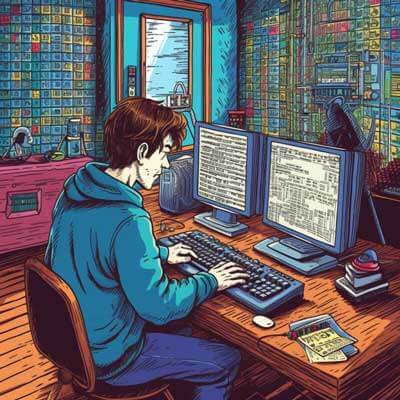
Table of Contents
Data structures are used to store and organize data in different formats. These data structures can be accessed and manipulated dynamically using square brackets. Dynamic access refers to the ability to access elements in a data structure based on specific conditions or variables.
To dynamically access a data structure in Python, you can use variables inside square brackets to indicate the index or key of the element you want to access. This allows you to retrieve or modify elements based on runtime conditions or user input.
Let's look at an example of dynamically accessing a list in Python:
fruits = ["apple", "banana", "orange", "kiwi"] index = 2 print(fruits[index]) # Output: orange index = 3 print(fruits[index]) # Output: kiwi
In this example, we have a list of fruits and we are using a variable index
inside square brackets to dynamically access elements in the list. The value of index
is changed at runtime, allowing us to access different elements based on the value of the variable.
Similarly, you can dynamically access elements in other data structures like dictionaries, sets, and tuples using square brackets and appropriate keys or indices.
Different Types of Data Structures
Python provides several built-in data structures that serve different purposes and have unique characteristics. These data structures include:
1. Lists: Lists are ordered collections of elements that can be of any type. They are mutable, meaning their elements can be modified. Lists are denoted by square brackets and elements are separated by commas.
2. Dictionaries: Dictionaries are unordered collections of key-value pairs. They are mutable and allow fast access to values based on unique keys. Dictionaries are denoted by curly braces and key-value pairs are separated by colons.
3. Sets: Sets are unordered collections of unique elements. They are mutable and support operations like union, intersection, and difference. Sets are denoted by curly braces and elements are separated by commas.
4. Tuples: Tuples are ordered collections of elements that can be of any type. They are immutable, meaning their elements cannot be modified. Tuples are denoted by parentheses and elements are separated by commas.
These data structures have different properties and are suited for different use cases. Understanding their characteristics and how to access their elements dynamically is essential for effective programming in Python.
Related Article: How To Check If Key Exists In Python Dictionary
Using Square Brackets to Access Elements in a Data Structure
Square brackets are used in Python to access elements in data structures like lists and dictionaries. The syntax for accessing elements with square brackets varies depending on the type of data structure.
Let's look at some examples of using square brackets to access elements in different data structures:
Indexing in Python
Indexing is the process of accessing elements in a sequence using their position or index. In Python, indexing starts from 0, meaning the first element of a sequence is at index 0, the second element is at index 1, and so on.
To access elements in a list or a tuple, you can use square brackets with the index of the element you want to access. Here's an example:
fruits = ["apple", "banana", "orange", "kiwi"] print(fruits[0]) # Output: apple print(fruits[2]) # Output: orange
In this example, we are using square brackets with the index of the element we want to access. The first print statement accesses the element at index 0, which is "apple", and the second print statement accesses the element at index 2, which is "orange".
It's important to note that trying to access an index that is out of range will result in an error. For example, if we try to access fruits[4]
, which is beyond the length of the list, we will get an IndexError
:
print(fruits[4]) # Output: IndexError: list index out of range
Accessing a Specific Element in a List Using Square Brackets
You can access a specific element in a list by using its index inside square brackets. This allows you to retrieve or modify individual elements in the list.
Here's an example of accessing a specific element in a list:
fruits = ["apple", "banana", "orange", "kiwi"] print(fruits[1]) # Output: banana fruits[2] = "grape" print(fruits) # Output: ["apple", "banana", "grape", "kiwi"]
In this example, we are accessing the element at index 1 in the list fruits
, which is "banana". We can also modify this element by assigning a new value to it. In the second print statement, we change the value at index 2 to "grape", resulting in the modified list ["apple", "banana", "grape", "kiwi"]
.
Related Article: How to Manipulate Strings in Python and Check for Substrings
Accessing a Value in a Dictionary Using a Key
Dictionaries store data in key-value pairs. To access a specific value in a dictionary, you can use the corresponding key inside square brackets.
Here's an example of accessing a value in a dictionary using a key:
student = {"name": "John", "age": 25, "grade": "A"} print(student["name"]) # Output: John print(student["age"]) # Output: 25
In this example, we have a dictionary student
with keys like "name", "age", and "grade". By using the respective key inside square brackets, we can access the corresponding value. The first print statement retrieves the value associated with the key "name", which is "John", and the second print statement retrieves the value associated with the key "age", which is 25.
It's important to note that if you try to access a key that does not exist in the dictionary, you will get a KeyError
:
print(student["address"]) # Output: KeyError: 'address'
Changing the Size of a Data Structure Dynamically
Some data structures like lists and dictionaries can be modified dynamically, meaning you can add or remove elements from them during runtime. This allows you to change the size of the data structure based on your needs.
