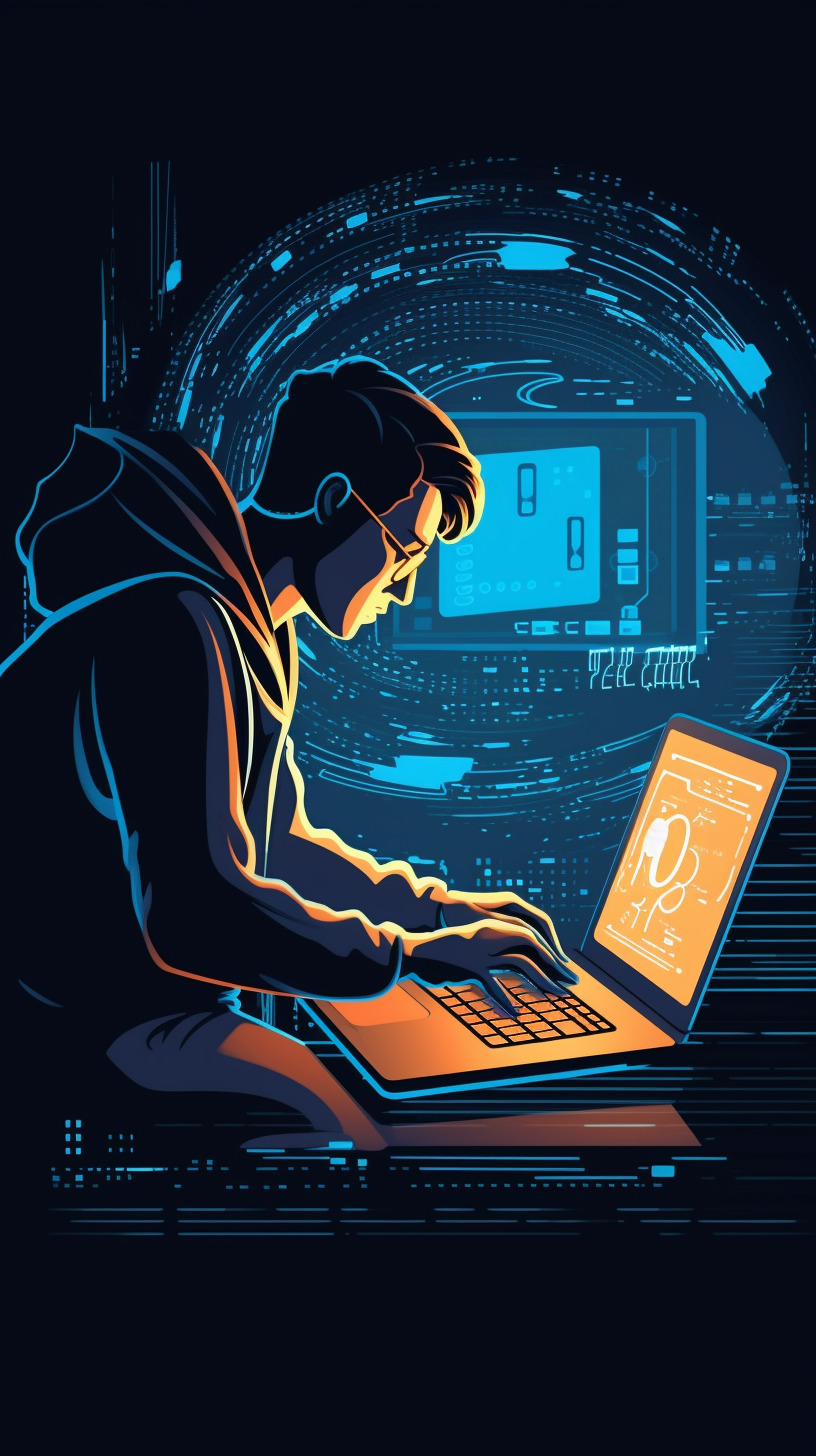
- Getting Started: Basics of Creating, Accessing, and Modifying Lists
- Creating Lists
- Accessing List Items
- Modifying Lists
- Slicing Lists
- Modifying Slices
- Stepping through Lists
- Negative Indexing
- Expand and Modify: Add, Remove, and Manipulate List Elements
- Adding Elements to a List
- Removing Elements from a List
- Modifying List Elements
- Sorting Lists
- Sorting and Reversing
- Sorting Lists
- Reversing Lists
- Sorting Lists of Dictionaries
- List Comprehensions
- Basic List Comprehensions
- Filtering with List Comprehensions
- Nested List Comprehensions
- Nested Lists: Multi-dimensional Arrays
- Creating Nested Lists
- Accessing Nested Lists
- Modifying Nested Lists
- Looping Through Lists
- For Loops
- List Comprehensions
- Enumerate
- Lists and Arrays: Choosing the Right Data Structure
- Lists
- Arrays
- Choosing the Right Data Structure
- Real-Life Applications: Lists in Data Analysis and Visualization
- Storing Data
- Filtering Data
- Visualizing Data
- Practical Examples: Putting Lists to Work in Various Scenarios
- Data Processing
- Web Scraping
- Sorting and Searching
- Advanced Techniques: Generators, Itertools, and Functional Programming for Lists
- Generators
- Itertools
- Functional Programming
- Handling Exceptions: Tackling Errors in List Operations
- Index Errors
- Value Errors
- Type Errors
- Use the Right Data Structure
- Memory Errors
- Performance Optimization: Tips and Tricks for Faster List Manipulation
- Use List Comprehensions
- Use Generators
- Use Built-in Functions
- Best Practices: Writing Clean and Maintainable List Code
- Use Descriptive Variable Names
- Avoid Magic Numbers
- Use List Comprehensions
- Avoid Nested Lists
- Exploring NumPy Arrays
- Creating NumPy Arrays
- Advantages of NumPy Arrays
- Broadcasting and Vectorization
- Advanced Array Manipulation Techniques
- Reshaping Arrays
- Transposing Arrays
- Flattening Arrays
- Concatenating Arrays
- Thinking Outside the List and Array Boundaries
- Dictionaries
- Sets
- Pandas DataFrames
Getting Started: Basics of Creating, Accessing, and Modifying Lists
Python lists are an important data structure used for storing and manipulating a collection of items. We will discuss the basics of creating, accessing, and modifying lists.
Related Article: How To Convert a Dictionary To JSON In Python
Creating Lists
To create a list in Python, simply enclose a comma-separated sequence of values within square brackets. For example, to create a list of integers from 1 to 5, we can write:
my_list = [1, 2, 3, 4, 5]
We can also create a list of strings, like this:
my_list = ['apple', 'banana', 'cherry']
Accessing List Items
We can access individual items in a list using the index operator []
. The index starts at 0 for the first item in the list and increases by 1 for each subsequent item. For example, to access the first item in my_list
, we can write:
first_item = my_list[0]
We can also access the last item in the list using a negative index. For example, to access the last item in my_list
