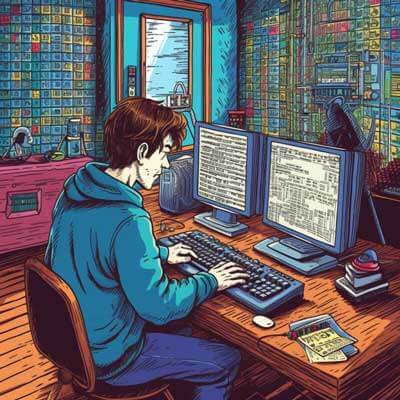
Table of Contents
Serialization
Serialization is the process of converting complex data types, such as Python objects, into a format that can be easily transmitted and stored, such as JSON or XML. In Django REST Framework (DRF), serialization is a fundamental concept that allows us to convert our database models into JSON responses that can be consumed by client applications.
DRF provides a useful serializer class, aptly named Serializer
, that allows us to define the structure of our serialized data. Let's take a look at an example:
from rest_framework import serializers class BookSerializer(serializers.Serializer): title = serializers.CharField(max_length=100) author = serializers.CharField(max_length=100) publication_date = serializers.DateField() book_data = { 'title': 'Deep Dive into Django REST Framework', 'author': 'John Doe', 'publication_date': '2022-01-01' } serializer = BookSerializer(data=book_data) serializer.is_valid() serializer.save()
In the above example, we define a BookSerializer
class that extends the Serializer
class provided by DRF. We specify the fields we want to include in our serialized representation and their corresponding data types. Once we have defined our serializer, we can use it to validate and save data.
Related Article: How to Print an Exception in Python
Filtering
Filtering is a crucial aspect of building RESTful APIs to allow clients to retrieve only the data they need. DRF provides various ways to filter data, including query parameters, custom filter backends, and complex lookups.
Let's consider an example where we want to filter a list of books based on their publication date. We can achieve this by using the django_filters
package along with DRF:
from django_filters.rest_framework import DjangoFilterBackend from rest_framework import filters class BookFilter(filters.FilterSet): publication_date = filters.DateFilter(field_name='publication_date', lookup_expr='gte') class Meta: model = Book fields = ['publication_date'] class BookViewSet(viewsets.ModelViewSet): queryset = Book.objects.all() serializer_class = BookSerializer filter_backends = [DjangoFilterBackend] filterset_class = BookFilter
In the above example, we define a BookFilter
class that extends the FilterSet
class provided by django_filters
. We specify the field we want to filter on (publication_date
) and the lookup expression (gte
for 'greater than or equal to').
We then use the DjangoFilterBackend
as one of the filter backends for our BookViewSet
. This allows us to filter the list of books based on the provided query parameters.
Authentication
Authentication is the process of identifying and verifying the identity of a user. DRF provides various authentication methods out of the box, including token-based authentication, session-based authentication, and OAuth.
Let's consider an example where we want to implement token-based authentication in our API:
from rest_framework.authentication import TokenAuthentication from rest_framework.permissions import IsAuthenticated class BookViewSet(viewsets.ModelViewSet): queryset = Book.objects.all() serializer_class = BookSerializer authentication_classes = [TokenAuthentication] permission_classes = [IsAuthenticated]
In the above example, we add the TokenAuthentication
class as one of the authentication classes for our BookViewSet
. This enables token-based authentication for our API.
We also specify the IsAuthenticated
class as one of the permission classes. This ensures that only authenticated users can access the API endpoints.
Authorization
Authorization is the process of granting or denying access to specific resources based on the user's permissions. DRF provides various authorization methods, including role-based access control (RBAC), object-level permissions, and custom authorization logic.
Let's consider an example where we want to implement object-level permissions in our API:
from rest_framework.permissions import IsAuthenticated, BasePermission class IsOwnerOrReadOnly(BasePermission): def has_object_permission(self, request, view, obj): if request.method in permissions.SAFE_METHODS: return True return obj.owner == request.user class BookViewSet(viewsets.ModelViewSet): queryset = Book.objects.all() serializer_class = BookSerializer permission_classes = [IsAuthenticated, IsOwnerOrReadOnly]
In the above example, we define a custom permission class IsOwnerOrReadOnly
that extends the BasePermission
class provided by DRF. We override the has_object_permission
method to implement our custom authorization logic.
We then add IsAuthenticated
and IsOwnerOrReadOnly
as the permission classes for our BookViewSet
. This ensures that only authenticated users can perform write operations on the books, while allowing read operations for all users.
Related Article: How To Handle Ambiguous Truth Value In Python Series
Pagination
Pagination is the process of splitting large datasets into smaller, more manageable chunks. This can greatly improve the performance of API endpoints by reducing the amount of data transferred over the network.
DRF provides built-in pagination classes that can be easily integrated into our views. Let's consider an example where we want to paginate the list of books in our API:
from rest_framework.pagination import PageNumberPagination class BookPagination(PageNumberPagination): page_size = 10 page_size_query_param = 'page_size' max_page_size = 100 class BookViewSet(viewsets.ModelViewSet): queryset = Book.objects.all() serializer_class = BookSerializer pagination_class = BookPagination
In the above example, we define a BookPagination
class that extends the PageNumberPagination
class provided by DRF. We specify the page_size
(number of items per page), page_size_query_param
(query parameter to control the page size), and max_page_size
(maximum page size).
