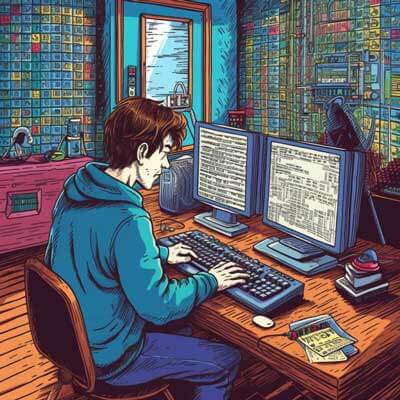
Cross-Site Scripting (XSS)
Cross-Site Scripting (XSS) is a common security vulnerability that allows attackers to inject malicious scripts into web pages viewed by other users. This can lead to various attacks, such as stealing sensitive information or performing actions on behalf of the victim.
In Django, you can prevent XSS attacks by using the built-in template engine’s auto-escaping feature. This feature automatically escapes special characters in user-generated content before rendering it in the browser. To enable auto-escaping, you need to ensure that the django.middleware.security.SecurityMiddleware
middleware is enabled in your Django settings.
Here’s an example of how to enable XSS protection in Django templates:
{% autoescape on %} {{ user_input }} {% endautoescape %}
In this example, the user_input
variable will be automatically escaped to prevent any potential XSS attacks.
Additionally, you should also validate and sanitize user input before storing or displaying it. Django provides various utility functions and libraries, such as django.utils.html.escape
and bleach
, which can help in sanitizing user input and preventing XSS attacks.
Related Article: 16 Amazing Python Libraries You Can Use Now
Cross-Site Request Forgery (CSRF)
Cross-Site Request Forgery (CSRF) is another common web application vulnerability where an attacker tricks a user into performing unintended actions on a website without their consent. Django provides built-in protection against CSRF attacks through the use of CSRF tokens.
To protect against CSRF attacks in Django, you need to ensure that the django.middleware.csrf.CsrfViewMiddleware
middleware is enabled in your Django settings. This middleware adds a CSRF token to every form rendered by Django and validates the token on form submissions.
Here’s an example of how to use CSRF protection in Django templates:
{% csrf_token %} <!-- form fields -->
In this example, the {% csrf_token %}
template tag generates a hidden input field with the CSRF token. When the form is submitted, Django will validate the token to ensure that the request is legitimate.
It’s important to note that CSRF protection is automatically applied to all POST, PUT, PATCH, and DELETE requests in Django. However, for AJAX requests, you need to include the CSRF token in the request headers or as a parameter in the request payload.
Password Hashing
Password hashing is a crucial aspect of security when it comes to user authentication. Storing passwords in plain text is a major security risk, as it allows attackers to easily obtain user passwords if the database is compromised.
In Django, password hashing is handled automatically by the authentication system. When a user registers or changes their password, Django uses a strong cryptographic hash function, such as PBKDF2 or bcrypt, to hash the password. This ensures that even if the database is compromised, the passwords remain secure.
Here’s an example of how to hash passwords in Django:
from django.contrib.auth.hashers import make_password password = 'my_password' hashed_password = make_password(password)
In this example, the make_password
function takes the plain text password as input and returns the hashed password.
When authenticating users, Django automatically compares the provided password with the stored hashed password. You don’t need to manually handle the password hashing during authentication.
It’s important to use a strong hashing algorithm and set a sufficiently high iteration count to make password cracking more difficult. Django’s default settings already provide secure defaults for password hashing, but you can customize them if needed.
Two-Factor Authentication
Two-Factor Authentication (2FA) adds an extra layer of security to user authentication by requiring users to provide a second factor in addition to their password. This second factor can be something they have (e.g., a mobile device) or something they are (e.g., biometric data).
Django does not provide built-in support for 2FA, but there are several third-party libraries available that can be used to implement 2FA in Django applications. One popular library is django-two-factor-auth, which provides support for various 2FA methods, such as SMS, email, TOTP, and more.
