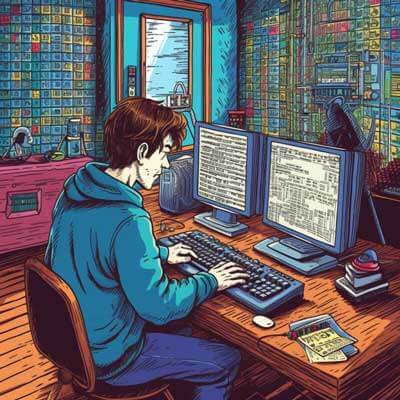
Table of Contents
Understanding Python Dictionaries
Python dictionaries are a powerful data structure that allow you to store and access data using key-value pairs. Unlike lists or tuples, dictionaries are unordered, meaning that the elements are not stored in any particular order. This allows for efficient lookup and retrieval of values based on their associated keys.
To create a dictionary in Python, you use curly braces {} and separate each key-value pair with a colon. For example:
my_dict = {'name': 'John', 'age': 25, 'city': 'New York'}
In this example, the keys are 'name', 'age', and 'city', and the corresponding values are 'John', 25, and 'New York' respectively. You can also create an empty dictionary by simply using empty curly braces.
Dictionaries are mutable, meaning that you can modify their contents by adding, updating, or removing key-value pairs. You can access the value associated with a particular key by using square brackets [] and specifying the key. For example:
print(my_dict['name']) # Output: John
If you try to access a key that does not exist in the dictionary, a KeyError will be raised. To avoid this, you can use the get()
method, which returns None if the key is not found, or a default value that you specify. For example:
print(my_dict.get('name')) # Output: John print(my_dict.get('gender')) # Output: None print(my_dict.get('gender', 'Unknown')) # Output: Unknown
You can loop through the keys, values, or key-value pairs of a dictionary using various methods. One common method is to use the items()
method, which returns a list of tuples containing the key-value pairs. For example:
for key, value in my_dict.items(): print(key, value)
This will output:
name John age 25 city New York
Another method is to use the keys()
method, which returns a list of all the keys in the dictionary. You can then iterate over these keys to access the corresponding values. For example:
for key in my_dict.keys(): print(key, my_dict[key])
This will produce the same output as before.
Lastly, you can use the values()
method to loop through all the values in the dictionary. For example:
for value in my_dict.values(): print(value)
This will output:
John 25 New York
Related Article: Optimizing FastAPI Applications: Modular Design, Logging, and Testing
Basic Operations: Accessing and Modifying Dictionary Elements
In this chapter, we will explore the basic operations for accessing and modifying elements in a Python dictionary. Dictionaries in Python are mutable, meaning that you can change their values or add new key-value pairs.
To access a value in a dictionary, you can use square brackets and provide the key of the desired element. For example:
# Define a dictionary student = {'name': 'John', 'age': 20, 'grade': 'A'} # Accessing values print(student['name']) # Output: John print(student['age']) # Output: 20
In the code snippet above, we created a dictionary called student
with keys 'name', 'age', and 'grade'. We accessed the values of the keys 'name' and 'age' using square brackets.
If you try to access a key that doesn't exist in the dictionary, a KeyError
will be raised. To avoid this, you can use the get()
method, which allows you to provide a default value if the key is not found:
# Accessing a non-existent key print(student.get('city')) # Output: None # Accessing a non-existent key with a default value print(student.get('city', 'Unknown')) # Output: Unknown
In the first get()
example, we tried to access the key 'city', which doesn't exist in the dictionary. The method returned None
. In the second example, we provided a default value of 'Unknown', which was returned since the key 'city' is not present in the dictionary.
To modify the value of a key in a dictionary, you can simply assign a new value to it:
# Modifying a value student['grade'] = 'B' print(student['grade']) # Output: B
In the code snippet above, we changed the value of the key 'grade' from 'A' to 'B'. The new value is then printed.
If the key doesn't exist in the dictionary, assigning a value to it will create a new key-value pair:
# Adding a new key-value pair student['city'] = 'New York' print(student['city']) # Output: New York
In the code snippet above, we added a new key-value pair to the dictionary. The key 'city' with the value 'New York' is now present in the dictionary.
These are the basic operations for accessing and modifying dictionary elements in Python. Now that you know how to work with dictionary values, you can move on to more complex operations such as iterating and looping through dictionaries.
Looping Through Dictionary Keys
When working with Python dictionaries, it is often necessary to iterate over the keys of the dictionary to perform certain operations. In this section, we will explore different ways to loop through dictionary keys.
One way to loop through dictionary keys is by using a for
loop. We can directly iterate over the dictionary object, which will return the keys of the dictionary. Here's an example:
# Creating a dictionary fruits = {"apple": 1, "banana": 2, "orange": 3} # Looping through keys using a for loop for key in fruits: print(key)
Output:
apple banana orange
In the above example, the for
loop iterates over the dictionary fruits
and assigns each key to the variable key
. We then simply print the value of key
.