We then add BookPagination
as the pagination class for our BookViewSet
. This enables pagination for the list of books in our API.
Viewsets
Viewsets in DRF provide a way to combine common CRUD (Create, Retrieve, Update, Delete) operations into a single class, simplifying the code and reducing duplication. DRF provides various types of viewsets, including ModelViewSet, ReadOnlyModelViewSet, and GenericViewSet.
Let's consider an example where we want to use a ModelViewSet for our Book model:
from rest_framework import viewsets class BookViewSet(viewsets.ModelViewSet): queryset = Book.objects.all() serializer_class = BookSerializer
In the above example, we define a BookViewSet
class that extends the ModelViewSet
class provided by DRF. We specify the queryset (list of books) and the serializer class (BookSerializer
).
With this single viewset, we get all the CRUD operations (list, create, retrieve, update, delete) for our Book model.
Serializers
Serializers in DRF provide a way to define the structure of our serialized data and handle the conversion between complex data types and JSON representations. DRF provides a useful serializer class, aptly named Serializer
, that allows us to define the structure of our serialized data.
Let's consider an example where we want to create a custom serializer for our Book model:
from rest_framework import serializers class BookSerializer(serializers.ModelSerializer): class Meta: model = Book fields = ['title', 'author', 'publication_date']
In the above example, we define a BookSerializer
class that extends the ModelSerializer
class provided by DRF. We specify the model (Book
) and the fields we want to include in our serialized representation.
With this serializer, we can easily serialize and deserialize Book objects to and from JSON representations.
Fields
Fields in serializers allow us to customize the serialization and deserialization process. DRF provides a wide range of field types, including built-in fields like CharField, IntegerField, and DateTimeField, as well as custom fields that we can define ourselves.
Let's consider an example where we want to use a custom field in our BookSerializer:
from rest_framework import serializers class CustomIntegerField(serializers.Field): def to_representation(self, value): return value * 100 class BookSerializer(serializers.ModelSerializer): custom_field = CustomIntegerField() class Meta: model = Book fields = ['title', 'author', 'publication_date', 'custom_field']
In the above example, we define a custom field CustomIntegerField
that extends the Field
class provided by DRF. We override the to_representation
method to customize the serialization process.
We then add the custom_field
to our BookSerializer
and include it in the fields
list. This custom field will multiply the value by 100 before serializing it.
Related Article: How to Select Multiple Columns in a Pandas Dataframe
Advantages of using Python for web development
Python is a popular programming language for web development due to its simplicity, readability, and vast ecosystem of libraries and frameworks. Here are some advantages of using Python for web development:
1. Easy to learn and read: Python has a clean and readable syntax, making it easy for developers to understand and write code. This reduces the learning curve for new developers and enhances collaboration among team members.
2. Rich ecosystem: Python has a large and active community that has developed a wide range of libraries and frameworks for web development. Django and Flask are two popular web frameworks in Python that provide useful tools and features for building web applications.
3. Scalability: Python's scalability is a key advantage for web development. It offers multiple solutions for scaling applications, including horizontal scaling with load balancing and vertical scaling with multiple processes or threads.
4. Integration capabilities: Python can easily integrate with other languages and technologies, making it a suitable choice for building complex web applications. It can be used alongside JavaScript frameworks like React or Angular, and can interact with databases, messaging systems, and other external services.
5. Extensive testing support: Python has robust testing frameworks, such as Pytest and unittest, that enable developers to write comprehensive tests for their web applications. This helps ensure the quality and reliability of the codebase.
6. Community support: Python has a vibrant and supportive community that actively contributes to its development and provides a wealth of resources, tutorials, and documentation. This makes it easier for developers to find solutions to their problems and stay up-to-date with the latest trends and best practices.
Why is Django a popular framework for building web applications?
Django is a high-level web framework written in Python that follows the Model-View-Controller (MVC) architectural pattern. It is known for its simplicity, scalability, and robustness, which have contributed to its popularity among developers. Here are some reasons why Django is a popular framework for building web applications:
1. Batteries included: Django is often referred to as a "batteries included" framework because it comes with a wide range of built-in features and tools. These include an ORM (Object-Relational Mapping) for database management, a templating engine for rendering HTML templates, and an authentication system for handling user authentication and authorization.
2. Scalability: Django provides scalability options that allow applications to handle high traffic and large datasets. It supports horizontal scaling with load balancing, database replication, and caching, as well as vertical scaling with multiple processes or threads.
3. Security: Django has built-in security features that help developers build secure web applications. It includes protection against common security vulnerabilities, such as cross-site scripting (XSS) and cross-site request forgery (CSRF), as well as user authentication and authorization mechanisms.
4. ORM and database support: Django's ORM makes it easy to interact with databases and perform database operations without writing raw SQL queries. It supports multiple databases, including PostgreSQL, MySQL, SQLite, and Oracle, allowing developers to choose the most suitable database for their application.
5. Community and ecosystem: Django has a large and active community that contributes to its development and provides a vast ecosystem of third-party packages, libraries, and extensions. This makes it easier for developers to find solutions to common problems, reuse existing code, and enhance the functionality of their applications.
6. Documentation and community support: Django has comprehensive and well-organized documentation that covers all aspects of the framework, including tutorials, guides, and reference materials. Additionally, the Django community is known for its helpfulness and responsiveness, providing support through mailing lists, forums, and online communities.
Additional Resources
- Django Official Website