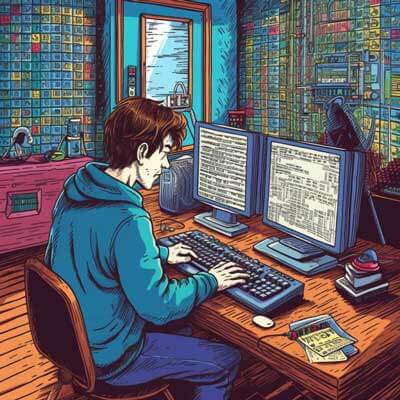
To read an Xlsx file using the Pandas library in Python, you can follow these steps:
Step 1: Install the Pandas library
Before you can start reading Xlsx files, you need to make sure that the Pandas library is installed. You can install it using pip by running the following command in your terminal or command prompt:
pip install pandas
Related Article: How To Read JSON From a File In Python
Step 2: Import the necessary libraries
Once you have installed the Pandas library, you need to import it along with any other libraries that you will be using. In this case, you will also need to import the pandas
library and the xlrd
library, which is required to read Xlsx files. Here’s an example of how to import these libraries:
import pandas as pd import xlrd
Step 3: Read the Xlsx file
To read the Xlsx file, you can use the pd.read_excel()
function provided by the Pandas library. This function takes the path to the Xlsx file as its first argument. Here’s an example of how to read an Xlsx file named “data.xlsx”:
data = pd.read_excel('data.xlsx')
data = pd.read_excel('data.xlsx', sheet_name='Sheet2')
Step 4: Explore the data
Once you have read the Xlsx file into a Pandas DataFrame, you can start exploring the data. You can use various DataFrame methods provided by Pandas to analyze and manipulate the data. For example, you can use the head()
method to view the first few rows of the DataFrame:
print(data.head())
You can also use the info()
method to get information about the DataFrame, such as the column names, data types, and non-null values:
print(data.info())
Related Article: How to Convert JSON to CSV in Python
Step 5: Handle missing data
If your Xlsx file contains missing data, Pandas provides several methods to handle it. For example, you can use the fillna()
method to fill the missing values with a specific value. Here’s an example:
data.fillna(0, inplace=True)
Alternatively, you can use the dropna()
method to remove rows or columns that contain missing values. Here’s an example:
data.dropna(inplace=True)
Step 6: Save the data to a new Xlsx file
If you want to save the modified data to a new Xlsx file, you can use the to_excel()
method provided by Pandas. This method takes the path to the output file as its first argument. Here’s an example:
data.to_excel('output.xlsx', index=False)
The index=False
parameter is used to exclude the index column from the output Xlsx file.
Step 7: Additional tips
– If you have multiple sheets in your Xlsx file and want to read all of them, you can pass None
or omit the sheet_name
parameter. This will return a dictionary of DataFrames, with each DataFrame corresponding to a sheet in the Xlsx file.
– If your Xlsx file contains large amounts of data, you can specify the number of rows to read using the nrows
parameter of the pd.read_excel()
function. For example, to read only the first 100 rows:
data = pd.read_excel('data.xlsx', nrows=100)
– If your Xlsx file contains multiple header rows, you can specify the row number of the header using the header
parameter of the pd.read_excel()
function. For example, to use the second row as the header:
data = pd.read_excel('data.xlsx', header=1)
– If your Xlsx file contains date columns, you can specify the date format using the parse_dates
parameter of the pd.read_excel()
function. For example, to parse the ‘date’ column as a datetime object:
data = pd.read_excel('data.xlsx', parse_dates=['date'])
– If your Xlsx file contains multiple data types in a column and you want to force a specific data type, you can use the dtype
parameter of the pd.read_excel()
function. For example, to force the ‘amount’ column to be of type float:
data = pd.read_excel('data.xlsx', dtype={'amount': float})
Related Article: How To Write Pandas Dataframe To CSV File
Alternative approach using openpyxl
Another way to read Xlsx files in Python is to use the openpyxl
library along with the Pandas library. openpyxl
is a useful library for reading and writing Xlsx files and provides more advanced features compared to the xlrd
library.
To use openpyxl
with Pandas, you need to install it using pip:
pip install openpyxl
Then, you can read the Xlsx file using the pd.read_excel()
function and specify the engine
parameter as ‘openpyxl’. Here’s an example:
data = pd.read_excel('data.xlsx', engine='openpyxl')
This approach is useful when you need to read Xlsx files that contain advanced features such as formulas, charts, or rich formatting.