Another way to loop through dictionary keys is by using the keys()
method. This method returns a view object that contains the keys of the dictionary. We can then iterate over this view object using a for
loop. Here's an example:
# Creating a dictionary fruits = {"apple": 1, "banana": 2, "orange": 3} # Looping through keys using the keys() method for key in fruits.keys(): print(key)
Output:
apple banana orange
In this example, we use the keys()
method to obtain a view object containing the keys of the fruits
dictionary. We then loop through this view object using a for
loop and print each key.
We can also use the dict.keys()
method to check if a particular key exists in the dictionary. This can be useful when we want to perform certain operations based on the presence of a specific key. Here's an example:
# Creating a dictionary fruits = {"apple": 1, "banana": 2, "orange": 3} # Checking if a key exists in the dictionary if "apple" in fruits.keys(): print("The key 'apple' exists in the dictionary.")
Output:
The key 'apple' exists in the dictionary.
In this example, we use the in
keyword to check if the key "apple" exists in the dictionary fruits
. If it does, we print a message indicating its presence.
To summarize, looping through dictionary keys in Python can be done using a for
loop or by using the keys()
method to obtain a view object containing the keys of the dictionary. This allows us to perform various operations on the keys of a dictionary.
Looping Through Dictionary Values
When working with dictionaries in Python, you often need to iterate or loop through the values stored in the dictionary. This allows you to access and manipulate each individual value in the dictionary.
There are several ways to loop through dictionary values in Python. Let's explore some of the most common methods.
Method 1: Using the values() method
The easiest way to loop through dictionary values is by using the values()
method. This method returns a view object that contains all the values in the dictionary.
Here's an example that demonstrates how to use the values()
method to loop through dictionary values:
fruits = {'apple': 'red', 'banana': 'yellow', 'orange': 'orange'} for color in fruits.values(): print(color)
Output:
red yellow orange
In this example, the values()
method returns a view object that contains the values 'red', 'yellow', and 'orange'. The for
loop then iterates over each value and prints it.
Method 2: Using the items() method
Another way to loop through dictionary values is by using the items()
method. This method returns a view object that contains all the key-value pairs in the dictionary as tuples.
Here's an example that demonstrates how to use the items()
method to loop through dictionary values:
fruits = {'apple': 'red', 'banana': 'yellow', 'orange': 'orange'} for fruit, color in fruits.items(): print(color)
Output:
red yellow orange
In this example, the items()
method returns a view object that contains the key-value pairs ('apple', 'red'), ('banana', 'yellow'), and ('orange', 'orange'). The for
loop then iterates over each key-value pair, but only the value is printed.
Method 3: Using a list comprehension
If you prefer a more concise syntax, you can use a list comprehension to loop through dictionary values and store them in a list.
Here's an example that demonstrates how to use a list comprehension to loop through dictionary values:
fruits = {'apple': 'red', 'banana': 'yellow', 'orange': 'orange'} values = [color for color in fruits.values()] print(values)
Output:
['red', 'yellow', 'orange']
In this example, the list comprehension loops through each value in the dictionary and adds it to a new list called values
.
These are just a few examples of how you can loop through dictionary values in Python. Depending on your specific use case, you may find one method more suitable than the others. Experiment with these techniques to find the one that best fits your needs.
Now that you know how to loop through dictionary values, you can easily access and manipulate them in your Python programs.
Related Article: How to Use Redis with Django Applications
Looping Through Dictionary Items
Iterating over a Python dictionary allows you to access each key-value pair in the dictionary and perform operations on them. In this section, we will explore different ways to loop through dictionary items.
Using a for loop
One common way to loop through dictionary items is by using a for loop. When using a for loop with a dictionary, it iterates over the dictionary keys by default. Here's an example:
fruits = {"apple": "red", "banana": "yellow", "orange": "orange"} for fruit in fruits: print(fruit) # prints the keys
Output:
apple banana orange
To access the corresponding values, you can use the keys to index the dictionary:
for fruit in fruits: print(fruits[fruit]) # prints the values
Output:
red yellow orange
If you want to loop through both the keys and values simultaneously, you can use the items()
method of the dictionary:
for fruit, color in fruits.items(): print(fruit, "is", color)
Output:
apple is red banana is yellow orange is orange
Using a while loop
Another way to loop through dictionary items is by using a while loop. You can iterate over the keys of the dictionary and access the corresponding values inside the loop. Here's an example:
fruits = {"apple": "red", "banana": "yellow", "orange": "orange"} keys = list(fruits.keys()) # convert dictionary keys to a list i = 0 while i < len(keys): fruit = keys[i] print(fruit, "is", fruits[fruit]) i += 1
Output:
apple is red banana is yellow orange is orange
Using comprehension
Python also provides comprehension syntax to loop through dictionary items and create a new dictionary or perform some operations. Here's an example of using a dictionary comprehension to create a new dictionary with modified values:
fruits = {"apple": "red", "banana": "yellow", "orange": "orange"} modified_fruits = {fruit: color.upper() for fruit, color in fruits.items()} print(modified_fruits)
Output:
{'apple': 'RED', 'banana': 'YELLOW', 'orange': 'ORANGE'}
In this example, we loop through the key-value pairs of the fruits
dictionary using a dictionary comprehension and create a new dictionary modified_fruits
with the same keys but uppercase values.
