the same. Python has libraries like FuzzyWuzzy and difflib that provide advanced string comparison methods such as the Levenshtein distance, Jaro-Winkler distance, and others. These methods take into account various factors like character similarity, edit distance, and substring matching to determine the similarity between two strings.
Example code using the FuzzyWuzzy library:
from fuzzywuzzy import fuzz string1 = "apple" string2 = "aple" # Calculate Levenshtein distance levenshtein_distance = fuzz.distance(string1, string2) print("Levenshtein distance:", levenshtein_distance) # Calculate Jaro-Winkler similarity jaro_winkler_similarity = fuzz.jaro_winkler(string1, string2) print("Jaro-Winkler similarity:", jaro_winkler_similarity)
Regular Expressions
Regular expressions are powerful tools for pattern matching and string manipulation. Python has a built-in re module that allows for advanced checks using regular expressions. Regular expressions can be used to define complex patterns or search for specific substrings, making them highly versatile for advanced checks.
Example code using regular expressions:
import re string = "Hello, world!" # Search for a pattern in the string pattern = r"world" match = re.search(pattern, string) if match: print("Pattern found") else: print("Pattern not found")
Locale-Specific String Comparison
As mentioned earlier, string comparison behavior can be affected by the locale settings of the system. Python's locale module allows for locale-specific string comparisons, taking into account language-specific sorting rules or collation sequences. This can be useful when working with multilingual applications or dealing with strings in non-English languages.
Example code using the locale
module:
import locale # Set locale to a specific language locale.setlocale(locale.LC_COLLATE, 'en_US.UTF-8') string1 = "apple" string2 = "Äpfel" # Perform locale-specific string comparison result = locale.strcoll(string1, string2) if result == 0: print("Strings are equal") elif result < 0: print("String1 is less than String2") else: print("String1 is greater than String2")
Note: Advanced string comparison techniques may require additional libraries or modules to be installed or imported in your Python environment. Always check the documentation and requirements of the specific libraries or modules being used for advanced string comparisons.
Edge Cases
String comparison can involve various edge cases that need to be handled correctly to avoid bugs.
Related Article: How To Exit/Deactivate a Python Virtualenv
Handling Empty Strings
Comparing empty strings is straightforward but essential to handle correctly.
# file: empty_string_comparison.py a = "" b = "Hello, World!" print(a == b) # False print(a == "") # True print(b != "") # True
Dealing with NoneType
Comparing strings with None
values can lead to TypeError
. It's crucial to handle such cases.
# file: none_comparison.py a = None b = "Hello, World!" print(a == b) # False print(a is None) # True print(b is not None) # True # Safe comparison function def safe_compare(a, b): if a is None or b is None: return False return a == b print(safe_compare(a, b)) # False print(safe_compare(None, None)) # False
Mixed Type Comparisons
Ensure types are compatible when comparing strings with other data types.
# file: mixed_type_comparison.py a = "123" b = 123 print(a == str(b)) # True print(int(a) == b) # True # Function to safely compare different types def safe_mixed_compare(a, b): try: return str(a) == str(b) except ValueError: return False print(safe_mixed_compare(a, b)) # True print(safe_mixed_compare("abc", 123)) # False
Memory Usage
Understanding the memory usage of different string comparison methods can help optimize performance.
Related Article: How To Iterate Over Dictionaries Using For Loops In Python
Memory Efficiency Analysis
Using large strings can consume significant memory. It's important to choose memory-efficient methods.
# file: memory_usage.py import sys a = "a" * 1000000 b = "a" * 1000000 print(sys.getsizeof(a)) # Memory size of string 'a' print(sys.getsizeof(b)) # Memory size of string 'b' # Comparison does not create new strings print(a == b) # True print(sys.getsizeof(a) == sys.getsizeof(b)) # True
Best Practices for Memory Management
1. Avoid Unnecessary Copies: Use in-place modifications when possible.
2. Use Generators: For large data processing, use generators to save memory.
# file: generator_example.py # Large data processing with generator def read_large_file(file_path): with open(file_path, 'r') as file: for line in file: yield line.strip() # Usage for line in read_large_file('large_text_file.txt'): print(line)
How python compares strings internally
In Python, string comparisons are typically performed using the Unicode character encoding standard. Python uses a concept called "code points" to represent characters in a string, and these code points are compared when performing comparisons.
When comparing strings in Python, the comparison is done character by character, starting from the leftmost character (i.e., the first character) of each string. The Unicode code points of the corresponding characters in the two strings are compared to determine their relative order. The comparison is based on the numerical value of the code points, which represent the Unicode character's position in the Unicode character set.
Python follows lexicographic or dictionary order for string comparisons. This means that the comparison is based on the relative position of characters in the Unicode character set. For example, in the Unicode character set, the uppercase letters come before the lowercase letters, and special characters or digits may have their own specific positions.
Python's string comparisons are case-sensitive by default, meaning that uppercase and lowercase letters are treated as distinct characters. For example, "Hello" and "hello" are considered different strings in Python.
It's worth noting that the behavior of comparisons can be affected by the locale settings of the system, which may introduce additional considerations related to language-specific sorting rules or collation sequences.
Object id
In Python, the "object id" is a unique identifier assigned to each object created during the runtime of a Python program. It is an internal reference used by Python to uniquely identify objects in memory. When it comes to string comparison in Python, the "object id" is not relevant, as string comparison is based on the lexicographical order of the characters in the string.
Related Article: How to Create Multiline Comments in Python
Wrapping Up
Strings are sequences of characters, enclosed in single quotes (' ') or double quotes (" "). They are used to represent text data in Python programs. Strings are one of the fundamental data types in Python and are widely used in various applications, including data manipulation, text processing, input/output operations, and more.
Strings are also immutable, which means that once a string is created, its contents cannot be changed. However, you can create new strings by applying various string methods and operations.
Furthermore, strings are unicode-based, which means they can represent characters from different scripts and languages, including ASCII characters, extended Latin characters, non-Latin characters, emoji, and more. Python supports a wide range of string manipulation operations, including string concatenation, slicing, formatting, and more.