, we can write:
last_item = my_list[-1]
Modifying Lists
We can modify the items in a list using the index operator []
. For example, to change the second item in my_list
to 'orange'
, we can write:
my_list[1] = 'orange'
We can also append new items to the end of a list using the append()
method. For example, to add the item 'pear'
to the end of my_list
, we can write:
my_list.append('pear')
Python lists are a powerful data structure used for storing and manipulating collections of items. By understanding the basics of creating, accessing, and modifying lists, you will be well-equipped to handle more complex tasks involving lists in Python.assistant:
Slice and Dice: Mastering List Operations
Slicing is one of the most powerful operations you can perform on a list in Python. It allows you to extract a portion of a list, which can be useful for a wide variety of tasks. We will discuss how to slice and dice lists in Python.
Related Article: How to Sort a Dictionary by Key in Python
Slicing Lists
To slice a list in Python, you use the colon :
operator. The syntax for slicing is as follows:
my_list[start:end:step]
where start
is the starting index, end
is the ending index, and step
is the step size. If you omit any of these values, Python will assume the default values of start=0
, end=len(my_list)
, and step=1
.
For example, to extract the second through fifth items in a list, we can write:
my_list = [1, 2, 3, 4, 5] my_slice = my_list[1:5]
This will create a new list containing the values [2, 3, 4, 5]
.
Modifying Slices
You can also modify slices of a list in Python. For example, to replace the second through fourth items in a list with a new list, we can write:
my_list = [1, 2, 3, 4, 5] my_list[1:4] = [10, 20, 30]
This will modify my_list
to be [1, 10, 20, 30, 5]
.
Stepping through Lists
In addition to slicing, you can also step through a list using the step
value. For example, to extract every other item in a list, we can write:
my_list = [1, 2, 3, 4, 5] my_slice = my_list[::2]
This will create a new list containing the values [1, 3, 5]
.
Related Article: How to Remove a Key from a Python Dictionary
Negative Indexing
You can also use negative indexing to slice a list. For example, to extract the last three items in a list, we can write:
my_list = [1, 2, 3, 4, 5] my_slice = my_list[-3:]
This will create a new list containing the values [3, 4, 5]
.
Slicing and dicing lists is a powerful technique that can be used for a wide variety of tasks. By mastering the art of list operations in Python, you will be well-equipped to handle complex data manipulation tasks with ease.
Expand and Modify: Add, Remove, and Manipulate List Elements
Lists in Python are mutable, which means that you can add, remove, and manipulate elements in a list.We will discuss how to expand and modify lists in Python.
Adding Elements to a List
You can add elements to a list using the append()
method. For example, to add a new item to the end of a list, we can write:
my_list = [1, 2, 3] my_list.append(4)
This will modify my_list
to be [1, 2, 3, 4]
.
