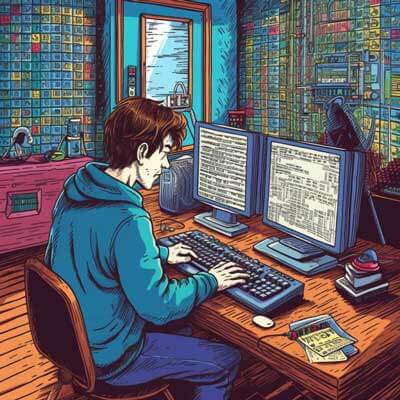
- Stacks Representation in Python
- Lists in Python
- Tuples in Python
- Arrays in Python
- Linked Lists in Python
- Queues in Python
- Deques in Python
- Dictionaries in Python
- What is a Stack in Python?
- Implementing a Stack in Python
- Operations on a Stack in Python
- The Difference Between a Stack and a Queue in Python
- Implementing a Stack in Python Using a Linked List
- Time Complexity of Push and Pop Operations in a Stack in Python
- Checking if a Stack in Python is Empty
- Purpose of Using a Stack in Python
- Storing Elements of Different Data Types in a Stack in Python
- Real-Life Examples of Using a Stack Data Structure in Python
- Additional Resources
Stacks Representation in Python
In Python, there are several data structures that can be used to represent a stack. These include lists, tuples, arrays, linked lists, queues, deques, and dictionaries. Each of these data structures has its own advantages and disadvantages, and the choice of which one to use depends on the specific requirements of the problem at hand.
Related Article: How To Convert a Dictionary To JSON In Python
Lists in Python
Lists in Python are one of the most commonly used data structures and can easily be used to represent a stack. A list is an ordered collection of elements, and new elements can be added to the end of the list using the append()
method. Similarly, elements can be removed from the end of the list using the pop()
method. Here’s an example of how a stack can be implemented using a list in Python:
stack = [] # Push operation stack.append(1) stack.append(2) stack.append(3) # Pop operation top_element = stack.pop() print(top_element) # Output: 3
Tuples in Python
Tuples are another type of data structure in Python that can be used to represent a stack. Tuples are similar to lists, but they are immutable, meaning that once a tuple is created, its elements cannot be modified. However, new tuples can be created by concatenating existing tuples. Here’s an example of how a stack can be implemented using tuples in Python:
stack = () # Push operation stack += (1,) stack += (2,) stack += (3,) # Pop operation top_element = stack[-1] stack = stack[:-1] print(top_element) # Output: 3
Arrays in Python
Arrays in Python provide a way to store homogeneous data types, such as integers or floats, in a contiguous block of memory. However, arrays in Python are not as flexible as lists or tuples when it comes to representing a stack, as their size cannot be dynamically changed. If you know the maximum size of the stack in advance, you can use an array to represent it. Here’s an example of how an array can be used to represent a stack in Python:
import array as arr stack = arr.array('i', []) # Push operation stack.append(1) stack.append(2) stack.append(3) # Pop operation top_element = stack.pop() print(top_element) # Output: 3
Related Article: How to Sort a Dictionary by Key in Python
Linked Lists in Python
Linked lists are a type of data structure in which each element, known as a node, contains a reference to the next node in the list. This allows for efficient insertion and deletion operations, making linked lists a good choice for representing a stack. In Python, linked lists can be implemented using classes and objects. Here’s an example of how a linked list can be used to represent a stack in Python:
class Node: def __init__(self, data=None): self.data = data self.next = None class Stack: def __init__(self): self.head = None # Push operation def push(self, data): new_node = Node(data) if self.head is None: self.head = new_node else: new_node.next = self.head self.head = new_node # Pop operation def pop(self): if self.head is None: raise Exception("Stack is empty") else: top_element = self.head.data self.head = self.head.next return top_element stack = Stack() stack.push(1) stack.push(2) stack.push(3) top_element = stack.pop() print(top_element) # Output: 3
Queues in Python
Queues are a type of data structure in which elements are inserted at one end and removed from the other end. While queues are typically used to implement a first-in-first-out (FIFO) behavior, they can also be used to represent a stack by reversing the order of insertion and removal. In Python, queues can be implemented using the queue
module. Here’s an example of how a queue can be used to represent a stack in Python:
import queue stack = queue.Queue() # Push operation stack.put(1) stack.put(2) stack.put(3) # Pop operation top_element = stack.get() print(top_element) # Output: 3
Deques in Python
Deques, short for double-ended queues, are a type of data structure that allow insertion and removal of elements at both ends. Deques can be used to represent a stack by restricting the insertion and removal operations to one end of the deque. In Python, deques can be implemented using the collections
module. Here’s an example of how a deque can be used to represent a stack in Python:
import collections stack = collections.deque() # Push operation stack.append(1) stack.append(2) stack.append(3) # Pop operation top_element = stack.pop() print(top_element) # Output: 3
Related Article: How to Remove a Key from a Python Dictionary
Dictionaries in Python
Dictionaries in Python are unordered collections of key-value pairs. While dictionaries are not commonly used to represent a stack, they can still be used for this purpose by using the keys as stack positions and the values as the elements. However, it’s important to note that dictionaries do not preserve the order of elements. Here’s an example of how a dictionary can be used to represent a stack in Python:
stack = {} # Push operation stack[0] = 1 stack[1] = 2 stack[2] = 3 # Pop operation top_element = stack.pop(2) print(top_element) # Output: 3
What is a Stack in Python?
