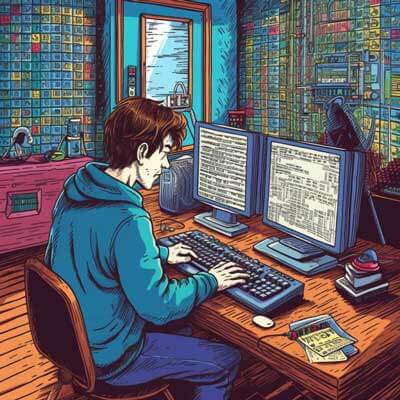
Python Data Types
Python is a dynamically typed programming language, which means that variables can hold values of different types. Python provides several built-in data types that can be used to represent different kinds of data. Some commonly used data types in Python include:
– Integer: This data type is used to represent whole numbers, both positive and negative. In Python, integers are represented using the “int” class. Here’s an example:
x = 5 print(x) # Output: 5
– Float: This data type is used to represent floating-point numbers, i.e., numbers with decimal points. In Python, floats are represented using the “float” class. Here’s an example:
y = 3.14 print(y) # Output: 3.14
– String: This data type is used to represent sequences of characters. In Python, strings are represented using the “str” class. Here’s an example:
name = "John Doe" print(name) # Output: John Doe
– List: This data type is used to represent ordered collections of items. In Python, lists are represented using the “list” class. Here’s an example:
numbers = [1, 2, 3, 4, 5] print(numbers) # Output: [1, 2, 3, 4, 5]
– Tuple: This data type is used to represent ordered collections of items, similar to lists. However, tuples are immutable, meaning their elements cannot be modified once defined. In Python, tuples are represented using the “tuple” class. Here’s an example:
coordinates = (10, 20) print(coordinates) # Output: (10, 20)
– Dictionary: This data type is used to represent key-value pairs. In Python, dictionaries are represented using the “dict” class. Here’s an example:
person = {"name": "John Doe", "age": 30} print(person) # Output: {'name': 'John Doe', 'age': 30}
These are just a few examples of the built-in data types available in Python. Understanding the different data types and how to work with them is essential for structuring data effectively in Python.
Related Article: String Comparison in Python: Best Practices and Techniques
Example 1: Working with Strings
# Define a string variable name = "Alice" # Print the length of the string print(len(name)) # Output: 5 # Access individual characters in the string print(name[0]) # Output: A print(name[2]) # Output: i # Concatenate two strings greeting = "Hello, " + name print(greeting) # Output: Hello, Alice
Example 2: Working with Lists
# Define a list of numbers numbers = [1, 2, 3, 4, 5] # Print the length of the list print(len(numbers)) # Output: 5 # Access individual elements in the list print(numbers[0]) # Output: 1 print(numbers[2]) # Output: 3 # Modify an element in the list numbers[1] = 10 print(numbers) # Output: [1, 10, 3, 4, 5] # Append an element to the list numbers.append(6) print(numbers) # Output: [1, 10, 3, 4, 5, 6]
These examples demonstrate basic operations with strings and lists, but Python provides many more functionalities and methods for working with different data types. It’s important to familiarize yourself with the available data types and their corresponding methods to effectively structure and manipulate data in Python.
Nested Data Structures in Python
In Python, nested data structures allow you to organize data in a hierarchical manner, where elements can contain other elements. This allows for more complex and structured representations of data. Some commonly used nested data structures in Python include:
– List of Lists: This is a simple form of a nested data structure, where a list contains other lists as its elements. Each inner list can have a different length. Here’s an example:
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] print(matrix) # Output: [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
– Dictionary of Dictionaries: This nested data structure uses dictionaries as its elements. Each key-value pair in the outer dictionary corresponds to an inner dictionary. Here’s an example:
students = { "Alice": {"age": 20, "grade": "A"}, "Bob": {"age": 18, "grade": "B"}, "Charlie": {"age": 19, "grade": "C"} } print(students) # Output: {'Alice': {'age': 20, 'grade': 'A'}, 'Bob': {'age': 18, 'grade': 'B'}, 'Charlie': {'age': 19, 'grade': 'C'}}
– List of Dictionaries: This nested data structure consists of a list that contains dictionaries as its elements. Each dictionary represents an entity or record, and the list represents a collection of such entities. Here’s an example:
employees = [ {"name": "Alice", "age": 30, "salary": 5000}, {"name": "Bob", "age": 25, "salary": 4000}, {"name": "Charlie", "age": 35, "salary": 6000} ] print(employees) # Output: [{'name': 'Alice', 'age': 30, 'salary': 5000}, {'name': 'Bob', 'age': 25, 'salary': 4000}, {'name': 'Charlie', 'age': 35, 'salary': 6000}]
Nested data structures can be used to represent more complex relationships between data elements. They allow for easy access and manipulation of data, making it easier to work with structured data in Python.