You can also insert elements at a specific position in the list using the insert()
method. For example, to insert a new item at the second position in my_list
, we can write:
my_list = [1, 2, 3] my_list.insert(1, 4)
This will modify my_list
to be [1, 4, 2, 3]
.
Related Article: How to Remove an Element from a List by Index in Python
Removing Elements from a List
You can remove elements from a list using the remove()
method. For example, to remove the item 2
from my_list
, we can write:
my_list = [1, 2, 3] my_list.remove(2)
This will modify my_list
to be [1, 3]
.
You can also use the pop()
method to remove an element at a specific position in the list. For example, to remove the second item in my_list
, we can write:
my_list = [1, 2, 3] my_list.pop(1)
This will modify my_list
to be [1, 3]
.
Modifying List Elements
You can modify elements in a list using the index operator []
. For example, to change the second item in my_list
to 10
, we can write:
my_list = [1, 2, 3] my_list[1] = 10
This will modify my_list
to be [1, 10, 3]
.
Sorting Lists
You can sort a list using the sort()
method. For example, to sort my_list
in ascending order, we can write:
my_list = [3, 1, 2] my_list.sort()
This will modify my_list
to be [1, 2, 3]
.
You can also sort a list in descending order by passing the reverse=True
argument to the sort()
method:
my_list = [3, 1, 2] my_list.sort(reverse=True)
This will modify my_list
to be [3, 2, 1]
.
Adding, removing, and manipulating elements in a list are important operations in Python. By mastering these techniques, you will be able to handle a wide variety of tasks involving lists with ease.
Related Article: How to Solve a Key Error in Python
Sorting and Reversing
Sorting and reversing are common operations in data manipulation tasks. Python provides built-in functions for sorting and reversing lists, which we will discuss in this section.
Sorting Lists
To sort a list in Python, you can use the sorted()
function. For example, to sort a list of integers in ascending order, we can write:
my_list = [3, 1, 4, 2] sorted_list = sorted(my_list)
This will create a new list called sorted_list
containing the values [1, 2, 3, 4]
.
You can also sort a list in descending order by passing the reverse=True
argument to the sorted()
function:
my_list = [3, 1, 4, 2] reverse_sorted_list = sorted(my_list, reverse=True)
This will create a new list called reverse_sorted_list
containing the values [4, 3, 2, 1]
.
If you want to sort the list in place (i.e., without creating a new list), you can use the sort()
method:
my_list = [3, 1, 4, 2] my_list.sort()
This will modify my_list
to be [1, 2, 3, 4]
.
Reversing Lists
To reverse a list in Python, you can use the reversed()
function. For example, to reverse a list of integers, we can write:
my_list = [1, 2, 3, 4] reversed_list = list(reversed(my_list))
This will create a new list called reversed_list
containing the values [4, 3, 2, 1]
.
If you want to reverse the list in place, you can use the reverse()
method:
my_list = [1, 2, 3, 4] my_list.reverse()
This will modify my_list
to be [4, 3, 2, 1]
.
Related Article: How to Add New Keys to a Python Dictionary
Sorting Lists of Dictionaries
Sorting lists of dictionaries in Python can be a bit more complex than sorting simple lists. To sort a list of dictionaries by a specific key, you can use the key
argument of the sorted()
function. For example, to sort a list of dictionaries by the "age"
key in ascending order, we can write:
my_list = [{"name": "Alice", "age": 25}, {"name": "Bob", "age": 30}, {"name": "Charlie", "age": 20}] sorted_list = sorted(my_list, key=lambda x: x["age"])
This will create a new list called sorted_list
containing the dictionaries sorted by the "age"
key in ascending order.
Sorting and reversing lists are important techniques that can be used to organize your data like a pro. By mastering these techniques, you will be able to handle a wide variety of data manipulation tasks with ease.
List Comprehensions
List comprehensions are a concise way to create new lists based on existing lists. They can be used to perform complex data transformations in a compact and readable way. We will explore how list comprehensions work and how they can be used to manipulate lists in Python.
Basic List Comprehensions
The basic syntax of a list comprehension in Python is as follows:
new_list = [expression for item in iterable]
This creates a new list called new_list
by applying the expression
to each item
in the iterable
. For example, to create a new list containing the squares of the numbers from 1 to 5, we can write:
squares = [x**2 for x in range(1, 6)]
This will create a new list called squares
containing the values [1, 4, 9, 16, 25]
.
Related Article: How to Read a File Line by Line into a List in Python
Filtering with List Comprehensions
List comprehensions can also be used to filter elements from an existing list. The basic syntax is as follows:
new_list = [expression for item in iterable if condition]
This creates a new list called new_list
by applying the expression
to each item
in the iterable
that satisfies the condition
. For example, to create a new list containing only the even numbers from 1 to 10, we can write:
even_numbers = [x for x in range(1, 11) if x % 2 == 0]
This will create a new list called even_numbers
containing the values [2, 4, 6, 8, 10]
.
