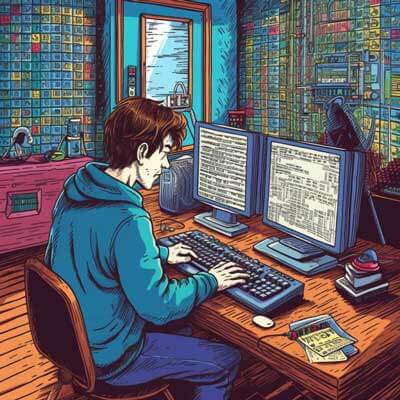
To detect duplicates in a Python list, you can use various approaches. Here are two possible methods:
Method 1: Using a Set
One simple way to detect duplicates in a Python list is to convert the list into a set and compare the lengths of the original list and the set. If the lengths are different, it means there are duplicates in the list.
Here’s an example:
my_list = [1, 2, 3, 4, 5, 5, 6, 7, 8, 9, 9] if len(my_list) != len(set(my_list)): print("Duplicates found!")
In this example, the original list my_list
contains duplicates (the numbers 5 and 9). By converting the list into a set using set(my_list)
, we eliminate the duplicates. If the lengths of the original list and the set are different, we know there are duplicates present.
Related Article: How To Convert a Dictionary To JSON In Python
Method 2: Using a Counter
Another approach is to use the Counter
class from the collections
module. The Counter
class provides a convenient way to count the occurrences of elements in a list.
Here’s an example:
from collections import Counter my_list = [1, 2, 3, 4, 5, 5, 6, 7, 8, 9, 9] counter = Counter(my_list) duplicates = [item for item, count in counter.items() if count > 1] if duplicates: print("Duplicates found:", duplicates)
In this example, we use the Counter
class to count the occurrences of each element in the list my_list
. We then filter the counter items to find the elements that have a count greater than 1, indicating duplicates. Finally, we check if the duplicates
list is not empty, and if so, print the duplicates.
Best Practices
Here are some best practices to consider when detecting duplicates in a Python list:
1. Use the most appropriate method based on your specific requirements. If you only need to know if duplicates exist, the set approach may be sufficient. If you need to access the actual duplicate elements, the Counter approach is more suitable.
2. Consider the time complexity of the chosen method. The set approach has a time complexity of O(n), where n is the length of the list, while the Counter approach has a time complexity of O(n + k), where n is the length of the list and k is the number of unique elements.
3. If the order of the duplicates is important, use the Counter approach, as it preserves the order of the elements.
4. If memory usage is a concern, the set approach may be more efficient, as it eliminates duplicates by converting the list into a set, which discards duplicate elements.
5. If you need to perform additional operations with the duplicates, such as removing or modifying them, consider using a different data structure, such as a dictionary or a custom class, to keep track of the duplicates and their occurrences.
These best practices can help you choose the most suitable approach and optimize the detection of duplicates in a Python list.
Related Article: How to Sort a Dictionary by Key in Python