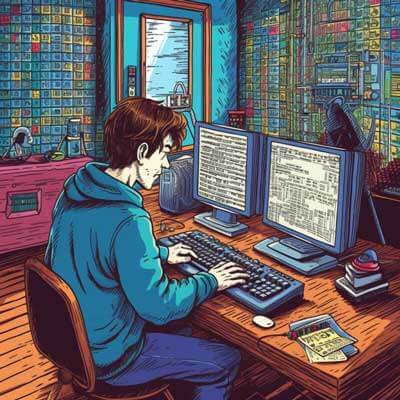
Table of Contents
To convert a tensor to a NumPy array in TensorFlow, you can use the numpy()
method. This method allows you to extract the values from a tensor and convert them into a NumPy array, which can then be further processed or used in other Python libraries. Here are two possible ways to convert a tensor to a NumPy array in TensorFlow:
Method 1: Using the numpy()
method
One straightforward way to convert a tensor to a NumPy array is by using the numpy()
method. This method is available for TensorFlow tensors and returns a NumPy array with the same shape and values as the original tensor. Here's an example:
import tensorflow as tf import numpy as np # Create a TensorFlow tensor tensor = tf.constant([[1, 2, 3], [4, 5, 6]]) # Convert the tensor to a NumPy array numpy_array = tensor.numpy() # Print the NumPy array print(numpy_array)
Output:
array([[1, 2, 3], [4, 5, 6]])
In this example, we create a TensorFlow tensor using the tf.constant()
function. Then, we use the numpy()
method to convert the tensor to a NumPy array. Finally, we print the NumPy array to verify the conversion.
Related Article: How to Create and Fill an Empty Pandas DataFrame in Python
Method 2: Using the eval()
method
Another way to convert a tensor to a NumPy array is by using the eval()
method. This method is available for TensorFlow tensors and allows you to evaluate the tensor in a TensorFlow session and retrieve its value as a NumPy array. Here's an example:
import tensorflow as tf import numpy as np # Create a TensorFlow tensor tensor = tf.constant([[1, 2, 3], [4, 5, 6]]) # Start a TensorFlow session with tf.Session() as sess: # Evaluate the tensor and convert it to a NumPy array numpy_array = tensor.eval() # Print the NumPy array print(numpy_array)
Output:
array([[1, 2, 3], [4, 5, 6]])
In this example, we create a TensorFlow tensor using the tf.constant()
function. Then, we start a TensorFlow session using the tf.Session()
context manager. Inside the session, we use the eval()
method to evaluate the tensor and convert it to a NumPy array. Finally, we print the NumPy array to verify the conversion.
Best Practices
Related Article: How to Plot a Histogram in Python Using Matplotlib with List Data
When converting a tensor to a NumPy array in TensorFlow, keep the following best practices in mind:
1. Make sure to have TensorFlow and NumPy installed in your Python environment. You can install them using pip
:
pip install tensorflow numpy
2. Use the numpy()
method whenever possible, as it is a more concise and efficient way to convert a tensor to a NumPy array.
3. If you need to perform additional operations on the tensor before conversion, consider using TensorFlow's built-in functions and operations instead of converting to a NumPy array prematurely. This can help maintain better performance and compatibility with TensorFlow's computational graph.
4. Be mindful of the memory usage when working with large tensors. Converting a tensor to a NumPy array creates a copy of the data in memory. If memory is a concern, consider manipulating the tensor directly using TensorFlow operations or using TensorFlow's streaming capabilities.