Nested List Comprehensions
List comprehensions can also be nested within one another to perform more complex operations. For example, to create a new list containing the products of all pairs of elements from two existing lists, we can write:
list_1 = [1, 2, 3] list_2 = [4, 5, 6] product_list = [x * y for x in list_1 for y in list_2]
This will create a new list called product_list
containing the values [4, 5, 6, 8, 10, 12, 12, 15, 18]
.
List comprehensions are a powerful tool for manipulating lists in Python. They can be used to perform complex data transformations in a compact and readable way. By mastering list comprehensions, you will be able to write more efficient and elegant code.
Nested Lists: Multi-dimensional Arrays
In Python, lists can contain other lists, which allows us to create multi-dimensional arrays. These are also known as nested lists. We will explore how to create and manipulate nested lists.
Related Article: How to Check If Something Is Not In A Python List
Creating Nested Lists
To create a nested list, we simply include another list as an item in the outer list. For example, to create a 2-dimensional array containing the numbers from 1 to 9, we can write:
array_2d = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
This will create a nested list called array_2d
containing the values [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
.
Similarly, we can create a 3-dimensional array by including nested lists within nested lists. For example, to create a 3-dimensional array containing the numbers from 1 to 27, we can write:
array_3d = [[[1, 2, 3], [4, 5, 6], [7, 8, 9]], [[10, 11, 12], [13, 14, 15], [16, 17, 18]], [[19, 20, 21], [22, 23, 24], [25, 26, 27]]]
This will create a nested list called array_3d
containing the values [[[1, 2, 3], [4, 5, 6], [7, 8, 9]], [[10, 11, 12], [13, 14, 15], [16, 17, 18]], [[19, 20, 21], [22, 23, 24], [25, 26, 27]]]
.
Accessing Nested Lists
To access an element in a nested list, we use the same indexing syntax as with regular lists, but we need to specify the index for each level of nesting. For example, to access the value 5
in the array_2d
list we created earlier, we can write:
value = array_2d[1][1]
This will assign the value 5
to the variable value
.
Similarly, to access the value 14
in the array_3d
list we created earlier, we can write:
value = array_3d[1][1][1]
This will assign the value 14
to the variable value
.
Modifying Nested Lists
To modify an element in a nested list, we use the same syntax as with regular lists. For example, to change the value 5
in the array_2d
list to 10
, we can write:
array_2d[1][1] = 10
This will change the value of the element at index [1][1]
from 5
to 10
.
Similarly, to change the value 14
in the array_3d
list to 20
, we can write:
array_3d[1][1][1] = 20
This will change the value of the element at index [1][1][1]
from 14
to 20
.
Nested lists allow us to create multi-dimensional arrays in Python. We can access and modify elements in nested lists using the same indexing syntax as with regular lists. By understanding how to create and manipulate nested lists, we can work with more complex data structures in Python.
Related Article: How to Pretty Print Nested Dictionaries in Python
Looping Through Lists
One of the most common operations we perform on lists is to iterate over their elements. We will explore different techniques for iterating over lists in Python.
For Loops
The most common way to iterate over a list is to use a for
loop. The syntax for a for
loop in Python is as follows:
for item in list: # do something with item
In this syntax, item
is a variable that takes on the value of each element in the list, one at a time, and the indented block of code is executed for each element.
For example, let’s say we have a list of numbers and we want to calculate their sum. We can use a for
loop to iterate over the list and accumulate the sum as follows:
numbers = [1, 2, 3, 4, 5] total = 0 for num in numbers: total += num print(total) # Output: 15
List Comprehensions
List comprehensions are a concise way to create lists by iterating over another list. The syntax for a list comprehension in Python is as follows:
new_list = [expression for item in list]
In this syntax, expression
is a computation that is performed on each element of the list, and item
is a variable that takes on the value of each element in the list, one at a time.
For example, let’s say we have a list of numbers and we want to create a new list containing their squares. We can use a list comprehension as follows:
numbers = [1, 2, 3, 4, 5] squares = [num**2 for num in numbers] print(squares) # Output: [1, 4, 9, 16, 25]
Related Article: How to Find a Value in a Python List
Enumerate
Sometimes it is useful to iterate over a list and also have access to the index of each element. We can achieve this using the enumerate
function in Python. The syntax for using enumerate
is as follows:
for index, item in enumerate(list): # do something with index and item
In this syntax, index
is a variable that takes on the value of the index of each element in the list, and item
is a variable that takes on the value of each element in the list, one at a time.