A stack is a linear data structure that follows the Last-In-First-Out (LIFO) principle. In a stack, elements are inserted and removed from the same end, known as the top of the stack. The last element inserted is the first one to be removed. This behavior is similar to a stack of plates, where the topmost plate is the one that can be easily accessed.
Implementing a Stack in Python
As seen in the previous examples, a stack can be implemented in Python using various data structures such as lists, tuples, arrays, linked lists, queues, deques, or dictionaries. The choice of implementation depends on the specific requirements of the problem and the desired operations.
Related Article: How to Remove an Element from a List by Index in Python
Operations on a Stack in Python
A stack supports two main operations: push and pop. The push operation inserts an element onto the top of the stack, while the pop operation removes the topmost element from the stack. Additionally, a stack can also support other operations such as peek, which returns the topmost element without removing it, and is_empty, which checks if the stack is empty.
The Difference Between a Stack and a Queue in Python
While both stacks and queues are linear data structures, they differ in their behavior. In a stack, elements are inserted and removed from the same end, known as the top, following the LIFO principle. On the other hand, in a queue, elements are inserted at one end and removed from the other end, following the FIFO principle. This means that the first element inserted in a queue is the first one to be removed.
Implementing a Stack in Python Using a Linked List
As shown in a previous example, a linked list can be used to implement a stack in Python. By using a linked list, we can efficiently insert and remove elements at the top of the stack. The implementation involves creating a class for the nodes of the linked list and another class for the stack itself. The stack class keeps track of the head node of the linked list, which represents the top of the stack.
Related Article: How to Solve a Key Error in Python
Time Complexity of Push and Pop Operations in a Stack in Python
The time complexity of the push and pop operations in a stack depends on the underlying data structure used to implement the stack. For data structures like lists, tuples, arrays, and deques, the push and pop operations have a time complexity of O(1), meaning they can be performed in constant time regardless of the size of the stack. However, for data structures like linked lists and queues, the time complexity of the push and pop operations is O(1) for the best case and O(n) for the worst case, where n is the number of elements in the stack.
Checking if a Stack in Python is Empty
To check if a stack in Python is empty, we can use the len()
function to get the length of the stack. If the length is 0, it means the stack is empty. Here’s an example:
stack = [] if len(stack) == 0: print("Stack is empty") else: print("Stack is not empty")
Purpose of Using a Stack in Python
Stacks are commonly used in programming for various purposes. Some of the common use cases of stacks include:
– Reversing a string or a list
– Implementing function calls and recursion
– Evaluating arithmetic expressions
– Parsing and evaluating postfix expressions
– Undo and redo functionality in text editors
– Backtracking algorithms
Related Article: How to Check If Something Is Not In A Python List
Storing Elements of Different Data Types in a Stack in Python
In Python, stacks can store elements of different data types. This is because Python is a dynamically typed language, meaning that variables can hold values of different types. When using a list, tuple, array, or deque to represent a stack, elements of different data types can be added to the stack without any restrictions. However, when using a linked list or a dictionary, it’s important to ensure that the elements are compatible with the data type expected by the data structure.
Real-Life Examples of Using a Stack Data Structure in Python
Stacks have a wide range of applications in real-life scenarios. Here are some examples of how stacks are used in different domains:
1. Web Browsers: Web browsers use a stack to implement the back and forward buttons. Each page visit is added to a stack, allowing users to navigate back and forth through their browsing history.
2. Text Editors: Text editors use a stack to implement the undo and redo functionality. Each editing operation is stored in a stack, allowing users to undo or redo changes.
3. Call Stack: In programming, a call stack is used to keep track of function calls. When a function is called, its information is pushed onto the stack, and when the function returns, its information is popped from the stack.
4. Expression Evaluation: Stacks are often used to evaluate arithmetic expressions. In this case, the stack is used to store operands and operators, and the expression is evaluated based on the precedence of the operators.
5. Compiler Design: Stacks are used in compiler design for parsing and syntax analysis. The stack is used to keep track of grammar rules and to perform reductions and shifts.
Additional Resources
– GeeksforGeeks – Stack in Python
– Real Python – Python Lists: A Beginner’s Guide