Related Article: How To Limit Floats To Two Decimal Points In Python
Example 1: Working with a List of Lists
# Define a list of lists matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] # Access individual elements in the matrix print(matrix[0]) # Output: [1, 2, 3] print(matrix[1][2]) # Output: 6 # Modify an element in the matrix matrix[2][1] = 10 print(matrix) # Output: [[1, 2, 3], [4, 5, 6], [7, 10, 9]]
Example 2: Working with a Dictionary of Dictionaries
# Define a dictionary of dictionaries students = { "Alice": {"age": 20, "grade": "A"}, "Bob": {"age": 18, "grade": "B"}, "Charlie": {"age": 19, "grade": "C"} } # Access individual elements in the dictionary print(students["Alice"]) # Output: {'age': 20, 'grade': 'A'} print(students["Bob"]["age"]) # Output: 18 # Modify an element in the dictionary students["Charlie"]["grade"] = "A" print(students) # Output: {'Alice': {'age': 20, 'grade': 'A'}, 'Bob': {'age': 18, 'grade': 'B'}, 'Charlie': {'age': 19, 'grade': 'A'}}
These examples demonstrate how nested data structures can be used to represent complex relationships between data elements. By nesting data structures, you can create more structured and organized representations of data in Python.
Data Modeling in Python
Data modeling is the process of designing the structure and relationships of data in a system. It involves identifying the entities, attributes, and relationships that need to be represented and deciding on the most appropriate data model to use. In Python, data modeling is an essential skill for effectively structuring and organizing data.
There are several approaches to data modeling in Python, depending on the requirements and nature of the data. Some common techniques include:
– Entity-Relationship Modeling: This approach involves identifying the entities (objects or concepts) in the system and their relationships. Entities are represented as objects or classes, and relationships are represented using attributes or methods. This approach is commonly used in object-oriented programming. Here’s an example:
class Student: def __init__(self, name, age, grade): self.name = name self.age = age self.grade = grade class Course: def __init__(self, name, students): self.name = name self.students = students # Create instances of the classes alice = Student("Alice", 20, "A") bob = Student("Bob", 18, "B") course = Course("Math", [alice, bob]) # Access attributes of the instances print(course.name) # Output: Math print(course.students[0].name) # Output: Alice
– Relational Modeling: This approach involves representing data using tables and defining relationships between the tables. Each table represents an entity, and the columns represent attributes of the entity. Relationships between entities are defined using foreign keys. This approach is commonly used in relational databases. Here’s an example using the SQLite database:
import sqlite3 # Connect to the database conn = sqlite3.connect("students.db") cursor = conn.cursor() # Create a table for students cursor.execute(""" CREATE TABLE students ( id INTEGER PRIMARY KEY, name TEXT, age INTEGER, grade TEXT ) """) # Insert data into the table cursor.execute(""" INSERT INTO students (name, age, grade) VALUES ("Alice", 20, "A") """) # Query data from the table cursor.execute("SELECT * FROM students") print(cursor.fetchall()) # Output: [(1, 'Alice', 20, 'A')] # Close the database connection conn.close()
These examples demonstrate different approaches to data modeling in Python. Depending on the nature of the data and the requirements of the system, you can choose the most appropriate data modeling technique to structure and represent data effectively.
Related Article: How To Rename A File With Python
Data Organization Techniques
Organizing data effectively is crucial for efficient data processing and analysis. In Python, there are several techniques and best practices for organizing data. Some commonly used techniques include:
– Using Data Structures: Python provides built-in data structures like lists, dictionaries, and sets that can be used to organize and store data. Choosing the right data structure depends on the nature of the data and the operations you need to perform on it. For example, lists are useful for storing ordered collections of items, while dictionaries are suitable for storing key-value pairs. Here’s an example:
# Using a list to store numbers numbers = [1, 2, 3, 4, 5] # Using a dictionary to store student information student = {"name": "Alice", "age": 20, "grade": "A"}
– Using Modules and Packages: Python allows you to organize your code into modules and packages, which help in organizing related data and functionalities. Modules are individual files containing Python code, while packages are directories containing multiple modules. This helps in modularizing your codebase and makes it easier to manage and reuse data and functionality. Here’s an example:
# Importing a module import math # Using a function from the math module radius = 5 area = math.pi * math.pow(radius, 2)
– Using Classes and Objects: Python is an object-oriented programming language, and classes and objects can be used to organize and encapsulate data and functionality. Classes define the structure and behavior of objects, while objects are instances of classes. This allows you to group related data and behaviors together. Here’s an example:
# Defining a class for a car class Car: def __init__(self, make, model, year): self.make = make self.model = model self.year = year # Creating an object of the Car class my_car = Car("Toyota", "Camry", 2021)
– Using Naming Conventions: Using meaningful and consistent naming conventions for variables, functions, classes, and modules helps in organizing and understanding the data and code. Choose descriptive names that accurately represent the purpose and content of the data. Here’s an example:
# Using descriptive variable names name = "Alice" age = 20 # Using descriptive function names def calculate_area(radius): return math.pi * math.pow(radius, 2)
Additional Resources
– Python Data Structures
– Python Dictionaries
– Python Sets