Here’s an example of how to implement 2FA using django-two-factor-auth:
1. Install the library:
pip install django-two-factor-auth
2. Add the library to your Django project’s INSTALLED_APPS
:
INSTALLED_APPS = [ ... 'two_factor', ... ]
3. Configure the authentication backend in your Django settings:
AUTHENTICATION_BACKENDS = ( 'django.contrib.auth.backends.ModelBackend', 'two_factor.auth_backends.OTPBackend', )
4. Include the necessary URLs in your project’s URL configuration:
urlpatterns = [ ... path('two-factor/', include('two_factor.urls', 'two_factor')), ... ]
5. Migrate the database to create the necessary tables:
python manage.py migrate
6. Customize the 2FA settings and templates as needed.
Related Article: 19 Python Code Snippets for Everyday Issues
Rate Limiting
Rate limiting is a technique used to prevent abuse and attacks by limiting the number of requests that can be made within a certain time period. This helps protect your application from brute force attacks, denial of service (DoS) attacks, and other malicious activities.
In Django, you can implement rate limiting using third-party libraries such as Django Ratelimit or Django Redis Ratelimit. These libraries provide decorators and middleware that allow you to easily apply rate limiting to specific views or API endpoints.
Here’s an example of how to implement rate limiting using Django Redis Ratelimit:
1. Install the library:
pip install django-redis-ratelimit
2. Add the library to your Django project’s INSTALLED_APPS
:
INSTALLED_APPS = [ ... 'ratelimit', ... ]
3. Configure the cache backend to use Redis in your Django settings:
CACHES = { 'default': { 'BACKEND': 'django_redis.cache.RedisCache', 'LOCATION': 'redis://localhost:6379/0', 'OPTIONS': { 'CLIENT_CLASS': 'django_redis.client.DefaultClient', } } }
4. Apply rate limiting to a view or API endpoint using the ratelimit_key
and ratelimit_rate
decorators:
from django_ratelimit.decorators import ratelimit_key, ratelimit_rate @ratelimit_key('user', rate='5/m') @ratelimit_rate('5/m') def my_view(request): # View logic here
In this example, the ratelimit_key
decorator specifies that the rate limiting should be applied based on the user’s IP address. The ratelimit_rate
decorator sets the rate limit to 5 requests per minute.
Content Security Policy (CSP)
Content Security Policy (CSP) is a security mechanism that allows web developers to control and restrict the types of content that can be loaded and executed on a web page. It helps protect against various types of attacks, such as XSS and data injection.
In Django, you can implement CSP by configuring the django-csp
library. This library provides a middleware that adds the necessary CSP headers to HTTP responses.
Here’s an example of how to implement CSP using the django-csp
library:
1. Install the library:
pip install django-csp
2. Add the library to your Django project’s INSTALLED_APPS
:
INSTALLED_APPS = [ ... 'csp', ... ]
3. Configure the CSP middleware in your Django settings:
MIDDLEWARE = [ ... 'csp.middleware.CSPMiddleware', ... ] CSP_DEFAULT_SRC = ("'self'",) CSP_SCRIPT_SRC = ("'self'", "https://cdn.example.com") CSP_STYLE_SRC = ("'self'", "https://cdn.example.com")
In this example, the CSP_DEFAULT_SRC
setting specifies that content should only be loaded from the same origin. The CSP_SCRIPT_SRC
and CSP_STYLE_SRC
settings allow loading scripts and stylesheets from both the same origin and a CDN.
HTTPS
Using HTTPS (HTTP over SSL/TLS) is essential for secure communication between a web server and a client. It encrypts the data exchanged between the server and the client, preventing eavesdropping and tampering.
In Django, enabling HTTPS involves configuring your web server (e.g., Nginx or Apache) to use SSL/TLS certificates and redirecting HTTP requests to the HTTPS version of your site. Django itself does not handle the encryption or certificate management.