Related Article: How To Convert a Python Dict To a Dataframe
Nested Dictionaries: A Closer Look
What happens when you need to store more complex data structures within a dictionary? That's where nested dictionaries come in.
A nested dictionary is a dictionary that contains other dictionaries as its values. This allows you to create a hierarchical structure, similar to a tree, where each level represents a different category or subcategory of data.
Let's take a closer look at how to work with nested dictionaries in Python.
Creating a Nested Dictionary
To create a nested dictionary, you simply assign a dictionary as the value to a key within another dictionary. Here's an example:
person = { "name": "John Doe", "age": 30, "address": { "street": "123 Main St", "city": "New York", "state": "NY" } }
In this example, the person
dictionary contains a nested dictionary under the key "address". The nested dictionary stores the person's street, city, and state.
Accessing Values in a Nested Dictionary
Accessing values in a nested dictionary follows a similar syntax to accessing values in a regular dictionary. You use square brackets to specify the key or keys needed to access the desired value. Here's an example:
print(person["address"]["city"]) # Output: New York
In this example, we access the value of the "city" key within the nested "address" dictionary using the square bracket notation.
Looping Through a Nested Dictionary
Iterating over a nested dictionary requires nested loops. You can use a combination of for
loops and dictionary methods to loop through the keys and values at each level. Here's an example:
for key1, value1 in person.items(): if isinstance(value1, dict): for key2, value2 in value1.items(): print(key2, ":", value2) else: print(key1, ":", value1)
In this example, we use the items()
method to iterate over the key-value pairs in the person
dictionary. If a value is itself a dictionary (checked using isinstance()
), we iterate over its key-value pairs as well. This allows us to print all the keys and values within the nested dictionary.
Related Article: Converting cURL Commands to Python
Modifying Values in a Nested Dictionary
To modify a value in a nested dictionary, you can use the same square bracket notation as when accessing the value. Here's an example:
person["address"]["city"] = "San Francisco"
In this example, we modify the value of the "city" key within the nested "address" dictionary to "San Francisco".
Common Use Cases: Filtering and Transforming Dictionary Data
Filtering and transforming dictionary data is a common task when working with Python dictionaries. In this chapter, we will explore some common use cases and techniques for filtering and transforming dictionary data.
Filtering Dictionary Data
Filtering dictionary data involves selecting specific key-value pairs based on certain criteria. There are multiple ways to achieve this, depending on the requirements of your task.
One common approach is to use a dictionary comprehension. This allows you to create a new dictionary by iterating over the original dictionary and applying a condition to filter the data.
# Filtering dictionary data using dictionary comprehension original_dict = {'apple': 2, 'banana': 3, 'orange': 4, 'grape': 1} filtered_dict = {key: value for key, value in original_dict.items() if value > 2} print(filtered_dict) # Output: {'banana': 3, 'orange': 4}
In the above example, we filter the original dictionary by only including key-value pairs where the value is greater than 2.
Another approach is to use the filter()
function along with a lambda function. This function allows you to apply a condition to filter the dictionary data.
# Filtering dictionary data using the filter() function original_dict = {'apple': 2, 'banana': 3, 'orange': 4, 'grape': 1} filtered_dict = dict(filter(lambda item: item[1] > 2, original_dict.items())) print(filtered_dict) # Output: {'banana': 3, 'orange': 4}
In this example, we use the filter()
function to create an iterator that filters the dictionary items based on the condition provided by the lambda function. We then convert the filtered items back into a dictionary using the dict()
function.
Transforming Dictionary Data
Transforming dictionary data involves modifying the values of the dictionary based on certain rules or operations. Let's explore a couple of common techniques for transforming dictionary data.
One common approach is to use a dictionary comprehension with an expression that transforms the values of the dictionary.
# Transforming dictionary data using dictionary comprehension original_dict = {'apple': 2, 'banana': 3, 'orange': 4, 'grape': 1} transformed_dict = {key: value * 2 for key, value in original_dict.items()} print(transformed_dict) # Output: {'apple': 4, 'banana': 6, 'orange': 8, 'grape': 2}
In this example, we multiply each value in the original dictionary by 2 using a dictionary comprehension.
Another technique is to use the map()
function along with a lambda function to apply a transformation to each value in the dictionary.
# Transforming dictionary data using the map() function original_dict = {'apple': 2, 'banana': 3, 'orange': 4, 'grape': 1} transformed_dict = {key: value for key, value in map(lambda item: (item[0], item[1] * 2), original_dict.items())} print(transformed_dict) # Output: {'apple': 4, 'banana': 6, 'orange': 8, 'grape': 2}
In this example, we use the map()
function to apply the lambda function to each item in the dictionary, which multiplies the value by 2. We then convert the transformed items back into a dictionary using a dictionary comprehension.
