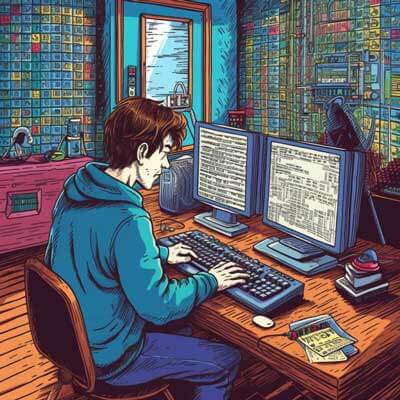
- Introduction to Numpy Where
- Syntax and Parameters of np.where
- Return Values of np.where
- Use Case: Filtering Data with np.where
- Best Practice: Efficient Usage of np.where
- Real World Example: Data Analysis with np.where
- Performance Consideration: Space Complexity of np.where
- Performance Consideration: Time Complexity of np.where
- Advanced Technique: Combining np.where with Other Numpy Functions
- Advanced Technique: Using np.where with Multi-dimensional Arrays
- Code Snippet: Basic Usage of np.where
- Code Snippet: Using np.where to Replace Values in an Array
- Code Snippet: Using np.where with Conditionals
- Code Snippet: Using np.where for Indexing
- Code Snippet: Using np.where with Scalar Inputs
- Error Handling: Common Errors and How to Avoid Them
Introduction to Numpy Where
In Python, the numpy.where()
function is a powerful tool that allows you to perform conditional operations on arrays. It provides a concise and efficient way to select elements from an array based on a specified condition.
The numpy.where()
function takes three parameters: condition
, x
, and y
. The condition
parameter is a boolean array that specifies the condition for selecting elements. The x
parameter is the value to be selected when the condition is True
, and the y
parameter is the value to be selected when the condition is False
.
Here is a basic example that demonstrates the usage of numpy.where()
:
import numpy as np arr = np.array([1, 2, 3, 4, 5]) condition = arr > 2 result = np.where(condition, arr, 0) print(result)
This code snippet creates an array arr
and defines a condition where the elements of arr
are greater than 2. The numpy.where()
function is then used to select the elements satisfying the condition, replacing the rest with zeros. The resulting array is printed, which will be [0 0 3 4 5]
.
Related Article: How To Exit Python Virtualenv
Syntax and Parameters of np.where
The syntax for the numpy.where()
function is as follows:
numpy.where(condition, x, y)
The parameters of the numpy.where()
function are:
condition
: A boolean array that specifies the condition for selecting elements.x
: The value to be selected when the condition isTrue
.y
: The value to be selected when the condition isFalse
.
It is important to note that x
and y
must have the same shape or be broadcastable to the same shape.
Here is an example that demonstrates the usage of different data types for x
and y
:
import numpy as np arr = np.array([1, 2, 3, 4, 5]) condition = arr > 2 result = np.where(condition, arr, np.array([0.1, 0.2, 0.3, 0.4, 0.5])) print(result)
This code snippet uses a different data type for y
by passing an array of floats. The resulting array will be [0.1 0.2 3. 4. 5.]
because the condition is satisfied for the elements greater than 2 and replaced with the corresponding elements from arr
, while the rest are replaced with the corresponding elements from the provided float array.
Return Values of np.where
The numpy.where()
function returns an array with the same shape as the input arrays x
and y
. The elements of the output array are selected based on the condition specified.
Here is an example that demonstrates the return values of numpy.where()
:
import numpy as np arr = np.array([1, 2, 3, 4, 5]) condition = arr > 2 result = np.where(condition, arr, 0) print("Result:", result) print("Type:", type(result)) print("Shape:", result.shape)
This code snippet prints the result, type, and shape of the output array. The output will be:
Result: [0 0 3 4 5] Type: Shape: (5,)
The result is an array of type numpy.ndarray
with a shape of (5,)
, which is the same as the input array arr
.
Use Case: Filtering Data with np.where
One common use case of numpy.where()
is filtering data based on a condition. You can easily select elements from an array that satisfy a specific condition and ignore the rest.
Here is an example that demonstrates how to filter data with numpy.where()
:
import numpy as np arr = np.array([1, 2, 3, 4, 5]) condition = arr % 2 == 0 result = np.where(condition, arr, 0) print(result)
This code snippet filters the elements of arr
by selecting only the even numbers and replacing the odd numbers with zeros. The resulting array will be [0 2 0 4 0]
.
