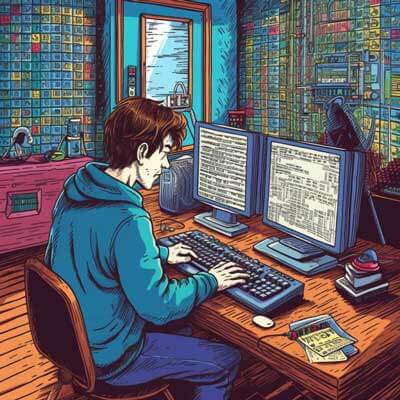
Concatenating lists in Python allows you to combine two or more lists into a single list. This can be useful in various situations, such as merging data from multiple sources or combining the results of different operations. In this guide, we will explore different approaches to concatenate lists in Python and provide examples of best practices.
Why is this question asked?
The question of how to concatenate lists in Python is common because it is a fundamental operation in many programming tasks. The ability to combine lists is important for manipulating and organizing data efficiently. Understanding how to concatenate lists in Python allows developers to write cleaner and more concise code.
Related Article: How To Convert a Dictionary To JSON In Python
Possible Answers
1. Using the ‘+’ Operator
In Python, you can use the ‘+’ operator to concatenate two lists. This operator combines the elements of the lists in the order they appear. Here’s an example:
list1 = [1, 2, 3] list2 = [4, 5, 6] concatenated_list = list1 + list2 print(concatenated_list)
Output:
[1, 2, 3, 4, 5, 6]
In this example, we first define two lists, list1
and list2
. We then use the ‘+’ operator to concatenate the two lists into concatenated_list
. Finally, we print the concatenated list to the console.
2. Using the extend() Method
Python lists have a built-in method called extend()
that can be used to concatenate two or more lists. This method takes an iterable as an argument and appends its elements to the original list. Here’s an example:
list1 = [1, 2, 3] list2 = [4, 5, 6] list1.extend(list2) print(list1)
Output:
[1, 2, 3, 4, 5, 6]
In this example, we use the extend()
method to concatenate list2
to list1
. The elements of list2
are appended to list1
, resulting in a single concatenated list.
Best Practices
When concatenating lists in Python, it is important to keep a few best practices in mind:
– Use the ‘+’ operator when you want to create a new list that combines the elements of two or more existing lists. This approach is more concise and easier to read.
– Use the extend()
method when you want to modify the original list by adding elements from another list. This method is useful when you want to avoid creating a new list and instead update an existing list in-place.
– If you need to concatenate more than two lists, you can chain the ‘+’ operator or extend()
method. For example:
list1 = [1, 2, 3] list2 = [4, 5, 6] list3 = [7, 8, 9] concatenated_list = list1 + list2 + list3 print(concatenated_list)
Output:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
In this example, we use the ‘+’ operator to concatenate three lists, list1
, list2
, and list3
, into a single concatenated_list
.
Alternative Ideas
While the methods described above are commonly used for concatenating lists in Python, there are alternative approaches worth considering:
– Using the +=
operator: The +=
operator can be used as a shorthand for the extend()
method. It performs the same operation of appending elements from one list to another. Here’s an example:
list1 = [1, 2, 3] list2 = [4, 5, 6] list1 += list2 print(list1)
Output:
[1, 2, 3, 4, 5, 6]
In this example, we use the +=
operator to concatenate list2
to list1
. The elements of list2
are appended to list1
, resulting in a single concatenated list.
– Using list comprehension: List comprehension is a powerful feature in Python that allows you to create new lists based on existing lists. It can also be used to concatenate lists. Here’s an example:
list1 = [1, 2, 3] list2 = [4, 5, 6] concatenated_list = [x for x in [list1, list2]] print(concatenated_list)
Output:
[[1, 2, 3], [4, 5, 6]]
In this example, we use list comprehension to create a new list concatenated_list
that contains the two input lists, list1
and list2
.
While list comprehension can be a useful tool for various list operations, it may not be the most intuitive or efficient approach for concatenating lists. It is generally recommended to use the ‘+’ operator or extend()
method for concatenation, as they are more straightforward and performant.
Related Article: How to Sort a Dictionary by Key in Python