Advanced Techniques: Merging and Updating Dictionaries
One of the most useful features of dictionaries is the ability to merge or update them, which can be essential when working with large datasets or when combining information from multiple sources.
In this chapter, we will explore advanced techniques for merging and updating dictionaries in Python. We will cover different methods and approaches to accomplish this task efficiently.
Merging Dictionaries
Merging dictionaries involves combining the key-value pairs of multiple dictionaries into a single dictionary. Python provides several methods to achieve this, and we will discuss two of the most common approaches.
Method 1: Using the update() method
The update()
method allows you to merge two or more dictionaries into one. It takes another dictionary as an argument and adds its key-value pairs to the original dictionary. If there are any common keys between the dictionaries, the values from the argument dictionary will overwrite the values from the original dictionary.
Here's an example that demonstrates the usage of the update()
method:
dict1 = {'a': 1, 'b': 2} dict2 = {'c': 3, 'd': 4} dict1.update(dict2) print(dict1) # Output: {'a': 1, 'b': 2, 'c': 3, 'd': 4}
In this example, the update()
method merges dict2
into dict1
, resulting in a new dictionary with all the key-value pairs from both dictionaries.
Method 2: Using the double asterisk operator (**)
Another way to merge dictionaries is by using the double asterisk operator (**). This operator can be used to unpack a dictionary into another dictionary, effectively merging them together.
Here's an example that demonstrates the usage of the double asterisk operator:
dict1 = {'a': 1, 'b': 2} dict2 = {'c': 3, 'd': 4} merged_dict = {**dict1, **dict2} print(merged_dict) # Output: {'a': 1, 'b': 2, 'c': 3, 'd': 4}
In this example, the double asterisk operator unpacks both dictionaries (dict1
and dict2
) into a new dictionary, resulting in a merged dictionary with all the key-value pairs.
Updating Dictionaries
Updating dictionaries involves modifying the values of existing keys in a dictionary. Python provides different methods to update dictionaries, and we will discuss two common approaches.
Method 1: Using the update() method
The update()
method can also be used to update the values of existing keys in a dictionary. When a key is present in both dictionaries, the update()
method will replace the old value with the new value.
Here's an example that demonstrates updating a dictionary using the update()
method:
dict1 = {'a': 1, 'b': 2} dict2 = {'b': 3, 'c': 4} dict1.update(dict2) print(dict1) # Output: {'a': 1, 'b': 3, 'c': 4}
In this example, the update()
method updates the value of the key 'b'
from 2
to 3
.
Method 2: Using the square bracket notation
Another way to update a dictionary is by using the square bracket notation. This method allows you to directly assign a new value to a specific key in the dictionary.
Here's an example that demonstrates updating a dictionary using the square bracket notation:
dict1 = {'a': 1, 'b': 2} dict1['b'] = 3 print(dict1) # Output: {'a': 1, 'b': 3}
In this example, the value of the key 'b'
is updated from 2
to 3
using the square bracket notation.
Real World Examples: Analyzing Data and Creating Reports
In this chapter, we will explore real-world examples of how to iterate and loop through Python dictionaries to analyze data and create reports. Dictionaries are a powerful data structure in Python that allow us to store and retrieve data using key-value pairs.
Related Article: How To Sort A Dictionary By Value In Python
Analyzing Data
One common use case for iterating through dictionaries is to analyze data. Let's say we have a dictionary that represents the sales of various products:
sales = { 'apple': 10, 'banana': 5, 'orange': 7, 'grape': 3 }
We can iterate through the dictionary to calculate the total number of sales:
total_sales = 0 for product, quantity in sales.items(): total_sales += quantity print(f'Total sales: {total_sales}')
This will output:
Total sales: 25
We can also find the product with the highest sales:
max_sales = 0 best_selling_product = '' for product, quantity in sales.items(): if quantity > max_sales: max_sales = quantity best_selling_product = product print(f'Best selling product: {best_selling_product}')
This will output:
Best selling product: apple
Creating Reports
Another common use case for iterating through dictionaries is to create reports. Let's say we have a dictionary that represents the sales of various products in different regions:
sales = { 'apple': { 'north': 5, 'south': 3, 'east': 2, 'west': 4 }, 'banana': { 'north': 2, 'south': 1, 'east': 3, 'west': 3 }, 'orange': { 'north': 3, 'south': 2, 'east': 4, 'west': 1 } }
We can iterate through the dictionary to create a report that shows the sales of each product in each region:
for product, region_data in sales.items(): print(f'Sales of {product}:') for region, quantity in region_data.items(): print(f'- {region.capitalize()}: {quantity}') print()
This will output:
Sales of apple: - North: 5 - South: 3 - East: 2 - West: 4 Sales of banana: - North: 2 - South: 1 - East: 3 - West: 3 Sales of orange: - North: 3 - South: 2 - East: 4 - West: 1
This report provides a clear overview of the sales of each product in each region.