For example, let’s say we have a list of names and we want to print each name along with its index. We can use enumerate
as follows:
names = ['Alice', 'Bob', 'Charlie'] for index, name in enumerate(names): print(f'{index}: {name}') # Output: # 0: Alice # 1: Bob # 2: Charlie
There are several techniques we can use to iterate over lists in Python, including for
loops, list comprehensions, and enumerate
. By understanding these techniques, we can efficiently process lists and perform complex operations on their elements.
Lists and Arrays: Choosing the Right Data Structure
In Python, we have two main data structures for storing collections of values: lists and arrays. While both data structures can store collections of values, they have different performance characteristics and are optimized for different use cases. We will explore the differences between lists and arrays and when to use each one.
Lists
Lists are dynamic arrays that can grow and shrink as needed. They can store values of any data type and can be nested to create more complex data structures. Lists are very flexible and easy to use, making them a good choice for most general-purpose programming tasks.
For example, let’s say we want to store the heights of a group of people in a list. We can create a list of integers as follows:
heights = [68, 72, 64, 70, 76]
We can then use list methods to manipulate the list as needed. For example, we can append a new height to the list as follows:
heights.append(74)
Related Article: How to Extract Unique Values from a List in Python
Arrays
Arrays are fixed-size collections of values that are optimized for numerical operations. They are implemented in Python as part of the NumPy library, which provides a wide range of numerical operations on arrays. Arrays are faster and more memory-efficient than lists for numerical computations, making them a good choice for scientific computing and data analysis.
For example, let’s say we want to perform some numerical operations on a collection of values. We can create an array of numbers as follows:
import numpy as np numbers = np.array([1, 2, 3, 4, 5])
We can then use NumPy functions to perform numerical operations on the array. For example, we can calculate the mean of the array as follows:
mean = np.mean(numbers)
Choosing the Right Data Structure
When choosing between lists and arrays, it is important to consider the specific needs of your program. If you need a flexible data structure that can store values of any data type, use a list. If you need a data structure that is optimized for numerical computations and operates on large datasets, use an array.
In general, if you are working with numerical data, you should use arrays. If you are working with non-numerical data, lists are usually the better choice. However, there are exceptions to this rule, so it is important to understand the trade-offs between the two data structures and choose the one that best fits your specific needs.
Lists and arrays are both useful data structures in Python, but they have different performance characteristics and are optimized for different use cases. By understanding the differences between the two data structures, we can choose the best one for our specific needs and optimize the performance of our programs.
Real-Life Applications: Lists in Data Analysis and Visualization
Lists are essential in data analysis and visualization. They allow us to store, manipulate, and display data in a structured and organized way. We will explore some of the most common use cases for lists in data analysis and visualization.
Related Article: How to Remove Duplicates From Lists in Python
Storing Data
Lists are an excellent way to store data in Python. They allow us to group related data together and access it easily. For example, suppose we have a dataset of customer orders from an online store. We could store this data in a list of dictionaries, where each dictionary represents a single order:
orders = [ {'id': 1, 'customer': 'Alice', 'product': 'Widget', 'price': 10.99}, {'id': 2, 'customer': 'Bob', 'product': 'Gizmo', 'price': 4.99}, {'id': 3, 'customer': 'Charlie', 'product': 'Widget', 'price': 7.99}, {'id': 4, 'customer': 'Alice', 'product': 'Thingamajig', 'price': 3.99}, ]
We can then easily access individual orders or perform operations on the entire dataset. For example, we could calculate the total revenue for all orders:
total_revenue = sum(order['price'] for order in orders)
Filtering Data
Lists are also useful for filtering data. Suppose we want to find all orders where the customer is ‘Alice’. We can use a list comprehension to create a new list containing only the matching orders:
alice_orders = [order for order in orders if order['customer'] == 'Alice']
We can then perform further analysis on this filtered dataset, such as calculating the average order price for Alice:
alice_avg_price = sum(order['price'] for order in alice_orders) / len(alice_orders)
Visualizing Data
Lists are essential for data visualization, as they allow us to represent data in a structured and organized way. For example, suppose we want to create a bar chart showing the total revenue for each product. We could use our list of orders to create a dictionary mapping each product to its total revenue:
revenue_by_product = {} for order in orders: if order['product'] not in revenue_by_product: revenue_by_product[order['product']] = 0 revenue_by_product[order['product']] += order['price']
We can then use a plotting library such as Matplotlib to create a bar chart:
import matplotlib.pyplot as plt plt.bar(revenue_by_product.keys(), revenue_by_product.values()) plt.show()
Related Article: How to Compare Two Lists in Python and Return Matches
Practical Examples: Putting Lists to Work in Various Scenarios
We will explore some practical examples of using lists in various scenarios. We will cover examples of data processing, web scraping, and more.