Here’s an example of how to enable HTTPS in Nginx:
1. Obtain an SSL/TLS certificate from a trusted certificate authority (CA).
2. Configure Nginx to use the SSL/TLS certificate:
server { listen 443 ssl; server_name example.com; ssl_certificate /path/to/certificate.crt; ssl_certificate_key /path/to/private.key; # Other server configuration }
3. Redirect HTTP requests to HTTPS:
server { listen 80; server_name example.com; return 301 https://$host$request_uri; }
In this example, the first server block listens on port 443 (the default HTTPS port) and specifies the SSL/TLS certificate and private key paths. The second server block listens on port 80 (the default HTTP port) and redirects all requests to the HTTPS version of the site.
Related Article: A Guide to Python heapq and Heap in Python
SQL Injection
SQL Injection is a severe security vulnerability that occurs when an attacker is able to manipulate an SQL query to execute unintended commands or access unauthorized data. Django provides built-in protection against SQL Injection by using parameterized queries and prepared statements.
Here’s an example of how to prevent SQL Injection in Django:
from django.db import connection def get_user(username): with connection.cursor() as cursor: cursor.execute("SELECT * FROM users WHERE username = %s", [username]) row = cursor.fetchone() return row
In this example, the SQL query uses a parameterized query with the placeholder %s
and passes the username
value as a parameter. Django automatically escapes the parameter value, preventing any SQL injection attempts.
It’s important to always use parameterized queries or the ORM’s query building methods (e.g., filter
, exclude
, get
) when interacting with the database to ensure protection against SQL Injection.
Authentication Backend
Django provides a flexible authentication system that allows you to customize the authentication process by creating your own authentication backends. An authentication backend is responsible for verifying user credentials and retrieving user information during the authentication process.
Here’s an example of how to implement a custom authentication backend in Django:
from django.contrib.auth.backends import BaseBackend from django.contrib.auth import get_user_model class CustomBackend(BaseBackend): def authenticate(self, request, username=None, password=None, **kwargs): User = get_user_model() try: user = User.objects.get(username=username) if user.check_password(password): return user except User.DoesNotExist: return None def get_user(self, user_id): User = get_user_model() try: return User.objects.get(pk=user_id) except User.DoesNotExist: return None
In this example, the CustomBackend
class is a subclass of BaseBackend
and overrides the authenticate
and get_user
methods. The authenticate
method performs the authentication logic, checking the username and password against the database. The get_user
method retrieves the user object based on the user ID.
To use the custom authentication backend, you need to include it in the AUTHENTICATION_BACKENDS
setting in your Django project’s settings:
AUTHENTICATION_BACKENDS = [ 'myapp.backends.CustomBackend', ... ]
Brute Force Attacks
Brute force attacks are a common type of attack where an attacker tries all possible combinations of usernames and passwords to gain unauthorized access to a system. Django provides built-in protection against brute force attacks by implementing a combination of rate limiting, account lockouts, and password strength requirements.
To protect against brute force attacks in Django, you can configure various settings in your Django project’s settings:
# Maximum number of login attempts before an account is locked AUTHENTICATION_LOCKOUT_THRESHOLD = 5 # Duration of the account lockout in seconds AUTHENTICATION_LOCKOUT_DURATION = 300 # Minimum password length AUTH_PASSWORD_MIN_LENGTH = 8 # Password validation requirements AUTH_PASSWORD_VALIDATORS = [ { 'NAME': 'django.contrib.auth.password_validation.MinimumLengthValidator', 'OPTIONS': { 'min_length': 8, } }, ... ]
In this example, the AUTHENTICATION_LOCKOUT_THRESHOLD
setting specifies the maximum number of login attempts before an account is locked, and the AUTHENTICATION_LOCKOUT_DURATION
setting specifies the duration of the account lockout in seconds.
Additionally, the AUTH_PASSWORD_MIN_LENGTH
setting specifies the minimum password length required, and the AUTH_PASSWORD_VALIDATORS
setting defines a list of password validation requirements.
Related Article: Advance Django Forms: Dynamic Generation, Processing, and Custom Widgets
Additional Resources
– Django documentation – Cross Site Scripting (XSS) protection
– OWASP – Cross-Site Scripting (XSS) Prevention Cheat Sheet
– Django documentation – Cross Site Request Forgery (CSRF) protection