Handling Missing Keys: Using Default Values and the 'get' Method
When working with Python dictionaries, it's common to encounter situations where a key may be missing. In these cases, it's important to handle the missing keys gracefully to avoid errors in your code. Python provides two convenient methods for handling missing keys: using default values and the 'get' method.
Using Default Values
One way to handle missing keys in a dictionary is to provide a default value that will be returned if the key is not found. This can be achieved by using the square bracket notation with the 'get' method.
# Create a dictionary my_dict = {'apple': 1, 'banana': 2, 'orange': 3} # Access a key with a default value value = my_dict.get('pear', 0) print(value) # Output: 0
In the example above, the 'get' method is used to access the key 'pear' with a default value of 0. Since 'pear' is not present in the dictionary, the default value of 0 is returned.
You can also provide a default value when accessing a key directly using the square bracket notation:
# Create a dictionary my_dict = {'apple': 1, 'banana': 2, 'orange': 3} # Access a key with a default value value = my_dict['pear'] if 'pear' in my_dict else 0 print(value) # Output: 0
This approach checks if the key 'pear' exists in the dictionary before accessing it. If the key is not found, the default value of 0 is returned.
Related Article: How to Fix Indentation Errors in Python
The 'get' Method
The 'get' method is a powerful tool for handling missing keys in dictionaries. It allows you to provide a default value that will be returned if the key is not found, without raising a KeyError.
# Create a dictionary my_dict = {'apple': 1, 'banana': 2, 'orange': 3} # Access a key using the 'get' method value = my_dict.get('apple') print(value) # Output: 1 # Access a missing key using the 'get' method value = my_dict.get('pear') print(value) # Output: None
In the example above, the 'get' method is used to access the keys 'apple' and 'pear'. Since 'apple' is present in the dictionary, its corresponding value of 1 is returned. However, 'pear' is not present in the dictionary, so the 'get' method returns None by default.
You can also provide a default value to the 'get' method:
# Create a dictionary my_dict = {'apple': 1, 'banana': 2, 'orange': 3} # Access a missing key using the 'get' method with a default value value = my_dict.get('pear', 0) print(value) # Output: 0
In this example, the 'get' method is used to access the key 'pear' with a default value of 0. Since 'pear' is not present in the dictionary, the default value of 0 is returned.
Using default values and the 'get' method allows you to handle missing keys in a flexible and robust way. This can be especially useful when working with large dictionaries or when dealing with user input.
Checking for Key Existence: 'in' Operator vs 'dict.get'
When working with Python dictionaries, it is common to check if a specific key exists before trying to access its value. There are multiple ways to accomplish this, but two popular methods are using the 'in' operator and the 'dict.get' method.
The 'in' Operator
The 'in' operator is a simple and straightforward way to check if a key exists in a dictionary. It returns a boolean value, True if the key is present, and False otherwise. Here's an example:
my_dict = {'name': 'John', 'age': 25, 'city': 'New York'} if 'name' in my_dict: print('The key "name" exists in the dictionary.') else: print('The key "name" does not exist in the dictionary.')
In this example, we check if the key 'name' exists in the dictionary 'my_dict'. If it does, we print a message confirming its existence; otherwise, we print a different message.
The 'in' operator is concise and easy to understand, making it a popular choice for checking key existence in Python dictionaries.
The 'dict.get' Method
The 'dict.get' method is another way to check for key existence in a dictionary. It returns the value associated with the specified key if it exists, and a default value if the key is not found. Here's an example:
my_dict = {'name': 'John', 'age': 25, 'city': 'New York'} name = my_dict.get('name', 'Default Name') print(f'The value associated with the key "name" is: {name}') address = my_dict.get('address', 'Default Address') print(f'The value associated with the key "address" is: {address}')
In this example, we use the 'dict.get' method to retrieve the value associated with the key 'name'. If the key exists, the method returns the corresponding value; otherwise, it returns the default value 'Default Name'. We also demonstrate using the 'dict.get' method for a key that does not exist, returning the default value 'Default Address'.
The 'dict.get' method provides a flexible way to handle key existence in a dictionary by allowing us to specify a default value to be returned when the key is not found.
Related Article: How to Use Python's Numpy.Linalg.Norm Function
Choosing Between 'in' Operator and 'dict.get'
Both the 'in' operator and the 'dict.get' method have their advantages and use cases. Here are some considerations to help you choose the appropriate method:
- Use the 'in' operator when you simply need to check if a key exists in a dictionary and do not require the associated value.
- Use the 'dict.get' method when you need to access the value associated with a key, and also want to specify a default value to be returned if the key is not found.
By understanding the differences between the 'in' operator and 'dict.get' method, you can effectively check for key existence in Python dictionaries and handle different scenarios accordingly.
Performance Considerations: Dictionary Size and Optimization
When working with large dictionaries in Python, it is important to consider the performance implications. As the size of a dictionary increases, the time taken to iterate and loop through it also increases. Therefore, it is crucial to optimize the code to ensure efficient execution.
