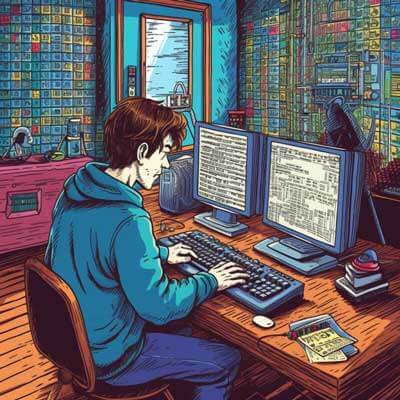
Table of Contents
To convert a string to bytes in Python 3, you can use the encode()
method or the bytes()
function. Both methods allow you to specify the encoding that you want to use.
Using the encode() method
The encode()
method is available on all string objects in Python. It returns a bytes object encoded with the specified encoding. Here's an example:
string = "Hello, World!" bytes_obj = string.encode('utf-8') print(bytes_obj)
This will output:
b'Hello, World!'
In this example, we used the 'utf-8'
encoding, which is a widely used encoding for Unicode text. You can replace 'utf-8'
with any other supported encoding, such as 'ascii'
, 'utf-16'
, or 'latin-1'
.
Related Article: Fixing 'Dataframe Constructor Not Properly Called' in Python
Using the bytes() function
The bytes()
function is another way to convert a string to bytes in Python. It takes two arguments: the string to convert and the encoding to use. Here's an example:
string = "Hello, World!" bytes_obj = bytes(string, 'utf-8') print(bytes_obj)
This will output the same result as the previous example:
b'Hello, World!'
The bytes()
function is particularly useful when you need to convert multiple strings to bytes and concatenate them. Here's an example:
string1 = "Hello" string2 = "World" bytes_obj = bytes(string1, 'utf-8') + bytes(string2, 'utf-8') print(bytes_obj)
This will output:
b'HelloWorld'
Best practices
Related Article: How to Implement Data Science and Data Engineering Projects with Python
When converting a string to bytes, it's important to choose the right encoding for your use case. The 'utf-8'
encoding is widely supported and can handle most Unicode characters. However, there may be cases where you need to use a different encoding, depending on the requirements of your application or the data you are dealing with.
It's also a good practice to handle encoding errors when converting strings to bytes. By default, the encode()
method and the bytes()
function will raise a UnicodeEncodeError
if the string contains characters that cannot be encoded with the specified encoding. You can catch this error and handle it gracefully in your code.
string = "Hello, 世界!" try: bytes_obj = string.encode('ascii') except UnicodeEncodeError: print("Error: Cannot encode string with ASCII encoding.")
This will output:
Error: Cannot encode string with ASCII encoding.
In this example, we tried to encode a string that contains non-ASCII characters with the 'ascii'
encoding, which only supports ASCII characters. The encode()
method raised a UnicodeEncodeError
, and we caught it to display an error message.
When working with binary data, such as files or network protocols, it's important to ensure that the encoding of the string matches the expected encoding. Using the wrong encoding can lead to data corruption or unexpected behavior.
In addition, it's worth noting that the encode()
method and the bytes()
function return immutable bytes objects. If you need a mutable version of the bytes object, you can use the bytearray()
function instead.
string = "Hello, World!" bytearray_obj = bytearray(string, 'utf-8') print(bytearray_obj)
This will output:
bytearray(b'Hello, World!')
The bytearray()
function works similarly to the bytes()
function, but it returns a mutable bytearray object instead of an immutable bytes object.