Another example:
import numpy as np arr = np.array([10, 20, 30, 40, 50]) condition = arr > 25 result = np.where(condition, arr, -1) print(result)
This code snippet filters the elements of arr
by selecting only the numbers greater than 25 and replacing the rest with -1. The resulting array will be [10 20 30 40 50]
, as all the elements satisfy the condition.
Related Article: How to Integrate Python with MySQL for Database Queries
Best Practice: Efficient Usage of np.where
To use numpy.where()
efficiently, it is important to consider the performance implications of its usage. Here are some best practices to follow:
- Minimize the number of
numpy.where()
calls: Performing multiplenumpy.where()
calls can be computationally expensive. Whenever possible, try to combine conditions into a singlenumpy.where()
call. - Use boolean indexing instead of
numpy.where()
: In some cases, using boolean indexing can be more efficient than usingnumpy.where()
. Consider using boolean indexing if you only need to select elements based on a simple condition. - Avoid unnecessary array creation: Creating unnecessary arrays can consume memory and slow down the execution. Instead of creating new arrays, consider modifying the existing array in-place or using boolean indexing to select elements.
Here is an example that demonstrates the efficient usage of numpy.where()
:
import numpy as np arr = np.array([1, 2, 3, 4, 5]) condition1 = arr > 2 condition2 = arr % 2 == 0 result = np.where(np.logical_and(condition1, condition2), arr, 0) print(result)
This code snippet combines the conditions condition1
and condition2
using the numpy.logical_and()
function to select elements that are both greater than 2 and even. The resulting array will be [0 0 3 4 0]
.
Real World Example: Data Analysis with np.where
numpy.where()
is widely used in data analysis to perform various operations. One common application is data cleaning, where you can use numpy.where()
to replace missing or invalid values with appropriate values.
Here is an example that demonstrates how to use numpy.where()
for data analysis:
import numpy as np data = np.array([1, 2, -999, 4, 5]) condition = data == -999 data_cleaned = np.where(condition, np.nan, data) print(data_cleaned)
This code snippet replaces the invalid value -999
with NaN
(Not a Number) using numpy.where()
. The resulting array will be [1. 2. nan 4. 5.]
.
Another example:
import numpy as np data = np.array([-1, 2, 3, 4, -5]) condition = data < 0 data_cleaned = np.where(condition, np.abs(data), data) print(data_cleaned)
This code snippet replaces the negative values in the array data
with their absolute values using numpy.where()
. The resulting array will be [1 2 3 4 5]
.
Performance Consideration: Space Complexity of np.where
The numpy.where()
function does not introduce any additional space complexity compared to the input arrays. It only creates an output array with the same shape as the input arrays, which requires the same amount of memory.
Here is an example that demonstrates the space complexity of numpy.where()
:
import numpy as np arr = np.array([1, 2, 3, 4, 5]) condition = arr > 2 result = np.where(condition, arr, 0) print("Input array size:", arr.nbytes) print("Output array size:", result.nbytes)
This code snippet prints the size of the input and output arrays in bytes. The output will be:
Input array size: 40 Output array size: 40
Both the input and output arrays have the same size of 40 bytes, indicating that the space complexity of numpy.where()
is O(n), where n is the size of the input arrays.
Related Article: 16 Amazing Python Libraries You Can Use Now
Performance Consideration: Time Complexity of np.where
The time complexity of the numpy.where()
function depends on the size of the input arrays. In the worst case, it has a time complexity of O(n), where n is the size of the input arrays.
Here is an example that demonstrates the time complexity of numpy.where()
:
import numpy as np import time arr = np.random.randint(0, 100, 1000000) condition = arr > 50 start_time = time.time() result = np.where(condition, arr, 0) end_time = time.time() print("Time taken:", end_time - start_time)
This code snippet generates a large random array of size 1,000,000 and measures the time taken to execute the numpy.where()
function. The output will vary depending on the system, but it will give you an idea of the time complexity.
Advanced Technique: Combining np.where with Other Numpy Functions
One of the powerful features of numpy.where()
is its ability to be combined with other NumPy functions to perform complex operations on arrays.
Here is an example that demonstrates how to combine numpy.where()
with other NumPy functions:
import numpy as np arr = np.array([1, 2, 3, 4, 5]) condition = arr > 2 result = np.sqrt(np.where(condition, arr, 0)) print(result)
This code snippet applies the square root function numpy.sqrt()
to the elements of arr
that satisfy the condition arr > 2
. The resulting array will be [0. 0. 1.73205081 2. 2.23606798]
.