Here are some performance considerations to keep in mind when working with large dictionaries:
1. Limit the Size of the Dictionary
One way to improve performance is to limit the size of the dictionary as much as possible. If you only need a subset of the data, consider filtering or reducing the dictionary size before iterating through it. This can be done using techniques such as dictionary comprehension or filtering functions like filter()
.
For example, let's say we have a large dictionary data
and we only need to iterate through the items with values greater than 100:
data = {'a': 50, 'b': 150, 'c': 200, 'd': 75} filtered_data = {key: value for key, value in data.items() if value > 100} for key, value in filtered_data.items(): print(key, value)
This approach reduces the size of the dictionary and improves performance by only iterating through the relevant items.
2. Use Dictionary Views
Python provides dictionary views, which are dynamic views of the dictionary's keys, values, or items. Views provide a way to iterate over the dictionary without creating a separate list or tuple, thereby reducing memory usage and improving performance.
There are three types of dictionary views available:
- keys()
view: This returns a view of the dictionary's keys.
- values()
view: This returns a view of the dictionary's values.
- items()
view: This returns a view of the dictionary's key-value pairs.
Here's an example of using the items()
view to iterate through a large dictionary:
data = {'a': 1, 'b': 2, 'c': 3, 'd': 4} for key, value in data.items(): print(key, value)
Using dictionary views instead of creating separate lists or tuples can significantly improve performance when working with large dictionaries.
Related Article: How to Access Python Data Structures with Square Brackets
3. Avoid Unnecessary Dictionary Operations
Performing unnecessary dictionary operations can impact performance. Whenever possible, avoid unnecessary operations such as dictionary updates, deletions, or lookups within loops.
For example, consider the following code:
data = {'a': 1, 'b': 2, 'c': 3, 'd': 4} for key, value in data.items(): if key in data: print(key, value)
In this case, the key in data
lookup is unnecessary since we are already iterating through the dictionary using data.items()
. Removing the unnecessary lookup can improve performance.
4. Use the get()
Method for Dictionary Lookups
When performing dictionary lookups, using the get()
method instead of direct indexing can be more efficient, especially when dealing with large dictionaries. The get()
method allows you to provide a default value if the key is not found, avoiding the need to handle key errors.
Here's an example:
data = {'a': 1, 'b': 2, 'c': 3, 'd': 4} value = data.get('e', 0) print(value) # Output: 0
Using the get()
method with a default value can improve performance and simplify the code when dealing with large dictionaries.
5. Consider Using Alternative Data Structures
In some cases, using alternative data structures may be more suitable than dictionaries, depending on the specific requirements of your program. For example, if you need to perform frequent lookups or maintain a specific order, you might consider using a different data structure such as a list, set, or OrderedDict.
Carefully evaluating the requirements of your program and choosing the appropriate data structure can greatly improve performance.
Optimizing the performance of dictionary operations is crucial when working with large dictionaries in Python. By limiting the size of the dictionary, using dictionary views, avoiding unnecessary operations, using the
get()
method, and considering alternative data structures, you can ensure efficient execution and improve the performance of your code.
Working with Dictionary Views: 'keys', 'values', and 'items'
Dictionary views in Python provide a dynamic view into the keys, values, or key-value pairs of a dictionary. They allow you to access and manipulate the dictionary contents without creating a new list or tuple. In this section, we will explore the three different dictionary views available in Python: 'keys', 'values', and 'items'.
Related Article: Database Query Optimization in Django: Boosting Performance for Your Web Apps
The 'keys' View
The 'keys' view provides a dynamic view of the keys present in a dictionary. It allows you to iterate over the keys or perform set operations like intersection, union, and difference. Here's an example of how to use the 'keys' view:
fruits = {'apple': 'red', 'banana': 'yellow', 'orange': 'orange'} # Iterate over the keys for key in fruits.keys(): print(key) # Check if a key exists if 'apple' in fruits.keys(): print('Apple is present in the dictionary')
The 'values' View
The 'values' view provides a dynamic view of the values present in a dictionary. It allows you to iterate over the values or perform set operations like intersection, union, and difference. Here's an example of how to use the 'values' view:
fruits = {'apple': 'red', 'banana': 'yellow', 'orange': 'orange'} # Iterate over the values for value in fruits.values(): print(value) # Check if a value exists if 'red' in fruits.values(): print('A fruit with red color is present in the dictionary')
The 'items' View
The 'items' view provides a dynamic view of the key-value pairs present in a dictionary. It allows you to iterate over the key-value pairs or perform set operations like intersection, union, and difference. Here's an example of how to use the 'items' view:
fruits = {'apple': 'red', 'banana': 'yellow', 'orange': 'orange'} # Iterate over the key-value pairs for key, value in fruits.items(): print(key, value) # Check if a key-value pair exists if ('apple', 'red') in fruits.items(): print('An apple with red color is present in the dictionary')
Using Dictionary Comprehensions
Python provides a concise and powerful way to create dictionaries using dictionary comprehensions. Similar to list comprehensions, dictionary comprehensions allow you to create dictionaries in a single line of code, making your code more readable and efficient.