Data Processing
Lists are an excellent tool for data processing tasks. They allow us to store and manipulate large amounts of data efficiently. For example, suppose we have a CSV file containing a list of employee salaries. We could load this data into a list of dictionaries using the csv
module:
import csv with open('salaries.csv') as f: reader = csv.DictReader(f) salaries = [row for row in reader]
We can then perform various operations on this data using list comprehensions. For example, we could calculate the average salary:
avg_salary = sum(float(row['salary']) for row in salaries) / len(salaries)
Web Scraping
Lists are also useful for web scraping tasks. They allow us to store and manipulate large amounts of data extracted from web pages. For example, suppose we want to extract a list of book titles and prices from a web page. We could use the requests
and beautifulsoup4
modules to download and parse the HTML:
import requests from bs4 import BeautifulSoup url = 'https://www.example.com/books' response = requests.get(url) soup = BeautifulSoup(response.content, 'html.parser')
We can then extract the relevant data using list comprehensions:
book_titles = [a.text for a in soup.find_all('a', class_='book-title')] book_prices = [float(span.text.strip('$')) for span in soup.find_all('span', class_='book-price')]
Related Article: How to Print a Python Dictionary Line by Line
Sorting and Searching
Lists are also useful for sorting and searching tasks. They allow us to quickly find and manipulate data based on specific criteria. For example, suppose we have a list of student grades:
grades = [87, 92, 78, 95, 84, 91, 76, 89]
We could sort this list in descending order using the sort()
method:
grades.sort(reverse=True)
We can then find the top three grades using list slicing:
top_three_grades = grades[:3]
Advanced Techniques: Generators, Itertools, and Functional Programming for Lists
We will explore some advanced techniques for working with Python lists. We will cover generators, itertools, and functional programming.
Generators
Generators are a powerful way to work with lists in Python. They allow us to create sequences of values on the fly, without needing to store them in memory. This can be particularly useful when working with large datasets or when we only need to process a subset of the data.
For example, suppose we have a list of numbers and we want to find all the even numbers. We could use a list comprehension to create a new list containing only the even numbers:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] even_numbers = [n for n in numbers if n % 2 == 0]
However, if the list is very large, this could use a lot of memory. Instead, we could use a generator expression to create a sequence of even numbers:
even_numbers = (n for n in numbers if n % 2 == 0)
We can then use this generator expression in a loop or other context where we need to process the even numbers one at a time.
Related Article: How to Use Hash Map In Python
Itertools
The itertools
module provides a variety of functions for working with lists and other iterable objects. These functions allow us to perform complex operations on lists with minimal code.
For example, suppose we have a list of numbers and we want to find all the pairwise combinations of those numbers. We could use a nested loop to generate the combinations:
numbers = [1, 2, 3, 4] combinations = [] for i in range(len(numbers)): for j in range(i + 1, len(numbers)): combinations.append((numbers[i], numbers[j]))
However, this can be quite verbose. Instead, we could use the combinations()
function from the itertools
module:
import itertools numbers = [1, 2, 3, 4] combinations = list(itertools.combinations(numbers, 2))
This generates all the pairwise combinations of the numbers in a single line of code.
Functional Programming
Functional programming is a programming paradigm that emphasizes the use of functions to perform operations on data. This can be a powerful way to work with lists, as it allows us to create reusable functions that can be applied to many different lists.
For example, suppose we have a list of numbers and we want to calculate the sum of the squares of those numbers. We could use a loop to calculate the squares and then sum them:
numbers = [1, 2, 3, 4, 5] squares = [] for n in numbers: squares.append(n ** 2) sum_of_squares = sum(squares)
However, this can be quite verbose. Instead, we could use the map()
and reduce()
functions from the functools
module to perform this operation in a more functional style:
import functools numbers = [1, 2, 3, 4, 5] squares = list(map(lambda n: n ** 2, numbers)) sum_of_squares = functools.reduce(lambda x, y: x + y, squares)
This generates a list of squares using the map()
function, and then sums the squares using the reduce()
function.
Handling Exceptions: Tackling Errors in List Operations
We will discuss how to handle exceptions that can occur when working with Python lists. Exceptions are errors that occur during program execution and can cause our programs to crash if not handled properly.
Related Article: How to Detect Duplicates in a Python List
Index Errors
One common exception that can occur when working with lists is the IndexError
. This occurs when we try to access an index that is outside the range of the list. For example:
my_list = [1, 2, 3] print(my_list[3])
This will raise an IndexError
since the list my_list
only has indices 0, 1, and 2.
To handle this exception, we can use a try
/except
block:
my_list = [1, 2, 3] try: print(my_list[3]) except IndexError: print("Index out of range")
This will catch the IndexError
and print a more informative message.
Value Errors
Another common exception that can occur when working with lists is the ValueError
. This occurs when we try to perform an operation on a list that is not valid for the values in the list. For example:
my_list = [1, 2, 3] my_list.remove(4)
This will raise a ValueError
since the value 4 is not in the list.