Let's look at some examples of changing the size of a data structure dynamically in Python:
Modifying a List
Lists in Python are mutable, which means you can modify their elements or change their size during runtime. You can add elements to a list using the append()
method, remove elements using the remove()
method, or change the value of an element using its index.
Here's an example of modifying a list dynamically:
fruits = ["apple", "banana", "orange"] fruits.append("kiwi") print(fruits) # Output: ["apple", "banana", "orange", "kiwi"] fruits.remove("banana") print(fruits) # Output: ["apple", "orange"] fruits[0] = "mango" print(fruits) # Output: ["mango", "orange"]
In this example, we start with a list of fruits. We can add a new fruit "kiwi" to the list using the append()
method. We can also remove the fruit "banana" using the remove()
method. Finally, we can change the value of the first element to "mango" by assigning a new value to its index.
Modifying a Dictionary
Dictionaries are also mutable, allowing you to modify their keys or values dynamically. You can add new key-value pairs, remove existing key-value pairs, or change the value associated with a specific key.
Here's an example of modifying a dictionary dynamically:
student = {"name": "John", "age": 25} student["grade"] = "A" print(student) # Output: {"name": "John", "age": 25, "grade": "A"} del student["age"] print(student) # Output: {"name": "John"} student["name"] = "Mike" print(student) # Output: {"name": "Mike"}
In this example, we have a dictionary student
with keys like "name" and "age". We can add a new key-value pair "grade": "A" by assigning a value to the respective key. We can also remove the key-value pair "age": 25 using the del
keyword. Finally, we can change the value associated with the key "name" from "John" to "Mike" by assigning a new value to it.
Related Article: Python's Dict Tutorial: Is a Dictionary a Data Structure?
Difference Between a List and a Dictionary in Python
Lists and dictionaries are both common data structures in Python, but they have distinct characteristics and use cases.
Lists
Lists are ordered collections of elements that can be of any type. They are mutable, meaning you can modify their elements or change their size dynamically. Lists are denoted by square brackets and elements are separated by commas.
Some key points about lists:
- Lists are ordered, meaning the order of elements is preserved.
- Elements in a list can be accessed and modified using indices.
- Lists can contain duplicate elements.
- Lists are suitable for storing homogenous or heterogenous data.
- Lists are useful when the order of elements is important or when you need to perform operations like sorting, appending, or removing elements.
Dictionaries
Dictionaries are unordered collections of key-value pairs. They are mutable, allowing you to add, remove, or modify key-value pairs dynamically. Dictionaries are denoted by curly braces and key-value pairs are separated by colons.
Some key points about dictionaries:
- Dictionaries are unordered, meaning the order of key-value pairs is not preserved.
- Elements in a dictionary can be accessed and modified using keys.
- Keys in a dictionary must be unique.
- Dictionaries are suitable for storing data that needs to be accessed quickly based on unique keys.
- Dictionaries are useful when you need to associate values with specific keys or perform operations like searching or updating values based on keys.
Understanding the differences between lists and dictionaries is crucial for choosing the appropriate data structure for your specific use case. Consider factors like ordering, uniqueness, and the type of operations you need to perform on the data.
Using Variables Inside Square Brackets for Dynamic Access
Python allows you to use variables inside square brackets to dynamically access elements in data structures. This provides flexibility in accessing and manipulating data based on runtime conditions or user input.
Let's look at an example of using variables inside square brackets for dynamic access:
fruits = ["apple", "banana", "orange", "kiwi"] index = 2 print(fruits[index]) # Output: orange index = 3 print(fruits[index]) # Output: kiwi
In this example, we have a list of fruits, and we are using the variable index
inside square brackets to dynamically access elements in the list. By changing the value of index
, we can access different elements from the list.
Using variables inside square brackets allows you to write more flexible and reusable code, as you can change the accessed element based on runtime conditions or user input.
Related Article: How to Force Pip to Reinstall the Current Version in Python
Limitations of Using Square Brackets for Data Structure Access in Python
While using square brackets for data structure access in Python provides flexibility and convenience, there are some limitations to be aware of.
1. Index Out of Range: When accessing elements in a list or a tuple using square brackets, it's important to ensure that the index is within the valid range. Trying to access an index that is out of range will result in an IndexError
.
fruits = ["apple", "banana", "orange"] print(fruits[3]) # Output: IndexError: list index out of range
2. Key Error: When accessing values in a dictionary using square brackets, it's crucial to provide a valid key. Trying to access a key that does not exist in the dictionary will result in a KeyError
.
student = {"name": "John", "age": 25} print(student["address"]) # Output: KeyError: 'address'
3. Immutability of Tuples: While lists and dictionaries are mutable, meaning their elements can be modified, tuples are immutable. This means that once a tuple is created, its elements cannot be modified. Attempting to modify a tuple using square brackets will result in a TypeError
.
person = ("John", 25) person[1] = 30 # Output: TypeError: 'tuple' object does not support item assignment
4. Limited Sorting: Square brackets are not suitable for sorting elements in a data structure. If you need to sort a list, you should use the sort()
method or the sorted()
function.
numbers = [3, 1, 2] numbers.sort() print(numbers) # Output: [1, 2, 3]
Despite these limitations, using square brackets for data structure access in Python remains a useful and efficient way to manipulate and retrieve elements dynamically. Understanding these limitations and appropriate usage will help you write more effective and error-free code.