The basic structure of a dictionary comprehension is:
{key_expression: value_expression for item in iterable}
Here, the key_expression
is evaluated for each item in the iterable, and the result is used as the key in the dictionary. Similarly, the value_expression
is evaluated for each item and used as the corresponding value in the dictionary.
Let's say we have a list of numbers and we want to create a dictionary where each number is the key and its square is the value:
numbers = [1, 2, 3, 4, 5] squared_dict = {num: num**2 for num in numbers} print(squared_dict)
Output:
{1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
In this example, the key expression num
is each number in the list numbers
, and the value expression num**2
calculates the square of each number.
You can also add conditions to filter items in the iterable. For example, let's create a dictionary where the keys are the even numbers from 1 to 10 and the values are their squares:
even_dict = {num: num**2 for num in range(1, 11) if num % 2 == 0} print(even_dict)
Output:
{2: 4, 4: 16, 6: 36, 8: 64, 10: 100}
In this case, the condition num % 2 == 0
filters out odd numbers, and only even numbers are used as keys in the dictionary.
You can also use dictionary comprehensions to transform an existing dictionary. Let's say we have a dictionary where the keys are names and the values are ages, and we want to create a new dictionary where the keys are ages and the values are lists of names:
people = {'Alice': 25, 'Bob': 30, 'Charlie': 35} age_dict = {age: [name for name, age in people.items() if age == age] for name, age in people.items()} print(age_dict)
Output:
{25: ['Alice'], 30: ['Bob'], 35: ['Charlie']}
In this example, we iterate over the items in the people
dictionary, and for each name and age pair, we check if the age matches the current key. If it does, we add the name to the corresponding list in the new dictionary.
Dictionary comprehensions are a powerful tool in Python for quickly creating dictionaries based on existing data or transforming data into a different structure. They can make your code more concise and easier to read, especially when dealing with complex data transformations.
To learn more about dictionary comprehensions, you can refer to the official Python documentation on dictionaries.
Related Article: How To Read JSON From a File In Python
Customizing Iteration Order with 'collections.OrderedDict'
Python dictionaries are unordered collections of key-value pairs. This means that when you iterate through a dictionary, you cannot guarantee the order in which the items will be returned. However, there may be situations where you need to iterate through a dictionary in a specific order.
To address this issue, Python provides the collections.OrderedDict
class, which is a subclass of the built-in dict
class. OrderedDict
maintains the order of items as they are added and allows you to customize the iteration order.
To use OrderedDict
, you need to import it from the collections
module:
from collections import OrderedDict
You can create an empty OrderedDict
using the following syntax:
ordered_dict = OrderedDict()
To add items to the OrderedDict
, you can use the same syntax as a regular dictionary:
ordered_dict['key1'] = 'value1' ordered_dict['key2'] = 'value2' ordered_dict['key3'] = 'value3'
When you iterate through an OrderedDict
, the items will be returned in the order they were added:
for key, value in ordered_dict.items(): print(key, value)
Output:
key1 value1 key2 value2 key3 value3
You can also customize the iteration order of an OrderedDict
using the move_to_end()
method. This method moves an existing key to either end of the dictionary, effectively changing its position in the iteration order. The default position is the end of the dictionary.
Here's an example:
ordered_dict = OrderedDict([('key1', 'value1'), ('key2', 'value2'), ('key3', 'value3')]) # Move 'key2' to the end ordered_dict.move_to_end('key2') for key, value in ordered_dict.items(): print(key, value)
Output:
key1 value1 key3 value3 key2 value2
In the example above, the key-value pair ('key2', 'value2')
is moved to the end of the OrderedDict
, changing the iteration order.
Using collections.OrderedDict
allows you to have control over the iteration order of dictionary items. This can be useful in scenarios where the order of items matters, such as when you need to process data in a specific sequence.
For more information on collections.OrderedDict
, you can refer to the official Python documentation.
Iterating Through Multiple Dictionaries Simultaneously
In Python, it is common to work with multiple dictionaries simultaneously, especially when dealing with related data or performing operations that require information from multiple sources. This chapter will guide you through different methods to iterate through multiple dictionaries at once.
Method 1: Using the zip() function
The zip() function allows you to combine multiple iterables, such as dictionaries, into a single iterable. By using this function, you can iterate through the dictionaries simultaneously.
Here's an example to illustrate this:
dict1 = {'name': 'John', 'age': 30, 'country': 'USA'} dict2 = {'name': 'Jane', 'age': 25, 'country': 'Canada'} for key1, key2 in zip(dict1, dict2): print(key1, dict1[key1], key2, dict2[key2])
Output:
name John name Jane age 30 age 25 country USA country Canada
In the above code snippet, we iterate through the keys of both dictionaries using the zip() function. We then print the key-value pairs for each corresponding key.
Method 2: Using the items() method
The items() method returns a view object that contains the key-value pairs of a dictionary. By using this method on multiple dictionaries, you can iterate through their key-value pairs simultaneously.