To handle this exception, we can again use a try
/except
block:
my_list = [1, 2, 3] try: my_list.remove(4) except ValueError: print("Value not found in list")
This will catch the ValueError
and print a more informative message.
Type Errors
A third common exception that can occur when working with lists is the TypeError
. This occurs when we try to perform an operation on a list that is not valid for the type of values in the list. For example:
my_list = [1, 2, 3] sum(my_list)
This will raise a TypeError
since the sum()
function expects a list of numbers, not a list of strings.
To handle this exception, we can again use a try
/except
block:
my_list = [1, 2, 3, 'four'] try: sum(my_list) except TypeError: print("List contains non-numeric values")
This will catch the TypeError
and print a more informative message.
Related Article: How To Find Index Of Item In Python List
Use the Right Data Structure
Finally, it is important to choose the right data structure for the job. Lists are great for many things, but they are not always the best choice. For example, if you need to perform a lot of lookups or insertions in the middle of a list, a dictionary or a deque might be a better choice.
Memory Errors
Memory errors can occur when working with large lists. These occur when you try to allocate more memory than is available on your system.
For example, consider the following code:
my_list = [0] * 1000000000
This will result in a memory error, since we are trying to allocate a gigabyte of memory for our list.
To troubleshoot memory errors, make sure that you are not creating lists that are too large for your system’s memory. You can also use generators or other data structures that are more memory-efficient.
Performance Optimization: Tips and Tricks for Faster List Manipulation
We will discuss some tips and tricks for optimizing the performance of our Python list operations. By following these techniques, we can make our code more efficient and reduce the time it takes to run.
Related Article: Extracting File Names from Path in Python, Regardless of OS
Use List Comprehensions
One of the easiest ways to improve the performance of list operations is to use list comprehensions instead of traditional loops. List comprehensions are a concise way to create new lists based on existing ones, and they are often faster than using loops.
For example, consider the following loop that squares the values in a list:
my_list = [1, 2, 3, 4, 5] squared = [] for num in my_list: squared.append(num**2)
We can rewrite this using a list comprehension:
my_list = [1, 2, 3, 4, 5] squared = [num**2 for num in my_list]
This is not only more concise, but it is also faster.
Use Generators
Generators are another way to improve performance when working with lists. Generators are functions that return iterators, and they can be used to create lists on the fly without storing them in memory.
For example, consider the following code that creates a list of even numbers:
even_nums = [] for num in range(1000000): if num % 2 == 0: even_nums.append(num)
We can rewrite this using a generator:
even_nums = (num for num in range(1000000) if num % 2 == 0)
This creates a generator that generates even numbers on the fly, without storing them in memory. This can be much faster than creating a list and appending to it.
Use Built-in Functions
Python has many built-in functions that can be used to manipulate lists, and these functions are often optimized for performance. When possible, it is best to use these built-in functions instead of writing custom code.
For example, consider the following code that sums the values in a list:
my_list = [1, 2, 3, 4, 5] total = 0 for num in my_list: total += num
We can use the built-in sum()
function instead:
my_list = [1, 2, 3, 4, 5] total = sum(my_list)
This is not only more concise, but it is also faster.
Related Article: How to Flatten a List of Lists in Python
Best Practices: Writing Clean and Maintainable List Code
We will discuss some best practices for writing clean and maintainable code when working with Python lists.
Use Descriptive Variable Names
When working with lists, it’s important to use descriptive variable names that accurately reflect the purpose of the list. This makes your code more readable and understandable.
For example, instead of using a variable name like my_list
, use a name that reflects the data contained in the list, such as ages
or names
.
Avoid Magic Numbers
Magic numbers are hard-coded values that appear throughout your code. They can make your code difficult to understand and maintain.
For example, consider the following code:
my_list = [1, 2, 3, 4, 5] for i in range(5): print(my_list[i])
In this code, the number 5 is a magic number. Instead of using a hard-coded value, we can use the len()
function to get the length of the list dynamically:
my_list = [1, 2, 3, 4, 5] for i in range(len(my_list)): print(my_list[i])
Related Article: How to Define Stacks Data Structures in Python
Use List Comprehensions
List comprehensions are a concise and readable way to create lists in Python. They are often faster and more memory-efficient than traditional for loops.
For example, instead of using a for loop to create a list of squares:
squares = [] for i in range(10): squares.append(i**2)
We can use a list comprehension:
squares = [i**2 for i in range(10)]
Avoid Nested Lists
Nested lists can make your code more difficult to read and understand. Instead of using nested lists, consider using tuples or dictionaries to organize your data.
For example, instead of using a nested list of student grades:
grades = [["Alice", 80], ["Bob", 90], ["Charlie", 85]]
We can use a list of dictionaries:
grades = [{"name": "Alice", "grade": 80}, {"name": "Bob", "grade": 90}, {"name": "Charlie", "grade": 85}]
Exploring NumPy Arrays
NumPy is a powerful Python library for numerical computing that provides a high-performance multidimensional array object, as well as tools for working with these arrays. We will explore NumPy arrays and their advantages over Python lists.