Another example:
import numpy as np arr1 = np.array([1, 2, 3, 4, 5]) arr2 = np.array([6, 7, 8, 9, 10]) condition = arr1 > 2 result = np.maximum(np.where(condition, arr1, 0), np.where(condition, arr2, 0)) print(result)
This code snippet combines two arrays arr1
and arr2
with the numpy.maximum()
function and numpy.where()
. It selects the maximum value between the corresponding elements of arr1
and arr2
when the condition arr1 > 2
is satisfied. The resulting array will be [0 0 3 4 5]
.
Advanced Technique: Using np.where with Multi-dimensional Arrays
numpy.where()
can also be used with multi-dimensional arrays, allowing you to perform element-wise operations across multiple dimensions.
Here is an example that demonstrates how to use numpy.where()
with multi-dimensional arrays:
import numpy as np arr = np.array([[1, 2], [3, 4], [5, 6]]) condition = arr > 2 result = np.where(condition, arr, -1) print(result)
This code snippet applies the condition arr > 2
to each element of the 2D array arr
. The elements that satisfy the condition are selected, while the rest are replaced with -1. The resulting array will be:
[[-1 -1] [ 3 4] [ 5 6]]
Another example:
import numpy as np arr = np.array([[1, 2], [3, 4], [5, 6]]) condition = arr % 2 == 0 result = np.where(condition, arr, np.array([0, 0])) print(result)
This code snippet selects the even elements from the 2D array arr
and replaces the rest with zeros. The resulting array will be:
[[0 2] [0 4] [0 6]]
Related Article: Database Query Optimization in Django: Boosting Performance for Your Web Apps
Code Snippet: Basic Usage of np.where
import numpy as np arr = np.array([1, 2, 3, 4, 5]) condition = arr > 2 result = np.where(condition, arr, 0) print(result)
This code snippet demonstrates the basic usage of numpy.where()
. It creates an array arr
and defines a condition where the elements of arr
are greater than 2. The numpy.where()
function is then used to select the elements satisfying the condition, replacing the rest with zeros. The resulting array is printed.
Code Snippet: Using np.where to Replace Values in an Array
import numpy as np arr = np.array([10, 20, 30, 40, 50]) condition = arr > 25 result = np.where(condition, arr, -1) print(result)
This code snippet demonstrates how to use numpy.where()
to replace values in an array. It creates an array arr
and defines a condition where the elements of arr
are greater than 25. The numpy.where()
function is then used to select the elements satisfying the condition, replacing the rest with -1. The resulting array is printed.
Code Snippet: Using np.where with Conditionals
import numpy as np arr = np.array([1, 2, 3, 4, 5]) condition = arr % 2 == 0 result = np.where(condition, arr, 0) print(result)
This code snippet demonstrates how to use numpy.where()
with conditionals. It creates an array arr
and defines a condition where the elements of arr
are even. The numpy.where()
function is then used to select the even elements, replacing the rest with zeros. The resulting array is printed.
Related Article: Converting Integer Scalar Arrays To Scalar Index In Python
Code Snippet: Using np.where for Indexing
import numpy as np arr = np.array([1, 2, 3, 4, 5]) condition = arr > 2 result = arr[np.where(condition)] print(result)
This code snippet demonstrates how to use numpy.where()
for indexing. It creates an array arr
and defines a condition where the elements of arr
are greater than 2. The numpy.where()
function is then used to select the indices where the condition is satisfied. The resulting array is printed.
Code Snippet: Using np.where with Scalar Inputs
import numpy as np arr = np.array([1, 2, 3, 4, 5]) condition = arr > 2 result = np.where(condition, 1, -1) print(result)
This code snippet demonstrates how to use numpy.where()
with scalar inputs. It creates an array arr
and defines a condition where the elements of arr
are greater than 2. The numpy.where()
function is then used to select the elements satisfying the condition, replacing them with 1, and the rest with -1. The resulting array is printed.
Error Handling: Common Errors and How to Avoid Them
When using numpy.where()
, there are some common errors that you may encounter. Here are a few examples and how to avoid them:
- TypeError: invalid type comparison: This error occurs when you try to compare arrays of different types. Make sure that the arrays you are comparing have the same data type or can be broadcasted to the same shape.
- ValueError: operands could not be broadcast together: This error occurs when the arrays you are passing to
numpy.where()
cannot be broadcasted to the same shape. Make sure that the arrays have compatible shapes or reshape them if necessary. - IndexError: index out of bounds: This error occurs when the indices you are using for indexing are out of bounds. Double-check your indices and make sure they are within the bounds of the array.
By being aware of these common errors and ensuring that your arrays have compatible shapes and data types, you can avoid most of the issues when using numpy.where()
.