Here's an example:
dict1 = {'name': 'John', 'age': 30, 'country': 'USA'} dict2 = {'name': 'Jane', 'age': 25, 'country': 'Canada'} for (key1, value1), (key2, value2) in zip(dict1.items(), dict2.items()): print(key1, value1, key2, value2)
Output:
name John name Jane age 30 age 25 country USA country Canada
In the above code snippet, we use the items() method on both dictionaries to get their key-value pairs. We then iterate through these pairs using the zip() function and print them accordingly.
Method 3: Using the zip_longest() function
The zip_longest() function from the itertools module is useful when you have dictionaries with different lengths. It fills in missing values with a specified fill value, allowing you to iterate through them simultaneously.
Here's an example:
from itertools import zip_longest dict1 = {'name': 'John', 'age': 30, 'country': 'USA'} dict2 = {'name': 'Jane', 'age': 25} for key1, key2 in zip_longest(dict1, dict2, fillvalue=''): print(key1, dict1.get(key1, ''), key2, dict2.get(key2, ''))
Output:
name John name Jane age 30 age 25 country USA
In the above code snippet, we use the zip_longest() function instead of zip() to handle the dictionaries with different lengths. We also use the get() method to retrieve values from the dictionaries, which allows us to handle missing keys gracefully.
Handling Large Dictionaries: Techniques for Memory Efficiency
Working with large dictionaries in Python can sometimes pose memory efficiency challenges. As dictionaries grow in size, the amount of memory required to store them increases. This can lead to performance issues and even cause your program to run out of memory. In this section, we will explore techniques for handling large dictionaries in a memory-efficient manner.
1. Use Generators
One way to handle large dictionaries is to use generators. Generators allow you to iterate over the items of a dictionary without loading the entire dictionary into memory. Instead, items are generated on-the-fly as you iterate over them, which can significantly reduce memory usage.
Here's an example that demonstrates how to use a generator to iterate over a large dictionary:
def dict_generator(d): for key, value in d.items(): yield key, value # Usage example: large_dict = {i: i * i for i in range(1, 10000001)} # A large dictionary for key, value in dict_generator(large_dict): print(f"Key: {key}, Value: {value}") ...
By using a generator, you can process each item of the dictionary one at a time, without loading the entire dictionary into memory.
Related Article: How to Flatten a List of Lists in Python
2. Use Itertools
The itertools
module in Python provides a set of tools for working with iterators. It includes functions that can help you iterate over large dictionaries efficiently.
One such function is islice
, which allows you to iterate over a dictionary in chunks. By specifying the start and stop positions, you can limit the number of items loaded into memory at once. This can be particularly useful when working with very large dictionaries.
Here's an example that demonstrates how to use islice
to iterate over a large dictionary in chunks:
import itertools large_dict = {...} # large dictionary # Using islice to iterate over the dictionary in chunks of 100 items for key, value in itertools.islice(large_dict.items(), 0, 100): # Do something with the key and value ...
By using islice
, you can process the dictionary in smaller batches, reducing memory usage and improving performance.
3. Consider Using Databases
If your dictionary is extremely large and memory efficiency is critical, you might consider using a database to store and retrieve the data. Databases are designed to handle large datasets efficiently and provide features such as indexing and querying.
Python provides several database libraries, including SQLite, PostgreSQL, and MySQL. You can choose the one that best fits your requirements and integrate it into your code to handle large dictionaries.
Here's an example that demonstrates how to use the SQLite database to store and retrieve items from a large dictionary:
import sqlite3 # Connect to the SQLite database conn = sqlite3.connect('large_dict.db') # Create a table to store the dictionary items conn.execute('''CREATE TABLE IF NOT EXISTS large_dict (key TEXT PRIMARY KEY, value TEXT)''') # Insert items into the table for key, value in large_dict.items(): conn.execute("INSERT INTO large_dict VALUES (?, ?)", (key, value)) # Retrieve items from the table cursor = conn.execute("SELECT key, value FROM large_dict") for key, value in cursor: # Do something with the key and value ... # Close the database connection conn.close()
By using a database, you can offload the memory-intensive operations to the database engine and efficiently handle large dictionaries.
4. Be Mindful of Memory Usage
In addition to the techniques mentioned above, it is important to be mindful of your code's memory usage when working with large dictionaries. Avoid unnecessary duplication of data and regularly clean up unused objects to free up memory.
Here are some general tips for reducing memory usage when working with large dictionaries:
- Delete unnecessary variables or objects when they are no longer needed.
- Use efficient data structures, such as sets or lists, instead of dictionaries when appropriate.
- Consider using memory-mapped files or other memory-efficient techniques for handling large datasets.
By following these tips and employing the appropriate techniques, you can effectively handle large dictionaries in a memory-efficient manner.
To learn more about memory management in Python, you can refer to the official Python documentation on sys.getsizeof
and garbage collection.
Now that you have learned various techniques for handling large dictionaries in a memory-efficient manner, you can confidently work with large datasets without worrying about memory constraints.