Related Article: What are The Most Popular Data Structures in Python?
Creating NumPy Arrays
NumPy arrays can be created using the np.array()
function. This function takes a Python list or tuple as input and returns a NumPy array.
import numpy as np Create a NumPy array from a Python list my_array = np.array([1, 2, 3, 4, 5])
Advantages of NumPy Arrays
NumPy arrays offer several advantages over Python lists, including:
– Faster performance: NumPy arrays are designed to be more efficient than Python lists, especially for large arrays and mathematical operations.
– Multidimensional support: NumPy arrays can have multiple dimensions, making them useful for working with data that has multiple features or dimensions.
– Broadcasting: NumPy arrays can perform mathematical operations on arrays of different shapes and sizes, using a concept called broadcasting.
– Vectorization: NumPy arrays can perform element-wise operations on entire arrays, without the need for explicit loops.
Broadcasting and Vectorization
Broadcasting and vectorization are powerful features of NumPy arrays that allow for efficient element-wise operations on arrays.
For example, consider the following code for adding two Python lists element-wise:
list1 = [1, 2, 3, 4, 5] list2 = [6, 7, 8, 9, 10] result = [] for i in range(len(list1)): result.append(list1[i] + list2[i])
We can accomplish the same thing much more efficiently using NumPy arrays:
import numpy as np array1 = np.array([1, 2, 3, 4, 5]) array2 = np.array([6, 7, 8, 9, 10]) result = array1 + array2
In this code, the +
operator performs element-wise addition on the two arrays, without the need for an explicit loop.
Related Article: Python's Dict Tutorial: Is a Dictionary a Data Structure?
Advanced Array Manipulation Techniques
We will explore some advanced array manipulation techniques that can help you work with arrays more efficiently and effectively.
Reshaping Arrays
NumPy arrays can be reshaped into different shapes and sizes using the reshape()
function. This function takes a tuple as input, indicating the desired shape of the array.
import numpy as np Create a 1D array my_array = np.array([1, 2, 3, 4, 5]) Reshape the array into a 2D array with 2 rows and 3 columns my_reshaped_array = my_array.reshape((2, 3))
Transposing Arrays
Arrays can be transposed using the transpose()
method or the T
attribute. Transposing an array swaps its rows and columns.
import numpy as np # Create a 2D array my_array = np.array([[1, 2, 3], [4, 5, 6]]) # Transpose the array my_transposed_array = my_array.transpose()
Related Article: How to Access Python Data Structures with Square Brackets
Flattening Arrays
Arrays can be flattened into a 1D array using the flatten()
method. This method returns a copy of the array in 1D form.
import numpy as np # Create a 2D array my_array = np.array([[1, 2, 3], [4, 5, 6]]) # Flatten the array into a 1D array my_flattened_array = my_array.flatten()
Concatenating Arrays
Arrays can be concatenated along different axes using the concatenate()
function. This function takes a tuple of arrays as input, along with the axis to concatenate along.
import numpy as np Create two 2D arrays array1 = np.array([[1, 2, 3], [4, 5, 6]]) array2 = np.array([[7, 8, 9], [10, 11, 12]]) Concatenate the arrays along the rows concatenated_array = np.concatenate((array1, array2), axis=0)
Thinking Outside the List and Array Boundaries
We will explore some techniques for working with data structures beyond Python lists and NumPy arrays.
Related Article: Implementation of Data Structures in Python
Dictionaries
Dictionaries are a built-in data structure in Python that allow you to store key-value pairs. You can use dictionaries to store and retrieve data in a more flexible way than with lists or arrays.
Create a dictionary my_dict = {'key1': 'value1', 'key2': 'value2', 'key3': 'value3'} Access a value using a key my_value = my_dict['key2']
Sets
Sets are another built-in data structure in Python that allow you to store unique values. You can use sets to perform operations such as intersection, union, and difference on your data.
# Create a set my_set = {1, 2, 3, 4, 5} # Add a value to the set my_set.add(6) # Perform a set operation other_set = {4, 5, 6, 7, 8} intersection = my_set.intersection(other_set)
Pandas DataFrames
Pandas is a powerful Python library for data manipulation and analysis. One of the key features of Pandas is the DataFrame object, which allows you to work with tabular data in a more flexible way than with lists or arrays.
import pandas as pd # Create a DataFrame from a dictionary my_dict = {'name': ['Alice', 'Bob', 'Charlie'], 'age': [25, 30, 35]} my_dataframe = pd.DataFrame(my_dict) # Access a column of data ages = my_dataframe['age'] # Perform a calculation on a column of data mean_age = my_dataframe['age'].mean()